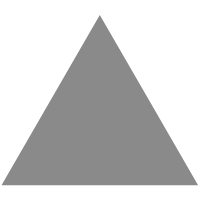
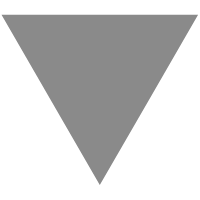
Github Copilot With C# .NET
source link: https://dotnetcoretutorials.com/2021/10/30/github-copilot-with-c-net/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
On the 29th of June 2021, Github Copilot was released to much fanfare. In the many gifs/videos/screenshots from developers across the world, it showed essentially an intellisense on steroids type experience where entire methods could be auto typed for you. It was pretty amazing but I did wonder how much of it was very very specific examples where it shined, and how much it could actually do to help day to day programming.
So off I went to download the extension and….
Access to GitHub Copilot is limited to a small group of testers during the technical preview of GitHub Copilot. If you don’t have access to the technical preview, you will see an error when you try to use this extension.
Boo! But that’s OK. I thought maybe they would open things up fairly quickly. So I signed up at https://copilot.github.com/
Now some 4 months later, I’m finally in! Dreams can come true! If you haven’t yet, sign up to the technical preview and cross your fingers for access soon. But for now you will have to settle for this little write up here!
What do I want to test? Well of the many examples I saw of Github Copilot, I can’t recall any being of C# .NET. There may be many reasons for that, but it does interest me because I think over the course of years, the style in which we develop C# has heavily changed. Just as an example, prior to .NET 4.5, we didn’t have the async/await keywords. But it would almost be impossible to find C# code written today that didn’t use asynchronous programming in one way or another. But if Github Copilot is using the full gamut of C# code written in the past 20 years, it may well fall into bad habits of yesteryear.
Let’s try it out anyway!
Add Two Numbers Together
My first attempt at using Copilot was a simple test. Adding two numbers together. Here’s how Copilot shows you what it thinks you want to do :
You can then press tab to accept the intellisense, much like you normally would in Visual Studio. There can be a bit of a lag between typing and the suggestions showing. It doesn’t “feel” like intellisense like you would with Visual Studio because of this. But it’s still responsive if you want to stop for a second or two and see what it thinks you should do.
Fetch Web Page Contents
Next, I wanted to see if I could trip it up a little by using an async method, but the actual logic was simple. All I wanted to do was to fetch a webpage, and return the string contents. Here’s what it gave me :
Now again, not rocket science, but pretty impressive. It knew that the method itself was async (So it could use await), and that we were using a string so it should return the entire contents.
Let’s say I wanted to get fancy though. Instead of returning the string contents of the page, I want to use HtmlAgilityPack (A common HTML parser) to return the parsed HtmlDocument. Now the thing here is, I 100% thought it was going to load the webpage as normal, and then try and parse it into a document. But instead :
Apparently HtmlAgilityPack has a class called “HtmlWeb” that does this for you. I never would have even known this without Github Copilot. This impressed the bejesus out of me because while yes, it’s a pretty common task, it’s also a third party library that I thought for sure would trip it up.
Code From Comments
One of the most impressive things I saw in Copilot demos was the ability to write a comment, and have it understand what code to write based on that comment alone. Again, this is something that I thought for sure would trip it up but instead :
OK, a pretty simple example but it completely blew my mind that from the comment alone, it knew what to do.
So instead, I tried to break it. I started with the following code. It doesn’t give too much away (I think).
static async Task Main(string[] args) { var searchQuery = args[0]; // Go to google and use the search query to find the first result }
And this time, Copilot fed me line by line to end up like so :
static async Task Main(string[] args) { var searchQuery = args[0]; // Go to google and use the search query to find the first result var url = $"https://www.google.com/search?q={searchQuery}"; var client = new HttpClient(); var html = await client.GetStringAsync(url); // Find the first result var startIndex = html.IndexOf("<h3 class=\"r\">"); var endIndex = html.IndexOf("</h3>", startIndex); var result = html.Substring(startIndex, endIndex - startIndex); // Print the result Console.WriteLine(result); }
Again, All I did was accept every suggestion by pressing Tab over and over, I did not modify this code at all. And it’s actually pretty good! There’s probably better ways to do this with Regex and/or HtmlAgilityPack, but this is actually pretty nice.
Algorithm Code
This is where I think I’ve seen Copilot shine the most. If you know “I need the algorithm that does XYZ”, Copilot is pretty dang efficient in whipping those up for you.
Here it is writing a quick Bubblesort for you based off nothing but the method name and input :
Amazing!
Sometime back, I wrote about the knapsack algorithm (https://dotnetcoretutorials.com/2020/04/22/knapsack-algorithm-in-c/). Mostly I was fascinated because I had seen the problem come up a lot in programming competitions and I always got stuck. So I took my code from there and started typing it as I did there and…
Legitimately, I think this thing could break programming competitions at times. Imagine the common “Traveling Salesman Problem” or pathfinding as part of a competition. You could almost just write the problem as a comment and let Copilot do the rest.
Github Copilot Final Verdict
I’ll be honest, I came into this being fairly sceptical. Having never had my hands on the actual tool, I had read a lot of other news articles that were fairly polarising. Either Copilot is the next great thing, or it’s just a gimmick. And I have to admit, I think Copilot really does have a place. To me, it’s more than a gimmick. You still need to be a programmer to use it, it’s not going to write code for you, but I think some people out there are really under selling this. It’s pretty fantastic. Be sure to sign up to the technical preview to get your hands on it and try it out for yourself!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK