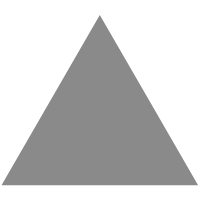
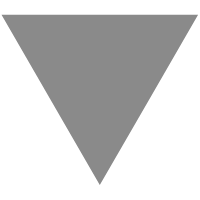
How to create a React application using Redux hooks? Example Tutorial
source link: https://javarevisited.blogspot.com/2022/02/react-tutorial-how-to-create-react-app.html#axzz8NfV7zHxO
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
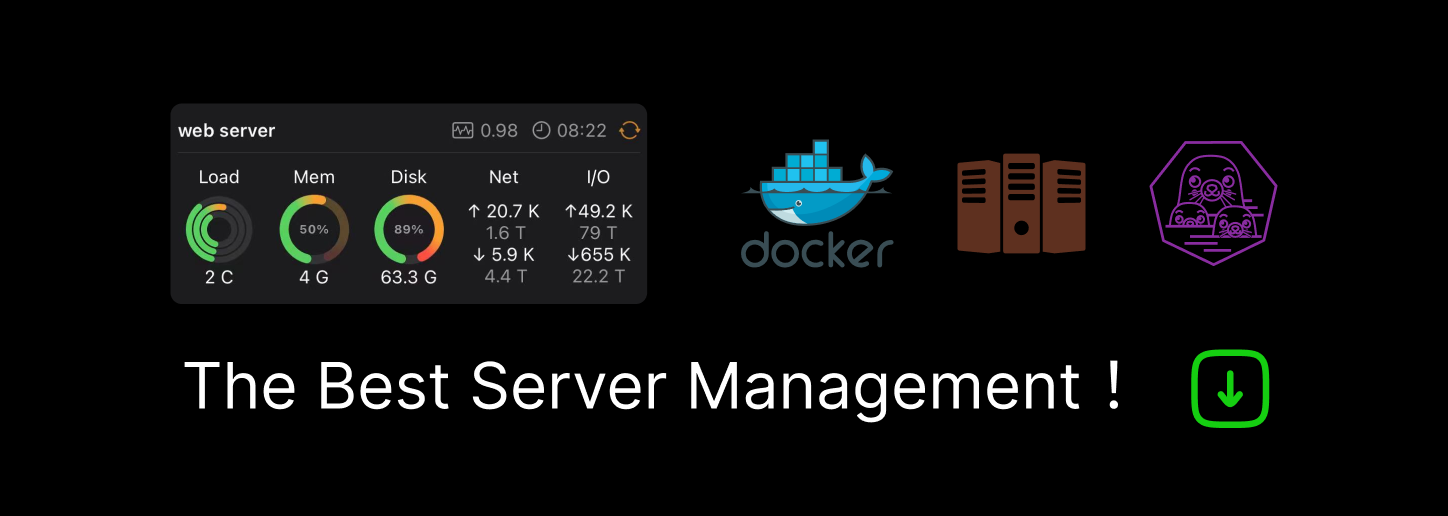
In other world, there are two react-redux hooks - useDisptach and useSelector.
The useDispatch hook returns a reference to the dispatch method from the store. The reference is stored in a variable and then, actions are dispatched using it.
The useSelector hook takes the current state as an argument and returns the global state or part of it.
Working with react-redux hooks
Now, let’s understand how to use both of these hooks with react with the help of an example.
First install, redux, and react-redux using the following commands.
npm install redux
npm install react-redux
First, we need to create a store. Create a new file in the “src“ folder and name it “store.js”. Add the following code to it.
import { createStore } from "redux";
const store = createStore()
export default store;
Next, open the “index.js” file and wrap the App component in the Provider. The store we created earlier must be provided as the value of the “store” attribute.
import React from "react";
import ReactDOM from "react-dom";
import { Provider } from "react-redux";
import App from "./App";
import reportWebVitals from "./reportWebVitals";
import store from "./store";
ReactDOM.rendr(
<Provider store={store}>
<App />
</Provider>,
document.getElementById("root")
reportWebVitals();
We will create three components and these three components will share the global state among them.
Component1:
import React from "react";
const Component1 = () => {
const incrementHandler = () => {};
const decrementHandler = () => {};
return (
<div>
<button onClick={incrementHandler}>Increment</button>
<button onClick={decrementHandler}>Decrement</button>
<hr />
</div>
export default Component1;
Two buttons are defined in the “Component1” - one for incrementing value and the other for decrementing.
Component2:
import React from "react";
const Component2 = () => {
return (
<div>
<h2>
</h2>
</div>
export default Component2;
“Component2” will display the state.
Component3:
import React from "react";
const Component3 = () => {
const colorHandler = () => {
let color;
return color;
return (
<div style={{ backgroundColor: colorHandler(), padding: "20px" }}></div>
export default Component3;
In “Component3”, “count” will be used to determine the color of the div.
We will create two actions - one to increment the value of “count” and the other to decrement it.
Create a new folder in “src” and name it “Actions”. In this folder, create a new file “actionCreators.js”. Add the following code to it.
export const incrementAction = (count) => {
return {
type: "INCREMENT",
payload: count,
export const decrementAction = (count) => {
return {
type: "DECREMENT",
payload: count,
Next, create a new folder “Reducers” and add a new file “reducer.js” to it with the following code.
export const reducer = (state = { count: 0 }, action) => {
switch (action.type) {
case "INCREMENT":
return {
...state,
count: action.payload + 1,
case "DECREMENT":
return {
...state,
count: action.payload - 1,
default:
return { ...state };
The reducer receives the state and action as parameters. Then, according to the “type” of action, the new state is returned. The “payload” is used to make changes in the “count”.
Let’s make some changes to the store.
import { createStore } from "redux";
import { reducer } from "./Reducers/reducers";
const initialState = {
count: 0,
const store = createStore(reducer, initialState);
export default store;
An initial state is added to the store. Now we can use react-redux hooks.
Let’s use the useSelector hook to fetch the global state in “Component2”.
import React from "react";
import { useSelector } from "react-redux";
const Component2 = () => {
const { count } = useSelector((state) => state);
return (
<div>
<h2>{count}</h2>
<hr />
</div>
export default Component2;
In the above component, “count” is extracted from the global state using the useSelector hook.
Similarly, we can use the useSelector hook to extract “count” from the global state in “Component3”
import React from "react";
import { useSelector } from "react-redux";
const Component3 = () => {
const { count } = useSelector((state) => state);
const colorHandler = () => {
let color = count > 0 ? "green" : count < 0 ? "red" : "grey";
return color;
return (
<div style={{ backgroundColor: colorHandler(), padding: "20px" }}></div>
export default Component3;
The “count” is used in the “colorHandler” to determine the color of the div.
Now, let’s use the useSelector hook as well as the useDispatch hook in the “Component1”.
import React from "react";
import { useSelector, useDispatch } from "react-redux";
import {
decrementAction,
incrementAction,
} from "../ActionCreators/actionCreators";
const Component1 = () => {
const dispatch = useDispatch();
const { count } = useSelector((state) => state);
const incrementHandler = () => {
dispatch(incrementAction(count));
const decrementHandler = () => {
dispatch(decrementAction(count));
return (
<div>
<button onClick={incrementHandler}>Increment</button>
<button onClick={decrementHandler}>Decrement</button>
<hr />
</div>
export default Component1;
In the “Component1”, first, the reference from the useDispatch hook is stored in a variable and then, the dispatch is used inside the “incrementHandler” and “decrementHandler” to dispatch the actions. We need the “count” because it should be passed to the actions creators and for this, we used the useSelector hook.
Everything is ready. Let’s check the output.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK