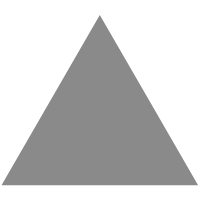
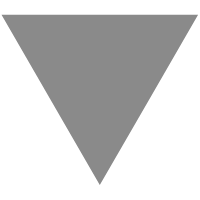
Golang html/template versus Python Jinja2 (6) - Template Inheritance (Extends)
source link: http://siongui.github.io/2015/03/08/python-jinja2-vs-go-html-template-extends/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Golang html/template versus Python Jinja2 (6) - Template Inheritance (Extends)
March 08, 2015
Template inheritance is a powerful feature of Jinja2. Here we show how to mimic the template extends of Jinja2 in Go html/template.
Go html/template versue Python Jinja2 - Template Inheritance (Extends)
Go html/template Python Jinja2
"base" template:
{{define "base"}} <!doctype html> <html> <head> <meta charset="utf-8"> <title>{{template "title" .}}</title> </head> <body> {{template "content" .}} </body> </html> {{end}}
"base" template:
<!doctype html> <html> <head> <meta charset="utf-8"> <title>{% block title %}{% endblock %}</title> </head> <body> {% block content %}{% endblock %} </body> </html>
index.html:
{{template "base" .}} {{define "title"}}my title{{end}} {{define "content"}} <div>hello {{.}}</div> {{end}}
index.html:
{% extends "base.html" %} {% block title %}my title{% endblock %} {% block content %} <div>hello {{ name }}</div> {% endblock %}
Complete Go html/template source code:
base-go.html | repository | view raw
{{define "base"}} <!doctype html> <html> <head> <meta charset="utf-8"> <title>{{template "title" .}}</title> </head> <body> {{template "content" .}} </body> </html> {{end}}
{{template "base" .}} {{define "title"}}my title{{end}} {{define "content"}} <div>hello {{.}}</div> {{end}}
package main import ( "html/template" "os" ) func main() { // cannot put base-go.html before index-go.html // the following will give empty output //t, _ := template.ParseFiles("base-go.html", "index-go.html") t, _ := template.ParseFiles("index-go.html", "base-go.html") name := "world" t.Execute(os.Stdout, name) }
Complete Python Jinja2 source code:
base.html | repository | view raw
<!doctype html> <html> <head> <meta charset="utf-8"> <title>{% block title %}{% endblock %}</title> </head> <body> {% block content %}{% endblock %} </body> </html>
{% extends "base.html" %} {% block title %}my title{% endblock %} {% block content %} <div>hello {{ name }}</div> {% endblock %}
#!/usr/bin/env python # -*- coding:utf-8 -*- import jinja2 import os JINJA_ENVIRONMENT = jinja2.Environment( loader=jinja2.FileSystemLoader(os.path.dirname(__file__)), extensions=['jinja2.ext.autoescape'], autoescape=True) if __name__ == '__main__': template_values = { 'name': 'world', } template = JINJA_ENVIRONMENT.get_template('index.html') print(template.render(template_values))
Tested on: Ubuntu Linux 14.10, Go 1.4, Python 2.7.8, Jinja2 2.7.3
Golang html/template versus Python Jinja2 series:
[1]Golang html/template versus Python Jinja2 (1)
[2]Golang html/template versus Python Jinja2 (2)
[3]Golang html/template versus Python Jinja2 (3) - Arrays and Slices
[4]Golang html/template versus Python Jinja2 (4) - Arrays and Slices Index
[5]Golang html/template versus Python Jinja2 (5) - Maps and Dictionaries
[6]Golang html/template versus Python Jinja2 (6) - Template Inheritance (Extends)
[7]Golang html/template versus Python Jinja2 (7) - Custom Functions and Filters
References:
[a]Template Inheritance - Jinja2 Documentation
[b]Nested template definitions - template - The Go Programming Language
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK