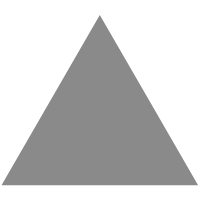
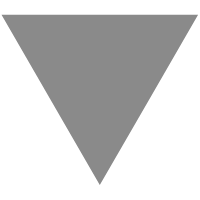
API with NestJS #136. Raw SQL queries with Prisma and PostgreSQL range types
source link: https://wanago.io/2023/12/04/api-nestjs-raw-sql-prisma-postgresql-range-types/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
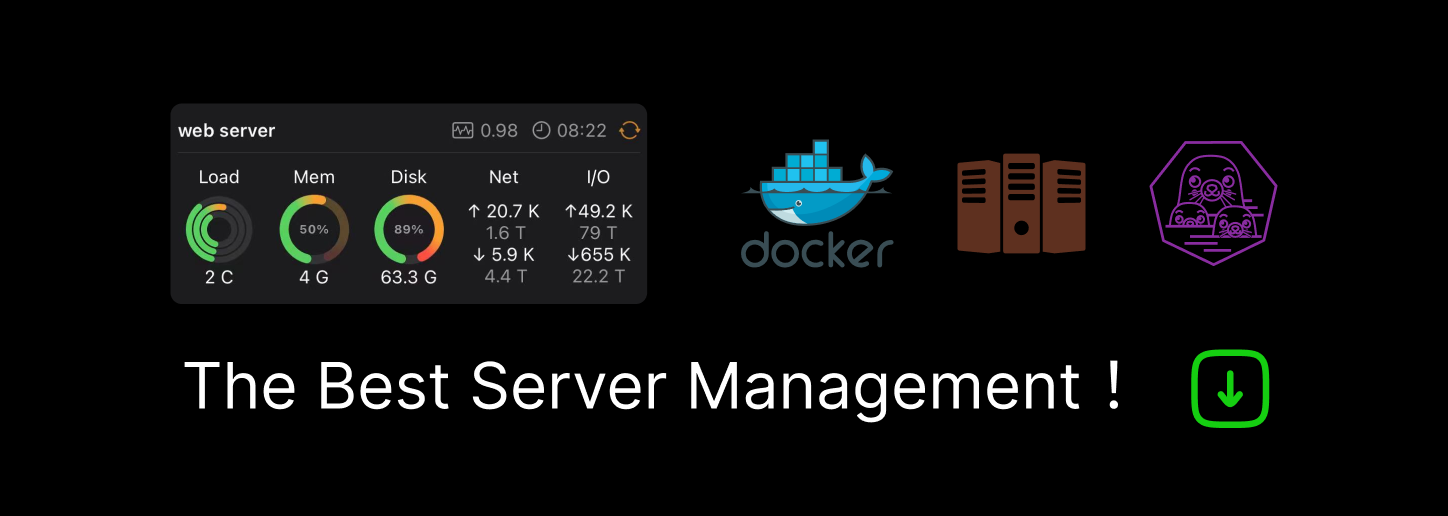

December 4, 2023
- 1. API with NestJS #1. Controllers, routing and the module structure
- 2. API with NestJS #2. Setting up a PostgreSQL database with TypeORM
- 3. API with NestJS #3. Authenticating users with bcrypt, Passport, JWT, and cookies
- 4. API with NestJS #4. Error handling and data validation
- 5. API with NestJS #5. Serializing the response with interceptors
- 6. API with NestJS #6. Looking into dependency injection and modules
- 7. API with NestJS #7. Creating relationships with Postgres and TypeORM
- 8. API with NestJS #8. Writing unit tests
- 9. API with NestJS #9. Testing services and controllers with integration tests
- 10. API with NestJS #10. Uploading public files to Amazon S3
- 11. API with NestJS #11. Managing private files with Amazon S3
- 12. API with NestJS #12. Introduction to Elasticsearch
- 13. API with NestJS #13. Implementing refresh tokens using JWT
- 14. API with NestJS #14. Improving performance of our Postgres database with indexes
- 15. API with NestJS #15. Defining transactions with PostgreSQL and TypeORM
- 16. API with NestJS #16. Using the array data type with PostgreSQL and TypeORM
- 17. API with NestJS #17. Offset and keyset pagination with PostgreSQL and TypeORM
- 18. API with NestJS #18. Exploring the idea of microservices
- 19. API with NestJS #19. Using RabbitMQ to communicate with microservices
- 20. API with NestJS #20. Communicating with microservices using the gRPC framework
- 21. API with NestJS #21. An introduction to CQRS
- 22. API with NestJS #22. Storing JSON with PostgreSQL and TypeORM
- 23. API with NestJS #23. Implementing in-memory cache to increase the performance
- 24. API with NestJS #24. Cache with Redis. Running the app in a Node.js cluster
- 25. API with NestJS #25. Sending scheduled emails with cron and Nodemailer
- 26. API with NestJS #26. Real-time chat with WebSockets
- 27. API with NestJS #27. Introduction to GraphQL. Queries, mutations, and authentication
- 28. API with NestJS #28. Dealing in the N + 1 problem in GraphQL
- 29. API with NestJS #29. Real-time updates with GraphQL subscriptions
- 30. API with NestJS #30. Scalar types in GraphQL
- 31. API with NestJS #31. Two-factor authentication
- 32. API with NestJS #32. Introduction to Prisma with PostgreSQL
- 33. API with NestJS #33. Managing PostgreSQL relationships with Prisma
- 34. API with NestJS #34. Handling CPU-intensive tasks with queues
- 35. API with NestJS #35. Using server-side sessions instead of JSON Web Tokens
- 36. API with NestJS #36. Introduction to Stripe with React
- 37. API with NestJS #37. Using Stripe to save credit cards for future use
- 38. API with NestJS #38. Setting up recurring payments via subscriptions with Stripe
- 39. API with NestJS #39. Reacting to Stripe events with webhooks
- 40. API with NestJS #40. Confirming the email address
- 41. API with NestJS #41. Verifying phone numbers and sending SMS messages with Twilio
- 42. API with NestJS #42. Authenticating users with Google
- 43. API with NestJS #43. Introduction to MongoDB
- 44. API with NestJS #44. Implementing relationships with MongoDB
- 45. API with NestJS #45. Virtual properties with MongoDB and Mongoose
- 46. API with NestJS #46. Managing transactions with MongoDB and Mongoose
- 47. API with NestJS #47. Implementing pagination with MongoDB and Mongoose
- 48. API with NestJS #48. Definining indexes with MongoDB and Mongoose
- 49. API with NestJS #49. Updating with PUT and PATCH with MongoDB and Mongoose
- 50. API with NestJS #50. Introduction to logging with the built-in logger and TypeORM
- 51. API with NestJS #51. Health checks with Terminus and Datadog
- 52. API with NestJS #52. Generating documentation with Compodoc and JSDoc
- 53. API with NestJS #53. Implementing soft deletes with PostgreSQL and TypeORM
- 54. API with NestJS #54. Storing files inside a PostgreSQL database
- 55. API with NestJS #55. Uploading files to the server
- 56. API with NestJS #56. Authorization with roles and claims
- 57. API with NestJS #57. Composing classes with the mixin pattern
- 58. API with NestJS #58. Using ETag to implement cache and save bandwidth
- 59. API with NestJS #59. Introduction to a monorepo with Lerna and Yarn workspaces
- 60. API with NestJS #60. The OpenAPI specification and Swagger
- 61. API with NestJS #61. Dealing with circular dependencies
- 62. API with NestJS #62. Introduction to MikroORM with PostgreSQL
- 63. API with NestJS #63. Relationships with PostgreSQL and MikroORM
- 64. API with NestJS #64. Transactions with PostgreSQL and MikroORM
- 65. API with NestJS #65. Implementing soft deletes using MikroORM and filters
- 66. API with NestJS #66. Improving PostgreSQL performance with indexes using MikroORM
- 67. API with NestJS #67. Migrating to TypeORM 0.3
- 68. API with NestJS #68. Interacting with the application through REPL
- 69. API with NestJS #69. Database migrations with TypeORM
- 70. API with NestJS #70. Defining dynamic modules
- 71. API with NestJS #71. Introduction to feature flags
- 72. API with NestJS #72. Working with PostgreSQL using raw SQL queries
- 73. API with NestJS #73. One-to-one relationships with raw SQL queries
- 74. API with NestJS #74. Designing many-to-one relationships using raw SQL queries
- 75. API with NestJS #75. Many-to-many relationships using raw SQL queries
- 76. API with NestJS #76. Working with transactions using raw SQL queries
- 77. API with NestJS #77. Offset and keyset pagination with raw SQL queries
- 78. API with NestJS #78. Generating statistics using aggregate functions in raw SQL
- 79. API with NestJS #79. Implementing searching with pattern matching and raw SQL
- 80. API with NestJS #80. Updating entities with PUT and PATCH using raw SQL queries
- 81. API with NestJS #81. Soft deletes with raw SQL queries
- 82. API with NestJS #82. Introduction to indexes with raw SQL queries
- 83. API with NestJS #83. Text search with tsvector and raw SQL
- 84. API with NestJS #84. Implementing filtering using subqueries with raw SQL
- 85. API with NestJS #85. Defining constraints with raw SQL
- 86. API with NestJS #86. Logging with the built-in logger when using raw SQL
- 87. API with NestJS #87. Writing unit tests in a project with raw SQL
- 88. API with NestJS #88. Testing a project with raw SQL using integration tests
- 89. API with NestJS #89. Replacing Express with Fastify
- 90. API with NestJS #90. Using various types of SQL joins
- 91. API with NestJS #91. Dockerizing a NestJS API with Docker Compose
- 92. API with NestJS #92. Increasing the developer experience with Docker Compose
- 93. API with NestJS #93. Deploying a NestJS app with Amazon ECS and RDS
- 94. API with NestJS #94. Deploying multiple instances on AWS with a load balancer
- 95. API with NestJS #95. CI/CD with Amazon ECS and GitHub Actions
- 96. API with NestJS #96. Running unit tests with CI/CD and GitHub Actions
- 97. API with NestJS #97. Introduction to managing logs with Amazon CloudWatch
- 98. API with NestJS #98. Health checks with Terminus and Amazon ECS
- 99. API with NestJS #99. Scaling the number of application instances with Amazon ECS
- 100. API with NestJS #100. The HTTPS protocol with Route 53 and AWS Certificate Manager
- 101. API with NestJS #101. Managing sensitive data using the AWS Secrets Manager
- 102. API with NestJS #102. Writing unit tests with Prisma
- 103. API with NestJS #103. Integration tests with Prisma
- 104. API with NestJS #104. Writing transactions with Prisma
- 105. API with NestJS #105. Implementing soft deletes with Prisma and middleware
- 106. API with NestJS #106. Improving performance through indexes with Prisma
- 107. API with NestJS #107. Offset and keyset pagination with Prisma
- 108. API with NestJS #108. Date and time with Prisma and PostgreSQL
- 109. API with NestJS #109. Arrays with PostgreSQL and Prisma
- 110. API with NestJS #110. Managing JSON data with PostgreSQL and Prisma
- 111. API with NestJS #111. Constraints with PostgreSQL and Prisma
- 112. API with NestJS #112. Serializing the response with Prisma
- 113. API with NestJS #113. Logging with Prisma
- 114. API with NestJS #114. Modifying data using PUT and PATCH methods with Prisma
- 115. API with NestJS #115. Database migrations with Prisma
- 116. API with NestJS #116. REST API versioning
- 117. API with NestJS #117. CORS – Cross-Origin Resource Sharing
- 118. API with NestJS #118. Uploading and streaming videos
- 119. API with NestJS #119. Type-safe SQL queries with Kysely and PostgreSQL
- 120. API with NestJS #120. One-to-one relationships with the Kysely query builder
- 121. API with NestJS #121. Many-to-one relationships with PostgreSQL and Kysely
- 122. API with NestJS #122. Many-to-many relationships with Kysely and PostgreSQL
- 123. API with NestJS #123. SQL transactions with Kysely
- 124. API with NestJS #124. Handling SQL constraints with Kysely
- 125. API with NestJS #125. Offset and keyset pagination with Kysely
- 126. API with NestJS #126. Improving the database performance with indexes and Kysely
- 127. API with NestJS #127. Arrays with PostgreSQL and Kysely
- 128. API with NestJS #128. Managing JSON data with PostgreSQL and Kysely
- 129. API with NestJS #129. Implementing soft deletes with SQL and Kysely
- 130. API with NestJS #130. Avoiding storing sensitive information in API logs
- 131. API with NestJS #131. Unit tests with PostgreSQL and Kysely
- 132. API with NestJS #132. Handling date and time in PostgreSQL with Kysely
- 133. API with NestJS #133. Introducing database normalization with PostgreSQL and Prisma
- 134. API with NestJS #134. Aggregating statistics with PostgreSQL and Prisma
- 135. API with NestJS #135. Referential actions and foreign keys in PostgreSQL with Prisma
- 136. API with NestJS #136. Raw SQL queries with Prisma and PostgreSQL range types
- 137. API with NestJS #137. Recursive relationships with Prisma and PostgreSQL
While Prisma gradually adds various features, PostgreSQL still has a lot of functionalities that Prisma does not support yet. One of them is range types. In this article, we learn how to use a column type not supported by Prisma and how to make raw SQL queries.
Range types
Sometimes, when working with our database, we might want to represent a range of values. For example, we might want to define a set of available dates. One way would be to create two columns that hold the bound values.
schema.prisma
model Event { id Int @id @default(autoincrement()) name String startDate DateTime @db.Timestamptz endDate DateTime @db.Timestamptz |
Above, we use the timestamp with timezone data type. If you want to know more, check out API with NestJS #108. Date and time with Prisma and PostgreSQL
Unfortunately, this approach does not ensure data integrity. Nothing stops the user from storing the end date that happens before the start date.
Thankfully, PostgreSQL has various built-in range types that can make working with ranges a lot more straightforward.
Creating the migration
For example, to represent a range of timestamps with timezones, we need the tstzrange type. Since Prisma does not support it, we must use the Unsupported type. It allows us to define fields in the schema for types that are not yet supported.
schema.prisma
model Event { id Int @id @default(autoincrement()) name String dateRange Unsupported("tstzrange") |
Let’s create a migration that adds the above table.
npx prisma migrate dev --name add_event_table |
If you would like to read more about database migrations with Prisma, check out API with NestJS #115. Database migrations with Prisma
migrate.sql
-- CreateTable CREATE TABLE "Event" ( "id" SERIAL NOT NULL, "name" TEXT NOT NULL, "dateRange" tstzrange NOT NULL, CONSTRAINT "Event_pkey" PRIMARY KEY ("id") |
As you can see, Prisma generated a correct migration despite not supporting the tstzrange column.
Defining a range
Let’s allow the user to create an event with a date range.
Ranges in PostgreSQL
Ranges in PostgreSQL have the lower bound and the upper bound. Every value in between is considered to be within the range.
INSERT INTO "Event"( name, "dateRange" VALUES ( 'Festival', '[2023-12-10 10:00, 2023-12-15 18:00)' RETURNING *; |
Notice that in the above code, we specify our range between the [ and ) characters.
The square brackets represent inclusive bounds. This means that the bound value is included in the range.
The round brackets represent exclusive bounds. Using them ensures that the bound value is not included in the range.
Considering that, we can see that our lower bound is inclusive, and our upper bound is exclusive.
Creating ranges with Prisma
Let’s require the user to provide the start and end dates separately in the request body. Let’s assume that all our event date ranges have inclusive bounds to keep the API straightforward.
create-event.dto.ts
import { IsString, IsNotEmpty, IsISO8601 } from 'class-validator'; export class CreateEventDto { @IsString() @IsNotEmpty() name: string; @IsISO8601({ strict: true, startDate: string; @IsISO8601({ strict: true, endDate: string; |
Fortunately, we don’t have to create the range manually using the startDate and endDate properties. Instead, we can use the postgres-range library.
npm install postgres-range |
A downside of the postgres-range library is that the Range constructor is quite peculiar and requires us to pass a single number representing which bounds are inclusive or exclusive. The easiest way to do that is to use the bitwise OR operator with the RANGE_LB_INC and RANGE_UB_INC constants that represent the inclusive lower bound and inclusive upper bound.
import { Range, RANGE_LB_INC, RANGE_UB_INC, serialize } from 'postgres-range'; const range = new Range( '2023-12-10 10:00', '2023-12-15 18:00', RANGE_LB_INC | RANGE_UB_INC, // '[2023-12-10 10:00, 2023-12-15 18:00)' console.log(serialize(range)); |
We stringify the range using the serialize function.
Since the tstzrange data is not supported by Prisma, we need to use the $queryRaw tagged template that allows us to make a raw SQL query. It returns an array of results, but in our case, this array should have only one element.
events.service.ts
import { Injectable, InternalServerErrorException } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; import { Range, RANGE_LB_INC, RANGE_UB_INC, serialize } from 'postgres-range'; import { CreateEventDto } from './dto/create-event.dto'; @Injectable() export class EventsService { constructor(private readonly prismaService: PrismaService) {} async create(eventData: CreateEventDto) { const range = new Range( eventData.startDate, eventData.endDate, RANGE_LB_INC | RANGE_UB_INC, const queryResponse = await this.prismaService.$queryRaw` INSERT INTO "Event"( name, "dateRange" VALUES ( ${eventData.name}, ${serialize(range)}::tstzrange RETURNING id, name, "dateRange"::text if (Array.isArray(queryResponse) && queryResponse.length === 1) { return queryResponse[0]; throw new InternalServerErrorException(); |
Prisma requires us to cast the serialized range using ::tstzrange. If we don’t do that, it throws an error.
Simiarly, we need to cast the data returned by the database back to a string using ::text.
A crucial thing about the $queryRaw is that it sends the SQL query to the database separately from the arguments, such as the eventData.name, using parametrized queries to prevent SQL injection vulnerabilities.
Fetching existing data from the database
We must also make a raw SQL query to fetch the events from the database.
events.service.ts
import { Injectable } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; @Injectable() export class EventsService { constructor(private readonly prismaService: PrismaService) {} getAll() { return this.prismaService.$queryRaw` SELECT id, name, "dateRange"::text FROM "Event" // ... |
Instead of sending the users the date ranges that are plain strings, let’s split it back into the start and end dates. To do that, we can use the class-transformer library combined with the postgres-range.
If you want to know more about response serialization, check out API with NestJS #112. Serializing the response with Prisma
event-response.dto.ts
import { Exclude, Expose, Transform } from 'class-transformer'; import { parse } from 'postgres-range'; export class EventResponseDto { name: string; @Exclude() dateRange: string; @Expose() @Transform(({ obj }) => { const parsedRange = parse(obj.dateRange); return parsedRange.lower; startDate: string; @Expose() @Transform(({ obj }) => { const parsedRange = parse(obj.dateRange); return parsedRange.upper; endDate: string; |
Above, we use the parse function to parse the dateRange string into an instance of the Range class. It contains the lower and upper properties that represent our lower and upper bounds.
The most straightforward way of creating instances of our EventResponseDto class is by using the @TransformPlainToInstance() decorator provided by the class-transformer library.
events.controller.ts
import { Body, Controller, Get, Post } from '@nestjs/common'; import { EventsService } from './events.service'; import { CreateEventDto } from './dto/create-event.dto'; import { EventResponseDto } from './dto/event-response.dto'; import { TransformPlainToInstance } from 'class-transformer'; @Controller('events') export class EventsController { constructor(private readonly eventsService: EventsService) {} @Get() @TransformPlainToInstance(EventResponseDto) getAll() { return this.eventsService.getAll(); @Post() @TransformPlainToInstance(EventResponseDto) create(@Body() event: CreateEventDto) { return this.eventsService.create(event); |

The benefits of the range columns
Above, the user provides the start and end dates separately, and we store them in a tstzrange column. While this adds quite a bit of work, it has its benefits. For example, PostgreSQL gives us various operators we can use with ranges.
One of the most important operators related to ranges is @>. With it, we can check if a range contains a particular value. We can use it to implement a search feature that returns all events happening on a particular date.
events.service.ts
import { Injectable } from '@nestjs/common'; import { PrismaService } from '../database/prisma.service'; @Injectable() export class EventsService { constructor(private readonly prismaService: PrismaService) {} search(date: string) { return this.prismaService.$queryRaw` SELECT id, name, "dateRange"::text FROM "Event" WHERE "dateRange" @> ${date}::timestamptz // ... |
We can improve the performance of this operation by creating a GiST index on the dateRange column. If you want to know more, check out API with NestJS #106. Improving performance through indexes with Prisma
Let’s allow users to provide the date they’re looking for through a query parameter. To make sure that they are using the correct format, we can use the class-validator library.
find-events-params.dto.ts
import { IsOptional, IsISO8601 } from 'class-validator'; export class FindEventsParamsDto { @IsOptional() @IsISO8601({ strict: true, date?: string; |
The last step is to use the new method and DTO in our controller.
events.controller.ts
import { Controller, Get, Query } from '@nestjs/common'; import { EventsService } from './events.service'; import { EventResponseDto } from './dto/event-response.dto'; import { TransformPlainToInstance } from 'class-transformer'; import { FindEventsParamsDto } from './dto/find-events-params.dto'; @Controller('events') export class EventsController { constructor(private readonly eventsService: EventsService) {} @Get() @TransformPlainToInstance(EventResponseDto) getAll(@Query() { date }: FindEventsParamsDto) { if (date) { return this.eventsService.search(date); return this.eventsService.getAll(); // ... |
Summary
In this article, we’ve shown how to use data types Prisma does not support yet, such as date ranges. To do that, we had to learn how to use the Unsupported type built into Prisma and how to make raw SQL queries. By understanding how the data range type works, we used it to our advantage by using operators not available with other data types. Thanks to all of that, we’ve learned quite a few valuable skills we can use with other features from PostgreSQL that Prisma does not implement.
Series Navigation<< API with NestJS #135. Referential actions and foreign keys in PostgreSQL with PrismaAPI with NestJS #137. Recursive relationships with Prisma and PostgreSQL >>
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK