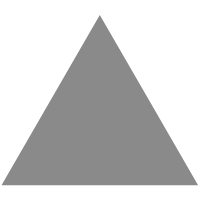
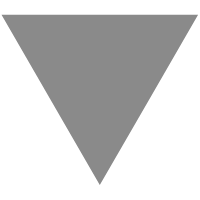
How to make HTTP requests in Node.js
source link: https://www.laravelcode.com/post/how-to-make-http-requests-in-node-js
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
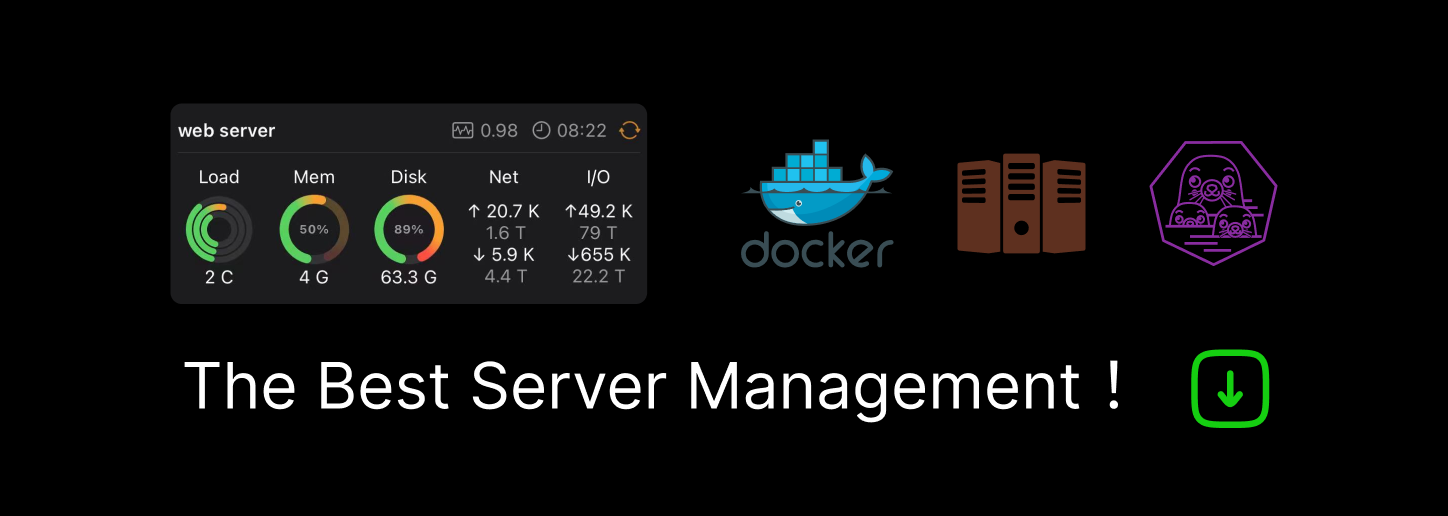
How to make HTTP requests in Node.js
There are so many packages available to make http request in Node.js. Some popular packages are Axios, node-fetch, SuperAgent, Got etc.
In this article we will see Http module from standard library. We will also check with Axios and Request library.
Below is the example of Http library get method.
const https = require('https')
// create request
const options = {
hostname: 'https://api.laravelcode.com',
port: 443,
path: '/api/get-user',
method: 'GET'
}
// send request
const req = https.request(options, res => {
// request success
res.on('data', d => {
process.stdout.write(d)
})
})
// on error in request
req.on('error', error => {
console.error(error)
})
// close request
req.end()
And here is how to create Post request in Node.js
const https = require('https')
const data = JSON.stringify({
name: 'laravelcode',
email: '[email protected]',
}
// create post request
const options = {
hostname: 'https://api.laravelcode.com',
port: 443,
path: '/create/user',
method: 'POST',
headers: {
'Content-Type': 'application/json'
}
}
const req = https.request(options, res => {
// request success
res.on('data', d => {
process.stdout.write(d)
})
})
// on request error
req.on('error', error => {
console.error(error)
})
// close request
req.write(data)
req.end()
Create request using Axios library
Axios is promise-based http request library. It can be used in Browser and Node.js also. Axios is useful when lots of requests are chained with complicated logic. To send request using Axios, first we need to install using below command.
npm install axios --save
Then we can send the request using below code:
const axios = require('axios');
axios.get('https://api.laravelcode.com/api/get-user')
.then(res => {
console.log(res.status);
const users = res.users;
})
// error
.catch(err => {
console.log(err.message);
});
Using Request library
Request is simple http request library and same as Python's Request. It can be used for simple http requests. To install Request library, run below command.
npm install request --save
Now you can use Request module to create Http request. Here is simple example code for above one.
const request = require('request');
request('https://api.laravelcode.com/api/get-user', { json: true }, (err, res, body) => {
if (err) {
console.log(err);
}
console.log(body.user);
});
Conclusion
There are also other popular library available. You can choose any of them which you need. It all depends upon requirement and preference.
I hope it will help you.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK