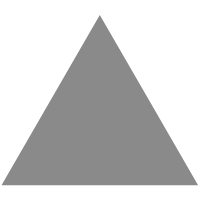
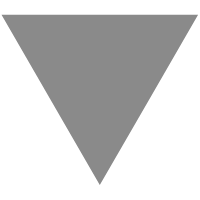
Vue.js设计与实现18-KeepAlive的原理与实现
source link: https://www.fly63.com/article/detial/11437
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
扫一扫分享
1.写在前面
前面文章介绍了vue.js通过渲染器实现组件化的能力,介绍了有状态组件和无状态组件的构造与实现,还有异步组件对于框架的意义。本文将主要介绍Vue.js的重要内置组件和模块--KeepAlive组件。
2.KeepAlive组件
KeepAlive字面意思理解就是保持鲜活,就是建立持久连接的意思,可以避免组件或连接频繁地创建和销毁。
<template>
<KeepAlive>
<Tab v-if="currentTab === 1"/>
<Tab v-if="currentTab === 2"/>
<Tab v-if="currentTab === 3"/>
</KeepAlive>
</template>
在上面代码中,会根据currentTab变量的值频繁切换Tab组件,会导致不停地卸载和重建对应的Tab组件,为了避免因此产生的性能开销,可以使用KeepAlive组件保持组件的鲜活。那么KeepAlive组件是如何保持组件的鲜活的,其实就会对组件进行缓存管理,避免组件频繁的卸载和重建。
其实,就是通过一个隐藏的组件缓存容器,将组件需要的时候将其放到容器里,在需要重建使用的时候将其取出,这样对于用户感知是进行了“卸载”和“重建”组件。在组件搬运到缓存容器和搬出,就是对应组件的生命周期activated和deactivated。
3.组件的失活和激活
那么,应该如何实现组件的缓存管理呢?
const KeepAlive = {
// keepAlive组件的标识符
_isKeepAlive:true,
setup(props,{slots}){
//缓存容器
const cache = new Map();
const instance = currentInstance;
const { move, createElement } = instance.keepAliveCtx;
//隐藏容器
const storageContainer = createElement("div");
instance._deActivate = (vnode)=>{
move(vnode, storageContainer)
};
instance._activate = (vnode, container, anchor)=>{
move(vnode, container, anchor)
};
return ()=>{
let rawNode = slots.default();
// 非组件的虚拟节点无法被keepAlive
if(typeof rawNode.type !== "object"){
return rawNode;
}
//在挂在时先获取缓存的组件vnode
const cacheVNode = cache.get(rawNode.type);
if(cacheVNode){
rawVNode.component = cacheVNode.component;
rawVNode.keptAlive = true;
}else{
cache.set(rawVNode.type, rawVNode);
}
rawVNode.shouldKeepAlive = true;
rawVNode.keepAliveInstance = instance;
// 渲染组件vnode
return rawVNode
}
}
}
在上面代码中,KeepAlive组件本身不会渲染额外的内容,渲染函数只返回被KeepAlive的组件,被称为“内部组件”,KeepAlive会在“内部组件”的Vnode对象上添加标记属性,便于渲染器执行特定逻辑。
- shouldKeepAlive属性会被添加到“内部组件”的vnode对象上,当渲染器卸载“内部组件”时,可以通过检查属性得知“内部组件”是否需要被KeepAlive。
- keepAliveInstance:内部组件的vnode对象会持有keepAlive组件实例,在unmount函数中通过keepAliveInstance访问_deactivate函数。
- keptAlive:内部组件已被缓存则添加keptAlive标记,判断内部组件重新渲染时是否需要重新挂载还是激活。
function unmount(vnode){
if(vnode.type === Fragment){
vnode.children.forEach(comp=>unmount(comp));
return;
}else if(typeof vnode.type === "object"){
if(vnode.shouldKeepAlive){
vnode.keepAliveInstance._deactivate(vnode);
}else{
unmount(vnode.component.subTree);
}
return
}
const parent = vnode.el.parentVNode;
if(parent){
parent.removeChild(vnode.el);
}
}
组件失活的本质是将组件所渲染的内容移动到隐藏容器中,激活的本质是将组件所要渲染的内容从隐藏容器中搬运回原来的容器。
const { move, createElement } = instance.keepAliveCtx;
instance._deActivate = (vnode)=>{
move(vnode, storageContainer);
}
instance._activate = (vnode, container, anchor)=>{
move(vnode, container, anchor);
}
4.include和exclude
我们看到上面的代码会对组件所有的"内部组件"进行缓存,但是使用者又想自定义缓存规则,只对特定组件进行缓存,对此KeepAlive组件需要支持两个props:include和exclude。
- include:用于显式配置应被缓存的组件
- exclude:用于显式配置不应该被缓存的组件
const cache = new Map();
const keepAlive = {
__isKeepAlive: true,
props:{
include: RegExp,
exclude: RegExp
},
setup(props, {slots}){
//...
return ()=>{
let rawVNode = slots.default();
if(typeof rawVNode.type !== "object"){
return rawVNode;
}
const name = rawVNode.type.name;
if(name && (
(props.include && !props.include.test(name)) ||
(props.exclude && props.include.test(name))
)){
//直接渲染内部组件,不对其进行缓存操作
return rawVNode
}
}
}
}
上面代码中,为了简便阐述问题进行设置正则类型的值,在KeepAlive组件被挂载时,会根据"内部组件"的名称进行匹配,根据匹配结果判断是否要对组件进行缓存。
5.缓存管理
在前面小节中使用Map对象实现对组件的缓存,Map的键值对分别对应的是组件vnode.type属性值和描述该组件的vnode对象。因为用于描述组件的vnode对象存在对组件实例的引用,对此缓存用于描述组件的vnode对象,等价于缓存了组件实例。
前面介绍的keepAlive组件实现缓存的处理逻辑是:
- 缓存存在时继承组件实例,将描述组件的vnode对象标记为keptAlive,渲染器不会重新创建新的组件实例
- 缓存不存在时,则设置缓存
但是,如果缓存不存在时,那么总是会设置新的缓存,这样导致缓存不断增加,会占用大量内存。对此,我们需要设置个内存阈值,在缓存数量超过指定阈值时需要对缓存进行修剪,在Vue.js中使用的是"最新一次访问"策略。
"最新一次访问"策略本质上就是通过设置当前访问或渲染的组件作为最新一次渲染的组件,并且该组件在修剪过程中始终是安全的,即不会被修剪。
缓存实例中需要满足固定的格式:
const _cache = new Map();
const cache: KeepAliveCache = {
get(key){
_cache.get(key);
},
set(key, value){
_cache.set(key, value);
},
delete(key){
_cache.delete(key);
},
forEach(fn){
_cache.forEach(fn);
}
}
6.写在最后
本文简单介绍了Vue.js中KeepAlive组件的设计与实现原理,可以实现对组件的缓存,避免组件实例不断地销毁和重建。KeepAlive组件卸载时渲染器并不会真实地把它进行卸载,而是将该组件搬运到另外一个隐藏容器里,从而使得组件能够维持当前状态。在KeepAlive组件挂载时,渲染器将其从隐藏容器中搬运到原容器中。此外,我们还讨论了KeepAlive组件的include和exclude自定义缓存,以及缓存管理。
来源: 前端一码平川
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK