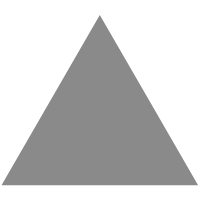
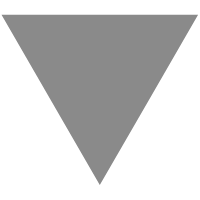
Python中使用函数管道简化数据处理
source link: https://www.jdon.com/72573.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
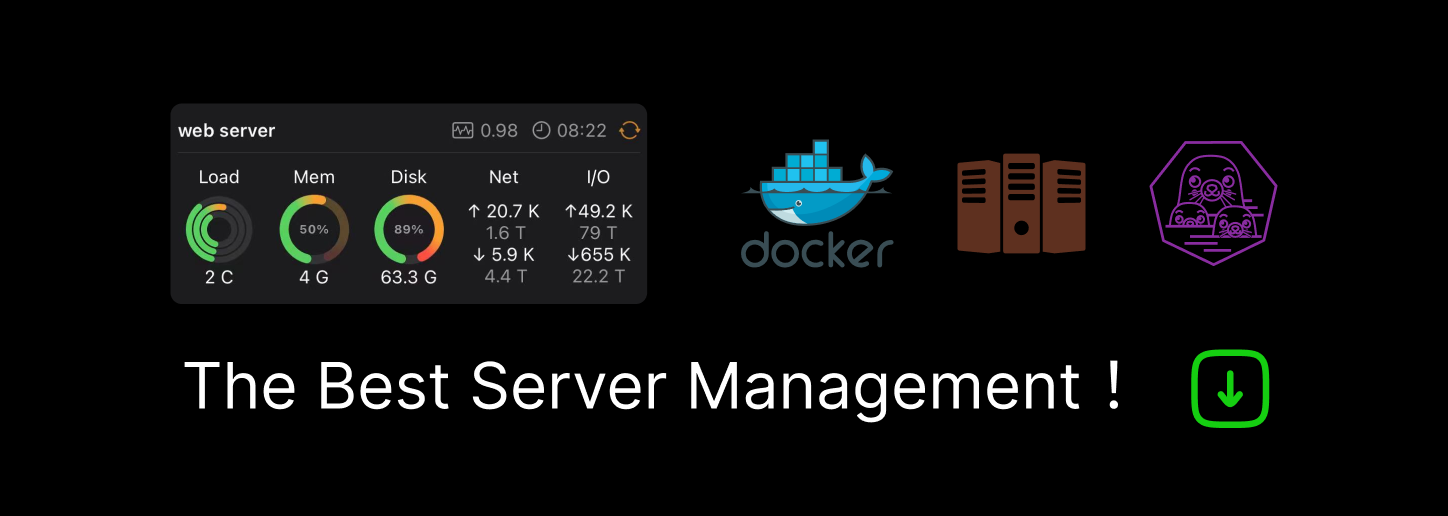
Python中使用函数管道简化数据处理
函数管道允许以顺序方式无缝执行多个函数,其中一个函数的输出作为下一个函数的输入。
什么是函数管道
函数管道允许以顺序方式无缝执行多个函数,其中一个函数的输出作为下一个函数的输入。这种方法有助于将复杂的任务分解为更小、更易于管理的步骤,使代码更加模块化、可读和可维护。函数管道通常用于函数式编程范例中,通过一系列操作来转换数据。他们提倡简洁且函数式的编码风格,强调函数的组合以实现期望的结果。
在 Python 中创建函数管道
在本节中,我们将探讨函数管道的两个实例。在最初的示例中,我们将定义三个函数——“add”、“multiply”和“subtract”——每个函数都设计用于执行基本算术运算,如其名称所暗示的那样。
def add(x, y): |
接下来,创建一个管道函数,该函数可以接收任意数量的函数作为参数,并返回一个新函数。这个新函数将管道中的每个函数依次应用于输入数据。
# 管道将多个函数作为参数,并返回一个内部函数 |
让我们来了解一下管道:
- 管道函数接受任意数量的函数(*funcs)作为参数,并返回一个新函数(inner)。
- 内部函数接受一个参数(数据),代表要由管道函数处理的输入数据。
- 在内部函数中,循环遍历 funcs 列表中的每个函数。
- 对于 funcs 列表中的每个函数 func,内部函数都会将 func 应用于结果变量,而结果变量最初保存的是输入数据。每次函数调用的结果都会成为 result 的新值。
- 管道中的所有函数都应用到输入数据后,内部函数返回最终结果。
接下来,我们创建一个名为 "calculative_pipeline "的函数,将 "add加法"、"multiply乘法 "和 "substract减法 "传递给管道函数。
# Create function pipeline |
然后,我们可以通过管道传递输入值来测试函数管道。
result = calculation_pipeline(10) |
再举一个例子:
def validate(text): |
通过正确的输入对管道进行测试:
# Test the function pipeline
result = str_pipeline("Test@!!!%Abcd")
print(result) # TESTABCD
如果是空字符串或空字符串:
result = str_pipeline("")
print(result) # Error
在示例中,我们建立了一个管道,首先对输入进行验证,确保输入不是空的。如果输入通过了验证,则进入 "remove_special_chars "函数,然后是 "Capitalize "函数。
总结
创建函数管道的好处
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK