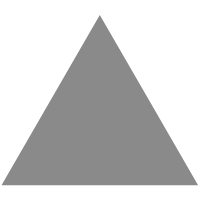
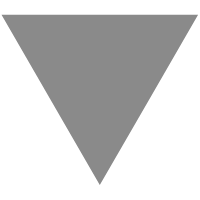
Converting a text file to PDF in Go
source link: https://blog.logrocket.com/converting-text-file-pdf-go/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
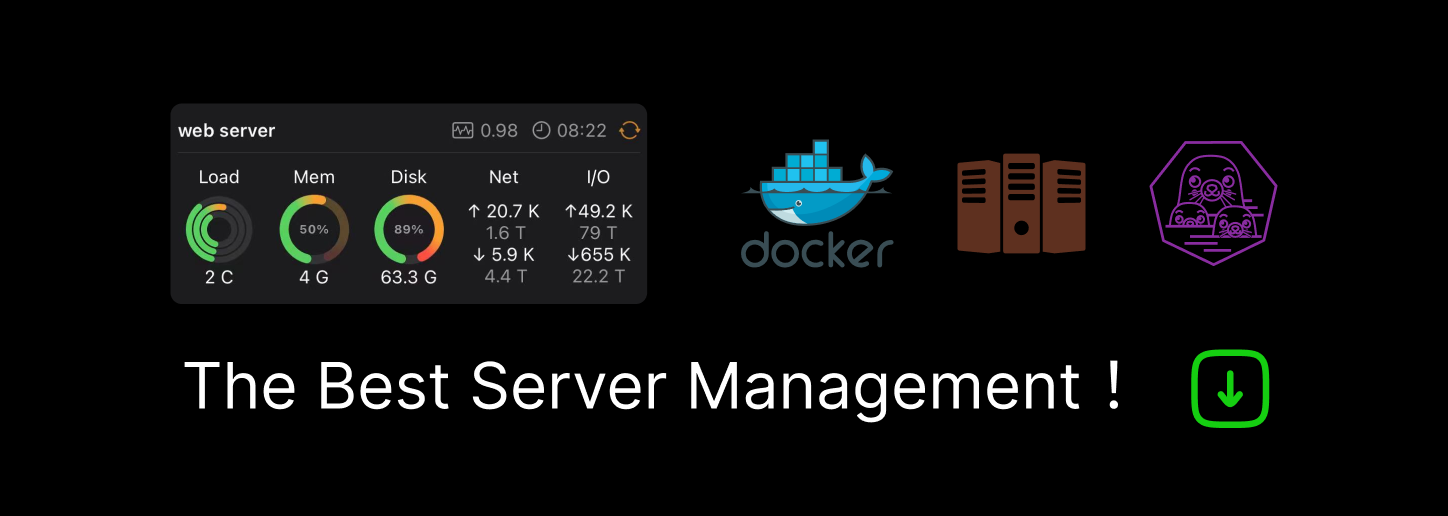
Golang is one of the fastest growing languages today. Its popularity has no doubt given rise to a lot of packages being built by the Go community, and we will take a look at one of these packages today.
In this article, we will be taking a look at how we can convert a text file to PDF format with Golang. Excited? So am I! Let’s get into it.
A look at the gofpdf package
The gofpdf package is a document generator with high-level support for text, drawing and images. It was created by Jung Kurt and you can find the GitHub repository here. The repository is currently archived and read-only, but the package is stable enough for our use case.
The package comes with a lot of features like page compression; clipping; barcodes; inclusion of JPEG, PNG, GIF, TIFF and basic path-only SVG images; document protection; charts, etc. The package has no other dependencies other than the Golang standard library.
Installing dependencies
We’ll install two packages, which in this case are the fmt
library for printing text out to the console and the github.com/jung-kurt/gofpdf
package for converting a text file to PDF format.
Create a file called main.go
and paste in the following code:
package main import ( "fmt" "github.com/jung-kurt/gofpdf" )
Now, in your terminal, navigate to the directory containing your main.go
file and run the command go mod init go-pdf
to initialize Go modules in the current directory.
Next, run go mod tidy
to download all the required packages.
Creating our first PDF file
In order to create our first PDF, we’ll update the main.go
file with the following code:
... func main() { pdf := gofpdf.New("P", "mm", "A4", "") pdf.AddPage() pdf.SetFont("Arial", "B", 16) pdf.MoveTo(0, 10) pdf.Cell(1, 1, "Hello world") err := pdf.OutputFileAndClose("hello.pdf") if err == nil { fmt.Println("PDF generated successfully") } }
Here, we create a new PDF object with gofpdf.New
, set the orientation to portrait, the unit system to millimeters, the paper size to A4, and we leave the option for the font directory as a blank string. Then, we set the font to Arial with a weight of bold and set the font size to 16.
We proceed to create a cell that’s 40mm wide and 10mm high and we write the text “Hello world” in there. Then, we save it to a file called "hello.pdf"
and we write out a message saying the PDF was created successfully.
After this, run the command go run main.go
to run the code, and you should see a PDF file generated in your current directory.
Converting a text file to PDF
To convert a text file to a PDF, first we read the file using the io/ioutil library and write the output to a PDF file. Replace the code in your main.go
file with the following:
package main import ( "fmt" "io/ioutil" "github.com/jung-kurt/gofpdf" ) func main() { text, err := ioutil.ReadFile("lorem.txt") pdf := gofpdf.New("P", "mm", "A4", "") pdf.AddPage() pdf.SetFont("Arial", "B", 16) pdf.MoveTo(0, 10) pdf.Cell(1, 1, "Lorem Ipsum") pdf.MoveTo(0, 20) pdf.SetFont("Arial", "", 14) width, _ := pdf.GetPageSize() pdf.MultiCell(width, 10, string(text), "", "", false) err = pdf.OutputFileAndClose("hello.pdf") if err == nil { fmt.Println("PDF generated successfully") } }
First, we read the text from the lorem.txt
file, then we write the title of the document as we did before except we change the text to Lorem Ipsum
. We move the cursor to the start of the line, then, we set the font size to 14, the font weight to normal, and we create a pdf.Multicell
that spans the entire width of the page.
Here, we write the text content in the cell and save to a file called hello.pdf
.
Create a file called lorem.txt
and paste the following text:
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec varius leo quis augue finibus gravida sit amet vitae ligula. Ut sit amet scelerisque eros. Vivamus molestie purus nec orci porta commodo vel ut erat. Sed tincidunt est vitae ligula sodales, pellentesque aliquet libero ullamcorper. Pellentesque pretium lacinia aliquet. Sed at enim ut nunc aliquet fringilla. Morbi rutrum sed velit non volutpat. Vivamus in venenatis eros. Phasellus purus mauris, mollis in condimentum sed, convallis dapibus neque. Etiam pharetra ut ex eu blandit. Morbi mattis consectetur posuere. Suspendisse tincidunt nunc vitae nibh condimentum lacinia. Suspendisse pulvinar velit a lectus tristique, sed congue nisi mattis. Proin a aliquet mauris, sed rutrum lorem. Morbi nec tellus ac mi ornare blandit eu sit amet velit.
Now, save and run the command go run main.go
and a hello.pdf
file should be generated in the current directory.
Conclusion
In this article, we took a look at the gofpdf library and some of its features for generating documents. We also have seen how easy it is to convert a text file to PDF format using the gofpdf library.
Unipdf is a gofpdf alternative that you would like to consider if you are interested in advanced page manipulations. It allows you to create and read PDF files, compress and optimize PDF files, watermark PDF files, process PDF files, and much more.
Check out the gofpdf GitHub repository if you are interested in exploring more about the package and its features.
LogRocket: Full visibility into your web apps
LogRocket is a frontend application monitoring solution that lets you replay problems as if they happened in your own browser. Instead of guessing why errors happen, or asking users for screenshots and log dumps, LogRocket lets you replay the session to quickly understand what went wrong. It works perfectly with any app, regardless of framework, and has plugins to log additional context from Redux, Vuex, and @ngrx/store.
In addition to logging Redux actions and state, LogRocket records console logs, JavaScript errors, stacktraces, network requests/responses with headers + bodies, browser metadata, and custom logs. It also instruments the DOM to record the HTML and CSS on the page, recreating pixel-perfect videos of even the most complex single-page apps.
Try it for free.Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK