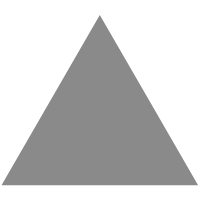
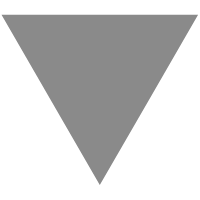
Rust中分割字符串的7种方法
source link: https://www.jdon.com/71818.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
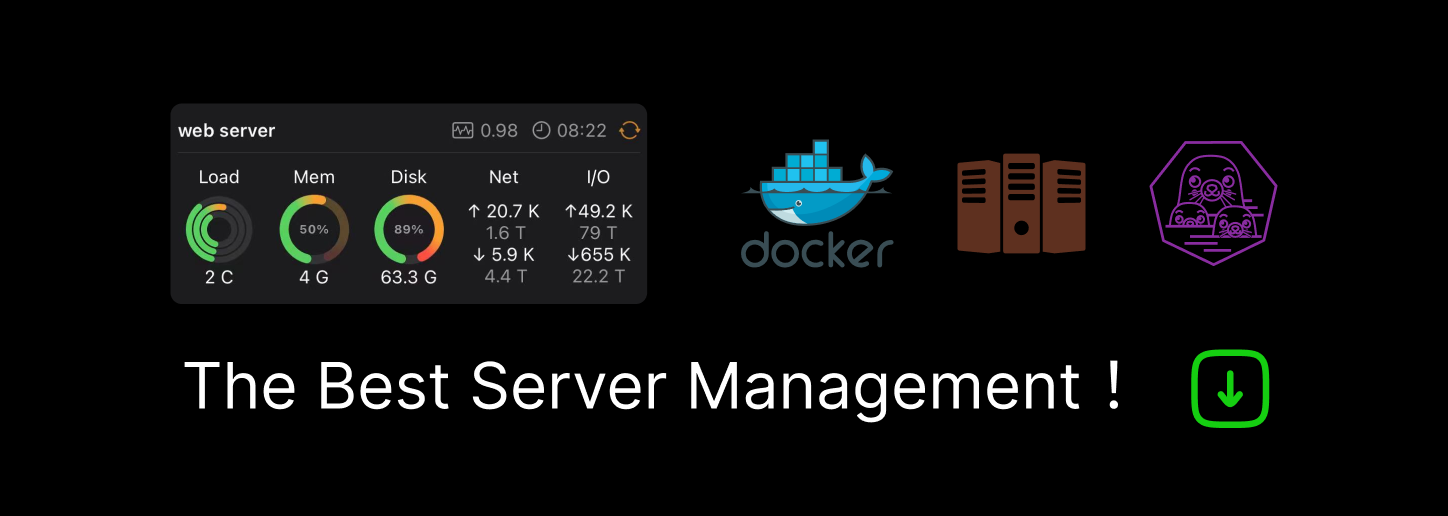
Rust中分割字符串的7种方法
在 Rust 中分割字符串是一项简单的任务,这要归功于该语言强大的标准库。Rust 中的类型str提供了多种方法来以各种方式分割字符串。让我们通过代码示例探索一些常见的方法。
1、按字符分割
分割字符串的最简单方法是使用该split方法按特定字符。它返回子字符串上的迭代器。
fn main() { |
2、按字符串分割
要按字符串模式而不是单个字符进行分割,您可以split轻松地使用该方法。
let text = "apple>>banana>>cherry"; |
3、用闭包分割
对于更复杂的拆分逻辑,您可以传递一个闭包来split确定拆分逻辑。
fn main() { |
4、split_whitespace
split_whitespace方法是一种按空格分割字符串的便捷方法。
fn main() { |
5、split_once
有时,您可能希望在模式第一次出现时将字符串分成两部分。该split_once方法非常适合于此。
fn main() { |
6、拆分并保留结果中的模式
Rust 还允许拆分,而不会忽略结果子字符串中的模式。split_inclusive方法在分割后将模式包含在子串中。
fn main() { |
7、处理空子字符串
请注意,如果存在连续的分割模式,split方法将包含空子字符串。
fn main() { |
为了避免空字符串,您可以使用filter排除它们。
fn main() { |
结论
Rust 提供了多种分割字符串的方法,适应简单和复杂的场景。通过利用这些方法,您可以在 Rust 应用程序中有效地操作和处理字符串。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK