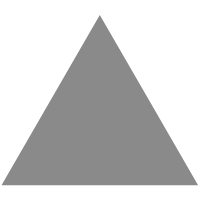
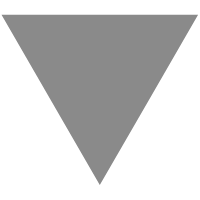
Should you use Bun or Node.js or Deno in 2024?
source link: https://blog.bitsrc.io/should-you-use-bun-or-node-js-or-deno-in-2024-b7c21da085ba
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
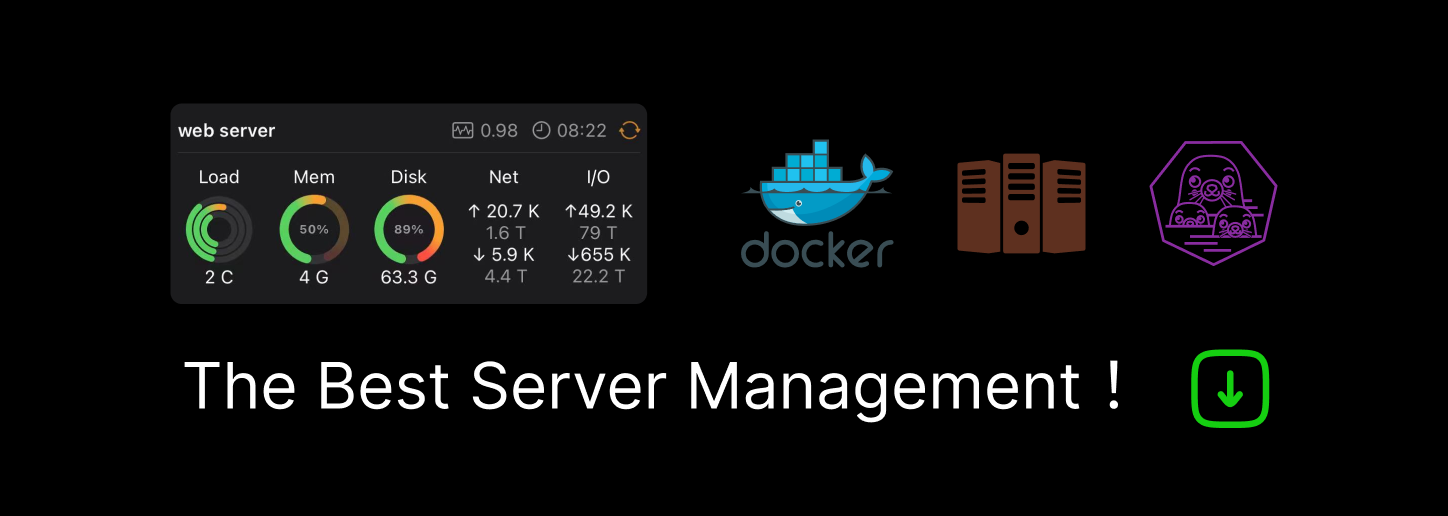
Runtime 01: Node.js

Node.js is a is the most widely use JavaScript runtime for server-side development.
It runs ontop of the JavaScript V8 engine from Google Chrome, ensuring lightning-fast and highly reliable performance. One of the most beneficial aspects on Node.js is the event loop.
The event loop lets you run your entire application on a single thread without facing any blocking. It’s able to intelligently offshore asyncronous blocking operations onto a third party library — libuv that executes all asynchronous I/O operations, and lets the Node.js primary thread process the callbacks when the callstack is free. Additionally, with its introduction of Worker Threads, developers are now able to spin up isolated JavaScript runtimes and emulate multi-threading and parallel processing.
Pros of Node.js for API Development:
- High scalability and performance: Node.js offers increased performance through non-blocking I/O and scalability, supported by an event-driven architecture, making it perfect for real-time, data-intensive applications with growing user bases.
- A mature ecosystem with numerous libraries and frameworks: Node.js features a vibrant ecosystem rich with libraries and frameworks, providing developers with a comprehensive toolkit for efficient coding across web development and real-time applications.
- Large and active community support: Node.js has a dynamic and vibrant community that translates to regular updates and improvements and extensively published modules that developers can easily incorporate into their projects.
Cons of Node.js for API Development:
- Performance limitations due to single-threaded nature: Since Node.js is single-threaded, it’s unsuitable for heavy computing or tasks requiring CPU intensiveness. However, after worker threads have been introduced, Node.js has the capability of executing CPU intensive operations without experience performance issues.
- Callback hell in asynchronous programming: Callback hell is when asynchronous functions in Node.js are so deeply nested within each other that the code becomes complex and confusing, like trying to untangle a bowl of spaghetti. Luckily, this can be avoided by using solutions like Promises and
async
/await
, which helps make the code cleaner and easier to read.
Runtime 02: Deno
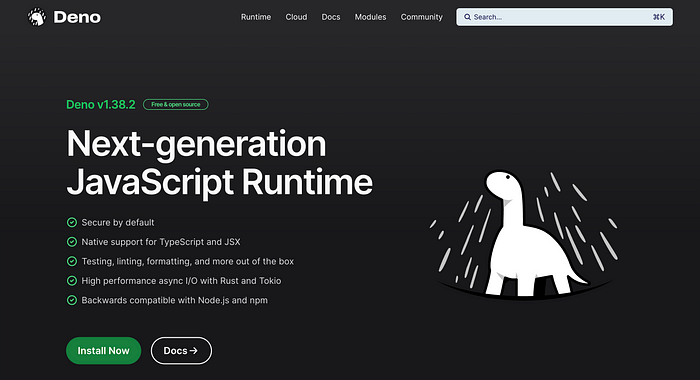
Deno is an emerging JavaScript and TypeScript runtime that strives to address certain shortcomings of Node.js.
Deno prioritizes security by default.
This ensures that your code cannot access files or networks without proper permission. It’s running ontop of the JavaScript V8 engine and has been designed with Rust, meaning that it’s lightening fast!.
Additionally, Deno also adopts current web standards by incorporating built-in utilities like fetch for networking, aligning with how browsers handle JavaScript and delivering a more cohesive coding experience.
Pros of Deno for API Development:
- Built-in security: Deno operates in a secure sandbox environment, requiring explicit permission to access file systems, networks, and the environment, thus reducing the risk of vulnerabilities.
- Improved developer experience: Deno enhances developer workflow with built-in tools such as a dependency inspector and code formatting and offers native TypeScript support, freeing developers to concentrate on coding instead of configuration.
- Simplified module management with URLs: Deno simplifies module management by utilizing URLs to fetch dependencies directly from the web without a package manager, streamlining module resolution within your codebase.
Cons of Deno for API Development:
- Less mature than the Node.js ecosystem: Deno, as a fresher alternative to Node.js, is developing its ecosystem, anticipating growth through community contributions. Developers may currently see fewer ready-made solutions than in Node’s robust ecosystem.
- Limited third-party library availability: While Deno is building momentum, its selection of third-party libraries isn’t as vast as Node.js’s treasure trove. Developers might find themselves on the frontier, sometimes needing to get creative with available resources or even crafting their own. As the Deno landscape develops, the library count will grow, broadening the spectrum of tools for everyone.
Runtime 03: Bun

Bun is an emerging runtime and toolkit that was introduced a couple of months back.
Bun is a fast, all-in-one toolkit for running, building, testing, and debugging JavaScript and TypeScript, from a single file to a full-stack application.
And, with Bun, all you have to do is immediately start using. For instance, you no longer need to install tools like nodemon
, dot-env
because Bun is capable of hot-reloading in developer mode right out of the box, while picking up .env
files by default as well!
Additionally, it offers a built-in websocket server, and uses its own package manager — bunx
that’s five times faster than NPM. But, that’s not all. Bun is not only a JavaScript runtime. It’s an all-in-one toolkit. This means that it offers:
- Bundling
- Package Management
- Testing
Right out of the box!
So, you don’t need to spend time configuring your project, or have to maintain complex boiler plate projects. Instead, you can just spin up a Bun project and get started right away!
Pros of using Bun.js for API development:
- Easy learning curve: Bun is an all-in-one toolkit! This means that you don’t have to spend time learning module bundling, configuring test frameworks. It does this by default. So you can get started quicker!
- Higher Performance: Bun uses the JavaScriptCore Engine while runtimes like Node.js, Deno use the JavaScript V8 Engine. The JavaScriptCore Engine has been optimized for faster startup times and is generally higher performing than the two runtimes.
Cons of using Bun.js or Bun Router for API development:
- Reduced Community Support: Bun was launched a few months ago. Therefore, it still does not have a matured community for questions. So, if you’re somebody that heavily relies on community support, you might have to check for the right support before proceeding.
What’s Better — Node.js, Deno or Bun?
Comparion 01: Performance comparison
Let’s put Bun, Deno, and Nodejs to the test.
We’ll write some memory-hungry math code in JavaScript that crunches big data sets.
Think complex calculations and lots of them.
A classic example is matrix operations. Here’s an example of a matrix multiplication function that flexes its muscles when dealing large matrices.
function generateRandomMatrix(rows, cols) {
const matrix = [];
for (let i = 0; i < rows; i++) {
matrix[i] = [];
for (let j = 0; j < cols; j++) {
matrix[i][j] = Math.random();
}
}
return matrix;
}
function matrixMultiplication(a, b) {
const rowsA = a.length;
const colsA = a[0].length;
const rowsB = b.length;
const colsB = b[0].length;
if (colsA !== rowsB) {
throw new Error("Incompatible matrices for multiplication");
}
const result = new Array(rowsA);
for (let i = 0; i < rowsA; i++) {
result[i] = new Array(colsB).fill(0);
}
for (let i = 0; i < rowsA; i++) {
for (let j = 0; j < colsB; j++) {
for (let k = 0; k < colsA; k++) {
result[i][j] += a[i][k] * b[k][j];
}
}
}
return result;
}
const matrixSize = 1000; // Adjust the size of the matrix to increase memory usage
const matrixA = generateRandomMatrix(matrixSize, matrixSize);
const matrixB = generateRandomMatrix(matrixSize, matrixSize);
console.time("Matrix Multiplication");
const resultMatrix = matrixMultiplication(matrixA, matrixB);
console.timeEnd("Matrix Multiplication");
We have generateRandomMatrix
that creates a random matrix of any size. Then there's matrixMultiplication
that multiplies these matrices.
The fun part? You get to play around with the
matrixSize
variable, deciding the size of these matrices.
As the matrix size grows, you'll notice that memory usage follows suit. Let's see how Bun, NodeJs, and Deno perform with this code.
We'll use a benchmarking tool called hyperfine. Ready to start the benchmark?
Let's run the command and see what happens!
hyperfine "bun index.js" "node index.js" "deno run index.js" --warmup=100 -i
The above shell command will execute the above code in different runtimes, and it will take several minutes to provide the benchmark results.

Feel free to use the above code example and try benchmarks through this link.
Bun’s proficiency in managing memory and CPU-intensive tasks is not merely coincidental. It is designed for speed and optimal performance. Bun proves to be an excellent option if your project requires swiftness and efficiency.
It doesn’t just keep up with Node.js and Deno; it frequently outpaces them. Therefore, considering Bun is a worthwhile choice if you want to build an application capable of providing both speed and efficiency without sacrificing functionality.
Comparison 02: Community Comparison
Community support on the other hand is favorable for runtimes that have been around for a while. For example:
- Node.js: As a seasoned player, Node.js boasts a bustling community. This reflects its long-standing presence and wide acceptance in API development.
- Deno: Deno is quickly carving out its niche. It’s backed by an energetic, forward-thinking community eager to push the boundaries and innovate.
- Bun: The Bun community is relatively small compared to the two. This is mainly due to the fact of it being newer than the two. But, based on the way Bun has been growing, it’s safe to say that it will have a large developer community in no time!
But, Node.js stands out significantly. Its extensive experience in API development has fostered a vibrant and active community. This community of tech enthusiasts is consistently prepared to assist, exchange resources, and collaborate.
Although Bun and Deno are making strides, Node.js’s community remains challenging to surpass.
Thus, if you prioritize a robust support network, Node.js is a reliable choice.
Comparison 03: Security
Node.js, Deno, and Bun each have unique approaches to security. Here’s a straightforward look at how they differ:
- Node.js: It’s open to your system by default, depending on third-party packages that can introduce risks. Tools like
npm audit
help catch vulnerabilities. For example:
npm audit
Plus, using security-focused middleware like helmet
can beef up your Node.js app's defence:
const helmet = require('helmet');
const app = require('express')();
app.use(helmet());
- Deno: It’s like a vault, with scripts locked down tightly unless you explicitly give them permissions. Run a Deno server with limited access like so:
deno run --allow-net=example.com server.ts
- Bun: The new kid on the block aims for speed and provides built-in security features. Yet, it’s more recent, so it might not have been tested through as many security scenarios as the others.
Clearly, Deno takes a highly permissive approach. It’s cautious over the permissions that the app has. It is constructed with security as a top priority, operating within a secure sandbox environment that restricts file and network access unless explicitly authorized.
While Node.js and Bun incorporate their security measures, Deno’s additional layer of built-in security distinguishes it as a top choice for those prioritizing security in API development.
So, if security is your priority, go with Deno!
What should you use? Node.js, Bun or Deno?
There’s no silver bullet. It depends on your priorities. So, use this article as a baseline to compare these JavaScript runtimes.
- Node.js: This is the go-to runtime if you prefer a stable and reliable ecosystem that has been tried and tested over the years.
- Deno: Deno is recommended if you prioritize security and the latest programming environment features. It also supports Typescript out of the box.
- Bun: This runtime should be your preference if you require high speed, especially when working with JavaScript or TypeScript.
Wrapping Up
Choosing the right runtime for your 2024 project may appear daunting, but understanding Bun, Node.js, and Deno can streamline decision-making.
Ultimately, your project’s requirements, reliance on community support, and readiness to engage with documentation may play pivotal roles in determining the most fitting choice.
I hope you found this article helpful.
Thank you for reading.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK