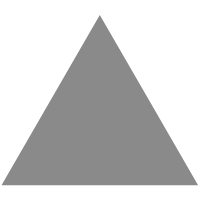
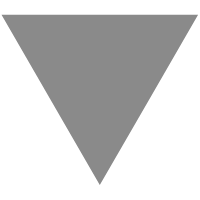
API with NestJS #127. Arrays with PostgreSQL and Kysely
source link: https://wanago.io/2023/10/02/api-nestjs-postgresql-arrays-kysely/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
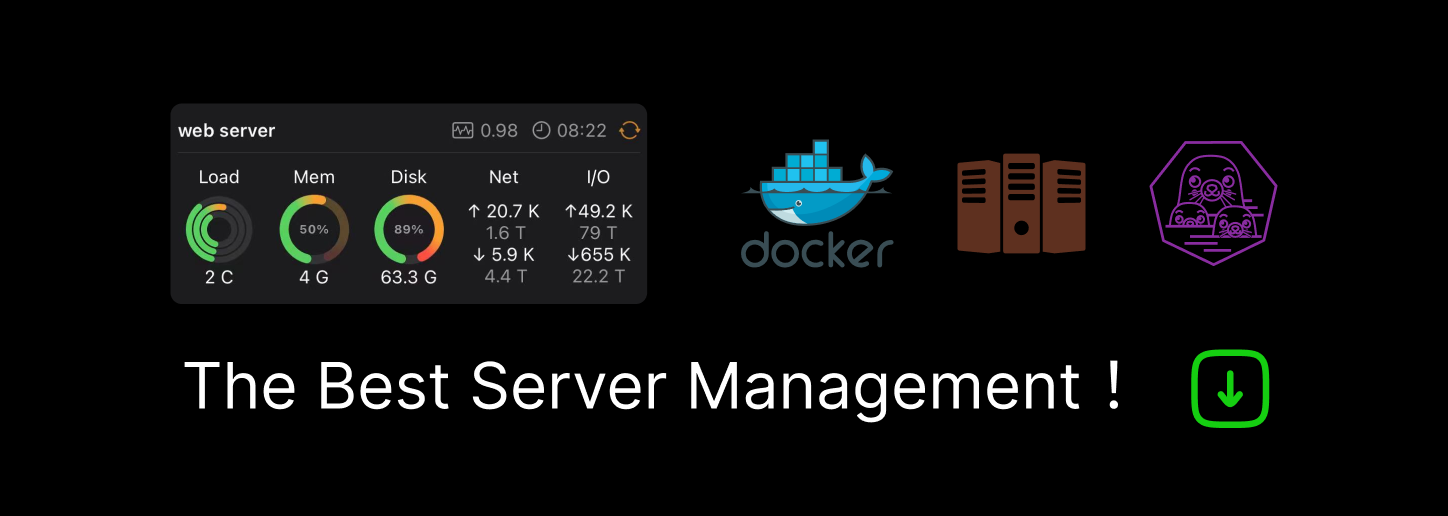
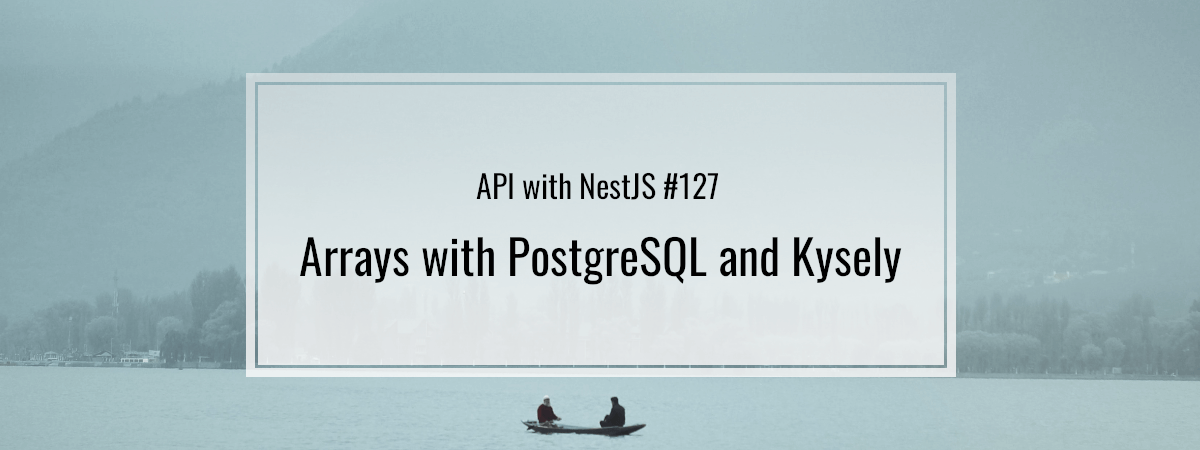
October 2, 2023
- 1. API with NestJS #1. Controllers, routing and the module structure
- 2. API with NestJS #2. Setting up a PostgreSQL database with TypeORM
- 3. API with NestJS #3. Authenticating users with bcrypt, Passport, JWT, and cookies
- 4. API with NestJS #4. Error handling and data validation
- 5. API with NestJS #5. Serializing the response with interceptors
- 6. API with NestJS #6. Looking into dependency injection and modules
- 7. API with NestJS #7. Creating relationships with Postgres and TypeORM
- 8. API with NestJS #8. Writing unit tests
- 9. API with NestJS #9. Testing services and controllers with integration tests
- 10. API with NestJS #10. Uploading public files to Amazon S3
- 11. API with NestJS #11. Managing private files with Amazon S3
- 12. API with NestJS #12. Introduction to Elasticsearch
- 13. API with NestJS #13. Implementing refresh tokens using JWT
- 14. API with NestJS #14. Improving performance of our Postgres database with indexes
- 15. API with NestJS #15. Defining transactions with PostgreSQL and TypeORM
- 16. API with NestJS #16. Using the array data type with PostgreSQL and TypeORM
- 17. API with NestJS #17. Offset and keyset pagination with PostgreSQL and TypeORM
- 18. API with NestJS #18. Exploring the idea of microservices
- 19. API with NestJS #19. Using RabbitMQ to communicate with microservices
- 20. API with NestJS #20. Communicating with microservices using the gRPC framework
- 21. API with NestJS #21. An introduction to CQRS
- 22. API with NestJS #22. Storing JSON with PostgreSQL and TypeORM
- 23. API with NestJS #23. Implementing in-memory cache to increase the performance
- 24. API with NestJS #24. Cache with Redis. Running the app in a Node.js cluster
- 25. API with NestJS #25. Sending scheduled emails with cron and Nodemailer
- 26. API with NestJS #26. Real-time chat with WebSockets
- 27. API with NestJS #27. Introduction to GraphQL. Queries, mutations, and authentication
- 28. API with NestJS #28. Dealing in the N + 1 problem in GraphQL
- 29. API with NestJS #29. Real-time updates with GraphQL subscriptions
- 30. API with NestJS #30. Scalar types in GraphQL
- 31. API with NestJS #31. Two-factor authentication
- 32. API with NestJS #32. Introduction to Prisma with PostgreSQL
- 33. API with NestJS #33. Managing PostgreSQL relationships with Prisma
- 34. API with NestJS #34. Handling CPU-intensive tasks with queues
- 35. API with NestJS #35. Using server-side sessions instead of JSON Web Tokens
- 36. API with NestJS #36. Introduction to Stripe with React
- 37. API with NestJS #37. Using Stripe to save credit cards for future use
- 38. API with NestJS #38. Setting up recurring payments via subscriptions with Stripe
- 39. API with NestJS #39. Reacting to Stripe events with webhooks
- 40. API with NestJS #40. Confirming the email address
- 41. API with NestJS #41. Verifying phone numbers and sending SMS messages with Twilio
- 42. API with NestJS #42. Authenticating users with Google
- 43. API with NestJS #43. Introduction to MongoDB
- 44. API with NestJS #44. Implementing relationships with MongoDB
- 45. API with NestJS #45. Virtual properties with MongoDB and Mongoose
- 46. API with NestJS #46. Managing transactions with MongoDB and Mongoose
- 47. API with NestJS #47. Implementing pagination with MongoDB and Mongoose
- 48. API with NestJS #48. Definining indexes with MongoDB and Mongoose
- 49. API with NestJS #49. Updating with PUT and PATCH with MongoDB and Mongoose
- 50. API with NestJS #50. Introduction to logging with the built-in logger and TypeORM
- 51. API with NestJS #51. Health checks with Terminus and Datadog
- 52. API with NestJS #52. Generating documentation with Compodoc and JSDoc
- 53. API with NestJS #53. Implementing soft deletes with PostgreSQL and TypeORM
- 54. API with NestJS #54. Storing files inside a PostgreSQL database
- 55. API with NestJS #55. Uploading files to the server
- 56. API with NestJS #56. Authorization with roles and claims
- 57. API with NestJS #57. Composing classes with the mixin pattern
- 58. API with NestJS #58. Using ETag to implement cache and save bandwidth
- 59. API with NestJS #59. Introduction to a monorepo with Lerna and Yarn workspaces
- 60. API with NestJS #60. The OpenAPI specification and Swagger
- 61. API with NestJS #61. Dealing with circular dependencies
- 62. API with NestJS #62. Introduction to MikroORM with PostgreSQL
- 63. API with NestJS #63. Relationships with PostgreSQL and MikroORM
- 64. API with NestJS #64. Transactions with PostgreSQL and MikroORM
- 65. API with NestJS #65. Implementing soft deletes using MikroORM and filters
- 66. API with NestJS #66. Improving PostgreSQL performance with indexes using MikroORM
- 67. API with NestJS #67. Migrating to TypeORM 0.3
- 68. API with NestJS #68. Interacting with the application through REPL
- 69. API with NestJS #69. Database migrations with TypeORM
- 70. API with NestJS #70. Defining dynamic modules
- 71. API with NestJS #71. Introduction to feature flags
- 72. API with NestJS #72. Working with PostgreSQL using raw SQL queries
- 73. API with NestJS #73. One-to-one relationships with raw SQL queries
- 74. API with NestJS #74. Designing many-to-one relationships using raw SQL queries
- 75. API with NestJS #75. Many-to-many relationships using raw SQL queries
- 76. API with NestJS #76. Working with transactions using raw SQL queries
- 77. API with NestJS #77. Offset and keyset pagination with raw SQL queries
- 78. API with NestJS #78. Generating statistics using aggregate functions in raw SQL
- 79. API with NestJS #79. Implementing searching with pattern matching and raw SQL
- 80. API with NestJS #80. Updating entities with PUT and PATCH using raw SQL queries
- 81. API with NestJS #81. Soft deletes with raw SQL queries
- 82. API with NestJS #82. Introduction to indexes with raw SQL queries
- 83. API with NestJS #83. Text search with tsvector and raw SQL
- 84. API with NestJS #84. Implementing filtering using subqueries with raw SQL
- 85. API with NestJS #85. Defining constraints with raw SQL
- 86. API with NestJS #86. Logging with the built-in logger when using raw SQL
- 87. API with NestJS #87. Writing unit tests in a project with raw SQL
- 88. API with NestJS #88. Testing a project with raw SQL using integration tests
- 89. API with NestJS #89. Replacing Express with Fastify
- 90. API with NestJS #90. Using various types of SQL joins
- 91. API with NestJS #91. Dockerizing a NestJS API with Docker Compose
- 92. API with NestJS #92. Increasing the developer experience with Docker Compose
- 93. API with NestJS #93. Deploying a NestJS app with Amazon ECS and RDS
- 94. API with NestJS #94. Deploying multiple instances on AWS with a load balancer
- 95. API with NestJS #95. CI/CD with Amazon ECS and GitHub Actions
- 96. API with NestJS #96. Running unit tests with CI/CD and GitHub Actions
- 97. API with NestJS #97. Introduction to managing logs with Amazon CloudWatch
- 98. API with NestJS #98. Health checks with Terminus and Amazon ECS
- 99. API with NestJS #99. Scaling the number of application instances with Amazon ECS
- 100. API with NestJS #100. The HTTPS protocol with Route 53 and AWS Certificate Manager
- 101. API with NestJS #101. Managing sensitive data using the AWS Secrets Manager
- 102. API with NestJS #102. Writing unit tests with Prisma
- 103. API with NestJS #103. Integration tests with Prisma
- 104. API with NestJS #104. Writing transactions with Prisma
- 105. API with NestJS #105. Implementing soft deletes with Prisma and middleware
- 106. API with NestJS #106. Improving performance through indexes with Prisma
- 107. API with NestJS #107. Offset and keyset pagination with Prisma
- 108. API with NestJS #108. Date and time with Prisma and PostgreSQL
- 109. API with NestJS #109. Arrays with PostgreSQL and Prisma
- 110. API with NestJS #110. Managing JSON data with PostgreSQL and Prisma
- 111. API with NestJS #111. Constraints with PostgreSQL and Prisma
- 112. API with NestJS #112. Serializing the response with Prisma
- 113. API with NestJS #113. Logging with Prisma
- 114. API with NestJS #114. Modifying data using PUT and PATCH methods with Prisma
- 115. API with NestJS #115. Database migrations with Prisma
- 116. API with NestJS #116. REST API versioning
- 117. API with NestJS #117. CORS – Cross-Origin Resource Sharing
- 118. API with NestJS #118. Uploading and streaming videos
- 119. API with NestJS #119. Type-safe SQL queries with Kysely and PostgreSQL
- 120. API with NestJS #120. One-to-one relationships with the Kysely query builder
- 121. API with NestJS #121. Many-to-one relationships with PostgreSQL and Kysely
- 122. API with NestJS #122. Many-to-many relationships with Kysely and PostgreSQL
- 123. API with NestJS #123. SQL transactions with Kysely
- 124. API with NestJS #124. Handling SQL constraints with Kysely
- 125. API with NestJS #125. Offset and keyset pagination with Kysely
- 126. API with NestJS #126. Improving the database performance with indexes and Kysely
- 127. API with NestJS #127. Arrays with PostgreSQL and Kysely
- 128. API with NestJS #128. Managing JSON data with PostgreSQL and Kysely
PostgreSQL outshines various other SQL databases with its feature set. Unlike most SQL databases, PostgreSQL offers extensive support for array columns. Using them, we can store collections of values within a single column without creating separate tables. In this article, we explore the capabilities of arrays in PostgreSQL and implement examples using Kysely.
Creating the migration
In the previous parts of this series, we defined a table containing articles.
articlesTable.ts
import { Generated } from 'kysely'; export interface ArticlesTable { id: Generated<number>; title: string; article_content: string; author_id: number; |
Let’s use an array instead of a simple article_content column.
articlesTable.ts
import { Generated } from 'kysely'; export interface ArticlesTable { id: Generated<number>; title: string; paragraphs: string[]; author_id: number; |
The most straightforward way of approaching the SQL migration would be to drop the article_content column and add the paragraphs column.
20230928231458_add_paragraphs_column.ts
import { Kysely, sql } from 'kysely'; export async function up(database: Kysely<unknown>): Promise<void> { await database.schema .alterTable('articles') .dropColumn('article_content') .addColumn('paragraphs', sql`text[]`, (column) => column.notNull()) .execute(); export async function down(database: Kysely<unknown>): Promise<void> { await database.schema .alterTable('articles') .dropColumn('paragraphs') .addColumn('article_content', 'text', (column) => column.notNull()); |
Above, we use the sql template tag, because Kysely does not understand the text[] column type yet.
While the above approach would work, it has a significant downside. Dropping the article_content column removes its contents, which means losing a lot of data. Instead, let’s use the values from the article_content column in the paragraphs array.
20230928231458_add_paragraphs_column.ts
import { Migration, sql } from 'kysely'; export const up: Migration['up'] = async (database) => { await database.schema .alterTable('articles') .addColumn('paragraphs', sql`text[]`) .execute(); await database .updateTable('articles') .set({ paragraphs: sql`ARRAY[article_content]`, .execute(); await database.schema .alterTable('articles') .dropColumn('article_content') .execute(); await database.schema .alterTable('articles') .alterColumn('paragraphs', (column) => { return column.setNotNull(); .execute(); export const down: Migration['down'] = async (database) => { await database.schema .alterTable('articles') .dropColumn('paragraphs') .addColumn('article_content', 'text', (column) => column.notNull()); |
Above, we perform four steps in our migrations:
- we add the paragraphs column,
- we set the first element of the paragraphs array to be the value from the article_content column
- we remove the article_content column
- we make the paragraphs column non-nullable since now all rows have a value for it.
Adjusting our models
The first step in making the above change work with our application is to adjust our Article model.
article.model.ts
export interface ArticleModelData { id: number; title: string; paragraphs: string[]; author_id: number; export class Article { id: number; title: string; paragraphs: string[]; authorId: number; constructor({ id, title, paragraphs, author_id }: ArticleModelData) { this.id = id; this.title = title; this.paragraphs = paragraphs; this.authorId = author_id; |
What’s great about the type-safety that Kysely offers is that it will let us know about all the places we need to adjust to accommodate for the paragraphs array.

Thanks to that, we can change our repository to adjust the article_content to paragraphs in all appropriate places.
Creating and updating arrays
When using Kysely, inserting a new record into the table containing the array column is straightforward.
const databaseResponse = await this.database .insertInto('articles') .values({ title: 'My article', paragraphs: [ 'First paragraph', 'Second paragraph' author_id: 1 .returningAll() .executeTakeFirstOrThrow(); |
We can use the class-validator library to verify if the user provided a valid array of strings.
article.dto.ts
import { IsString, IsNotEmpty, IsOptional, IsNumber } from 'class-validator'; export class ArticleDto { @IsString() @IsNotEmpty() title: string; @IsString({ each: true }) @IsNotEmpty({ each: true }) paragraphs: string[]; @IsOptional() @IsNumber({}, { each: true }) categoryIds?: number[]; |
The most straightforward way of modifying the paragraphs array is to provide a new value.
const databaseResponse = await database .updateTable('articles') .set({ title: 'My article', paragraphs: [ 'My new first paragraph', 'Second paragraph', .where('id', '=', 1) .returningAll() .executeTakeFirst(); |
Besides providing the full value for the array, PostgreSQL allows us to use various functions. A good example is array_append, which adds a new element at the end of the array.
const databaseResponse = await this.database .updateTable('articles') .set(({ ref }) => ({ paragraphs: sql`array_append(${ref('paragraphs')}, ${'Last paragraph'})`, .where('id', '=', 1) .returningAll() .executeTakeFirst(); |
On the other hand, array_prepend adds a new element at the beginning of the array.
const databaseResponse = await this.database .updateTable('articles') .set(({ ref }) => ({ paragraphs: sql`array_prepend(${ref('paragraphs')}, ${'First paragraph'})`, .where('id', '=', 1) .returningAll() .executeTakeFirst(); |
The trim_array function can remove a given number of elements from the end of the array. For example, we can use it to delete the last element of the paragraphs array.
const databaseResponse = await this.database .updateTable('articles') .set(({ ref }) => ({ paragraphs: sql`trim_array(${ref('paragraphs')}, 1)`, .where('id', '=', 1) .returningAll() .executeTakeFirst(); |
Searching through arrays
PostgreSQL allows us to search through arrays using the ANY and ALL keywords.
To find articles where all paragraphs equal a particular string, we need to use the ALL operator.
const databaseResponse = await this.database .selectFrom('articles') .where( sql`${'Lorem ipsum'}`, ({ ref }) => sql`ALL(${ref('paragraphs')})`, .selectAll() .execute(); |
To get the articles where any of the paragraphs equal a particular string, we need to use the ANY operator.
const databaseResponse = await this.database .selectFrom('articles') .where( sql`${'Lorem ipsum'}`, ({ ref }) => sql`ANY(${ref('paragraphs')})`, .selectAll() .execute(); |
Another good example is filtering based on the number of elements in the array. To do that, we need the array_length function. Let’s find all articles with at least one paragraph.
const databaseResponse = await this.database .selectFrom('articles') .where(({ ref }) => sql`array_length(${ref('paragraphs')}, 1)`, '>', 0) .selectAll() .execute(); |
The second argument in the array_length specifies which dimension of the array we want to measure and can be useful for multi-dimensional arrays.
Summary
In this article, we’ve gone through the idea of array columns and implemented examples using Kysely. Array columns can help store multiple related values in a list within PostgreSQL. They come with built-in functions and operators that help with various tasks.
However, arrays may not be suitable for every situation. Indexing and querying arrays might not perform well when dealing with large datasets. Creating a separate table and establishing relationships could be a better choice in such cases, especially if you need to enforce specific data rules.
It’s important to carefully assess your application’s requirements and weigh the pros and cons before deciding whether to use array columns in PostgreSQL. An extra tool is always a good idea, regardless of your choice.
Series Navigation<< API with NestJS #126. Improving the database performance with indexes and KyselyAPI with NestJS #128. Managing JSON data with PostgreSQL and Kysely >>
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK