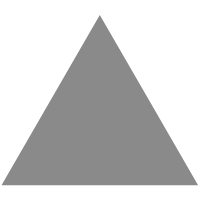
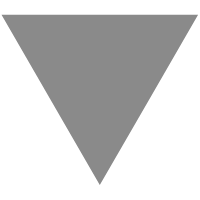
Azure App Configuration: Place for all application configuration
source link: https://dotnetgik.com/2021/10/30/azure-app-configuration-a-central-store-for-your-configuration/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
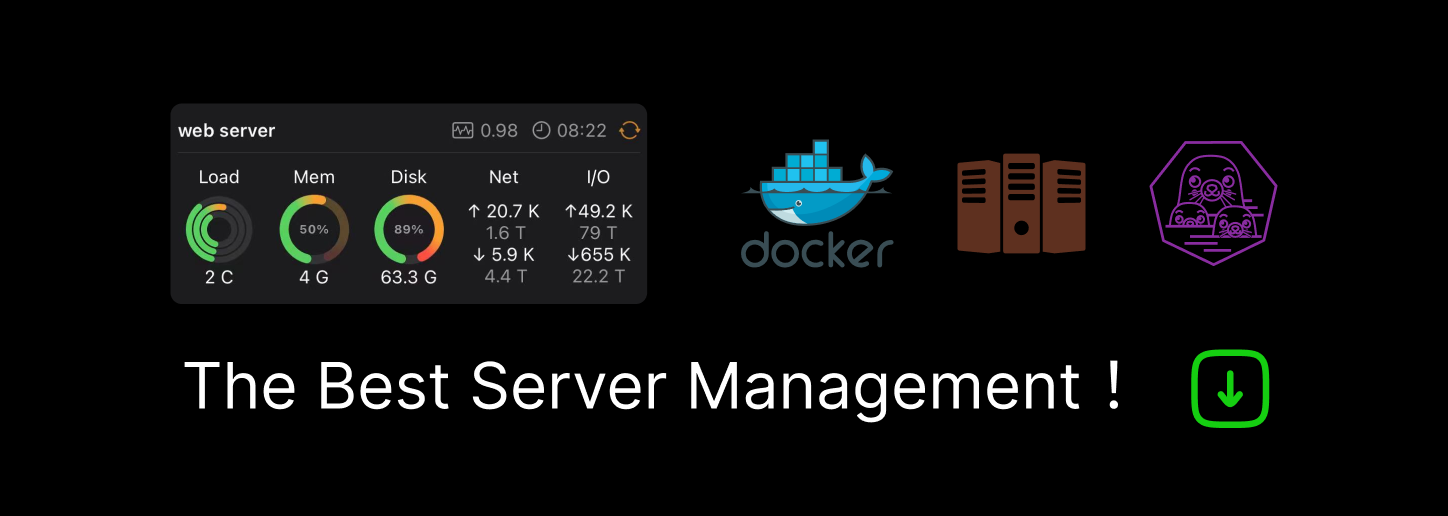
Azure App Configuration: Place for all application configuration
In Day to day development, we use configuration to store various things right from the database connection string to the other backend services that our application use. It can also contain credentials to the external services.
.Net 5.0 provides various configuration providers these providers read the data from key-value pairs using various configuration sources like appsettings.json, Azure key vault and one of the providers in that list is the Azure App Configuration. In this article let’s try to understand what Azure App configuration offers what are the advantages.
Azure App Configuration
Azure App Configuration is a service that provides the central location to manage the application settings and feature flags. Modern-day applications especially the applications running on the cloud can have many components like Virtual machines, serverless components, Container instances, App services. Imagine distributing the configuration settings across these components the situation will be like below
One of the key considerations while designing and using the distributed applications is that Configuration should be kept separate from code, It should be kept external to its executable and should be read from some external sources.
So with the Azure App configuration, we can centrally manage the configuration data for various components and regions. another thing that we can achieve is to dynamically change the application settings without the need to redeploy our application.
Azure App Configuration and .Net 5.0
In this section let’s see how can we connect the Azure app configuration with our .Net core app. We basically follow simple steps which can be summarised like below
- Create a Azure Subscription
The first step to start using the Azure app configuration is to create the Azure subscription. we can follow this article this will guide you for creating the subscription and getting started with Azure
2. Create Azure Configuration Store
To create the Azure configuration store first we need to sign in to Azure portal on the search bar type App configuration it will display following screen
App configuration
Once you select that auto complete option you will land on the following page which will allow you to create the app configuration
Once we click the create app application we will be asked for following details
Subscription details
Once we are on this page we need to fill the details as our azure setup the settings can be described as follows
Once we have added above settings creation wizard we can use Review + Create to validate the config store and deploy it . Once deployment is done . we can see following screen where we can add our configurations now
Now our deployment is done , Click on the Access Keys from the left side menu and note the primary connection string like below

we will need this connection string so as to connect our Api with the configuration store
Now for testing lets add one settings by following the steps in the below diagram

Once we are done with the adding of the Test settings lets check how can we connect and use this configuration store in our code for this we will create a .Net core 5.0 API project
3. Create a .Net 5.0 Web API and Connect to Azure App Configuration
Lets create the API application by following the steps shown in section below

Once we are done with the name of the project and solution we can set the Target framework and other needed things like below. click on create and it will create an API application for us .
Enough with this setup and clicking now

Install Package
Install-Package Microsoft.Extensions.Configuration.AzureAppConfiguration -Version 4.5.0
Add User Secrets using Secret Manager
This step will be needed so as to store the App config store connection string securely in the local environment , to add this lets run following command on the developer command prompt
dotnet user-secrets init
dotnet user-secrets
set
"ConnectionStrings:AzureAppConfigurationEndPoint"
"<Change Your primary connection string copied in Above section>"
Note : This secret manager is used only for local environment but in case of production we can use the ConnectionString Section of the application or the Enviornment variables in the configuration of our deployed application
Application Startup changes
Once we have setup the connection string and package installation its time to do some changes in the program.cs and Startup.cs classes we can replace our program.cs with the code snippet below
using
Microsoft.AspNetCore.Hosting;
using
Microsoft.Extensions.Configuration;
using
Microsoft.Extensions.Hosting;
namespace
AzureAppConfigurationDemo
{
public
class
Program
{
public
static
void
Main(
string
[] args)
{
CreateHostBuilder(args).Build().Run();
}
public
static
IHostBuilder CreateHostBuilder(
string
[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
webBuilder.ConfigureAppConfiguration(AddAppConfigFromAzure).UseStartup<Startup>());
private
static
void
AddAppConfigFromAzure(IConfigurationBuilder config)
{
var
appSettings = config.Build();
var
azureConfigStoreConnection = appSettings.GetConnectionString(
"AzureAppConfigurationEndPoint"
);
config.AddAzureAppConfiguration(azureConfigStoreConnection);
}
}
}
In Above code we are just registering the azure app configuration connection in the IConfigurationBuilder , by doing this we register azure config store as a source to read the configuration from.
using
Microsoft.AspNetCore.Builder;
using
Microsoft.AspNetCore.Hosting;
using
Microsoft.Extensions.Configuration;
using
Microsoft.Extensions.DependencyInjection;
using
Microsoft.Extensions.Hosting;
using
Microsoft.OpenApi.Models;
namespace
AzureAppConfigurationDemo
{
public
class
Startup
{
public
Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public
IConfiguration Configuration {
get
; }
// This method gets called by the runtime. Use this method to add services to the container.
public
void
ConfigureServices(IServiceCollection services)
{
services.AddAzureAppConfiguration()
.AddSwaggerGen(c => c.SwaggerDoc(
"v1"
,
new
OpenApiInfo { Title =
"AzureApp Configuration Demo"
, Version =
"v1"
}))
.AddControllers();
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public
void
Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if
(env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
app.UseSwagger();
app.UseSwaggerUI(c => c.SwaggerEndpoint(
"/swagger/v1/swagger.json"
,
"AzureAppConfigurationDemo v1"
));
}
app.UseHttpsRedirection();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
}
}
Above code is the Startup.cs code snippet in this we register the Azure App config store collection to the DI container so that we can use them in the application.
Read the Configuration
So far we have came across by setting up the configuration store and setting up the code to register the config store in the .Net 5 API application now its time to read this config and show the values which we have set in the configuration.
In Order to read the configuration we just add a new API controller in the application and replace that with the following snippet
using
Microsoft.AspNetCore.Http;
using
Microsoft.AspNetCore.Mvc;
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Threading.Tasks;
using
Microsoft.Extensions.Configuration;
namespace
AzureAppConfigurationDemo.Controllers
{
[Route(
"api/[controller]"
)]
[ApiController]
public
class
AppConfigurationDemo : ControllerBase
{
private
readonly
IConfiguration _configuration;
public
AppConfigurationDemo(IConfiguration configuration)
{
_configuration = configuration;
}
[HttpGet(
"GetConfigurationValue"
)]
public
IActionResult GetConfigurationValue()
{
var
demoSetting = _configuration[
"demotest:testsettings"
];
return
Ok(demoSetting);
}
}
}
if we see the code above it is simple code which reads the value from the configuration by using the simple configuration provider.if you start the application and navigate to the URL in above example we can see the output like below
In this we have seen how we can add the Azure config store and use this store in the .Net 5 API project
References
https://zimmergren.net/introduction-azure-app-configuration-store-csharp-dotnetcore/
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK