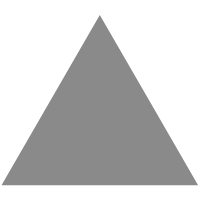
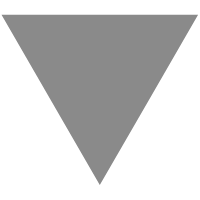
13个你不知道的Python技巧
source link: https://www.51cto.com/article/777240.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
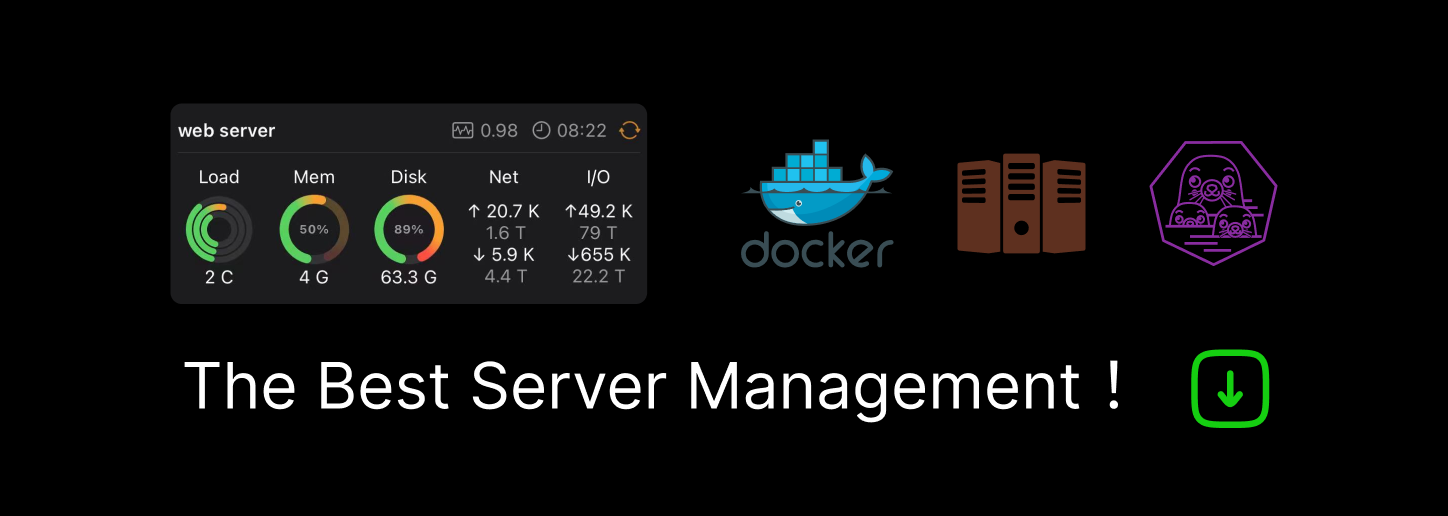
13个你不知道的Python技巧
Python 是顶级编程语言之一,它具有许多程序员从未使用过的许多隐藏功能。本文,我将分享13个你可能从未使用过的 Python 特性。
Python 是顶级编程语言之一,它具有许多程序员从未使用过的许多隐藏功能。本文,我将分享13个你可能从未使用过的 Python 特性。
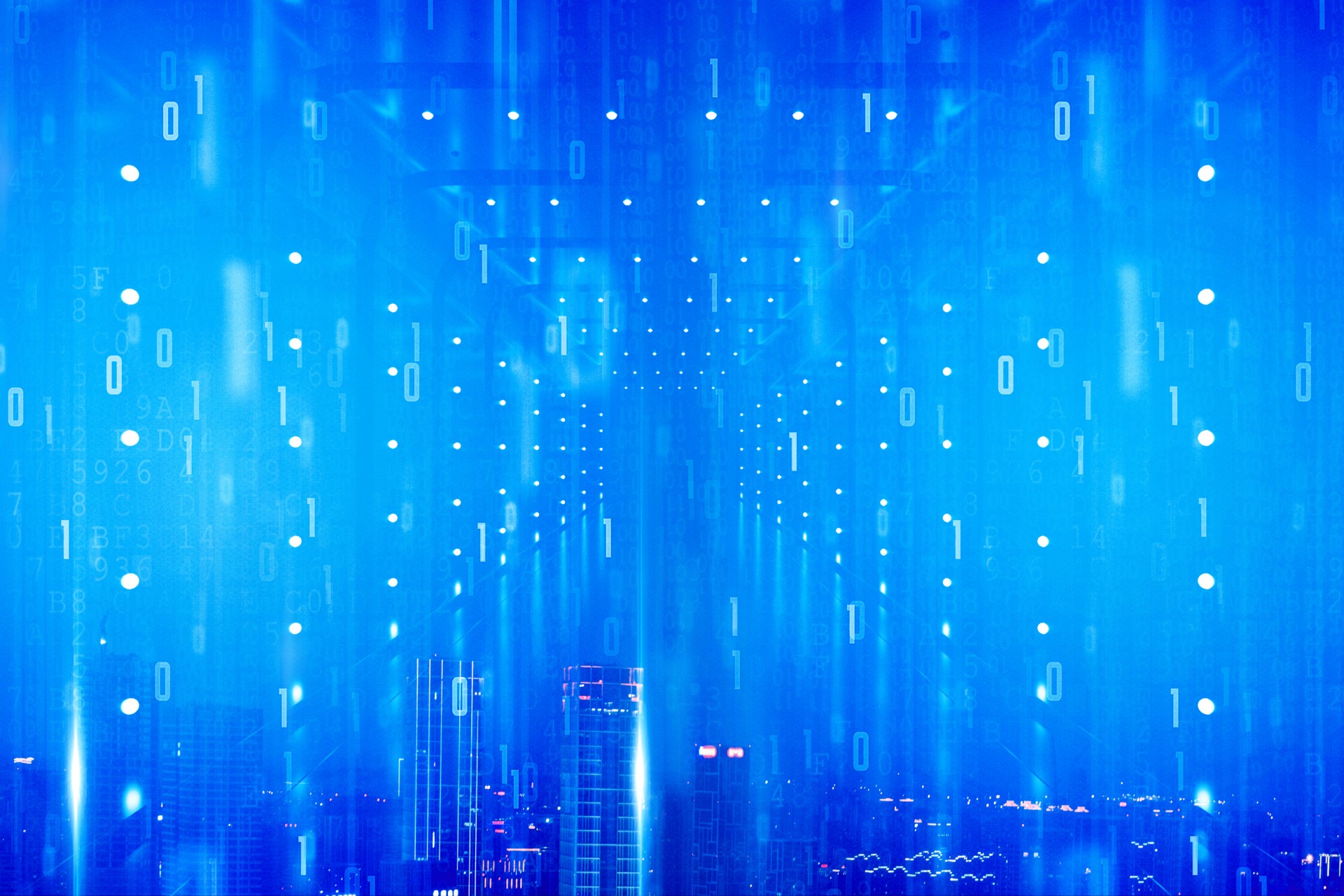
Python 是顶级编程语言之一,它具有许多程序员从未使用过的许多隐藏功能。
本文,我将分享13个你可能从未使用过的 Python 特性。不浪费时间,让我们开始吧。
1. 按步长取数
知识点: list[start:stop:step]
- start: 开始索引, 默认为0
- end: 结束索引, 默认为列表长度
- step: 步长, 默认为1, 可以为负数, 如果为负数, 则为倒序.
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
print(data[::2]) # [1, 3, 5, 7, 9]
print(data[::-1]) # [10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
print(data[2:7:-2]) # [] ⚠️注意:步长为负数时,结果为空
print(data[7:1:-2]) # [8,6,4] # ⚠️ index 属于 [7 -> 1),步长为2。
2. find 方法
知识点:list.find(obj, [start, [stop]])
- list: 列表或者字符串
- obj: 查找的元素
- start: 开始索引, 默认为0
- stop: 结束索引, 默认为列表长度
找不到返回-1
x = "Hello From Python"
print(x.find("o")) # 4
print(x.find("o", 5)) # 8
print(x.find("From Python")) # 6
print(x.find("No exist")) # -1
3. iter() 和 next()
# iter() 函数用于将一个可迭代对象转换为一个迭代器
# next() 函数用于获取迭代器的下一个返回值
values = [1, 3, 4, 6]
values = iter(values)
print(next(values)) # 1
print(next(values)) # 3
print(next(values)) # 4
print(next(values)) # 6
print(next(values)) # StopIteration
4. 测试文档
Doctest 功能将让您测试您的功能并显示您的测试报告。如果您检查下面的示例,您需要在三重引号中编写一个测试参数,其中>>>是固定的语法,你可以增加测试案例,并运行它!如下所示:
# Doctest
from doctest import testmod
def Mul(x, y) -> int:
"""
This function returns the mul of x and y argumets
incoking the function followed by expected output:
>>> Mul(4, 5)
20
>>> Mul(19, 20)
39
"""
return x * y
testmod(name='Mul')
# 输出如下:
"""
**********************************************************************
File "main.py", line 10, in Mul.Mul
Failed example:
Mul(19, 20)
Expected:
39
Got:
380
**********************************************************************
1 items had failures:
1 of 2 in Mul.Mul
***Test Failed*** 1 failures.
"""
5. yield
yield 语句是 Python 的另一个令人惊奇的特性,它的工作方式类似于 return 语句。但它不是终止函数并返回,而是返回到它返回给调用者的地方。
yield 返回的是一个生成器。可以使用 next() 函数来获取生成器的下一个值。也可以使用 for 循环来遍历生成器。
def func():
print(1)
yield "a"
print(2)
yield "aa"
print(3)
yield "aaa"
print(list(func())) ## ['a', 'aa', 'aaa']
for x in func():
print(x)
6. 字典缺失键的处理
dic = {1: "x", 2: "y"}
# 不能使用 dict_1[3] 获取值
print(dic[3]) # Key Error
# 使用 get() 方法获取值
print(dic.get(3)) # None
print(dic.get(3, "No")) # No
7.for-else, while-else
你知道 Python 支持带有 for-else, while-else 吗?这个 else 语句会在你的循环没有中断地运行完后执行,如果中途中断了循环,则不会执行。
# for-else
for x in range(5):
print(x)
else:
print("Loop Completed") # executed
# while-else
i = 0
while i < 5:
break
else:
print("Loop Completed") # Not executed
8. f-string的强大
a = "Python"
b = "Job"
# Way 1
string = "I looking for a {} Programming {}".format(a, b)
print(string) # I looking for a Python Programming Job
#Way 2
string = f"I looking for a {a} Programming {b}"
print(string) # I looking for a Python Programming Job
9. 改变递归深度
这是 Python 的另一个重要特性,它可以让您设置 Python 程序的递归限制。看一下下面的代码示例以更好地理解:
import sys
print(sys.getrecursionlimit()) # 1000 默认值
sys.setrecursionlimit = 2000
print(sys.getrecursionlimit) # 2000
10. 条件赋值
条件赋值功能使用三元运算符,可以在特定条件下为变量赋值。看看下面的代码示例:
x = 5 if 2 > 4 else 2
print(x) # 2
y = 10 if 32 > 41 else 24
print(y) # 24
11. 参数解包
您可以解压缩函数中的任何可迭代数据参数。看看下面的代码示例:
def func(a, b, c):
print(a, b, c)
x = [1, 2, 3]
y = {'a': 1, 'b': 2, 'c': 3}
func(*x) # 1 2 3
func(**y) # 1 2 3
12. 呼唤世界(没啥用)
import __hello__ # 你猜输出啥?
# other code
import os
print(os) # <module 'os' from '/usr/lib/python3.6/os.py'>
13. 多行字符串
此功能将向您展示如何编写没有三重引号的多行字符串。看看下面的代码示例:
# 多行字符串
str1= "Are you looking for free Coding " \
"Learning material then " \
"welcome to py2fun.com"
print(str1) # Are you looking for free Coding Learning material then welcome to Medium.com
# 三重引号字符串
str2 = """Are you looking for free Coding
Learning material then
welcome to py2fun.com
"""
print(str2) #和上面的是不同的,换行也会被输出。
这些就是今天分享的 Python 的 13 个特性,希望你觉得这篇文章读起来有趣且有用。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK