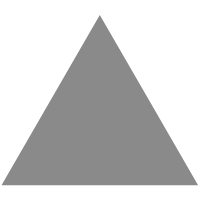
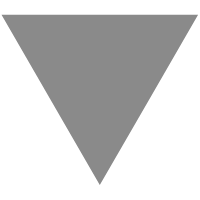
18个JavaScript技巧:编写简洁高效的代码
source link: https://www.51cto.com/article/780655.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
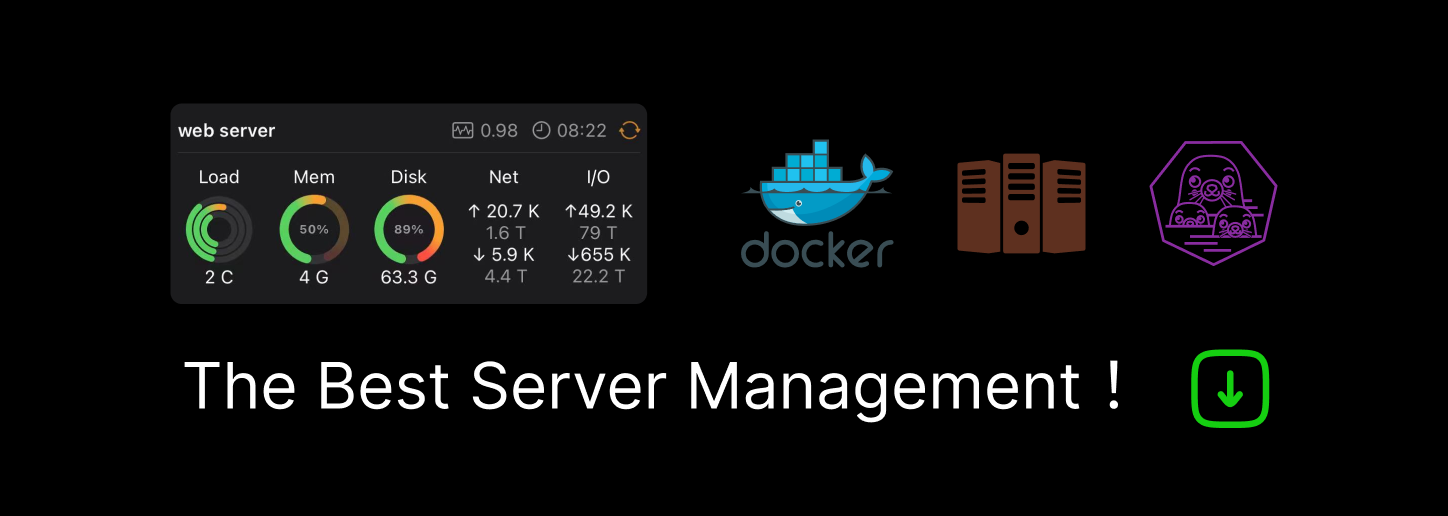
本文翻译自 18 JavaScript Tips : You Should Know for Clean and Efficient Code,作者:Shefali, 略有删改。
在这篇文章中,我将分享18个JavaScript技巧,以及一些你应该知道的示例代码,以编写简洁高效的代码。
让我们开始吧!🚀
可以使用箭头函数来简化函数声明。
function add(a, b) {
return a + b;
}
// Arrow function
const add = (a, b) => a + b;
Array.from()
Array.from()方法可用于将任何可迭代对象转换为数组。
const str = "Hello!";
const arr = Array.from(str);
console.log(arr); //Output: ['H', 'e', 'l', 'l', 'o', '!']
使用console.table显示数据
如果您希望在控制台中组织数据或以表格格式显示数据,则可以使用console.table()。
const person = {
name: 'John',
age: 25,
profession: 'Programmer'
}
console.table(person);
输出效果:

图片
使用const和let
对于不会被重新分配的变量使用const
const PI = 3.14;
let timer = 0;
使用解构提取对象属性
通过使用解构从对象中提取属性,可以增强代码的可读性。
const person = {
name: 'John',
age: 25,
profession: 'Programmer'
}
//Instead of this 👇
console.log(person.name);
console.log(person.age);
//Use this👇
const {name, age} = person;
console.log(name);
console.log(age);
使用逻辑OR运算符设置默认值
使用||操作符轻松设置默认值。
function greet(name) {
name = name || 'Person';
console.log(`Hello, ${name}!`);
}
greet(); //Output: Hello, Person!
greet("John"); //Output: Hello, John!
你可以使用length属性轻松清空数组。
let numbers = [1, 2, 3, 4];
numbers.length = 0;
console.log(numbers); //Output: []
JSON.parse()
使用JSON.parse()将JSON字符串转换为JavaScript对象,这确保了无缝的数据操作。
const jsonStr = '{"name": "John", "age": 25}';
const person = JSON.parse(jsonStr);
console.log(person);
//Output: {name: 'John', age: 25}
Map()函数
使用map()函数转换新数组中的元素,而不修改原始数组。
const numbers = [1, 2, 3, 4];
const doubled = numbers.map(num => num * 2);
console.log(numbers); //Output: [1, 2, 3, 4]
console.log(doubled); //Output: [2, 4, 6, 8]
Object.seal()
您可以使用Object.seal()方法来防止在对象中添加或删除属性。
const person = {
name: 'John',
age: 25
};
Object.seal(person);
person.profession = "Programmer";
console.log(person); //Output: {name: 'John', age: 25}
Object.freeze()
您可以使用Object.freeze()方法来阻止对对象的任何更改,包括添加,修改或删除属性。
const person = {
name: 'John',
age: 25
};
Object.freeze(person);
person.name = "Mark";
console.log(person); //Output: {name: 'John', age: 25}
删除数组重复项
您可以使用Set从数组中删除重复的元素。
const arrWithDuplicates = [1, 12, 2, 13, 4, 4, 13];
const arrWithoutDuplicates = [...new Set(arrWithDuplicates)];
console.log(arrWithoutDuplicates);
//Output: [1, 12, 2, 13, 4]
使用解构交换值
你可以使用解构轻松地交换两个变量。
let x = 7, y = 13;
[x, y] = [y, x];
console.log(x); //13
扩展运算符
您可以使用扩展运算符有效地复制或合并数组。
const arr1 = [1, 2, 3];
const arr2 = [9, 8, 7];
const arr3 = [...arr2];
const mergedArr = [...arr1, ...arr2];
console.log(arr3); //[9, 8, 7]
console.log(mergedArr); //[1, 2, 3, 9, 8, 7]
模板字符串
利用模板文字进行字符串插值并增强代码可读性。
const name = 'John';
const message = `Hello, ${name}!`;
三元运算符
可以用三元运算符简化条件语句。
const age = 20;
//Instead of this👇
if(age>=18){
console.log("You can drive");
}else{
console.log("You cannot drive");
}
//Use this👇
age >= 18 ? console.log("You can drive") : console.log("You cannot drive");
使用===代替==
通过使用严格相等(===)而不是==来防止类型强制转换问题。
const num1 = 5;
const num2 = '5';
//Instead of using ==
if (num1 == num2) {
console.log('True');
} else {
console.log('False');
}
//Use ===
if (num1 === num2) {
console.log('True');
} else {
console.log('False');
}
使用语义化变量和函数名称
为变量和函数使用有意义的描述性名称,以增强代码的可读性和可维护性。
// Don't declare variable like this
const a = 18;
// use descriptive names
const numberOfTips = 18;
今天的内容就到这里,希望对你有帮助。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK