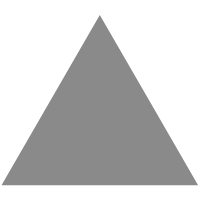
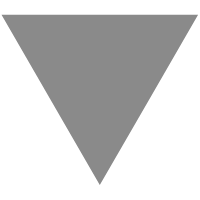
[Golang] Draggable (Movable) Element by GopherJS
source link: http://siongui.github.io/2016/01/17/go-draggable-movable-element-by-gopherjs/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Source Code
First we write a simple HTML for our demo:
index.html | repository | view raw
<!doctype html> <html> <head> <title>Golang Draggable (Movable) Element</title> </head> <body> <div id="dragMe" style="width: 200px; height: 200px; background: yellow;">Drag Me</div> <script src="demo.js"></script> </body> </html>
The basic idea is that we bind onmousedown event handler to the draggable element. In the onmousedown event handler, we bind onmousemove and onmouseup event handler to document object. In the onmousemove event handler, we calculate the movement and change the CSS property of the draggable element to make it move. In the onmouseup event handler, we remove the onmousemove and onmouseup event handler from document object.
draggable.go | repository | view raw
package main import "github.com/gopherjs/gopherjs/js" import "honnef.co/go/js/dom" import "strconv" func draggable(elm *dom.HTMLDivElement) { var startX, startY, initialMouseX, initialMouseY int var fmove, fup func(*js.Object) elm.Style().SetProperty("position", "absolute", "") d := dom.GetWindow().Document() mousemove := func(event dom.Event) { event.PreventDefault() e := event.(*dom.MouseEvent) dx := e.ClientX - initialMouseX dy := e.ClientY - initialMouseY elm.Style().SetProperty("top", strconv.Itoa(startY+dy)+"px", "") elm.Style().SetProperty("left", strconv.Itoa(startX+dx)+"px", "") } mouseup := func(event dom.Event) { d.RemoveEventListener("mousemove", false, fmove) d.RemoveEventListener("mouseup", false, fup) } elm.AddEventListener("mousedown", false, func(event dom.Event) { event.PreventDefault() e := event.(*dom.MouseEvent) startX = int(elm.OffsetLeft()) startY = int(elm.OffsetTop()) initialMouseX = e.ClientX initialMouseY = e.ClientY fmove = d.AddEventListener("mousemove", false, mousemove) fup = d.AddEventListener("mouseup", false, mouseup) }) } func main() { draggable(dom.GetWindow().Document().GetElementByID("dragMe").(*dom.HTMLDivElement)) }
Compile the Go code to JavaScript by:
$ gopherjs build draggable.go -o demo.js
Put demo.js together with the index.html in the same directory. Open the index.html with your browser, and start to drag the element in the browser!
Tested on: Ubuntu Linux 15.10, Go 1.5.3.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK