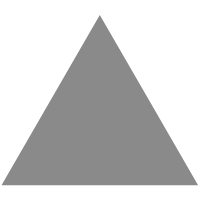
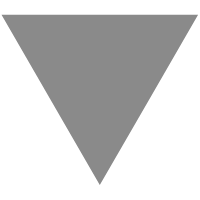
Seamless Integration of Azure Functions With SQL Server: A Developer's Perspecti...
source link: https://dzone.com/articles/seamless-integration-of-azure-functions-with-sql-s
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
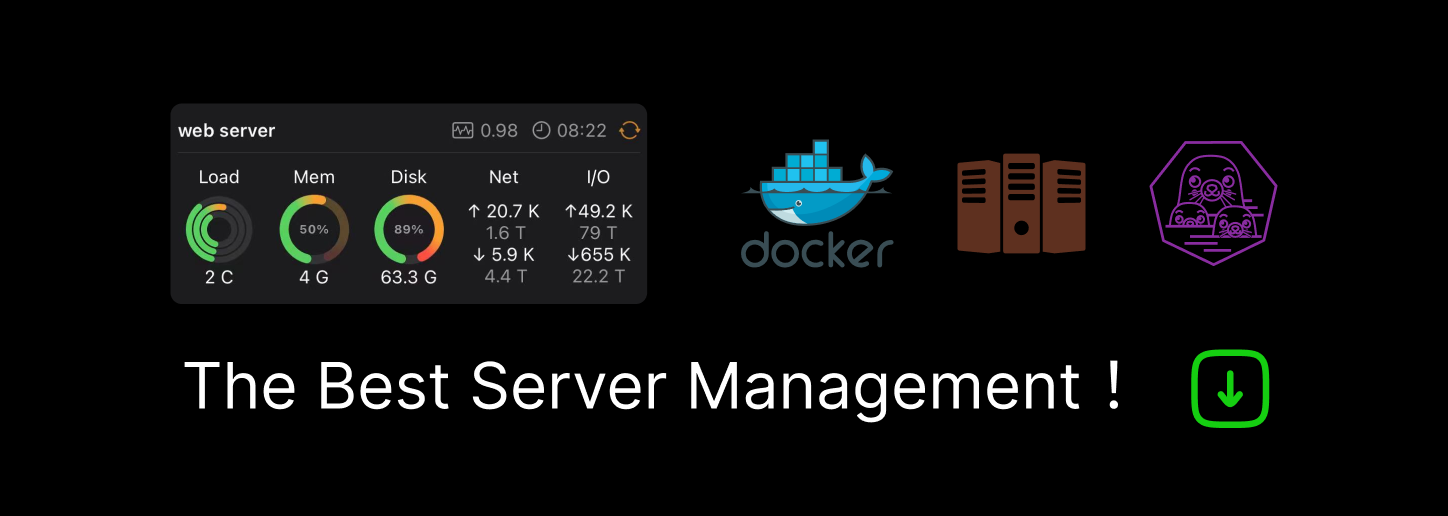
Azure Functions Overview
Azure Functions is a serverless compute service that enables developers to run code on-demand without having to worry about infrastructure. It provides an easy way to build and deploy event-driven, scalable, and cost-effective applications that can be triggered by a variety of sources such as HTTP requests, messages, and timers. In this article, we will explore how to invoke Azure Functions from SQL Server using C#.
As a developer, you may need to integrate Azure Functions with SQL Server to leverage the power of cloud computing and enhance your applications. In this article, we will explore how to accomplish this using C#.
Prerequisites
Before we start, make sure you have the following:
- An Azure subscription
- Visual Studio (2019 or later)
- SQL Server Management Studio (SSMS)
Creating an Azure Function App
The first thing to do is create an Azure Function App. Follow these steps:
- Log in to the Azure Portal and choose "Create a resource."
- Search for "Function App" and select it.
- Fill in the required information such as the subscription, resource group, name, and region.
- Under "Hosting," select "Consumption Plan" and choose the appropriate OS.
- Click "Create" and wait for the deployment to finish.
Creating an HTTP Triggered Azure Function
Next, we need to create an HTTP Triggered Azure Function. Follow these steps:
- Open Visual Studio and create a new Azure Functions project.
- Select "HTTP trigger" as the template for the Azure Function.
- Fill in the required information such as the name and namespace.
- Choose the appropriate authorization level (e.g. anonymous, function, admin).
- Click "Create" and wait for the project to be created.
Writing the C# Code
Now, we need to write the C# code that invokes the Azure Function from SQL Server. Follow these steps:
- Create a new C# class called "FunctionInvoker" in your Visual Studio project.
- Add the following using statements:
using System.Net.Http;
using System.Threading.Tasks;
- Add the following method to the class:
public static async Task<(string responseText, int responseCode)> InvokeAsync(string url)
{
using (var client = new HttpClient())
{
var response = await client.GetAsync(url);
var responseText = await response.Content.ReadAsStringAsync();
return (responseText, (int)response.StatusCode);
}
}
- Build the Visual Studio project and obtain the compiled DLL for the
FunctionInvoker
class. - Use SSMS to create a SQL Server stored procedure that invokes the Azure Function using the
FunctionInvoker
class. Here's an example:
CREATE PROCEDURE [dbo].[InvokeAzureFunction] @param1 NVARCHAR(50), @param2 NVARCHAR(50), @responseText NVARCHAR(MAX) OUTPUT, @responseCode INT OUTPUT
AS
BEGIN
SET NOCOUNT ON;
DECLARE @url NVARCHAR(MAX) = 'https://<functionappname>.azurewebsites.net/api/<functionname>?code=<functionkey>¶m1=' + @param1 + '¶m2=' + @param2;
BEGIN TRY
SELECT @responseText = responseText, @responseCode = responseCode FROM (SELECT * FROM (VALUES (FunctionInvoker.FunctionInvoker.InvokeAsync(@url))) AS value(responseText, responseCode)) AS result;
END TRY
BEGIN CATCH
SELECT ERROR_MESSAGE() AS ErrorMessage;
RETURN;
END CATCH;
SELECT @responseText AS ResponseText, @responseCode AS ResponseCode;
END
Replace <functionappname>
, <functionname>
, and <functionkey>
with the appropriate values for your Azure Function. Also, replace path/to/FunctionInvoker.dll
with the path to the compiled DLL of the FunctionInvoker
class.
Finally, we can use the SQL Server stored procedure to invoke the Azure Function. Here's an example:
DECLARE @responseText NVARCHAR(MAX);
DECLARE @responseCode INT;
EXEC [dbo].[InvokeAzureFunction] 'value1', 'value2', @responseText OUTPUT, @responseCode OUTPUT;
SELECT @responseText AS ResponseText, @responseCode AS ResponseCode;
Replace 'value1'
and 'value2'
with the appropriate values for your Azure Function parameters.
Conclusion
In this article, we explored how to integrate Azure Functions with SQL Server using C#. We started by creating an Azure Function App and an HTTP Triggered Azure Function. Then, we wrote a C# class that invokes the Azure Function and created a SQL Server stored procedure that uses the class to invoke the Azure Function. Finally, we used the stored procedure to invoke the Azure Function with SQL Server parameters.
By integrating Azure Functions with SQL Server, you can enhance your applications with cloud computing capabilities and take advantage of the scalability and flexibility offered by the Azure cloud.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK