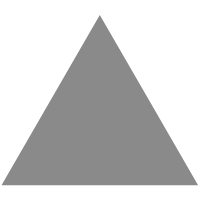
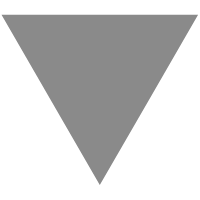
Harnessing Docker for Streamlined Deployment - DZone
source link: https://dzone.com/articles/ai-prowess-harnessing-docker-for-streamlined-deplo
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
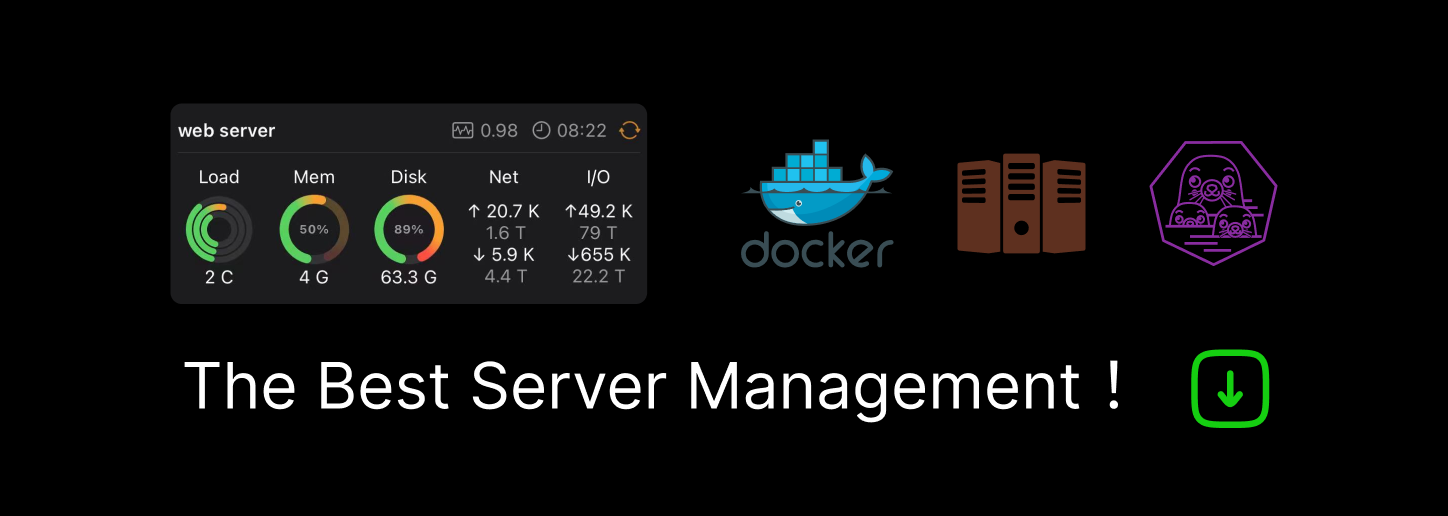
AI Prowess: Harnessing Docker for Streamlined Deployment and Scalability of Machine Learning Applications
Leveraging Docker's power: streamlining deployment solutions, ensuring scalability, and simplifying CI/CD processes for machine learning models.
Machine learning (ML) has seen explosive growth in recent years, leading to increased demand for robust, scalable, and efficient deployment methods. Traditional approaches often need help operationalizing ML models due to factors like discrepancies between training and serving environments or the difficulties in scaling up. This article proposes a technique using Docker, an open-source platform designed to automate application deployment, scaling, and management, as a solution to these challenges. The proposed methodology encapsulates the ML models and their environment into a standardized Docker container unit. Docker containers offer numerous benefits, including consistency across development and production environments, ease of scaling, and simplicity in deployment. The following sections present an in-depth exploration of Docker, its role in ML model deployment, and a practical demonstration of deploying an ML model using Docker, from the creation of a Dockerfile to the scaling of the model with Docker Swarm, all exemplified by relevant code snippets. Furthermore, the integration of Docker in a Continuous Integration/Continuous Deployment (CI/CD) pipeline is presented, culminating with the conclusion and best practices for efficient ML model deployment using Docker.
What Is Docker?
As a platform, Docker automates software application deployment, scaling, and operation within lightweight, portable containers. The fundamental underpinnings of Docker revolve around the concept of 'containerization.' This virtualization approach allows software and its entire runtime environment to be packaged into a standardized unit for software development.
A Docker container encapsulates everything an application needs to run (including libraries, system tools, code, and runtime) and ensures that it behaves uniformly across different computing environments. This facilitates the process of building, testing, and deploying applications quickly and reliably, making Docker a crucial tool for software development and operations (DevOps).
When it comes to machine learning applications, Docker brings forth several advantages. Docker's containerized nature ensures consistency between ML models' training and serving environments, mitigating the risk of encountering discrepancies due to environmental differences. Docker also simplifies the scaling process, allowing multiple instances of an ML model to be easily deployed across numerous servers. These features have the potential to significantly streamline the deployment of ML models and reduce associated operational complexities.
Why Dockerize Machine Learning Applications?
In the context of machine learning applications, Docker offers numerous benefits, each contributing significantly to operational efficiency and model performance.
Firstly, the consistent environment provided by Docker containers ensures minimal discrepancies between the development, testing, and production stages. This consistency eliminates the infamous "it works on my machine" problem, making it a prime choice for deploying ML models, which are particularly sensitive to changes in their operating environment.
Secondly, Docker excels in facilitating scalability. Machine learning applications often necessitate running multiple instances of the same model for handling large volumes of data or high request rates. Docker enables horizontal scaling by allowing multiple container instances to be deployed quickly and efficiently, making it an effective solution for scaling ML models.
Finally, Docker containers run in isolation, meaning they have their runtime environment, including system libraries and configuration files. This isolation provides an additional layer of security, ensuring that each ML model runs in a controlled and secure environment. The consistency, scalability, and isolation provided by Docker make it an attractive platform for deploying machine learning applications.
Setting up Docker for Machine Learning
This section focuses on the initial setup required for utilizing Docker with machine learning applications. The installation process of Docker varies slightly depending on the operating system in use. For Linux distributions, Docker is typically installed via the command-line interface, whereas for Windows and MacOS, a version of Docker Desktop is available. In each case, the Docker website provides detailed installation instructions that are straightforward to follow. The installation succeeded by pulling a Docker image from Docker Hub, a cloud-based registry service allowing developers to share applications or libraries. As an illustration, one can pull the latest Python image for use in machine learning applications using the command:
docker pull python:3.8-slim-buster
Subsequently, running the Docker container from the pulled image involves the docker run command. For example, if an interactive Python shell is desired, the following command can be used:
docker run -it python:3.8-slim-buster /bin/bash
This command initiates a Docker container with an interactive terminal (-it
) and provides a shell (/bin/bash
) inside the Python container. By following this process, Docker is effectively set up to assist in deploying machine learning models.
Creating a Dockerfile for a Simple ML Model
At the heart of Docker's operational simplicity is the Dockerfile, a text document that contains all the commands required to assemble a Docker image. Users can automate the image creation process by executing the Dockerfile through the Docker command line.
A Dockerfile comprises a set of instructions and arguments laid out in successive lines. Instructions are Docker commands like FROM
(specifies the base image), RUN
(executes a command), COPY
(copies files from the host to the Docker image), and CMD
(provides defaults for executing the container).
Consider a simple machine learning model built using Scikit-learn's Linear Regression algorithm as a practical illustration. The Dockerfile for such an application could look like this:
# Use an official Python runtime as a parent image
FROM python:3.8-slim-buster
# Set the working directory in the container to /app
WORKDIR /app
# Copy the current directory contents into the container at /app
ADD . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Run app.py when the container launches
CMD ["python", "app.py"]
The requirements.txt
file mentioned in this Dockerfile lists all the Python dependencies of the machine learning model, such as Scikit-learn, Pandas, and Flask. On the other hand, the app.py
script contains the code that loads the trained model and serves it as a web application.
By defining the configuration and dependencies in this Dockerfile, an image can be created that houses the machine learning model and the runtime environment required for its execution, facilitating consistent deployment.
Building and Testing the Docker Image
Upon successful Dockerfile creation, the subsequent phase involves constructing the Docker image. The Docker image is constructed by executing the docker build
command, followed by the directory that contains the Docker file. The -t
flag tags the image with a specified name. An instance of such a command would be:
docker build -t ml_model_image:1.0
Here, ml_model_image:1.0
is the name (and version) assigned to the image, while '.
' indicates that the Dockerfile resides in the current directory.
After constructing the Docker image, the following task involves initiating a Docker container from this image, thereby allowing the functionality of the machine learning model to be tested. The docker run
command aids in this endeavor:
docker run -p 4000:80 ml_model_image:1.0
In this command, the -p
flag maps the host's port 4000 to the container's port 80 (as defined in the Dockerfile). Therefore, the machine learning model is accessible via port 4000 of the host machine.
Testing the model requires sending a request to the endpoint exposed by the Flask application within the Docker container. For instance, if the model provides a prediction based on data sent via a POST request, the curl
command can facilitate this:
curl -d '{"data":[1, 2, 3, 4]}' -H 'Content-Type: application/json' http://localhost:4000/predict
The proposed method ensures a seamless flow from Dockerfile creation to testing the ML model within a Docker container.
Deploying the ML Model With Docker
Deployment of machine learning models typically involves exposing the model as a service that can be accessed over the internet. A standard method for achieving this is by serving the model as a REST API using a web framework such as Flask.
Consider an example where a Flask application encapsulates a machine learning model. The following Python script illustrates how the model could be exposed as a REST API endpoint:
from flask import Flask, request
from sklearn.externals import joblib
app = Flask(__name__)
model = joblib.load('model.pkl')
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json(force=True)
prediction = model.predict([data['features']])
return {'prediction': prediction.tolist()}
if __name__ == '__main__':
app.run(host='0.0.0.0', port=80)
In this example, the Flask application loads a pre-trained Scikit-learn model (saved as model.pkl
) and defines a single API endpoint /predict
. When a POST request is sent to this endpoint with a JSON object that includes an array of features, the model makes a prediction and returns it as a response.
Once the ML model is deployed and running within the Docker container, it can be communicated using HTTP requests. For instance, using the curl
command, a POST request can be sent to the model with an array of features, and it will respond with a prediction:
curl -d '{"features":[1, 2, 3, 4]}' -H 'Content-Type: application/json'
http://localhost:4000/predict
This practical example demonstrates how Docker can facilitate deploying machine learning models as scalable and accessible services.
Scaling the ML Model With Docker Swarm
As machine learning applications grow in scope and user base, the ability to scale becomes increasingly paramount. Docker Swarm provides a native clustering and orchestration solution for Docker, allowing multiple Docker hosts to be turned into a single virtual host. Docker Swarm can thus be employed to manage and scale deployed machine learning models across multiple machines.
Inaugurating a Docker Swarm is a straightforward process, commenced by executing the 'docker swarm init' command. This command initializes the current machine as a Docker Swarm manager:
docker swarm init --advertise-addr $(hostname -i)
In this command, the --advertise-addr
flag specifies the address at which the Swarm manager can be reached by the worker nodes. The hostname -i
command retrieves the IP address of the current machine.
Following the initialization of the Swarm, the machine learning model can be deployed across the Swarm using a Docker service. The service is created with the docker service create
command, where flags like --replicas
can dictate the number of container instances to run:
docker service create --replicas 3 -p 4000:80 --name ml_service ml_model_image:1.0
In this command, --replicas 3
ensures three instances of the container are running across the Swarm, -p 4000:80
maps port 4000 of the Swarm to port 80 of the container, and --name ml_service
assigns the service a name.
Thus, the deployed machine learning model is effectively scaled across multiple Docker hosts by implementing Docker Swarm, thereby bolstering its availability and performance.
Continuous Integration/Continuous Deployment (CI/CD) With Docker
Continuous Integration/Continuous Deployment (CI/CD) is a vital aspect of modern software development, promoting automated testing and deployment to ensure consistency and speed in software release cycles. Docker's portable nature lends itself well to CI/CD pipelines, as Docker images can be built, tested, and deployed across various stages in a pipeline.
An example of integrating Docker into a CI/CD pipeline can be illustrated using a Jenkins pipeline. The pipeline is defined in a Jenkinsfile, which might look like this:
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
sh 'docker build -t ml_model_image:1.0 .'
}
}
}
stage('Test') {
steps {
script {
sh 'docker run -p 4000:80 ml_model_image:1.0'
sh 'curl -d '{"features":[1, 2, 3, 4]}' -H 'Content-Type: application/json' http://localhost:4000/predict'
}
}
}
stage('Deploy') {
steps {
script {
sh 'docker service create --replicas 3 -p 4000:80 --name ml_service ml_model_image:1.0'
}
}
}
}
}
In this Jenkinsfile, the Build
stage builds the Docker image, the Test
stage runs the Docker container and sends a request to the machine learning model to verify its functionality, and the Deploy
stage creates a Docker service and scales it across the Docker Swarm.
Therefore, with Docker, CI/CD pipelines can achieve reliable and efficient deployment of machine learning models.
Conclusion and Best Practices
Wrapping up, this article underscores the efficacy of Docker in streamlining the deployment of machine learning models. The ability to encapsulate the model and its dependencies in an isolated, consistent, and lightweight environment makes Docker a powerful tool for machine learning practitioners. Further enhancing its value is Docker's potential to scale machine learning models across multiple machines through Docker Swarm and its seamless integration with CI/CD pipelines.
However, to extract the most value from Docker, certain best practices are recommended:
- Minimize Docker image size: Smaller images use less disk space, reduce build times, and speed up deployment. This can be achieved by using smaller base images, removing unnecessary dependencies, and efficiently utilizing Docker's layer caching.
- Use .dockerignore: Similar to .gitignore in Git, .dockerignore prevents unnecessary files from being included in the Docker image, reducing its size.
- Ensure that Dockerfiles are reproducible: Using specific versions of base images and dependencies can prevent unexpected changes when building Docker images in the future.
By adhering to these guidelines and fully embracing the capabilities of Docker, it becomes significantly more feasible to navigate the complexity of deploying machine learning models, thereby accelerating the path from development to production.
References
Recommend
-
111
Allo v22 is testing streamlined new chat UI, preparing to add transcriptions for audio messages and new camera effects [APK Teardown] By Cody...
-
113
XChange XChange is a Java library providing a simple and consistent API for interacting with 60+ Bitcoin and other crypto currency exchanges, providing a consistent interface for trading and accessing market data. Important!...
-
50
README.md
-
41
Release Streamlined and enhanced CLI capabilities · cuelang/cue · GitHub This release introduc...
-
11
Does Inlined Mean Streamlined? Part 1: Escape Analysis Feb 24, 2019 | Richard Startin | javaanalysis
-
24
🤖 Vaults: DeFi Investing Streamlined? Vaults enable easy DeFi investments with a streamlined management of the position for the end-users. What are the options and their tradeoffs?...
-
2
Why You Should Reduce Wait Time and Speed Up Your Deployment Pipeline ...
-
2
AWS announces a streamlined deployment experience for .NET applications by Irene Kors and Norm Johanson | on 06 JUL 2022 | in
-
2
Automating Java Application Deployment Across Multiple Cloud Regions ...
-
2
Ensuring Reliable Microservice Deployment With Spring Boot Build Info Maven Plugin ...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK