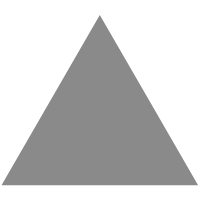
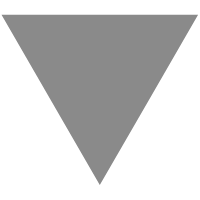
使用LRU加速python应用 - Mike_Zhang
source link: https://www.cnblogs.com/MikeZhang/p/2023031901-pythonLRUTest.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
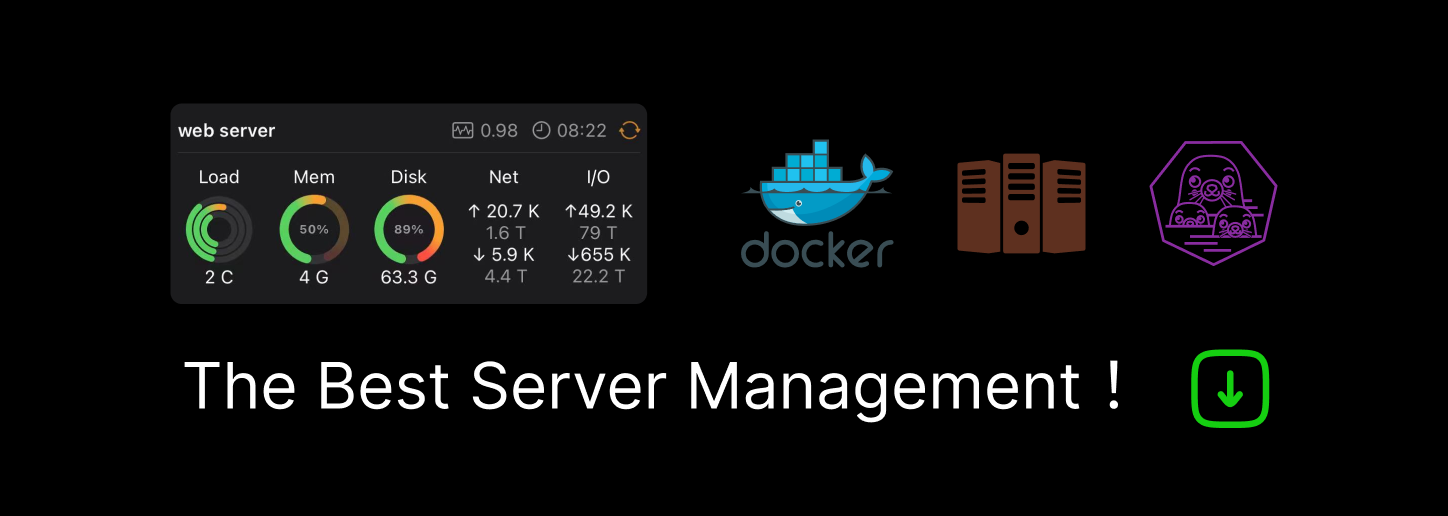
操作系统 :CentOS 7.6.1810_x64
Python 版本 : 3.9.12
一、背景描述
使用python开发过程中,会遇到需要使用缓存加速应用的情况,比如下面这些场景:
-
数据转换加速
字符串时间转换成int时间戳;
字符串时间转换成datetime类型;
-
数据解析加速
bytes数据转换为int(数据包解析场景的端口、序列号等);
bytes数据转换为string(数据包解析场景的ip地址等);
本文提供两种实现方式来加速应用,这里记录下,希望对你有帮助。
二、具体实现
1、使用python自带的OrderedDict实现LRU
实现思路:
1)使用OrderedDict作为缓存,并设置大小;
2)第一次解析时,将解析结果加入缓存;
3)缓存元素数量超过设定数量,执行pop操作;
示例代码如下 :
from collections import OrderedDict class LRU: def __init__(self, func, maxsize=128): self.func = func self.maxsize = maxsize self.cache = OrderedDict() def __call__(self, *args): if args in self.cache: value = self.cache[args] self.cache.move_to_end(args) return value value = self.func(*args) if len(self.cache) >= self.maxsize: self.cache.popitem(False) self.cache[args] = value return value
2、使用lru-dict库实现LRU
pypi地址:https://pypi.org/project/lru-dict/
github地址:https://github.com/amitdev/lru-dict
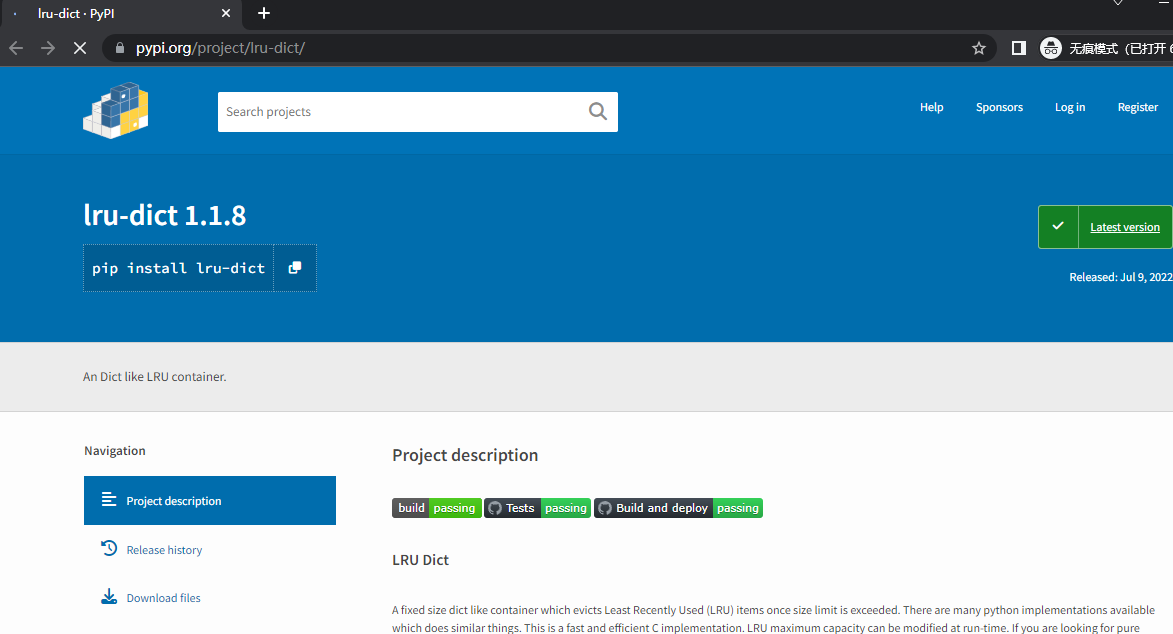
安装lru-dict库:
pip install lru-dict
示例代码:
from lru import LRU l = LRU(5) # Create an LRU container that can hold 5 items print l.peek_first_item(), l.peek_last_item() #return the MRU key and LRU key # Would print None None for i in range(5): l[i] = str(i) print l.items() # Prints items in MRU order # Would print [(4, '4'), (3, '3'), (2, '2'), (1, '1'), (0, '0')] print l.peek_first_item(), l.peek_last_item() #return the MRU key and LRU key # Would print (4, '4') (0, '0') l[5] = '5' # Inserting one more item should evict the old item print l.items() # Would print [(5, '5'), (4, '4'), (3, '3'), (2, '2'), (1, '1')]
由于lru-dict库是使用c实现的,使用源代码安装可能存在环境问题,可直接使用pypi上面提供的预编译whl文件:
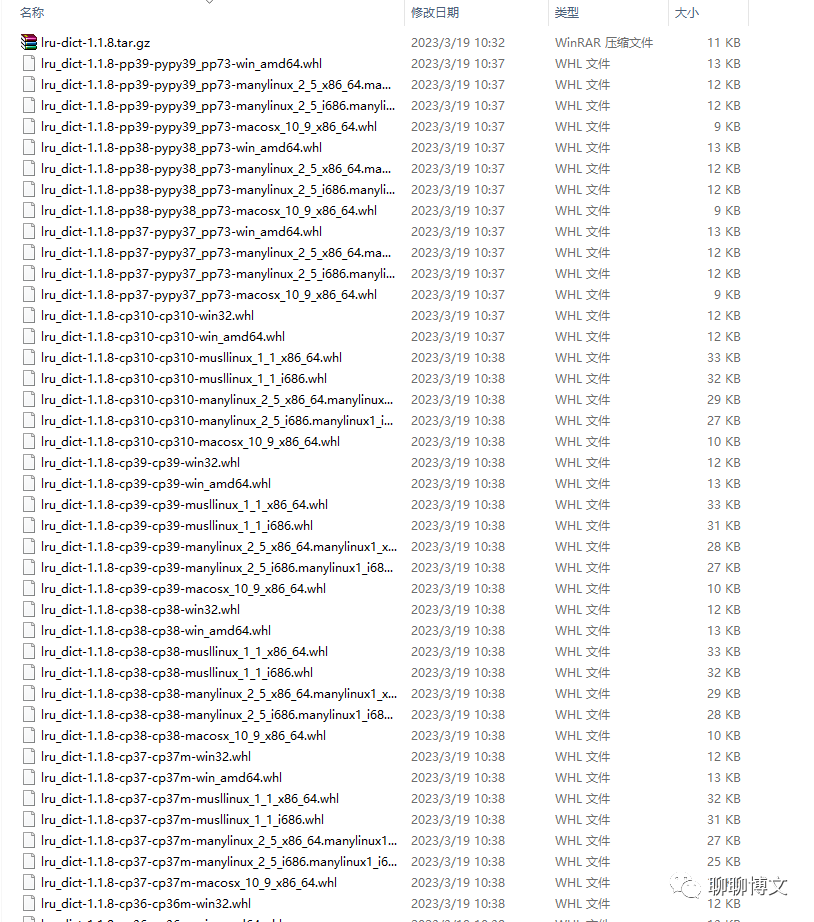
1)源码包为 lru-dict-1.1.8.tar.gz;
2)本文使用的whl文件是 lru_dict-1.1.8-cp39-cp39-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl ;
3)可从以下途径获取上述文件:
关注微信公众号(聊聊博文,文末可扫码)后回复 2023031901 获取。
三、运行效果
这里演示下两种实现方式的具体效果,并做下对比。
1、测试用例1(lru库实现)
测试代码:
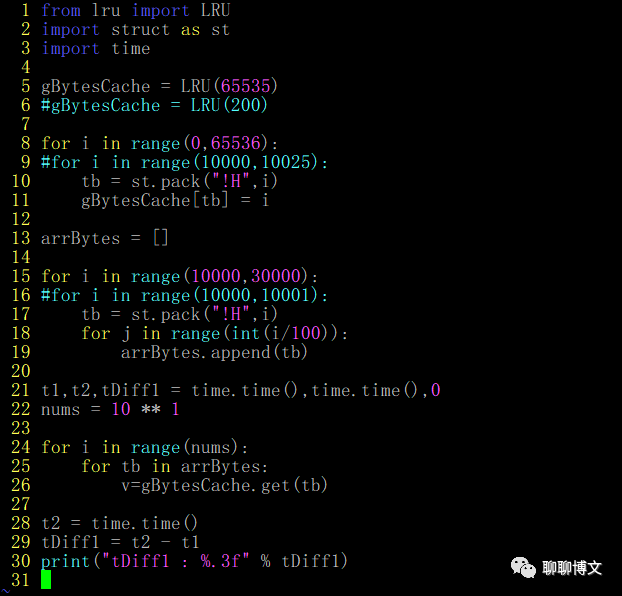
运行效果:
运行时间:15.046 秒
2、测试用例2( OrderedDict实现)
测试代码:
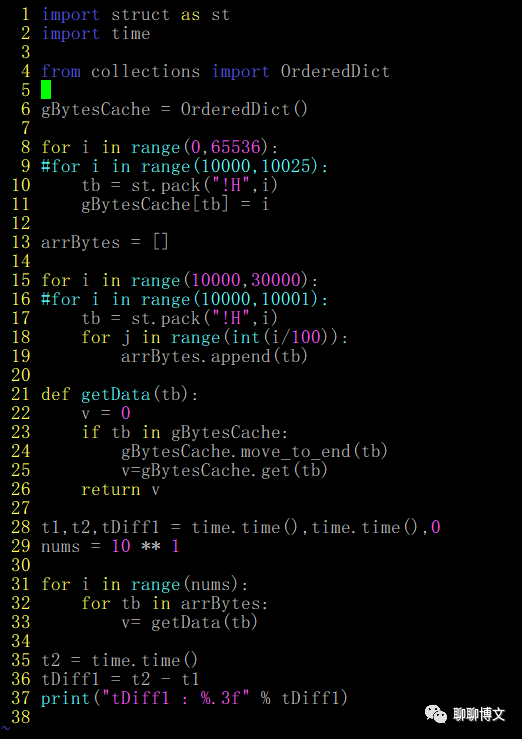
运行效果:
运行时间: 28.934秒
结论:
lru-dict库比较快。
说明:
1)使用OrderedDict实现LRU的优点在于不用安装额外的库;
2)lru-dict是使用c语言开发的第三方库,需要使用pip进行安装,性能比较好,但和平台相关性比较强;
四、资源下载
本文涉及示例代码及whl文件,可从百度网盘获取:
https://pan.baidu.com/s/1N6wWHhMkvXcyVI5mEhn1JA
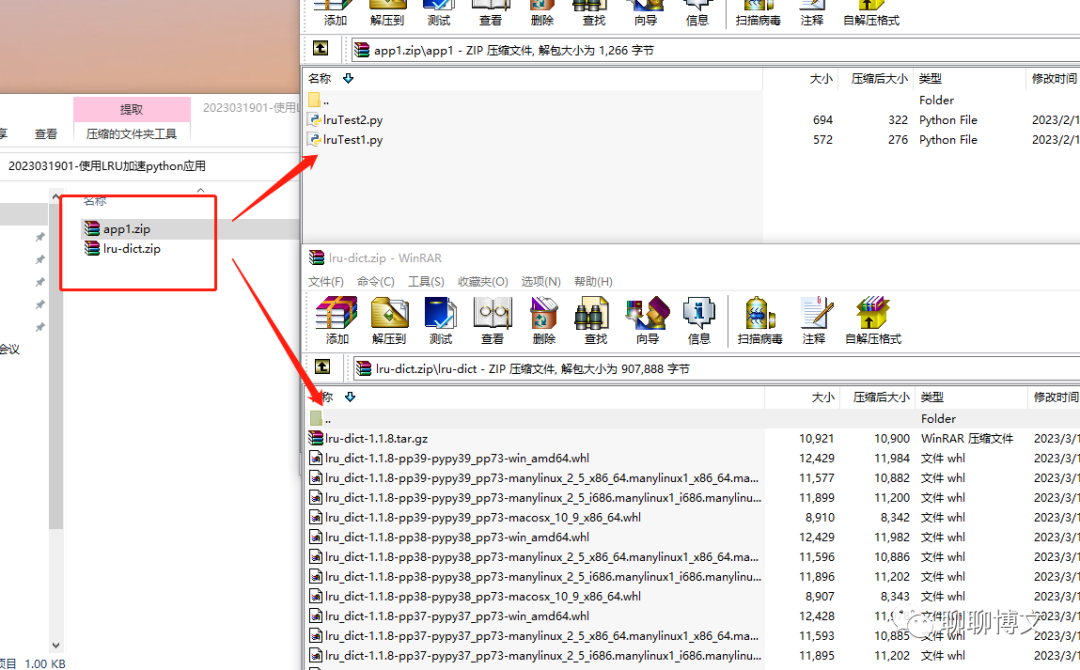
关注微信公众号(聊聊博文,文末可扫码)后回复 2023031901 获取。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK