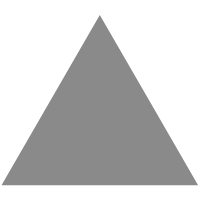
6
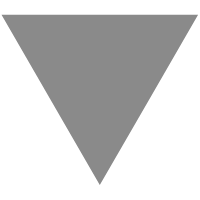
【基础】Golang异常处理
source link: https://wintrysec.github.io/2021/09/26/%E3%80%905%E3%80%91%E5%AE%89%E5%85%A8%E5%BC%80%E5%8F%91/Golang/%E3%80%90%E5%9F%BA%E7%A1%80%E3%80%91Golang%E5%BC%82%E5%B8%B8%E5%A4%84%E7%90%86/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
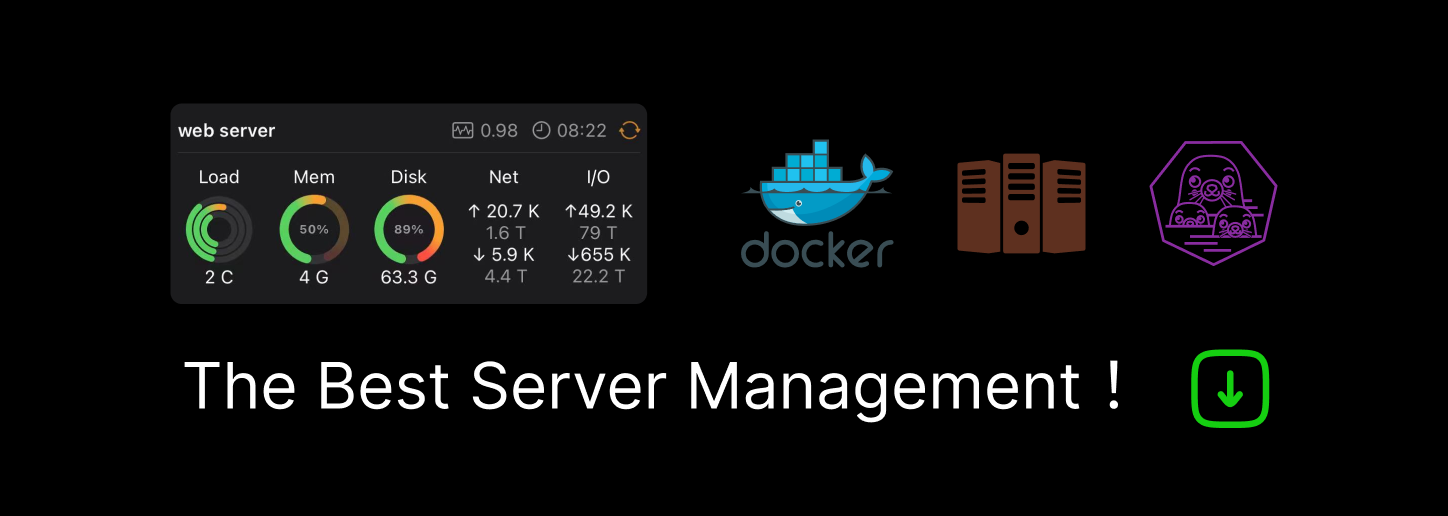
什么是异常?
使用Python的时候我们用try..catch.. finall...
处理异常,但是Go中则用defer, panic, recover,将异常和控制流程区分开。即通过panic抛出异常,然后在defer中,通过recover捕获这个异常,最后处理。
panic 的中文翻译为 恐慌,当程序遇到错误的时候就会恐慌造成程序崩溃!
比如如下代码(求玩具球的体积):
package main
import (
"fmt"
"math"
)
func GetBallVolumn(radius float64) float64{
if radius<1 {
panic("小球半径不能为负数")
}
return (4/3.0)*math.Pi*math.Pow(radius,3)
}
func main() {
volumn :=GetBallVolumn(-1)
fmt.Println("小球的体积是:",volumn)
}
异常的处理
上边的这个程序抛出了异常,然后程序也崩溃了,我们要做的是处理这个异常让程序继续跑。
package main
import (
"fmt"
"math"
)
func GetBallVolumn(radius float64) float64{
if radius<1 {
panic("小球半径不能为负数")
}
return (4/3.0)*math.Pi*math.Pow(radius,3)
}
func main() {
//在函数结束之前处理异常,从恐慌中复活,找到恐慌的原因
defer func() {
if err :=recover();err !=nil{
fmt.Println("引起错误的原因是:",err)
}
}()
volumn :=GetBallVolumn(-1)
fmt.Println("小球的体积是:",volumn)
}
错误与处理
一种比较温和的错误提示,而不是直接 panic 让程序崩溃掉。
限制小球的半径取值范围:
package main
import (
"errors"
"fmt"
"math"
)
func GetBallVolumn(radius float64) (vol float64, err error){
if radius < 0 {
panic("小球半径不能为负数")
}
//定义错误信息,如果存在则直接return
if radius < 5 || radius > 50 {
err :=errors.New("半径的合法范围是5~50")
return 0, err
}
return (4/3.0)*math.Pi*math.Pow(radius,3),nil //没错误错误返回nil
}
func main() {
//在函数结束之前处理异常
defer func() {
if err :=recover();err !=nil{
fmt.Println("引起错误的原因是:",err)
}
}()
volumn, err :=GetBallVolumn(1)
//如果存在错误则提示错误信息
if err != nil{
fmt.Println("获取体积失败,err=", err)
}
fmt.Println("小球的体积是:",volumn)
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK