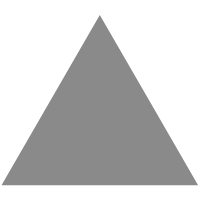
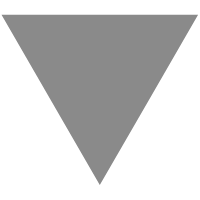
#CAP-TechBytes Input Validation
source link: https://blogs.sap.com/2023/07/07/cap-techbytes-input-validation/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
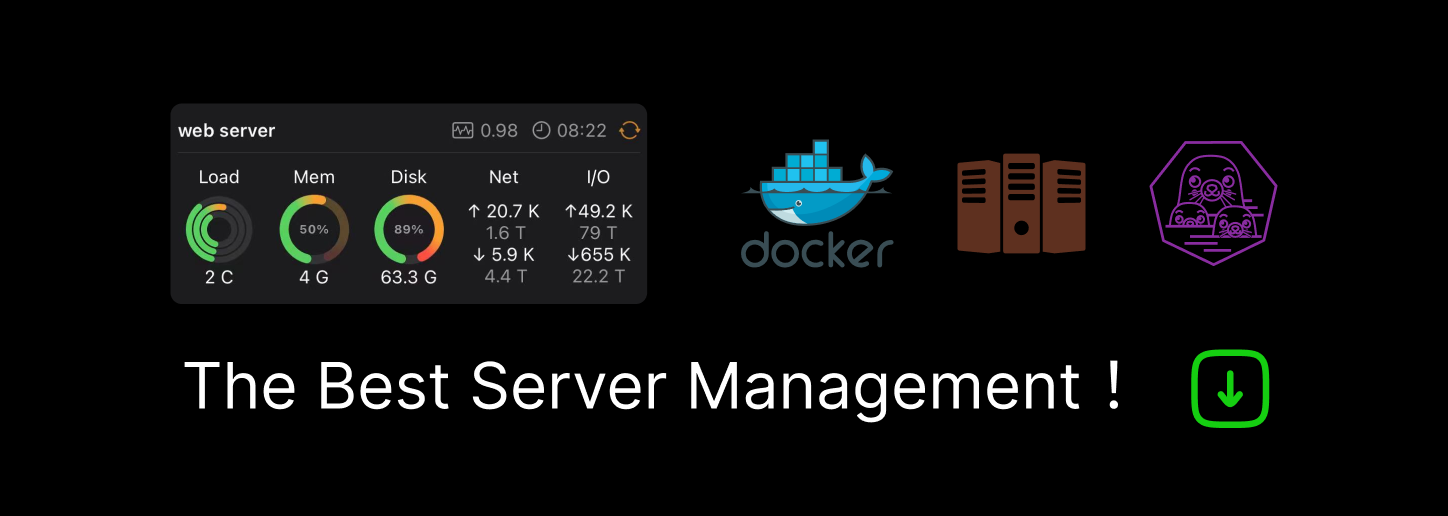
#CAP-TechBytes Input Validation
Input validation is a crucial aspect of developing robust and secure applications. In SAP Cloud Application Programming Model (CAP), input validation plays a vital role in ensuring the integrity and reliability of the data processed by your application. By validating user input, you can prevent common security vulnerabilities, data inconsistencies, and potential errors. In this blog post, we will explore the importance of input validation in CAP and how it is controlled by annotations.
CAP provides built-in validation annotations that you can apply to entity properties or service parameters to enforce basic validation rule.
Lets have a look on the basic and essential validation annotations in CAP by examining a sample entity, “Student,” and demonstrating how to apply various validation rules using annotations.
The code is available at GitHub.
Sample Entity: Student
Let’s dive into the sample entity, “Student,” and annotate its properties with input validation annotations:
entity Student : cuid, managed {
rollno : String(5) @mandatory @Core.Immutable @assert.unique;
name : String(50) @mandatory ;
age : Integer @assert.range: [ 18, 50 ];
dept : String(3) @assert.range enum { CSE; ECE; EEE; };
email : String(200) @mandatory;
telephone : String(132) @mandatory;
postCode : String(10) @mandatory;
}
annotate Student with {
name @assert.format: '/^[a-zA-Z]+ [a-zA-Z]+$/';
email @assert.format: '^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$';
telephone @assert.format: '^[\+]?[(]?[0-9]{3}[)]?[-\s\.]?[0-9]{3}[-\s\.]?[0-9]{4,6}$';
}
-
@mandatory
This annotation is used to specify that a property or field is mandatory, meaning it must have a non-null value.It also ensures that the property is required and must be provided when creating or modifying an entity instance. ‘*’ symbol is shown in the label of the property in UI Screen.If a mandatory property is not provided a value, the CAP framework will raise an error, indicating that the property is missing.
-
@assert.unique
This annotation is used to enforce uniqueness constraints on a property within an entity. It ensures that the values of the annotated property must be unique among all instances of the entity.
In the above example, the rollno
property is annotated with @assert.unique
. This means that each Student
entity instance must have a unique value for the rollno
property. When creating or updating a Student
entity, the CAP framework automatically checks the uniqueness of the rollno
value and raises an error if a duplicate value is detected.
-
@assert.range
This annotation is used to define range constraints [min,max] on numeric or date/time properties within an entity. It ensures that the values of the annotated property fall within a specified range.
In the above example, the age
property is annotated with @assert.range: [18, 50]
. This specifies that the age
property must have a value between 18 and 50, inclusive. When creating or updating a Student
entity, the CAP framework automatically checks the range of the age
value and raises an error if it falls outside the specified range.
-
@assert.range enum
If you want to enforce a specific range of values for an enum property in CAP, you can achieve this by combining @assert.range enum {values}
In the above example, the dept
property is annotated with @assert.range
and defined as an enumeration. It ensures that the department value is within the specified options: CSE, ECE, and EEE.This ensures that only valid department values are allowed for the dept
property.
-
@assert.format
This annotation is used to enforce a specific format or pattern on string properties within an entity. It allows you to validate that the value of the annotated property matches a particular format using a regular expression pattern.
In the above example, we have three properties (name
, email
, and telephone
) annotated with @assert.format
.
- For the
name
property, the regular expression/^[a-zA-Z]+ [a-zA-Z]+$/
is specified as the format. This pattern enforces that thename
value consists of two words separated by a space. - The
email
property is annotated with a regular expression pattern'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
, which validates that theemail
value follows a standard email format. - The
telephone
property uses the regular expression pattern'^[\+]?[(]?[0-9]{3}[)]?[-\s\.]?[0-9]{3}[-\s\.]?[0-9]{4,6}$'
to ensure thetelephone
value matches a specific phone number format.
It’s important to note that the regular expressions used in @assert.format
annotations should be carefully crafted to match the intended format and account for any variations or edge cases that may arise.
Error Handling:
Find the below output to showcase how the errors are handled when the validation fails.


These are standard message raised by @assert
annotation.These standard messages can be overridden based on the business purpose.
To override the standard messages in SAP Cloud Application Programming Model (CAP), you can create a messages.properties
file and define custom messages for specific validation errors or other standard messages. Here’s how you can set up the messages.properties
file:
- Create a
messages.properties
file: In your CAP project, create a new file namedmessages.properties
under thesrv
directory. - Define custom messages:In the
messages.properties
file and add key-value pairs representing the messages you want to override. The keys should correspond to the standard messages you wish to replace.
For example, let’s say you want to override the message for the assert.
validation error. Add the following lines to the messages.properties
file:
ASSERT_FORMAT=The input "{0}" contains invalid characters
ASSERT_RANGE=The input "{0}" should be between "{1}" and "{2}"
ASSERT_NOT_NULL={0} is mandatory input
ASSERT_ENUM=Please only enter a value from {{1}}
You can use placeholders in the messages.properties
file to provide dynamic and more informative messages. Placeholders allow you to include dynamic values or parameters within the messages that can be replaced with actual values at runtime.
In the above example, we have defined messages with placeholders. The {0}
, {1}
, and {2}
within the messages are placeholders that represent dynamic values.
When a validation error occurs, CAP will replace these placeholders with the actual values specified during runtime. For instance, if the assert.mandatory
validation fails for the name
field, the placeholder {0}
will be replaced with the field name, resulting in a message like “‘name’ is mandatory input.”
Similarly, for the assert.range
validation, if the value of the age
property falls outside the specified range, the placeholders {0}
, {1}
, and {2}
will be replaced with the property name and the range values, resulting in a message like “The input ‘age’ should be between 18 and 50.”
Note: Ensure that the messages.properties
file is placed in the correct location (srv
directory) and that the file name is spelled correctly. Also, make sure that the custom messages in the file are in the format key=value
, with one key-value pair per line.
This is how the standard messages are replaced through messages.properties.


Conclusion:
Input validation annotations in SAP Cloud Application Programming Model (CAP), provide a powerful mechanism to enforce validation rules . By leveraging these annotations, we can enhance the integrity and security of our application data. In this blog post, we explored a simple sample entity, “Student,” and demonstrated the application of various input validation annotations to enforce mandatory fields, unique values, value ranges, and specific formats for properties such as email and telephone numbers.
Thank you for reading this blog and I hope you were able to follow it and be useful.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK