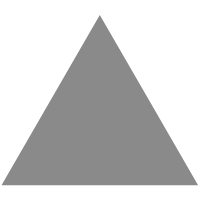
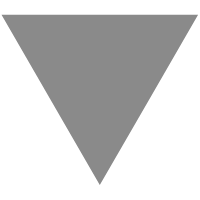
Reliable Microservice Deployment With Spring Boot - DZone
source link: https://dzone.com/articles/microservice-version-verification-with-spring-boot
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Ensuring Reliable Microservice Deployment With Spring Boot Build Info Maven Plugin
Discover how to verify microservice deployments with the Spring Boot Build Info Maven Plugin, increasing the dependability of your deployment pipeline.
In the case of microservices, we faced a unique challenge: when a container was deployed, there was no way for us to verify if the latest container build with the expected code had been deployed. It's easy to verify in cases where a new feature is being deployed and is testable in production. However, with fintech applications, we can't necessarily validate all-new production APIs. There are also instances where a bug fix is applied to an existing API, and you can't execute that API use case in production. There were times when the DevOps team informed us that the container had been lifted and deployed from a lower UAT environment, but we didn't see the bug fixes in action when real production traffic began. To circumvent this, we wanted to ensure that there was a way to validate the latest code when the container was pushed.
In our case, we were dealing with spring-based microservices. That's when the Spring Boot Maven plugin came to the rescue. It's necessary to add the build-info Maven plugin within the project's pom.xml file.
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<executions>
<execution>
<goals>
<goal>
build-info
</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
After configuring the build info, when the project is built, you can view the build-info.properties file under the target/classes/META-INFO directory. It will look something like this:
build.artifact=core-build-info-api
build.group=com.dzone
build.name=core-build-info-api
build.time=2023-07-29T15\:47\:25.387Z
build.version=1.0-SNAPSHOT
To access these properties at runtime, Spring provides the BuildProperties class, which exposes all the properties from the above file. Below is a code snippet for a generic service that constructs the build information for the deployed microservice:
@Service
public class BuildInfoService {
@Autowired
BuildProperties buildProperties;
@Autowired
Environment environment;
public BuildInfoResponse getBuildInfo(){
BuildInfoResponse buildInfoResponse = new BuildInfoResponse();
buildInfoResponse.setName(this.buildProperties.getName());
buildInfoResponse.setVersion(this.buildProperties.getVersion());
buildInfoResponse.setTime(this.buildProperties.getTime());
buildInfoResponse.setActiveProfile(this.environment.getActiveProfiles());
buildInfoResponse.setSpringVersion(this.buildProperties.getVersion());
buildInfoResponse.setGroup(this.buildProperties.getGroup());
buildInfoResponse.setArtifact(this.buildProperties.getArtifact());
return buildInfoResponse;
}
}
You can then inject this service and build a generic endpoint to expose the build information for the container. In our case, we built a common package known as core-build-info, which includes the aforementioned service and a common controller as below:
@RestController
@RequestMapping("/${build-info.path}")
public class BuildInfoController {
@Autowired
BuildInfoService buildInfoService;
@GetMapping("/build-info")
public BuildInfoResponse getBuildInfo() {
return this.buildInfoService.getBuildInfo();
}
}
All the business or utility microservices refer to the common package and configure the following property in the Spring profile properties file:
env=DEV
build-info.path=api/app-name/build
The app name could be your business base service route.
Once the common package has been integrated, you can hit the endpoint for your microservice using the following URL, which returns the following:
{
"name": "core-build-info-api",
"version": "0.0.1",
"time": "2023-07-29T16:13:42.251Z",
"artifact": "core-build-info-api",
"group": "com.dzone",
"activeProfile": [
"DEV"
],
"springVersion": "6.0.10"
}
If you have a version increment configured as part of your build, then you can verify the version. Alternatively, you can always fall back to checking the build time, which in this example is "2023-07-29T16:13:42.251Z".
In conclusion, dealing with microservices and ensuring the latest code deployment can present unique challenges. By leveraging the capabilities of the Spring Boot Maven plugin and creating a common package to expose build information, we have developed a reliable method to overcome this challenge. This process enables us to confirm that the correct version and build of the microservice has been deployed in production, providing confidence and assurance in our deployment pipeline. The real-world value of this approach is evident: it is now the first thing we verify upon deploying a container.
What's in store for Performance and Site Reliability in 2023
Our 2022 Performance and Site Reliability report takes a developer-focused assessment on the current state of observability, distributed tracing, common SRE practices, and lessons learned from failure with a blameless postmortem culture.
You can find the entire codebase on GitHub.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK