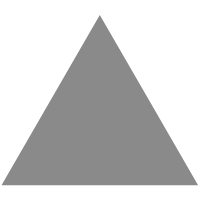
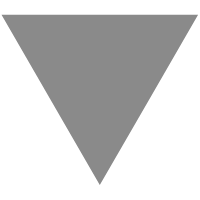
Basic rules of Hoisting in JavaScript
source link: https://codebase.sayfessyd.com/tips/programming/2022/04/09/basic-rules-of-hoisting-in-javascript.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
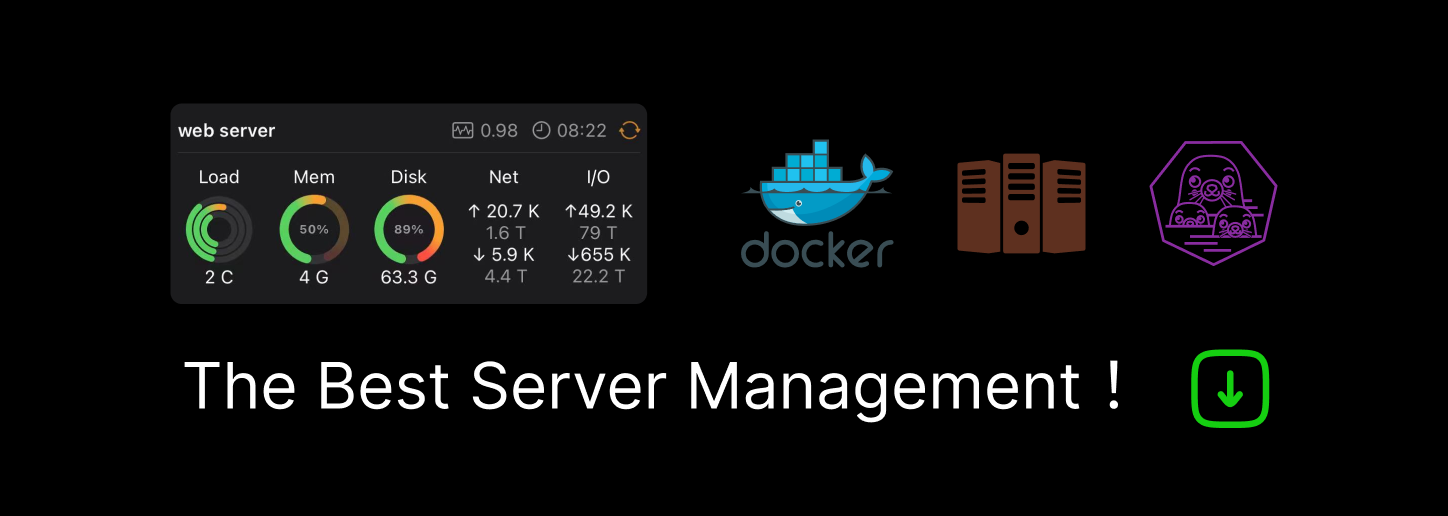
Introduction
Hoisting is a JavaScript feature that moves variable and function declarations to the top of their scope before the code is executed. Below are the rules that you should know about this mechanism.
Declarations are hoisted but assignments are left in place
var statements are relocated to the top of the global or function scope to which they belong, without their value initialization.
var bool = true;
function show() {
console.log(bool); // undefined
var bool = false;
}
show();
If we apply the hoisting mechanism the above lines of code will be equivalent to:
var bool = true;
function show() {
var bool;
console.log(bool); // undefined
bool = false;
}
show();
This example will show undefined
since the declaration of the bool
variable is moved to the top of the function scope.
It will not show the global value because a local variable is already declared.
Please note that even if we are dealing with functions, this rule remains valid which means that only function declarations are hoisted.
Declarations in the catch block are hoisted
Declarations are moved to the top of the global scope even when it’s inside a catch block.
console.log(object) // undefined
try {
throw new Error();
} catch (exception) {
var object = {};
}
let, const and class declarations are hoisted but stay uninitialized
let statements are hoisted to the top of the function scope. The only difference with var
statements is that they are not initialized with undefined
, a ReferenceError
exception is thrown instead when we try to access it.
var scope = "global";
function show() {
console.log(scope); // Reference error: scope is not defined
let scope = "local";
}
show();
What exactly happens…
Variable and function declarations are not physically moved to the top of our code, they are put in memory during the compilation phase and remain exactly where we typed them in our code.
Thank you for your time, if you want to receive updates from the author, you can follow him on Twitter @sayfessyd.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK