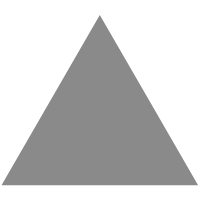
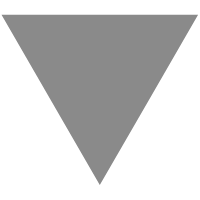
API with NestJS #126. Improving the database performance with indexes and Kysely
source link: https://wanago.io/2023/09/25/api-nestjs-kysely-sql-indexes/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
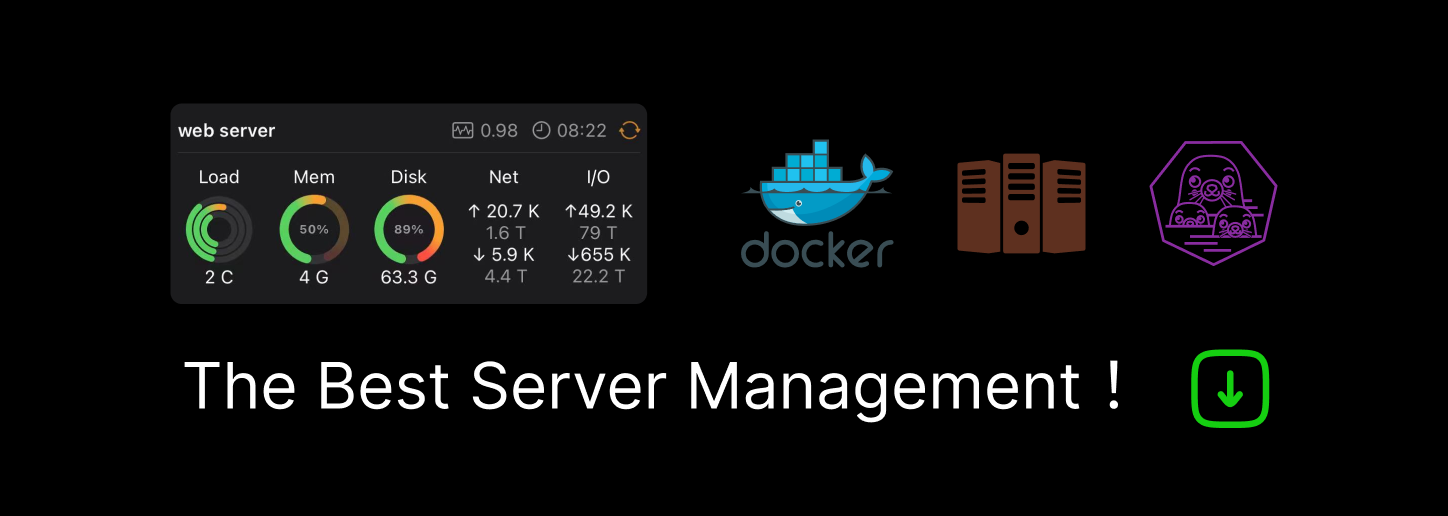
API with NestJS #126. Improving the database performance with indexes and Kysely
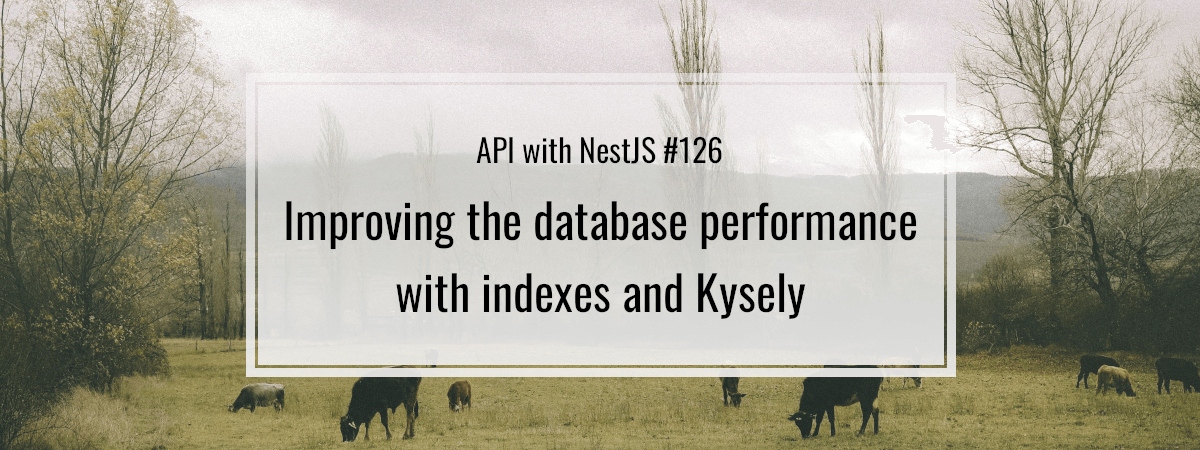
- 1. API with NestJS #1. Controllers, routing and the module structure
- 2. API with NestJS #2. Setting up a PostgreSQL database with TypeORM
- 3. API with NestJS #3. Authenticating users with bcrypt, Passport, JWT, and cookies
- 4. API with NestJS #4. Error handling and data validation
- 5. API with NestJS #5. Serializing the response with interceptors
- 6. API with NestJS #6. Looking into dependency injection and modules
- 7. API with NestJS #7. Creating relationships with Postgres and TypeORM
- 8. API with NestJS #8. Writing unit tests
- 9. API with NestJS #9. Testing services and controllers with integration tests
- 10. API with NestJS #10. Uploading public files to Amazon S3
- 11. API with NestJS #11. Managing private files with Amazon S3
- 12. API with NestJS #12. Introduction to Elasticsearch
- 13. API with NestJS #13. Implementing refresh tokens using JWT
- 14. API with NestJS #14. Improving performance of our Postgres database with indexes
- 15. API with NestJS #15. Defining transactions with PostgreSQL and TypeORM
- 16. API with NestJS #16. Using the array data type with PostgreSQL and TypeORM
- 17. API with NestJS #17. Offset and keyset pagination with PostgreSQL and TypeORM
- 18. API with NestJS #18. Exploring the idea of microservices
- 19. API with NestJS #19. Using RabbitMQ to communicate with microservices
- 20. API with NestJS #20. Communicating with microservices using the gRPC framework
- 21. API with NestJS #21. An introduction to CQRS
- 22. API with NestJS #22. Storing JSON with PostgreSQL and TypeORM
- 23. API with NestJS #23. Implementing in-memory cache to increase the performance
- 24. API with NestJS #24. Cache with Redis. Running the app in a Node.js cluster
- 25. API with NestJS #25. Sending scheduled emails with cron and Nodemailer
- 26. API with NestJS #26. Real-time chat with WebSockets
- 27. API with NestJS #27. Introduction to GraphQL. Queries, mutations, and authentication
- 28. API with NestJS #28. Dealing in the N + 1 problem in GraphQL
- 29. API with NestJS #29. Real-time updates with GraphQL subscriptions
- 30. API with NestJS #30. Scalar types in GraphQL
- 31. API with NestJS #31. Two-factor authentication
- 32. API with NestJS #32. Introduction to Prisma with PostgreSQL
- 33. API with NestJS #33. Managing PostgreSQL relationships with Prisma
- 34. API with NestJS #34. Handling CPU-intensive tasks with queues
- 35. API with NestJS #35. Using server-side sessions instead of JSON Web Tokens
- 36. API with NestJS #36. Introduction to Stripe with React
- 37. API with NestJS #37. Using Stripe to save credit cards for future use
- 38. API with NestJS #38. Setting up recurring payments via subscriptions with Stripe
- 39. API with NestJS #39. Reacting to Stripe events with webhooks
- 40. API with NestJS #40. Confirming the email address
- 41. API with NestJS #41. Verifying phone numbers and sending SMS messages with Twilio
- 42. API with NestJS #42. Authenticating users with Google
- 43. API with NestJS #43. Introduction to MongoDB
- 44. API with NestJS #44. Implementing relationships with MongoDB
- 45. API with NestJS #45. Virtual properties with MongoDB and Mongoose
- 46. API with NestJS #46. Managing transactions with MongoDB and Mongoose
- 47. API with NestJS #47. Implementing pagination with MongoDB and Mongoose
- 48. API with NestJS #48. Definining indexes with MongoDB and Mongoose
- 49. API with NestJS #49. Updating with PUT and PATCH with MongoDB and Mongoose
- 50. API with NestJS #50. Introduction to logging with the built-in logger and TypeORM
- 51. API with NestJS #51. Health checks with Terminus and Datadog
- 52. API with NestJS #52. Generating documentation with Compodoc and JSDoc
- 53. API with NestJS #53. Implementing soft deletes with PostgreSQL and TypeORM
- 54. API with NestJS #54. Storing files inside a PostgreSQL database
- 55. API with NestJS #55. Uploading files to the server
- 56. API with NestJS #56. Authorization with roles and claims
- 57. API with NestJS #57. Composing classes with the mixin pattern
- 58. API with NestJS #58. Using ETag to implement cache and save bandwidth
- 59. API with NestJS #59. Introduction to a monorepo with Lerna and Yarn workspaces
- 60. API with NestJS #60. The OpenAPI specification and Swagger
- 61. API with NestJS #61. Dealing with circular dependencies
- 62. API with NestJS #62. Introduction to MikroORM with PostgreSQL
- 63. API with NestJS #63. Relationships with PostgreSQL and MikroORM
- 64. API with NestJS #64. Transactions with PostgreSQL and MikroORM
- 65. API with NestJS #65. Implementing soft deletes using MikroORM and filters
- 66. API with NestJS #66. Improving PostgreSQL performance with indexes using MikroORM
- 67. API with NestJS #67. Migrating to TypeORM 0.3
- 68. API with NestJS #68. Interacting with the application through REPL
- 69. API with NestJS #69. Database migrations with TypeORM
- 70. API with NestJS #70. Defining dynamic modules
- 71. API with NestJS #71. Introduction to feature flags
- 72. API with NestJS #72. Working with PostgreSQL using raw SQL queries
- 73. API with NestJS #73. One-to-one relationships with raw SQL queries
- 74. API with NestJS #74. Designing many-to-one relationships using raw SQL queries
- 75. API with NestJS #75. Many-to-many relationships using raw SQL queries
- 76. API with NestJS #76. Working with transactions using raw SQL queries
- 77. API with NestJS #77. Offset and keyset pagination with raw SQL queries
- 78. API with NestJS #78. Generating statistics using aggregate functions in raw SQL
- 79. API with NestJS #79. Implementing searching with pattern matching and raw SQL
- 80. API with NestJS #80. Updating entities with PUT and PATCH using raw SQL queries
- 81. API with NestJS #81. Soft deletes with raw SQL queries
- 82. API with NestJS #82. Introduction to indexes with raw SQL queries
- 83. API with NestJS #83. Text search with tsvector and raw SQL
- 84. API with NestJS #84. Implementing filtering using subqueries with raw SQL
- 85. API with NestJS #85. Defining constraints with raw SQL
- 86. API with NestJS #86. Logging with the built-in logger when using raw SQL
- 87. API with NestJS #87. Writing unit tests in a project with raw SQL
- 88. API with NestJS #88. Testing a project with raw SQL using integration tests
- 89. API with NestJS #89. Replacing Express with Fastify
- 90. API with NestJS #90. Using various types of SQL joins
- 91. API with NestJS #91. Dockerizing a NestJS API with Docker Compose
- 92. API with NestJS #92. Increasing the developer experience with Docker Compose
- 93. API with NestJS #93. Deploying a NestJS app with Amazon ECS and RDS
- 94. API with NestJS #94. Deploying multiple instances on AWS with a load balancer
- 95. API with NestJS #95. CI/CD with Amazon ECS and GitHub Actions
- 96. API with NestJS #96. Running unit tests with CI/CD and GitHub Actions
- 97. API with NestJS #97. Introduction to managing logs with Amazon CloudWatch
- 98. API with NestJS #98. Health checks with Terminus and Amazon ECS
- 99. API with NestJS #99. Scaling the number of application instances with Amazon ECS
- 100. API with NestJS #100. The HTTPS protocol with Route 53 and AWS Certificate Manager
- 101. API with NestJS #101. Managing sensitive data using the AWS Secrets Manager
- 102. API with NestJS #102. Writing unit tests with Prisma
- 103. API with NestJS #103. Integration tests with Prisma
- 104. API with NestJS #104. Writing transactions with Prisma
- 105. API with NestJS #105. Implementing soft deletes with Prisma and middleware
- 106. API with NestJS #106. Improving performance through indexes with Prisma
- 107. API with NestJS #107. Offset and keyset pagination with Prisma
- 108. API with NestJS #108. Date and time with Prisma and PostgreSQL
- 109. API with NestJS #109. Arrays with PostgreSQL and Prisma
- 110. API with NestJS #110. Managing JSON data with PostgreSQL and Prisma
- 111. API with NestJS #111. Constraints with PostgreSQL and Prisma
- 112. API with NestJS #112. Serializing the response with Prisma
- 113. API with NestJS #113. Logging with Prisma
- 114. API with NestJS #114. Modifying data using PUT and PATCH methods with Prisma
- 115. API with NestJS #115. Database migrations with Prisma
- 116. API with NestJS #116. REST API versioning
- 117. API with NestJS #117. CORS – Cross-Origin Resource Sharing
- 118. API with NestJS #118. Uploading and streaming videos
- 119. API with NestJS #119. Type-safe SQL queries with Kysely and PostgreSQL
- 120. API with NestJS #120. One-to-one relationships with the Kysely query builder
- 121. API with NestJS #121. Many-to-one relationships with PostgreSQL and Kysely
- 122. API with NestJS #122. Many-to-many relationships with Kysely and PostgreSQL
- 123. API with NestJS #123. SQL transactions with Kysely
- 124. API with NestJS #124. Handling SQL constraints with Kysely
- 125. API with NestJS #125. Offset and keyset pagination with Kysely
- 126. API with NestJS #126. Improving the database performance with indexes and Kysely
- 127. API with NestJS #127. Arrays with PostgreSQL and Kysely
- 128. API with NestJS #128. Managing JSON data with PostgreSQL and Kysely
SQL indexes act like guides in our database and help us retrieve the data faster. The bigger our database is, the more emphasis we need to put on its performance. By using indexes, we can help our PostgreSQL database retrieve the data faster.
In this article, we learn what indexes are and how to create them with PostgreSQL, Kysely, and NestJS.
The idea behind indexes
Recently, we’ve added the articles table to our project.
articlesTable.ts
import { Generated } from 'kysely'; export interface ArticlesTable { id: Generated<number>; title: string; article_content: string; author_id: number; |
In our repository, we implemented a method to get a list of articles written by an author with a particular id.
articlesTable.ts
import { Database } from '../database/database'; import { Article } from './article.model'; import { Injectable } from '@nestjs/common'; @Injectable() export class ArticlesRepository { constructor(private readonly database: Database) {} async getByAuthorId( authorId: number, offset: number, limit: number | null, idsToSkip: number, const { data, count } = await this.database .transaction() .execute(async (transaction) => { let articlesQuery = transaction .selectFrom('articles') .where('author_id', '=', authorId) .where('id', '>', idsToSkip) .orderBy('id') .offset(offset) .selectAll(); if (limit !== null) { articlesQuery = articlesQuery.limit(limit); const articlesResponse = await articlesQuery.execute(); const { count } = await transaction .selectFrom('articles') .where('author_id', '=', authorId) .select((expressionBuilder) => { return expressionBuilder.fn.countAll().as('count'); .executeTakeFirstOrThrow(); return { data: articlesResponse, count, const items = data.map((articleData) => new Article(articleData)); return { items, count, // ... |
Above, we use pagination. If you want to learn more about it, check out API with NestJS #125. Offset and keyset pagination with Kysely
In the getByAuthorId method, we use where('author_id', '=', authorId). PostgreSQL needs to scan the entire articles table to find the matching records. Let’s visualize that using the EXPLAIN ANALYZE query.
EXPLAIN ANALYZE SELECT * FROM articles WHERE author_id = 1 |

In the query plan above, we can see that PostgreSQL does the sequential scan. While performing the sequential scan, the database reads all rows in the table one by one to find the data that matches the criteria. Sequential scans can be slow and resource-intensive, especially on large data sets. We can improve this situation by adding an index.
Introducing indexes
The SQL index acts similarly to a book’s index and helps the database find the information quickly. Let’s add an index on the author_id column to make the above query faster faster.
20230924200603_add_author_id_index.ts
import { Kysely } from 'kysely'; export async function up(database: Kysely<unknown>): Promise<void> { await database.schema .createIndex('article_author_id') .on('articles') .column('author_id') .execute(); export async function down(database: Kysely<unknown>): Promise<void> { await database.schema.dropIndex('article_author_id').execute(); |
Let’s run the above migration and try to analyze our SELECT query.
EXPLAIN ANALYZE SELECT * FROM articles WHERE author_id = 1 |

PostgreSQL considers quite a few factors when deciding whether to use an index. If it does not work for you, check out the VACUUM command.
The moment we create an index, PostgreSQL starts maintaining a data structure organized around a particular column. We can think of it as key and value pairs.
author_id | article_id |
---|---|
1 | 1 |
2 | 2 |
3 | 3 |
3 | 4 |
3 | 5 |
4 | 6 |
Under the hood, PostgreSQL uses a B-tree data structure where each leaf points to a particular row.
Now, PostgreSQL can quickly find all articles written by a particular author thanks to having a structure sorted by the author’s id. Unfortunately, indexes have some disadvantages, too.
Having to maintain an additional data structure takes extra space in our database. While it speeds up the queries that fetch data, maintaining indexes includes additional work for the database when inserting, updating, or deleting records from our database. Therefore, we must think our indexes through to avoid hurting the overall performance due to increased overhead.
Unique index
When working with Kysely, we’ve created the users table with the email column.
20230813165809_add_users_table.ts
import { Kysely } from 'kysely'; export async function up(database: Kysely<unknown>): Promise<void> { await database.schema .createTable('users') .addColumn('id', 'serial', (column) => { return column.primaryKey(); .addColumn('email', 'text', (column) => { return column.notNull().unique(); .addColumn('name', 'text', (column) => { return column.notNull(); .addColumn('password', 'text', (column) => { return column.notNull(); .execute(); export async function down(database: Kysely<unknown>): Promise<void> { await database.schema.dropTable('users').execute(); |
By marking the email column as unique, we tell PostgreSQL to look for email duplicates every time we insert or modify records in the articles table.
Since going through all elements in the table might be time-consuming, PostgreSQL creates indexes whenever we create a unique constraint. We can verify that with a simple SQL query.
SELECT tablename, indexname FROM pg_indexes WHERE tablename='articles'; |
Defining a primary key also creates a unique index. Because of that, the above screenshot also contains the articles_pkey index.
Thanks to that, the database can quickly search the existing users to determine if a particular email is unique. This can also benefit various SELECT queries and give them a performance boost.

Multi-column indexes
We can create queries that include multiple conditions. A good example is finding an article written by a particular user and containing a specific title.
const articles = await this.database .selectFrom('articles') .where('author_id', '=', 1) .where('title', '=', 'Hello world!') .selectAll() .execute(); |
If we create an index on the author_id or the title columns, we would speed up the above query. However, we can move it up a notch and create a multi-column index.
export async function up(database: Kysely<unknown>): Promise<void> { await database.schema .createIndex('article_author_id_title') .on('articles') .columns(['author_id', 'title']) .execute(); |
By creating a multi-column index, we can improve the performance of queries that use a specific combination of column values.
Index types
All of the indexes we mentioned so far used the B-tree structure. While it works fine for most cases, we also have other options.
Generalized Inverted Indexes (GIN)
The GIN indexes can help us when querying complex data types such as arrays or JSON. It might also come in handy when implementing text searching.
await database.schema .createIndex('article_title') .on('articles') .using('gin') .column('title') .execute(); |
To ensure that the GIN index is available in our database, we might need to enable the btree_gin and pg_trim extensions.
CREATE EXTENSION btree_gin; CREATE EXTENSION pg_trgm; |
Hash indexes
The hash SQL index uses hashes to locate specific values quickly. It might be a good fit for some use cases.
await database.schema .createIndex('article_title') .on('articles') .using('hash') .column('title') .execute(); |
Block Range Indexes (BRIN)
The Block Range Indexes (BRIN) are designed to handle very large tables.
await database.schema .createIndex('article_title') .on('articles') .using('brin') .column('title') .execute(); |
Generalized Search Tree (GIST)
The GIST indexes can be helpful for indexing geometric data and implementing text search. They might be preferable over GIN indexes in some cases.
await database.schema .createIndex('article_title') .on('articles') .using('gist') .column('title') .execute(); |
For them to work, we might need to enable the btree_gist extension.
CREATE EXTENSION btree_gist; |
Summary
In this article, we’ve covered the basic principles behind indexes and implemented examples that improved the performance of our SELECT queries. We also considered the disadvantages that come with an incorrect use of indexes.
The default type of index in PostgreSQL is a B-tree index. It works well for a wide range of queries and is the most commonly used index type. While that’s the case, we also mentioned other types of indexes, such as GIN and hash indexes.
Thanks to the above, we now know how to handle indexes when working with Kysely.
Series Navigation<< API with NestJS #125. Offset and keyset pagination with KyselyAPI with NestJS #127. Arrays with PostgreSQL and Kysely >>Use the right ORM to work with SQL databases
It’s easy to make a wrong choice when selecting the ORM library to use with Node.js and a SQL database.
Download a free PDF to help you choose the right tool for the job.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK