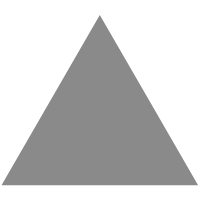
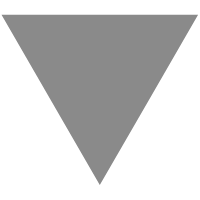
API with NestJS #143. Optimizing queries with views using PostgreSQL and Kysely
source link: https://wanago.io/2024/01/29/api-nestjs-postgresql-views-kysely/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
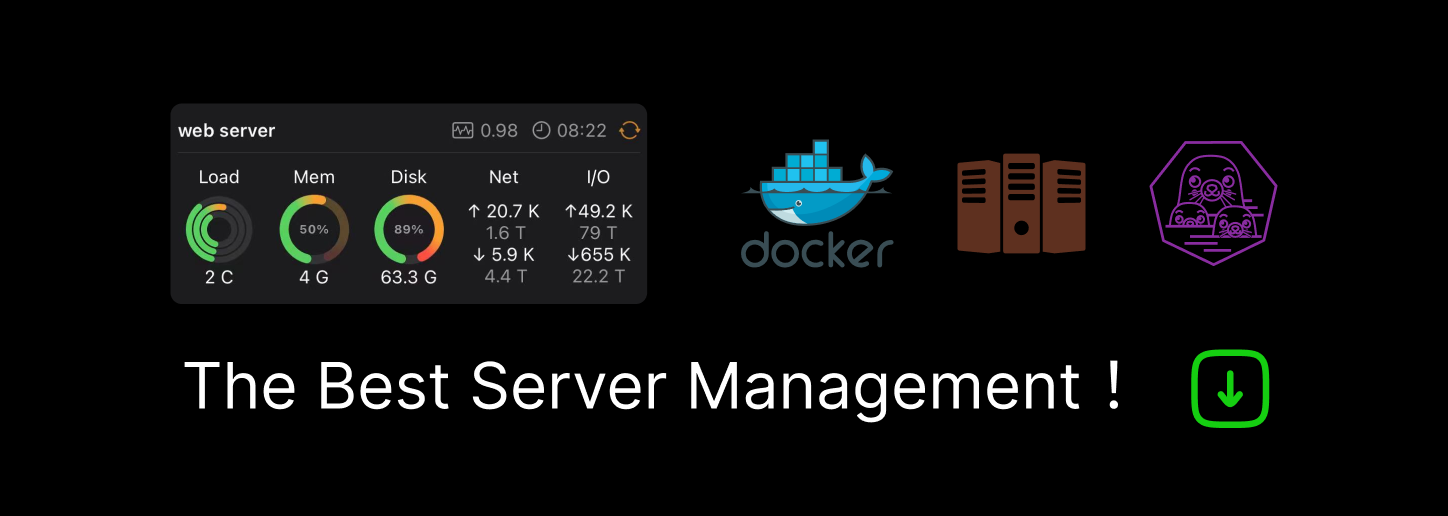

January 29, 2024
- 1. API with NestJS #1. Controllers, routing and the module structure
- 2. API with NestJS #2. Setting up a PostgreSQL database with TypeORM
- 3. API with NestJS #3. Authenticating users with bcrypt, Passport, JWT, and cookies
- 4. API with NestJS #4. Error handling and data validation
- 5. API with NestJS #5. Serializing the response with interceptors
- 6. API with NestJS #6. Looking into dependency injection and modules
- 7. API with NestJS #7. Creating relationships with Postgres and TypeORM
- 8. API with NestJS #8. Writing unit tests
- 9. API with NestJS #9. Testing services and controllers with integration tests
- 10. API with NestJS #10. Uploading public files to Amazon S3
- 11. API with NestJS #11. Managing private files with Amazon S3
- 12. API with NestJS #12. Introduction to Elasticsearch
- 13. API with NestJS #13. Implementing refresh tokens using JWT
- 14. API with NestJS #14. Improving performance of our Postgres database with indexes
- 15. API with NestJS #15. Defining transactions with PostgreSQL and TypeORM
- 16. API with NestJS #16. Using the array data type with PostgreSQL and TypeORM
- 17. API with NestJS #17. Offset and keyset pagination with PostgreSQL and TypeORM
- 18. API with NestJS #18. Exploring the idea of microservices
- 19. API with NestJS #19. Using RabbitMQ to communicate with microservices
- 20. API with NestJS #20. Communicating with microservices using the gRPC framework
- 21. API with NestJS #21. An introduction to CQRS
- 22. API with NestJS #22. Storing JSON with PostgreSQL and TypeORM
- 23. API with NestJS #23. Implementing in-memory cache to increase the performance
- 24. API with NestJS #24. Cache with Redis. Running the app in a Node.js cluster
- 25. API with NestJS #25. Sending scheduled emails with cron and Nodemailer
- 26. API with NestJS #26. Real-time chat with WebSockets
- 27. API with NestJS #27. Introduction to GraphQL. Queries, mutations, and authentication
- 28. API with NestJS #28. Dealing in the N + 1 problem in GraphQL
- 29. API with NestJS #29. Real-time updates with GraphQL subscriptions
- 30. API with NestJS #30. Scalar types in GraphQL
- 31. API with NestJS #31. Two-factor authentication
- 32. API with NestJS #32. Introduction to Prisma with PostgreSQL
- 33. API with NestJS #33. Managing PostgreSQL relationships with Prisma
- 34. API with NestJS #34. Handling CPU-intensive tasks with queues
- 35. API with NestJS #35. Using server-side sessions instead of JSON Web Tokens
- 36. API with NestJS #36. Introduction to Stripe with React
- 37. API with NestJS #37. Using Stripe to save credit cards for future use
- 38. API with NestJS #38. Setting up recurring payments via subscriptions with Stripe
- 39. API with NestJS #39. Reacting to Stripe events with webhooks
- 40. API with NestJS #40. Confirming the email address
- 41. API with NestJS #41. Verifying phone numbers and sending SMS messages with Twilio
- 42. API with NestJS #42. Authenticating users with Google
- 43. API with NestJS #43. Introduction to MongoDB
- 44. API with NestJS #44. Implementing relationships with MongoDB
- 45. API with NestJS #45. Virtual properties with MongoDB and Mongoose
- 46. API with NestJS #46. Managing transactions with MongoDB and Mongoose
- 47. API with NestJS #47. Implementing pagination with MongoDB and Mongoose
- 48. API with NestJS #48. Definining indexes with MongoDB and Mongoose
- 49. API with NestJS #49. Updating with PUT and PATCH with MongoDB and Mongoose
- 50. API with NestJS #50. Introduction to logging with the built-in logger and TypeORM
- 51. API with NestJS #51. Health checks with Terminus and Datadog
- 52. API with NestJS #52. Generating documentation with Compodoc and JSDoc
- 53. API with NestJS #53. Implementing soft deletes with PostgreSQL and TypeORM
- 54. API with NestJS #54. Storing files inside a PostgreSQL database
- 55. API with NestJS #55. Uploading files to the server
- 56. API with NestJS #56. Authorization with roles and claims
- 57. API with NestJS #57. Composing classes with the mixin pattern
- 58. API with NestJS #58. Using ETag to implement cache and save bandwidth
- 59. API with NestJS #59. Introduction to a monorepo with Lerna and Yarn workspaces
- 60. API with NestJS #60. The OpenAPI specification and Swagger
- 61. API with NestJS #61. Dealing with circular dependencies
- 62. API with NestJS #62. Introduction to MikroORM with PostgreSQL
- 63. API with NestJS #63. Relationships with PostgreSQL and MikroORM
- 64. API with NestJS #64. Transactions with PostgreSQL and MikroORM
- 65. API with NestJS #65. Implementing soft deletes using MikroORM and filters
- 66. API with NestJS #66. Improving PostgreSQL performance with indexes using MikroORM
- 67. API with NestJS #67. Migrating to TypeORM 0.3
- 68. API with NestJS #68. Interacting with the application through REPL
- 69. API with NestJS #69. Database migrations with TypeORM
- 70. API with NestJS #70. Defining dynamic modules
- 71. API with NestJS #71. Introduction to feature flags
- 72. API with NestJS #72. Working with PostgreSQL using raw SQL queries
- 73. API with NestJS #73. One-to-one relationships with raw SQL queries
- 74. API with NestJS #74. Designing many-to-one relationships using raw SQL queries
- 75. API with NestJS #75. Many-to-many relationships using raw SQL queries
- 76. API with NestJS #76. Working with transactions using raw SQL queries
- 77. API with NestJS #77. Offset and keyset pagination with raw SQL queries
- 78. API with NestJS #78. Generating statistics using aggregate functions in raw SQL
- 79. API with NestJS #79. Implementing searching with pattern matching and raw SQL
- 80. API with NestJS #80. Updating entities with PUT and PATCH using raw SQL queries
- 81. API with NestJS #81. Soft deletes with raw SQL queries
- 82. API with NestJS #82. Introduction to indexes with raw SQL queries
- 83. API with NestJS #83. Text search with tsvector and raw SQL
- 84. API with NestJS #84. Implementing filtering using subqueries with raw SQL
- 85. API with NestJS #85. Defining constraints with raw SQL
- 86. API with NestJS #86. Logging with the built-in logger when using raw SQL
- 87. API with NestJS #87. Writing unit tests in a project with raw SQL
- 88. API with NestJS #88. Testing a project with raw SQL using integration tests
- 89. API with NestJS #89. Replacing Express with Fastify
- 90. API with NestJS #90. Using various types of SQL joins
- 91. API with NestJS #91. Dockerizing a NestJS API with Docker Compose
- 92. API with NestJS #92. Increasing the developer experience with Docker Compose
- 93. API with NestJS #93. Deploying a NestJS app with Amazon ECS and RDS
- 94. API with NestJS #94. Deploying multiple instances on AWS with a load balancer
- 95. API with NestJS #95. CI/CD with Amazon ECS and GitHub Actions
- 96. API with NestJS #96. Running unit tests with CI/CD and GitHub Actions
- 97. API with NestJS #97. Introduction to managing logs with Amazon CloudWatch
- 98. API with NestJS #98. Health checks with Terminus and Amazon ECS
- 99. API with NestJS #99. Scaling the number of application instances with Amazon ECS
- 100. API with NestJS #100. The HTTPS protocol with Route 53 and AWS Certificate Manager
- 101. API with NestJS #101. Managing sensitive data using the AWS Secrets Manager
- 102. API with NestJS #102. Writing unit tests with Prisma
- 103. API with NestJS #103. Integration tests with Prisma
- 104. API with NestJS #104. Writing transactions with Prisma
- 105. API with NestJS #105. Implementing soft deletes with Prisma and middleware
- 106. API with NestJS #106. Improving performance through indexes with Prisma
- 107. API with NestJS #107. Offset and keyset pagination with Prisma
- 108. API with NestJS #108. Date and time with Prisma and PostgreSQL
- 109. API with NestJS #109. Arrays with PostgreSQL and Prisma
- 110. API with NestJS #110. Managing JSON data with PostgreSQL and Prisma
- 111. API with NestJS #111. Constraints with PostgreSQL and Prisma
- 112. API with NestJS #112. Serializing the response with Prisma
- 113. API with NestJS #113. Logging with Prisma
- 114. API with NestJS #114. Modifying data using PUT and PATCH methods with Prisma
- 115. API with NestJS #115. Database migrations with Prisma
- 116. API with NestJS #116. REST API versioning
- 117. API with NestJS #117. CORS – Cross-Origin Resource Sharing
- 118. API with NestJS #118. Uploading and streaming videos
- 119. API with NestJS #119. Type-safe SQL queries with Kysely and PostgreSQL
- 120. API with NestJS #120. One-to-one relationships with the Kysely query builder
- 121. API with NestJS #121. Many-to-one relationships with PostgreSQL and Kysely
- 122. API with NestJS #122. Many-to-many relationships with Kysely and PostgreSQL
- 123. API with NestJS #123. SQL transactions with Kysely
- 124. API with NestJS #124. Handling SQL constraints with Kysely
- 125. API with NestJS #125. Offset and keyset pagination with Kysely
- 126. API with NestJS #126. Improving the database performance with indexes and Kysely
- 127. API with NestJS #127. Arrays with PostgreSQL and Kysely
- 128. API with NestJS #128. Managing JSON data with PostgreSQL and Kysely
- 129. API with NestJS #129. Implementing soft deletes with SQL and Kysely
- 130. API with NestJS #130. Avoiding storing sensitive information in API logs
- 131. API with NestJS #131. Unit tests with PostgreSQL and Kysely
- 132. API with NestJS #132. Handling date and time in PostgreSQL with Kysely
- 133. API with NestJS #133. Introducing database normalization with PostgreSQL and Prisma
- 134. API with NestJS #134. Aggregating statistics with PostgreSQL and Prisma
- 135. API with NestJS #135. Referential actions and foreign keys in PostgreSQL with Prisma
- 136. API with NestJS #136. Raw SQL queries with Prisma and PostgreSQL range types
- 137. API with NestJS #137. Recursive relationships with Prisma and PostgreSQL
- 138. API with NestJS #138. Filtering records with Prisma
- 139. API with NestJS #139. Using UUID as primary keys with Prisma and PostgreSQL
- 140. API with NestJS #140. Using multiple PostgreSQL schemas with Prisma
- 141. API with NestJS #141. Getting distinct records with Prisma and PostgreSQL
- 142. API with NestJS #142. A video chat with WebRTC and React
- 143. API with NestJS #143. Optimizing queries with views using PostgreSQL and Kysely
Some of our SQL queries can become quite complicated. Fortunately, we can create views that act as aliases for the select queries. They have a form of virtual tables with rows and columns that we can select from but can’t insert any data into. We can also use materialized views that store the query results in the database, effectively creating a cached data version. In this article, we learn how to create and use views using PostgreSQL, NestJS, and Kysely.
If you want to know more about the basics of using NestJS with PostgreSQL and Kysely, check out API with NestJS #119. Type-safe SQL queries with Kysely and PostgreSQL
Creating views with Kysely
In the previous parts of this series, when working with Kysely, we’ve created a table for the articles.

Let’s take a closer look at the created_at column.
articlesTable.ts
import { ColumnType, Generated } from 'kysely'; export interface ArticlesTable { id: Generated<number>; title: string; paragraphs: string[]; author_id: number; scheduled_date?: ColumnType<Date, string | Date, string | Date>; created_at: ColumnType<Date, string | Date | undefined, string | Date>; |
Unfortunately, created_at: ColumnType<Date, string | Date | undefined, string | Date> looks intimidating, so let’s break it down. The ColumnType generic type accepts three arguments:
- Date is the data type we receive when selecting articles from the database,
- string | Date | undefined is the type we need to provide when creating a new article,
- string | Date is the data we need to use when updating an existing article.
If you want to know more about handling dates with Kysely, check out API with NestJS #132. Handling date and time in PostgreSQL with Kysely
The most important part above is string | Date | undefined. Providing the creation date when inserting a new article is optional because we set it up to default to the current date.
20231105201749_add_created_at_to_articles.ts
import { Kysely, sql } from 'kysely'; export async function up(database: Kysely<unknown>): Promise<void> { await database.schema .alterTable('articles') .addColumn('created_at', 'timestamptz', (column) => { return column.notNull().defaultTo(sql`now()`); .execute(); export async function down(database: Kysely<unknown>): Promise<void> { await database.schema.alterTable('articles').dropColumn('created_at'); |
Selecting articles from yesterday
To find all articles from yesterday, we can take advantage of the today and yesterday timestamps built into PostgreSQL.
SELECT * FROM articles WHERE articles.created_at < NOW() - INTERVAL '1 DAY' AND articles.created_at > NOW() - INTERVAL '2 DAYS' |
The most straightforward way of implementing this behavior with Kysely is to use the where function twice.
articles.repository.ts
import { Database } from '../database/database'; import { Article } from './article.model'; import { Injectable } from '@nestjs/common'; import { sql } from 'kysely'; @Injectable() export class ArticlesRepository { constructor(private readonly database: Database) {} async getArticlesFromYesterday() { const databaseResponse = await this.database .selectFrom('articles') .where('created_at', '<', sql`NOW() - INTERVAL '1 DAY'`) .where('created_at', '>', sql`NOW() - INTERVAL '2 DAYS'`) .selectAll() .execute(); return databaseResponse.map((articleData) => new Article(articleData)); // ... |
Creating the view
We can simplify the above code by creating the view.
20240127213806_add_articles_from_yesterday_view.ts
import { Migration, sql } from 'kysely'; export const up: Migration['up'] = async (database) => { await database.schema .createView('articles_from_yesterday') database .selectFrom('articles') .selectAll() .where('created_at', '<', sql`NOW() - INTERVAL '1 DAY'`) .where('created_at', '>', sql`NOW() - INTERVAL '2 DAYS'`), .execute(); export const down: Migration['down'] = async (database) => { await database.schema.dropView('articles_from_yesterday'); |
We also need to add articles_from_yesterday to our Tables interface.
database.ts
import { ArticlesTable } from '../articles/articlesTable'; import { Kysely } from 'kysely'; import { UsersTable } from '../users/usersTable'; import { AddressesTable } from '../users/addressesTable'; import { CategoriesTable } from '../categories/categoriesTable'; import { CategoriesArticlesTable } from '../categories/categoriesArticlesTable'; import { CommentsTable } from '../comments/commentsTable'; export interface Tables { articles: ArticlesTable; articles_from_yesterday: ArticlesTable; users: UsersTable; addresses: AddressesTable; categories: CategoriesTable; categories_articles: CategoriesArticlesTable; comments: CommentsTable; export class Database extends Kysely<Tables> {} |
We can now use the view in our queries.
articles.repository.ts
import { Database } from '../database/database'; import { Article } from './article.model'; import { Injectable } from '@nestjs/common'; @Injectable() export class ArticlesRepository { constructor(private readonly database: Database) {} async getArticlesFromYesterday() { const databaseResponse = await this.database .selectFrom('articles_from_yesterday') .selectAll() .execute(); return databaseResponse.map((articleData) => new Article(articleData)); |
Materialized views
It is crucial to realize that even though views resemble tables, they are not stored in our database. Let’s prove it using the EXPLAIN command that returns the execution plan.
EXPLAIN SELECT * FROM articles_from_yesterday; |

We can see that selecting the contents of the articles_from_yesterday view causes the database to query all articles and find the ones matching our filters. We can change this behavior by modifying our migration to create a materialized view.
20231105201749_add_created_at_to_articles.ts
import { Migration, sql } from 'kysely'; export const up: Migration['up'] = async (database) => { await database.schema .createView('articles_from_yesterday') database .selectFrom('articles') .selectAll() .where('created_at', '<', sql`NOW() - INTERVAL '1 DAY'`) .where('created_at', '>', sql`NOW() - INTERVAL '2 DAYS'`), .materialized() .execute(); export const down: Migration['down'] = async (database) => { await database.schema.dropView('articles_from_yesterday'); |
Thanks to calling the materialized() function, the articles_from_yesterday is materialized. This means that PostgreSQL stores a table containing the articles in the memory and does not need to filter them every time we select them.
EXPLAIN SELECT * FROM articles_from_yesterday |
It’s essential to notice that materialized views don’t update automatically. For it to update, we need to refresh it manually.
REFRESH MATERIALIZED VIEW articles_from_yesterday; |
Materialized views can be useful when we want to cache the results of some complex queries. For example, we could refresh the articles_from_yesterday every day at midnight to keep it up to date.
Temporary views
Views help simplify queries that are otherwise long and complex. This can be especially useful when writing raw SQL without the help of an ORM or a query builder such as Kysely. We should remember that PostgreSQL automatically stores all the view definitions we create. To remove these views, we can use the DROP VIEW command.
CREATE VIEW articles_from_yesterday AS SELECT * FROM articles WHERE articles.created_at < NOW() - INTERVAL '1 DAY' AND articles.created_at > NOW() - INTERVAL '2 DAYS' -- Perform operations on the articles_from_yesterday view DROP VIEW articles_from_yesterday; |
Thankfully, PostgreSQL offers a more straightforward solution. We can create temporary views with the TEMPORARY keyword, and PostgreSQL will automatically drop them at the end of the current session.
CREATE TEMPORARY VIEW articles_from_yesterday AS SELECT * FROM articles WHERE articles.created_at < NOW() - INTERVAL '1 DAY' AND articles.created_at > NOW() - INTERVAL '2 DAYS' -- Perform operations on the articles_from_yesterday view |
Summary
Views are helpful when we have complicated queries and need to make them easier to read. They can come in handy in various situations, for example, when changing old tables to new ones. For instance, if we’re getting rid of an old table and switching to a new one, we can use a view as a temporary replacement for the old table. Besides that, we can give access to views to the users who can’t access the tables directly. On the other hand, materialized views can come in handy for caching the data. It can be especially useful when dealing with large datasets or frequently accessed queries.
Thanks to all of the above, views and materialized views in PostgreSQL are valuable tools for simplifying complex queries, transitioning between table structures, and optimizing data access.
Series Navigation<< API with NestJS #142. A video chat with WebRTC and React
Recommend
-
5
-
2
API with NestJS #119. Type-safe SQL queries with Kysely and PostgreSQL We value your privacy
-
8
API with NestJS #120. One-to-one relationships with the Kysely query builder We value your privacy
-
8
API with NestJS #121. Many-to-one relationships with PostgreSQL and Kysely We value your privacy
-
7
-
2
-
13
API with NestJS #126. Improving the database performance with indexes and Kysely
-
8
-
6
The purpose of soft deletes The simplest way to add the soft delete feature is through a boolean flag.
-
13
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK