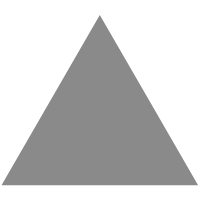
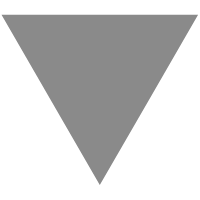
TypeScript Lambda Expressions: A Beginner's Guide to Syntax, Benefits
source link: https://saditya9211.hashnode.dev/how-to-use-lambda-expressions-in-typescript
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
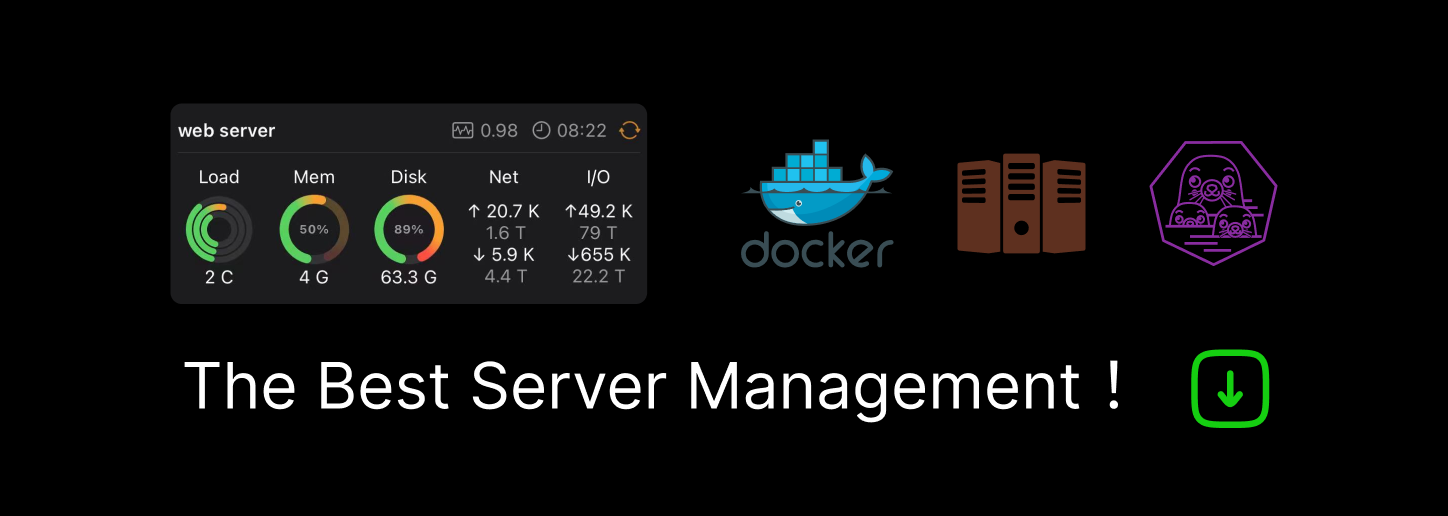
Table of contents
Lambda expression in TypeScript provides a concise and expressive way to define functions and can be used as class methods, object properties, and callbacks in higher-order functions.
In this article, we will explore the syntax of lambda expressions, their benefits over traditional functions, and how to use them effectively in TypeScript.
What are lambda expressions?
Lambda expressions were introduced in ECMAScript 6 and have since become a popular feature in modern JavaScript and TypeScript code. Lambda expressions, also known as arrow functions, are a shorthand syntax for writing functions in JavaScript and TypeScript. They are similar to traditional JavaScript functions, but with a few key differences.
Syntax
The basic syntax for declaring a lambda expression/arrow function in typescript is given below:
(parameter1: type, parameter2: type, ...) => expression
Here, the parameter1
, parameter2
, etc. are the parameters that the function takes, and type specifies the data type of each parameter. The expression can be a single expression or a block of statements enclosed in curly braces. In case, it is a single expression, it is evaluated and returned when the function is invoked.
Lambda expressions can also have an even shorter syntax when there is only one parameter. In that case, the parentheses can be omitted. Here's an example:
parameter1: type => expression;
This lambda expression takes a single parameter and expression can be a single or a block of statements enclosed in curly braces.
Using Lambda Expressions in TypeScript
To use lambda expressions in TypeScript, we simply declare a variable using the const or let keyword, followed by the lambda expression syntax.
Example 1
We can define traditional functions as lambda expressions and use the variable name to call the function by passing appropriate parameters. Here is an example of mulitiply function for two number type arguments.
// traditional function declaration
function multiplyTraditional(x: number, y: number): number {
return x * y;
}
// lambda expression or arrow function declaration
const multiplyLambda = (x: number, y: number): number => x * y;
console.log(`The output of multiplyTraditional function is: ${multiplyTraditional(4, 5)}`);
console.log(`The output of multiplyLambda function is: ${multiplyLambda(4, 5)}`);
Output
Example 2
We can also use lambda expressions as callbacks in functions such as array methods like map, filter, and reduce. Here's an example using the map method:
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map(x => x * x);
console.log(`Original array: ${numbers}`);
console.log(`Squared array: ${squaredNumbers}`);
This lambda expression takes a single parameter and returns its square. The map
method applies this lambda expression to each element of the array, returning a new array of squared numbers.
Output
Example 3
Lambda expressions can also be used as class methods or object properties. Here's an example of a class with a method written as a lambda expression:
class Calculator {
add = (x: number, y: number): number => x + y;
}
const obj = new Calculator();
console.log(`The result is: ${obj.add(4, 5)}`);
In this example, the add
method is declared as a lambda expression and is a property of the Calculator
class.
Output
Example 4
Higher-order functions, which are functions that take one or more functions as arguments and/or return a function as a result. Lambda expressions can be used to define these functions.
const applyOperation = (x: number, y: number, operation: (a: number, b: number) => number) => {
return operation(x, y);
};
const result1 = applyOperation(10, 20, (a, b) => a + b); // 30
const result2 = applyOperation(10, 20, (a, b) => a * b); // 200
In this example, we pass two numbers and a function to the applyOperation
function. It returns the result of the passed function with the passed two number parameters.
Output

Example 5
Lambda expressions have lexical scoping and are closures that have access to variables defined in the same scope as the lambda expression. When a lambda expression is defined, it captures the values of any variables that are in scope and uses them when the lambda expression is later executed.
Here is an example demonstrating the same.
function createCounter() {
let count = 0;
return () => {
count++;
console.log(`Current count is ${count}`);
};
}
const counter = createCounter();
counter(); // Current count is 1
counter(); // Current count is 2
In this example, the createCounter
function returns a lambda expression that increments and logs the count variable each time it is called. The count
variable is defined in the same scope as the lambda expression, so the lambda expression has access to its value and can modify it.
When the counter
function is called twice, it logs the current count, which starts at 1 and increments by 1 each time the lambda expression is executed.
Output

Benefits of Using Lambda Expressions in TypeScript
Lambda expressions offer several benefits over traditional functions:
Conciseness: Lambda expressions allow us to write functions in a more concise way, which can make our code easier to read and understand.
Clarity: Lambda expressions can make it clearer what a function does, especially when used as a callback in a higher-order function like map or filter.
Performance: Lambda expressions can sometimes be faster than traditional functions because they have a simpler syntax that can be optimized by the JavaScript engine.
Scope: Lambda expressions have lexical scoping, which means they have access to variables in the surrounding scope, allowing for powerful and flexible programming techniques such as currying and memoization.
Conclusion
Lambda expressions in TypeScript offer conciseness, clarity, scope, and performance. They can be used as class methods, object properties, and callbacks in higher-order functions. By taking advantage of the concise syntax and lexical scoping, you can write more expressive and modular code. However, complex lambda expressions can become difficult to read, so traditional functions may be preferred in such cases. Overall, lambda expressions improve code readability and maintainability.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK