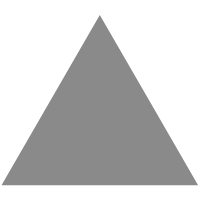
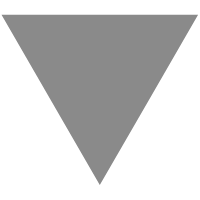
Golang html/template versus Python Jinja2 (1)
source link: http://siongui.github.io/2015/02/21/python-jinja2-vs-go-html-template-1/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Golang html/template versus Python Jinja2 (1)
Updated: March 06, 2015
This post compares the following two combinations:
to show how to serve a "Hello World" webpage.
Go html/template versue Python Jinja2 - Load and Serve Templates
Go html/template Python Jinja2
import "html/template"
import jinja2
// assume template and go source code are in the same directory.
# Tell Jinja2 where the template folder is # Template files and Python scripts are in the same directory in this example. import os JINJA_ENVIRONMENT = jinja2.Environment( loader=jinja2.FileSystemLoader(os.path.dirname(__file__)), extensions=['jinja2.ext.autoescape'], autoescape=True)
t, _ := template.ParseFiles("index.html")
template = JINJA_ENVIRONMENT.get_template('index.html')
in index.html:
Hello {{ . }}
in index.html:
Hello {{ name }}
setup tempalate value in Go:
template_values := "World"
setup tempalate value in Python:
template_values = {'name': 'World'}
use go net/http to serve webpage
// w http.ResponseWriter t.Execute(w, template_values)
use webapp2 to serve webpage
# self.response.write() => write to client browser self.response.write(template.render(template_values))
use web.py to serve webpage
return template.render(template_values)
Complete Jinja2 template:
index-jinja2.html | repository | view raw
<!doctype html> <html> <head> <meta charset="utf-8"> <title>Hello World</title> </head> <body> Hello {{ name }} </body> </html>
Complete web.py code for serving the above template:
webpy-jinja2.py | repository | view raw
#!/usr/bin/env python # -*- coding:utf-8 -*- import web import jinja2 import os JINJA_ENVIRONMENT = jinja2.Environment( loader=jinja2.FileSystemLoader(os.path.dirname(__file__)), extensions=['jinja2.ext.autoescape'], autoescape=True) urls = ( r"/", "MainPage" ) class MainPage: def GET(self): template_values = { 'name': 'World', } template = JINJA_ENVIRONMENT.get_template('index-jinja2.html') return template.render(template_values) if __name__ == '__main__': app = web.application(urls, globals()) app.run()
Complete webapp2 (run on GAE Python) code for serving the above template:
webapp2-jinja2.py | repository | view raw
#!/usr/bin/env python # -*- coding:utf-8 -*- import jinja2 import os import webapp2 JINJA_ENVIRONMENT = jinja2.Environment( loader=jinja2.FileSystemLoader(os.path.dirname(__file__)), extensions=['jinja2.ext.autoescape'], autoescape=True) class MainPage(webapp2.RequestHandler): def get(self): template_values = { 'name': 'World', } template = JINJA_ENVIRONMENT.get_template('index-jinja2.html') self.response.write(template.render(template_values)) application = webapp2.WSGIApplication([ ('/', MainPage), ], debug=True)
Complete html/template template:
index-go.html | repository | view raw
<!doctype html> <html> <head> <meta charset="utf-8"> <title>Hello World</title> </head> <body> Hello {{ . }} </body> </html>
Complete net/http code for serving the above template:
html-net.go | repository | view raw
package main import ( "html/template" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { t, _ := template.ParseFiles("index-go.html") name := "World" t.Execute(w, name) } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
Tested on: Ubuntu Linux 14.10, Go 1.4, Python 2.7.8, Google App Engine Python SDK 1.9.18, Jinja2 2.7.3
Golang html/template versus Python Jinja2 series:
[1]Golang html/template versus Python Jinja2 (1)
[2]Golang html/template versus Python Jinja2 (2)
[3]Golang html/template versus Python Jinja2 (3) - Arrays and Slices
[4]Golang html/template versus Python Jinja2 (4) - Arrays and Slices Index
[5]Golang html/template versus Python Jinja2 (5) - Maps and Dictionaries
[6]Golang html/template versus Python Jinja2 (6) - Template Inheritance (Extends)
[7]Golang html/template versus Python Jinja2 (7) - Custom Functions and Filters
References:
[a]html/template - The Go Programming Language
[b]src/html/template/ - The Go Programming Language
[c]text/template - The Go Programming Language
[d]src/text/template/ - The Go Programming Language
[e]Jinja2 (The Python Template Engine)
[g]Using Templates - Google App Engine for Python
[h]Go HTML Templates: Not Jinja2
[i]Go HTML Templates: Applying Data
[j]Go HTML Templates: Functions and Flow
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK