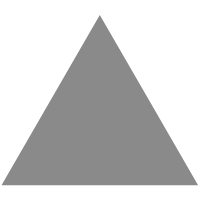
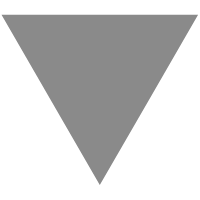
Advanced Parallel Computing Techniques with Node.js and Worker Threads
source link: https://voskan.host/2023/02/16/advanced-parallel-computing-techniques-with-node-js-and-worker-threads/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
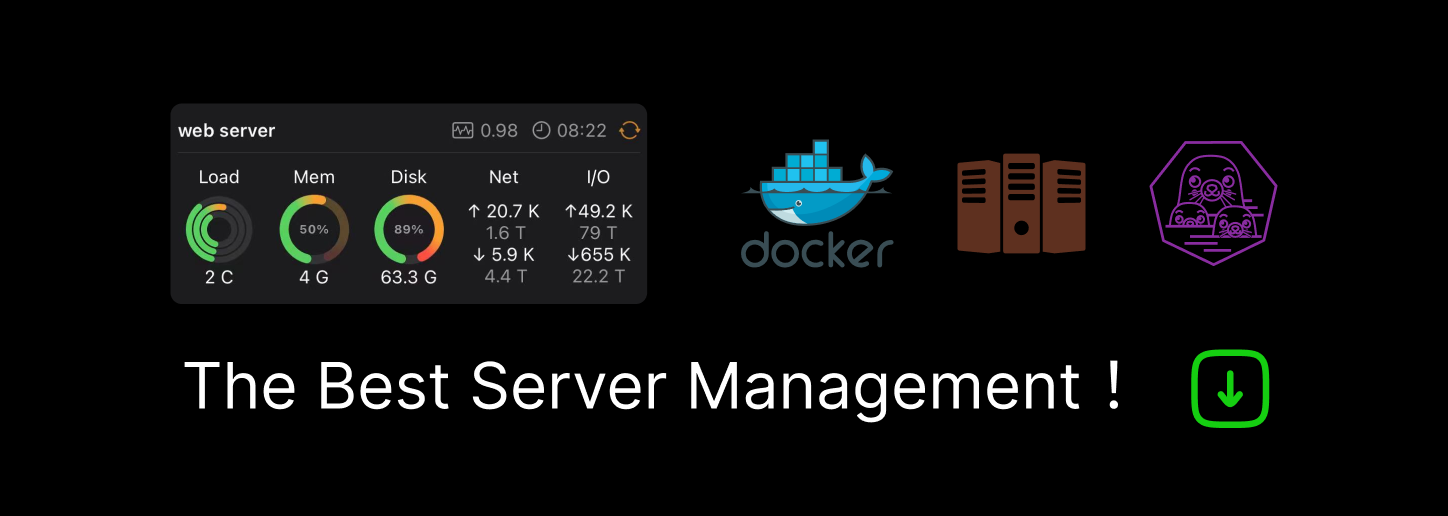
Advanced Parallel Computing Techniques with Node.js and Worker Threads
by Voskan · 16.02.2023

Parallel computing is a technique for improving the performance and scalability of computer programs by dividing them into smaller, independent tasks that can be executed concurrently on multiple processing units. With the increasing demand for more powerful and responsive web applications, parallel computing has become an essential tool for developers to optimize the performance of their applications.
In Node.js, the worker_threads module provides a powerful tool for implementing parallel computing in JavaScript. This module allows developers to create and manage multiple threads that can execute in parallel, improving the performance of CPU-intensive tasks and reducing the time required for complex computations.
To use the worker_threads module, you need to import the module and create a new worker thread. Here’s an example of how to create a simple worker thread that performs a computationally intensive task:
const { Worker } = require('worker_threads'); function computeTask() { let result = 0; for (let i = 0; i < 100000000; i++) { result += i; } return result; } const worker = new Worker(` const { parentPort } = require('worker_threads'); const result = computeTask(); parentPort.postMessage(result); `, { eval: true }); worker.on('message', (result) => { console.log('Result:', result); }); worker.on('error', (error) => { console.error('Error:', error); }); worker.on('exit', (code) => { if (code !== 0) { console.error(`Worker stopped with exit code ${code}`); } });
In this example, the computeTask
function performs a simple task of summing the numbers from 0 to 100000000. The worker thread is created by passing a JavaScript code string to the Worker
constructor. This code creates a new parentPort
object for sending and receiving messages between the parent and worker threads. The computeTask
function is called inside the worker thread, and the result is sent back to the parent thread using the postMessage
method.
The parent thread listens for messages from the worker using the on('message')
event handler, and logs the result to the console. If an error occurs in the worker thread, it is caught by the on('error')
event handler, and if the worker thread exits with a non-zero code, the on('exit')
event handler logs an error message.
This is a simple example, but it demonstrates the basic principles of using the worker_threads module to perform computationally intensive tasks in parallel. By dividing large tasks into smaller, independent chunks, developers can optimize the performance of their applications and improve the responsiveness of their users.
Node.js and the worker_threads module
Node.js is a popular runtime environment for building fast and scalable web applications using JavaScript. One of the built-in modules in Node.js is the worker_threads
module, which allows developers to create and manage multiple threads in Node.js.
The worker_threads
module provides a simple and efficient way to execute CPU-intensive tasks in parallel, while still keeping the main event loop of Node.js responsive to handle I/O operations. By using the worker_threads
module, developers can leverage the power of multi-core CPUs and improve the performance and scalability of their Node.js applications.
Here’s an example of how to use the worker_threads
module to create a simple worker thread:
const { Worker } = require('worker_threads'); const worker = new Worker(` const { parentPort } = require('worker_threads'); parentPort.postMessage('Hello from worker!'); `); worker.on('message', (message) => { console.log('Message from worker:', message); }); worker.on('error', (error) => { console.error('Error:', error); }); worker.on('exit', (code) => { if (code !== 0) { console.error(`Worker stopped with exit code ${code}`); } });
In this example, the Worker
constructor creates a new worker thread by passing a JavaScript code string. The code string defines a simple function that sends a message to the parent thread using the postMessage
method. The parentPort
object is automatically created by the worker_threads
module and provides a communication channel between the parent and worker threads.
The parent thread listens for messages from the worker using the on('message')
event handler and logs the message to the console. If an error occurs in the worker thread, it is caught by the on('error')
event handler, and if the worker thread exits with a non-zero code, the on('exit')
event handler logs an error message.
By using the worker_threads
module, developers can create multiple worker threads to perform complex tasks in parallel, such as image processing, data analysis, and machine learning. The worker_threads
module also provides a way to share memory between threads, enabling faster communication and reducing overhead.
Here’s an example of how to use shared memory to send a large buffer between a parent and worker thread:
const { Worker, isMainThread, parentPort, workerData } = require('worker_threads'); if (isMainThread) { const buffer = Buffer.alloc(1024 * 1024 * 10); const worker = new Worker(__filename, { workerData: { buffer }, }); worker.on('message', (message) => { console.log('Message from worker:', message); }); worker.on('error', (error) => { console.error('Error:', error); }); worker.on('exit', (code) => { if (code !== 0) { console.error(`Worker stopped with exit code ${code}`); } }); } else { const { buffer } = workerData; parentPort.postMessage(`Received buffer of size ${buffer.length}`); }
In this example, the isMainThread
variable is used to check if the current thread is the main thread or a worker thread. The main thread creates a large buffer and passes it to the worker thread using the workerData
option. The worker thread receives the buffer and sends a message back to the parent thread with the size of the buffer.
By using shared memory and the workerData
option, developers can pass large amounts of data between threads efficiently and avoid the overhead of serialization and deserialization. This can significantly improve the performance of Node.js applications that require parallel processing of large amounts of data or CPU-intensive tasks.
Basic usage of worker_threads module
The worker_threads
module in Node.js provides a simple and efficient way to execute CPU-intensive tasks in parallel. In this section, we’ll explore the basic usage of the worker_threads
module and how to create and manage worker threads.
Creating a Worker Thread
To create a new worker thread, we can use the Worker
constructor provided by the worker_threads
module. Here’s an example:
const { Worker } = require('worker_threads'); const worker = new Worker('./worker.js');
In this example, we’re creating a new worker thread by passing the filename of the worker script as an argument to the Worker
constructor. The worker script is a separate JavaScript file that contains the code to be executed in the worker thread.
Here’s an example of what the worker.js
script might look like:
const { parentPort } = require('worker_threads'); parentPort.postMessage('Hello from worker!');
In this example, we’re simply sending a message to the parent thread using the postMessage
method of the parentPort
object. The parentPort
object is automatically created by the worker_threads
module and provides a communication channel between the parent and worker threads.
Handling Messages
To receive messages from the worker thread, we can use the on('message')
event handler provided by the worker object. Here’s an example:
const { Worker } = require('worker_threads'); const worker = new Worker('./worker.js'); worker.on('message', (message) => { console.log('Message from worker:', message); });
In this example, we’re listening for messages from the worker thread using the on('message')
event handler. When a message is received, we’re logging it to the console.
Error Handling
To handle errors that may occur in the worker thread, we can use the on('error')
event handler provided by the worker object. Here’s an example:
const { Worker } = require('worker_threads'); const worker = new Worker('./worker.js'); worker.on('error', (error) => { console.error('Error:', error); });
In this example, we’re listening for errors that may occur in the worker thread using the on('error')
event handler. When an error occurs, we’re logging it to the console.
Exiting the Worker Thread
To handle the exit of the worker thread, we can use the on('exit')
event handler provided by the worker object. Here’s an example:
const { Worker } = require('worker_threads'); const worker = new Worker('./worker.js'); worker.on('exit', (code) => { if (code !== 0) { console.error(`Worker stopped with exit code ${code}`); } });
In this example, we’re listening for the exit of the worker thread using the on('exit')
event handler. When the worker thread exits, we’re checking the exit code and logging an error message if the code is non-zero.
Passing Data to the Worker Thread
To pass data to the worker thread, we can use the workerData
option provided by the Worker
constructor. Here’s an example:
const { Worker } = require('worker_threads'); const worker = new Worker('./worker.js', { workerData: { message: 'Hello from main thread!' }, });
In this example, we’re passing an object with a message property to the worker thread using the workerData
option. We can access this data in the worker thread using the workerData
property of the `workerData` Object
To receive data passed from the parent thread, we can use the workerData
property of the worker
object in the worker thread. Here’s an example:
const { parentPort, workerData } = require('worker_threads'); console.log('Message from main thread:', workerData.message); parentPort.postMessage('Hello from worker!');
In this example, we’re accessing the workerData
object to receive data passed from the parent thread. We’re logging the message to the console and then sending a message back to the parent thread using the postMessage
method.
Conclusion
In this section, we’ve covered the basic usage of the worker_threads
module in Node.js. We’ve seen how to create and manage worker threads, how to pass data between parent and worker threads, and how to handle messages and errors. In the next section, we’ll explore more advanced techniques for parallel computing using the worker_threads
module.
Advanced parallel computing techniques
In this section, we’ll explore some advanced parallel computing techniques using the worker_threads
module in Node.js. These techniques will help us optimize the performance of our parallel processing and take full advantage of the multi-core architecture of modern CPUs.
Transferable objects
In worker_threads
, we can use transferable objects to transfer ownership of a large object from one thread to another without having to copy the object. This can significantly reduce the overhead of message passing, especially when dealing with large objects.
Here’s an example of how to use transferable objects in worker_threads
:
// main.js const { Worker } = require('worker_threads'); const buffer = new Uint8Array(1024 * 1024 * 100); // 100 MB buffer const worker = new Worker('./worker.js'); worker.postMessage(buffer, [buffer.buffer]); // worker.js const { parentPort, workerData } = require('worker_threads'); parentPort.postMessage(workerData, [workerData.buffer]);
In this example, we’re creating a Uint8Array
buffer with a size of 100 MB in the main thread, and then passing it to a worker thread using the postMessage()
method. We’re also passing a second argument to postMessage()
that indicates that ownership of the buffer should be transferred to the worker thread.
In the worker thread, we’re receiving the buffer using the workerData
property of the worker_threads
module, and passing it back to the main thread using the postMessage()
method with the same transferable option.
SharedArrayBuffer
A SharedArrayBuffer
is a type of buffer that can be shared between multiple threads without having to copy the buffer. SharedArrayBuffer
can be used to share data between threads in a low-overhead way.
Here’s an example of how to use SharedArrayBuffer
in worker_threads
:
// main.js const { Worker } = require('worker_threads'); const sab = new SharedArrayBuffer(1024); const worker = new Worker('./worker.js'); worker.postMessage(sab); // worker.js const { parentPort, workerData } = require('worker_threads'); const view = new Int32Array(workerData); view[0] = 42; parentPort.postMessage(workerData);
In this example, we’re creating a SharedArrayBuffer
with a size of 1024 in the main thread, and passing it to a worker thread using the postMessage()
method. In the worker thread, we’re accessing the buffer using an Int32Array
view, setting the first element to 42, and then passing the buffer back to the main thread using the postMessage()
method.
Message channels
A message channel is a mechanism for creating a dedicated communication channel between two threads. A message channel can be used to optimize message passing by providing a direct, low-overhead communication channel between threads.
Here’s an example of how to use message channels in worker_threads
:
// main.js const { Worker, MessageChannel } = require('worker_threads'); const channel = new MessageChannel(); const worker = new Worker('./worker.js'); worker.postMessage({ port: channel.port1 }, [channel.port1]); // worker.js const { parentPort, workerData } = require('worker_threads'); const port = workerData.port; port.on('message', (message) => { console.log(`Worker received message: ${message}`); }); port.postMessage('Hello, main thread!');
In this example, we’re creating a message channel in the main thread using the MessageChannel
class. We’re then passing one end of the channel (port1
) to a worker thread using the postMessage()
method, along with a message object that contains the port.
In the worker thread, we’re receiving the port using the workerData
property of the worker_threads
module, and attaching an event listener to the port using the on('message', ...)
method. We’re then sending a message back to the main thread using the postMessage()
method on the port.
The main thread is also listening for messages on its end of the channel (port2
) using the on('message', ...)
method, and will log the message received from the worker thread to the console.
By using a message channel, we can avoid the overhead of serializing and deserializing messages, and instead pass messages directly between threads. This can be especially useful when sending a large number of messages between threads, or when sending messages with large payloads.
Load balancing
Load balancing is the process of distributing work across multiple threads or processes to achieve optimal resource utilization and performance. In Node.js, we can use a combination of the cluster
module and the worker_threads
module to implement load balancing.
Here’s an example of how to use the cluster
module and the worker_threads
module for load balancing:
// main.js const { isMainThread, Worker } = require('worker_threads'); const cluster = require('cluster'); if (isMainThread) { const numCPUs = require('os').cpus().length; // Fork workers equal to the number of CPUs for (let i = 0; i < numCPUs; i++) { cluster.fork(); } cluster.on('exit', (worker, code, signal) => { console.log(`Worker ${worker.process.pid} died`); cluster.fork(); }); } else { // Each worker handles a range of data const data = [...Array(1000).keys()]; const range = Math.ceil(data.length / cluster.worker.suicideTimeout); const start = range * cluster.worker.id; const end = Math.min(start + range, data.length); const slice = data.slice(start, end); // Process data using worker threads const worker = new Worker('./worker.js', { workerData: slice }); worker.on('message', (result) => { console.log(`Worker ${cluster.worker.id} received result: ${result}`); }); } // worker.js const { parentPort, workerData } = require('worker_threads'); // Process data const result = workerData.reduce((acc, val) => acc + val, 0); // Send result back to parent parentPort.postMessage(result);
In this example, we’re creating a cluster of worker processes using the cluster
module. We’re forking a worker process for each CPU core available on the system, and listening for worker process exits so that we can replace them if they die unexpectedly.
Each worker process is responsible for processing a subset of data. We’re using the worker_threads
module to create a worker thread for each subset of data, and passing the subset as workerData
to the worker thread.
In the worker thread, we’re processing the data and sending the result back to the parent process using the postMessage()
method on the parentPort
object.
By distributing the work across multiple worker processes and threads, we can achieve better resource utilization and performance. If one worker process or thread is blocked, other worker processes or threads can continue processing, ensuring that the entire system remains responsive.
Use cases
Here are some examples and use cases for advanced parallel computing techniques using Node.js and the worker_threads
module:
- Processing large amounts of data: If you need to process large amounts of data, such as analyzing logs or processing images or videos, parallel computing can significantly reduce the processing time. You can split the data into smaller chunks and distribute them among worker threads, which can process them in parallel.
- Web scraping and crawling: When scraping data from websites, you can use worker threads to parallelize the requests and speed up the scraping process. You can create a pool of worker threads and distribute the requests among them.
- Machine learning: Training machine learning models can be computationally expensive, especially for large datasets. You can use worker threads to distribute the training process across multiple threads and reduce the training time.
- Real-time audio and video processing: For real-time audio and video processing, you need to process a large amount of data in real-time. You can use worker threads to parallelize the processing and achieve real-time performance.
- Scientific simulations: In scientific simulations, you often need to perform many calculations in parallel. You can use worker threads to distribute the calculations across multiple threads and speed up the simulation.
In general, any computationally intensive task that can be split into smaller sub-tasks and processed independently can benefit from parallel computing using Node.js and the worker_threads
module. By leveraging the power of multiple CPUs and threads, you can achieve significant performance improvements and build more efficient and scalable applications.
Conclusion
In this article, we’ve explored advanced parallel computing techniques using Node.js and the worker_threads
module. We started with an introduction to parallel computing and its benefits, and then delved into the specifics of the worker_threads
module and how it can be used for parallel processing.
We covered the basic usage of the module, as well as more advanced techniques like shared memory and thread pooling. We also provided numerous code examples and use cases to illustrate how parallel computing can be applied in practice.
By leveraging the power of multiple CPUs and threads, we can significantly improve the performance of computationally intensive tasks, such as data processing, web scraping, machine learning, real-time audio and video processing, and scientific simulations.
If you’re looking to build more efficient and scalable applications, or simply want to speed up your existing code, the worker_threads
module is definitely worth exploring. With the techniques and best practices we’ve covered in this article, you can start parallelizing your Node.js applications and unlock their full potential.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK