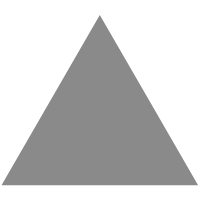
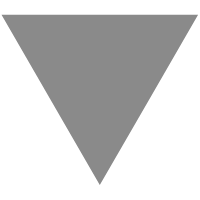
Document Your Python Code and Projects With ChatGPT
source link: https://realpython.com/document-python-code-with-chatgpt/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
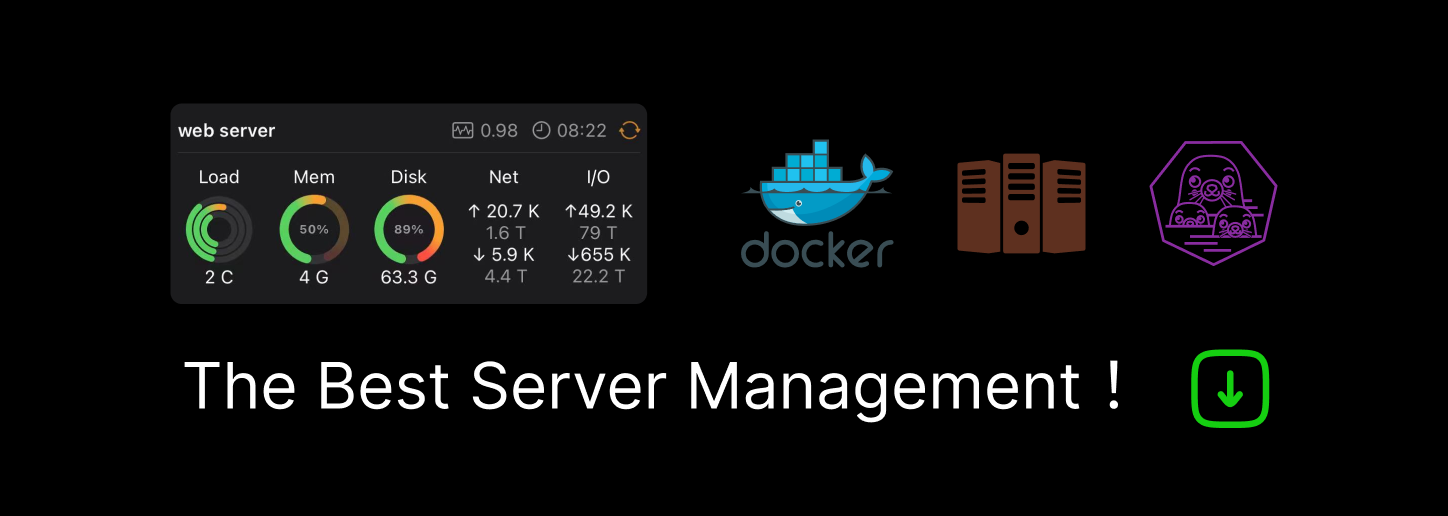
Document Your Python Code and Projects With ChatGPT – Real Python
Benefits of Using ChatGPT for Documenting Python Code
Having high-quality, up-to-date documentation is critical for any software project. Poor documentation can cause a project to fail or go unnoticed even if the codebase is well written and the project’s main idea is innovative and useful.
Writing good documentation takes considerable time and effort. That’s why using large language models (LLMs) like ChatGPT can be a viable alternative for providing your projects and code with proper documentation.
Note: Check out Episode 174: Considering ChatGPT’s Technical Review of a Programming Book of The Real Python Podcast for an interesting conversation about using ChatGPT to run a technical review on existing resources, such as books.
Some of the benefits of ChatGPT for documenting Python code include the following:
With ChatGPT, you can generate cool documentation for your Python code in almost no time. In the following sections, you’ll learn the basics of using ChatGPT as an assistant for creating coherent docstrings and user-friendly external documentation for your Python projects.
Effective ChatGPT Prompts for Writing Docstrings
The primary way to document Python code is through docstrings. In Python, a docstring is typically a triple-quoted string that occupies the first line of modules, functions, classes, and methods. This string has a special meaning for Python, which stores it in an attribute called .__doc__
.
Many Python tools, including code editors and IDEs, take advantage of docstrings to provide real-time help when you’re writing your code. Docstrings are also part of Python’s built-in help system, which you can access with the help()
function:
>>> help(str)
Help on class str in module builtins:
class str(object)
| str(object='') -> str
| str(bytes_or_buffer[, encoding[, errors]]) -> str
|
| Create a new string object from the given object. If encoding or
| errors is specified, then the object must expose a data buffer
| that will be decoded using the given encoding and error handler.
| Otherwise, returns the result of object.__str__() (if defined)
| or repr(object).
| encoding defaults to sys.getdefaultencoding().
| errors defaults to 'strict'.
|
| Methods defined here:
|
| __add__(self, value, /)
| Return self+value.
|
| __contains__(self, key, /)
| Return key in self.
...
In this example, you call help()
with the str
class as an argument, and you get the class’s documentation page, which includes the class’s docstring:
>>> print(str.__doc__)
str(object='') -> str
str(bytes_or_buffer[, encoding[, errors]]) -> str
Create a new string object from the given object. If encoding or
errors is specified, then the object must expose a data buffer
that will be decoded using the given encoding and error handler.
Otherwise, returns the result of object.__str__() (if defined)
or repr(object).
encoding defaults to sys.getdefaultencoding().
errors defaults to 'strict'.
In this case, you get the class’s docstring by accessing the .__doc__
attribute directly on the str
class. As you can conclude, docstrings add a lot of value to your code. They’re the primary documentation that you and other Python developers will use to learn about any Python object.
You can also take advantage of your code’s docstrings when you’re building project documentation with a tool like Sphinx or MkDocs. These tools have plugins and features that allow you to extract the docstrings and make them part of your project’s external documentation, which can save you a lot of time.
Python has well-established conventions for writing good docstrings. Package, module, class, method, and function docstrings all have specific goals and should follow specific guidelines. You can find these guidelines and conventions in PEP 257.
Note: To dive deeper into documenting Python projects, check out Documenting Python Code: A Complete Guide.
Although PEP 257 provides a standard, you’ll actually find a healthy variety of docstring styles across the Python ecosystem. Here are a few common alternatives:
- Google-style docstrings: This style comes from Google, and you’ll find many examples in the company’s open-source projects.
- NumPy docstring standard: This style was developed for the NumPy library, and many other Python projects have adopted it.
- reStructuredText (RST) or Sphinx docstring format: This style is based on reStructuredText and comes from Sphinx, which is a useful tool for automating documentation creation.
- Epytext style: This is a lightweight markup language used to format docstrings, originally created by the Epydoc project.
Each style has its own conventions. Generally, you should choose one style and use it consistently throughout your Python project.
In practice, choosing a docstring style is mostly a matter of personal preference. However, you should also consider the specific needs of your project, including the following characteristics:
- Your project’s complexity: If you have a large and complex project, then you may benefit from the Sphinx or NumPy style, which allows for detailed documentation. In contrast, small projects may just require basic documentation, and something like PEP 257 or Google-style docstrings will be okay.
- Your documentation tools: If you use something like Sphinx or MkDocs to generate your project’s documentation, then you should use the style that has the best support in your tool of choice.
With this short background on docstrings in Python, you’re ready to start prompting ChatGPT to write some docstrings for you.
Using the Target Code as Part of Your Prompts
The quickest way to create prompts for writing docstrings is to include the target code as part of the prompt itself. Consider the following example, where you create a prompt for generating the docstring of a function that adds two numbers together:
You: Write a single-line docstring for the following function:
Python
def add(a, b): return a + b
ChatGPT:
Python
def add(a, b): """Return the sum of a and b.""" return a + b
In this prompt, you ask ChatGPT to write a single-line docstring for your function. The result is quite straightforward and readable. This type of docstring doesn’t provide detailed information about the arguments and the return value of your function.
You can make your prompt a bit more detailed and ask for a specific docstring style. The following prompts make ChatGPT generate docstrings for your add()
function using Google, NumPy, and Sphinx styles, respectively:
You: Write a Google-style docstring for the following function:
Python
def add(a, b): return a + b
ChatGPT:
Python
def add(a, b): """Sum two numbers. Args: a (int or float): The first number. b (int or float): The second number. Returns: int or float: The sum of the two numbers. """ return a + b
You: Write a NumPy-style docstring for the following function:
Python
def add(a, b): return a + b
ChatGPT:
Python
def add(a, b): """ Sum two numbers. Parameters ---------- a : int or float The first number to be added. b : int or float The second number to be added. Returns ------- int or float The sum of a and b. """ return a + b
You: Write a Sphinx-style docstring for the following function:
Python
def add(a, b): return a + b
ChatGPT:
Python
def add(a, b): """ Calculate the sum of two numbers. :param a: The first number. :type a: int or float :param b: The second number. :type b: int or float :return: The sum of the two numbers. :rtype: int or float """ return a + b
These three docstrings look more complete and polished than the single-line docstring that you saw before. When you add information about the specific style that you want to use, ChatGPT can generate complete docstrings for your function. Of course, the initial result may not be perfect, but it’s a good starting point.
Note: ChatGPT is pretty good at formatting and styling docstrings. However, you should always cross-reference the corresponding documentation to make sure that the output is completely compliant with the selected style.
The most important point is that you’ve gotten complete docstrings by using a single, short sentence as a prompt. This is an incredible productivity boost for your project.
ChatGPT not only allows you to generate docstrings for functions. It can also take modules, classes, and so on. Here’s a short example of how to generate a docstring for a Circle
class and its methods:
You: Write NumPy-style docstrings for the following class and its methods:
Python
class Circle: def __init__(self, radius): self.radius = radius def calculate_area(self): return round(math.pi * self.radius ** 2, 2)
ChatGPT:
Python
class Circle: """ A Circle class to perform some circle-related operations. Parameters ---------- radius : float The radius of the circle. Attributes ---------- radius : float The radius of the circle. Methods ------- calculate_area(): Calculates the area of the circle. """ def __init__(self, radius): """ Constructs all the necessary attributes for the circle object. Parameters ---------- radius : float The radius of the circle. """ self.radius = radius def calculate_area(self): """ Calculate the area of the circle. Returns ------- float The area of the circle rounded to 2 decimal places. """ return round(math.pi * self.radius ** 2, 2)
Wow! That was neat! With a single-sentence prompt, you’ve made ChatGPT generate NumPy-style docstrings for your class and all its methods. That’s a great addition to your codebase.
Including Only Signatures in Your Prompts
Sometimes, you can’t use the target code as part of your prompts. This may be due to privacy requirements, or maybe you just want to keep your company’s code safe. In this scenario, you can create prompts that only use the signature of your classes, methods, and functions.
Note: There are lots of ongoing lawsuits around ChatGPT’s use of data. In any case, you should check ChatGPT’s terms of use to make sure the tool is right for you and that you’re using it correctly.
To understand how to create these types of prompts, consider the signature of a function that checks if a number falls within a given interval:
You: Write a Google-style docstring for a function with the following signature:
Python
def in_interval(number, start, end):
ChatGPT:
Python
def in_interval(number, start, end): """ Checks if a given number is within a specified interval. This function takes three arguments: a number, and the start and end points of an interval. It checks whether the given number falls within the specified interval or not. Args: number (int or float): The number to check. start (int or float): The starting point of the interval. end (int or float): The ending point of the interval. Returns: bool: True if the given number falls within the interval, False otherwise. Raises: ValueError: If the start is larger than the end, or if the number, start, or end are not numbers. """
In this example, the function’s signature is the only context that you provide to ChatGPT. The LLM is smart enough to examine the function’s name and arguments and generate a suitable docstring for you. Note that having descriptive function and argument names is key in this kind of prompt. Otherwise, the output may not be good enough.
In most cases, adding type hints to the function’s signature will improve the quality of the generated docstring.
Writing Prompts That Use No Code
You can also craft a prompt by just describing the input and output of your code or by providing a detailed description of what your code does. Again, these types of prompts are useful when you’re not allowed to upload your code as part of your prompt.
To illustrate, here’s a prompt that asks ChatGPT to write a docstring for a function that determines whether an integer number is prime:
You: Write a Google-style docstring for a function that takes an integer as an argument and determines whether it’s a prime number.
ChatGPT:
Python
def is_prime_number(n): """ Determines if an input integer is a prime number. This function takes an integer as an argument and checks whether it is a prime number. A prime number is a positive natural number greater than 1 that has no positive integer divisors other than 1 and itself. Args: n (int): The integer to check. Returns: bool: True if the input integer is a prime number, False otherwise. Raises: ValueError: If n is less than or equal to 1. """
This docstring is quite complete and useful. It even includes an explanation of what a prime number is, which is great! You can tweak it further and polish a few details. However, you already have a great starting point.
Asking for Tests and Usage Examples in Your Prompts
Usage examples are probably the most important part of good software documentation. Through code examples, your users can quickly understand how to use your code and how it works. Often, other developers will scan through your documentation until they find a code example.
So, adding usage examples to your docstrings is a big plus. With ChatGPT, providing usage examples is just a matter of including a short sentence in your prompts. Consider the following prompt and its output:
You: Write a NumPy-style docstring for the following function. Add a section with usage examples as
doctest
tests:Python
def add(a, b): return a + b
ChatGPT:
Python
def add(a, b): """ Add two numbers together. This function takes two numbers (either integers or floats) as arguments and returns their sum. Parameters ---------- a : int or float The first number to add. b : int or float The second number to add. Returns ------- int or float The sum of the two input numbers. Examples -------- >>> add(1, 2) 3 >>> add(5.5, 2.5) 8.0 >>> add(100, -50) 50 """ return a + b
The highlighted lines show the usage examples for your function. In the prompt, you instructed ChatGPT to use the doctest
test format for the examples. This will provide two important assets:
- Usage examples
- Basic tests for your code
That’s neat! You’ve gotten two benefits in one shot! Your documentation has cool usage examples for your user, and at the same time, it has tests that will help you make your code more robust. You can run these tests using the doctest
command.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK