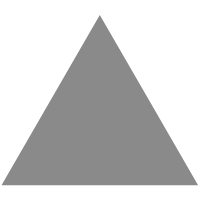
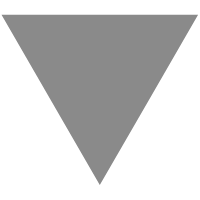
Spirally Reading Through 2D Matrices in C++ [Easy Guide]
source link: https://www.journaldev.com/60799/spirally-read-2d-matrices-cpp
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
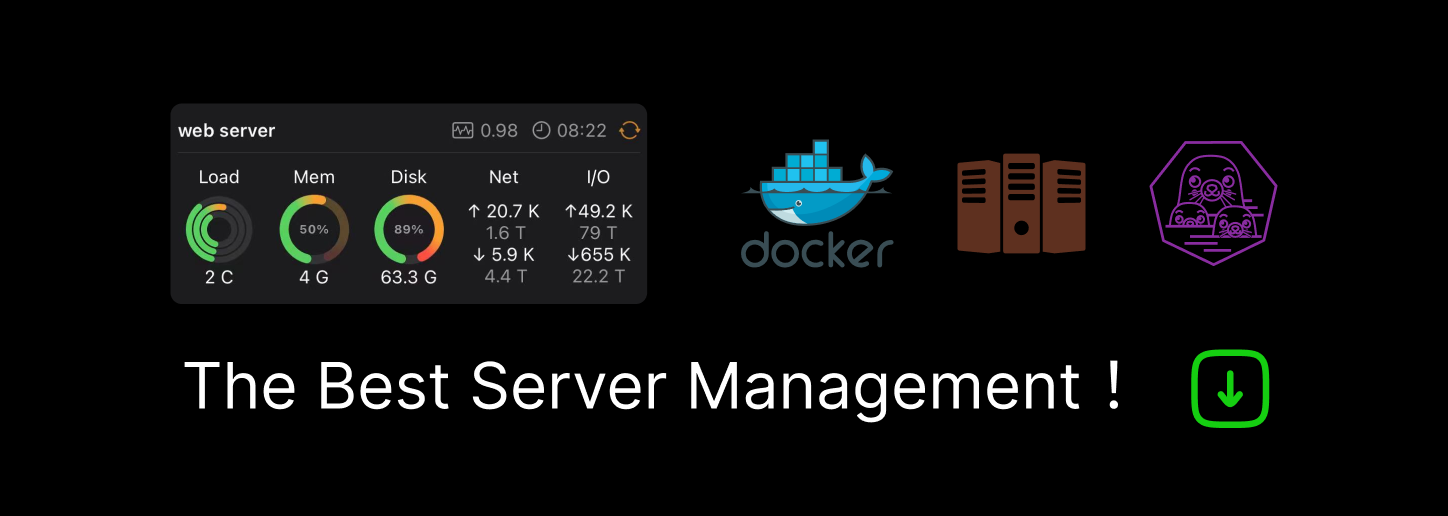
In this article, we will implement a spiral print of 2D matrices. This particular problem has already been asked about in interviews many times. To solve this problem, one must visualize what’s going on with the matrix. First, let’s have a look at the problem statement and see what does spiral print means?
What Is Spiral Reading?
You are given a two-dimensional matrix. Print the matrixes in such a way that the output forms a spiral in the clockwise direction.
For example,
|*********************|*********************|*********************|
Matrix:
|a b c|
|d e f|
|g h i|
Output:
a b c f i h g d e
|*********************|*********************|*********************|
Matrix:
|a b c d |
|e f g h |
|i j k l |
|l m n o|
Output:
a b c d h l o n m l i e f g k j
|*********************|*********************|*********************|
Let us now move towards the conceptual and implementation part:

Concept of Spirally Reading Through a 2D Matrix
We would follow the following steps to code the algorithm.
- We would use four variables to iterate over the matrix.
- Initialize start_row = 0, end_col = N – 1, end_row = M – 1 and the start_col = 0
- Now, start by printing the start-row, once it’s done, just update the value as
- start_row++
- Next, we would print the end column
- end_col–
- Then we move to the end row, print it
- We would print this row only if(start_row < end_row)
- Then update the pointer as end_row–
- And in the end, we print the first column
- Only if(start_col < end_col)
- first_col++
- Once these steps are over, we repeat the same steps but for the inner rows and columns
- Notice that, we are again left with a similar problem to solve
- We will do this until the following condition is true
- if(start_row <= end_row && start_col <= end_col)
Algorithm for Spiral Printing Numbers
void
spiral_print(
int
a[][1000],
int
m,
int
n)
{
// initialize the variables
int
start_row = 0;
int
start_col = 0;
int
end_row = m - 1;
int
end_col = n - 1;
// we will print the array until we
// hit some base case
// when do we stop?
// we should not let the start row
// exceed the end row
// or the start column exceed the
// end column
while
(start_row <= end_row && start_col <= end_col)
{
// first row
// in the first row only the columns are
// going to change
for
(
int
i = start_col; i <= end_col; i++)
cout << a[start_row][i] <<
" "
;
// update the value of the start_row
start_row++;
// print the end_column
for
(
int
i = start_row; i <= end_row; i++)
cout << a[i][end_col] <<
" "
;
// take this pointer, one step back
end_col--;
// print the last_row now
// this addition if condition prevents the
// repetition of the middle most row
if
(start_row < end_row)
{
for
(
int
i = end_col; i >= start_col; i--)
cout << a[end_row][i] <<
" "
;
end_row--;
}
// time to print the start_column
// this additional if conditions prevents
// the repetition of the middle most column
if
(start_col < end_col)
{
for
(
int
i = end_row; i >= start_row; i--)
cout << a[i][start_col] <<
" "
;
start_col++;
}
}
cout << endl;
return
;
}

Read Through 2D Matrices Spirally Using C++
#include <iostream>
using
namespace
std;
void
spiral_print(
int
a[][1000],
int
m,
int
n)
{
// initialize the variables
int
start_row = 0;
int
start_col = 0;
int
end_row = m - 1;
int
end_col = n - 1;
// we will print the array until we
// hit some base case
// when do we stop?
// we should not let the start row
// exceed the end row
// or the start column exceed the
// end column
while
(start_row <= end_row && start_col <= end_col)
{
// first row
// in the first row only the columns are
// going to change
for
(
int
i = start_col; i <= end_col; i++)
cout << a[start_row][i] <<
" "
;
// update the value of the start_row
start_row++;
// print the end_column
for
(
int
i = start_row; i <= end_row; i++)
cout << a[i][end_col] <<
" "
;
// take this pointer, one step back
end_col--;
// print the last_row now
// this addition if condition prevents the
// repetition of the middle most row
if
(start_row < end_row)
{
for
(
int
i = end_col; i >= start_col; i--)
cout << a[end_row][i] <<
" "
;
end_row--;
}
// time to print the start_column
// this additional if conditions prevents
// the repetition of the middle most column
if
(start_col < end_col)
{
for
(
int
i = end_row; i >= start_row; i--)
cout << a[i][start_col] <<
" "
;
start_col++;
}
}
cout << endl;
return
;
}
int
main()
{
int
a[1000][1000] = {0};
int
m, n;
cout <<
"Enter the values of rows and columns respectively"
<< endl;
cin >> m >> n;
cout <<
"Enter the elements"
<< endl;
for
(
int
i = 0; i < m; i++)
{
for
(
int
j = 0; j < n; j++)
{
int
ele;
cin >> ele;
a[i][j] = ele;
//cin >> a[i][j];
}
}
spiral_print(a, m, n);
return
0;
}
Output

Conclusion
In this article, we learned to print two-dimensional matrices in the clockwise spiral order. The approach is simple, one should try to visualize the scenario first and then write the code. That’s all for today, thanks for reading.
Further Readings
If you want to learn about vectors or linked lists, do check out the following articles
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK