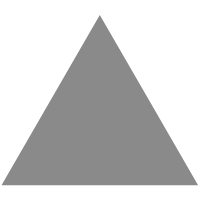
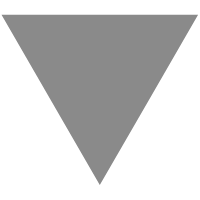
How to get multiple checkbox values in react
source link: https://www.laravelcode.com/post/how-to-get-multiple-checkbox-values-in-react
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
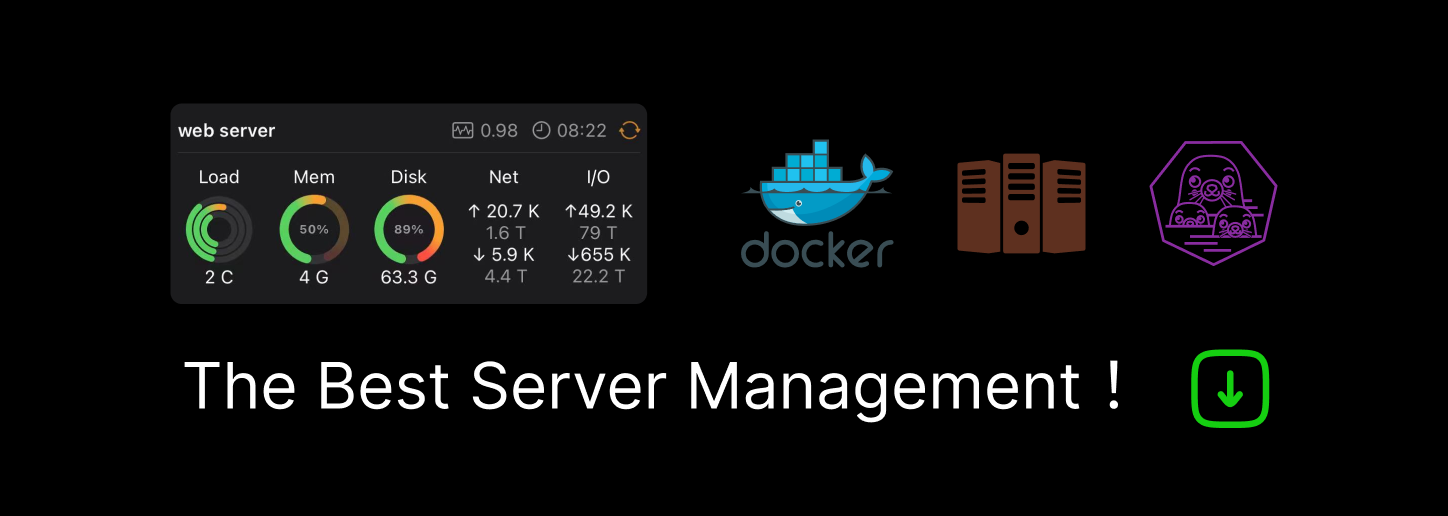
How to get multiple checkbox values in react
In this React Checkbox tutorial, we are going to look at how to handle and store multiple checkboxes values in React application. A checkbox is an HTML element, and It allows the user to choose one or multiple options from a limited number of choices.
We deal with various form controls to build an enterprise-level web or mobile application. There is various kind of form controls used to create an interactive form such as checkboxes, radio buttons, dropdown menus, etc. A checkbox is one of the widely used HTML element in web layout, which allows us to select one or multiple values among other various options.
React Store Multiple Checkboxes Values Tutorial
Let us create a basic form in React and get started with five checkboxes, and it will allow users to select multiple values using checkboxes in React app. We will also go one step further and learn to know how to store the checkboxes value in the MongoDB NoSQL database in string form.
Set up React App
Enter the following command to set up React project with CRA (create-react-app).
npx create-react-app react-multiple-checkboxes
Get inside the React project’s folder:
cd react-multiple-checkboxes
Start the React app by running the following command:
npm start
After running the above command your React project will open on this URL: localhost:3000
Install Bootstrap 4
Install Bootstrap 4 for showing checkboxes demo in our React app.
npm install bootstrap --save
Include bootstrap.min.css file in src/App.js
file:
import React, { Component } from 'react';
import '../node_modules/bootstrap/dist/css/bootstrap.min.css';
class App extends Component {
render() {
return (
<div className="App">
<!-- Form Goes Here -->
</div>
);
}
}
export default App;
Install Axios in React
Install Axios library in React to handle the GET, POST, UPDATE and Delete request to save the value manipulated with React checkboxes.
npm install axios --save
Setting up Checkboxes in React with Bootstrap 4
Let us create the simple form with five checkboxes elements. Keep all the below logic in src/App.js
file:
In the first step we set the initial state of fruits options.
state = {
isApple: false,
isOrange: false,
isBanana: false,
isCherry: false,
isAvocado: false
};
React Chckbox onChange
Define React onChange
event in HTML checkbox element and set the checkbox value in it. When updated by user, this method will update the checkbox’s state.
onChangeApple = () => {
this.setState(initialState => ({
isApple: !initialState.isAvocado,
}));
}
Go to src/App.js
file and add the following code for checkboxes demo:
import React, { Component } from 'react';
import '../node_modules/bootstrap/dist/css/bootstrap.min.css';
class App extends Component {
// Checkbox Initial State
state = {
isApple: false,
isOrange: false,
isBanana: false,
isCherry: false,
isAvocado: false
};
// React Checkboxes onChange Methods
onChangeApple = () => {
this.setState(initialState => ({
isApple: !initialState.isAvocado,
}));
}
onChangeOrange = () => {
this.setState(initialState => ({
isOrange: !initialState.isOrange,
}));
}
onChangeBanana = () => {
this.setState(initialState => ({
isBanana: !initialState.isBanana,
}));
}
onChangeCherry = () => {
this.setState(initialState => ({
isCherry: !initialState.isCherry,
}));
}
onChangeAvocado = () => {
this.setState(initialState => ({
isAvocado: !initialState.isAvocado,
}));
}
// Submit
onSubmit = (e) => {
e.preventDefault();
console.log(this.state);
}
render() {
return (
<div className="App">
<h2>Store Multiple Checkboxes Values in React</h2>
<form onSubmit={this.onSubmit}>
<div className="form-check">
<label className="form-check-label">
<input type="checkbox"
checked={this.state.isApple}
onChange={this.onChangeApple}
className="form-check-input"
/>
Apple
</label>
</div>
<div className="form-check">
<label className="form-check-label">
<input type="checkbox"
checked={this.state.isAvocado}
onChange={this.onChangeAvocado}
className="form-check-input"
/>
Avocado
</label>
</div>
<div className="form-check">
<label className="form-check-label">
<input type="checkbox"
checked={this.state.isBanana}
onChange={this.onChangeBanana}
className="form-check-input"
/>
Banana
</label>
</div>
<div className="form-check">
<label className="form-check-label">
<input type="checkbox"
checked={this.state.isCherry}
onChange={this.onChangeCherry}
className="form-check-input"
/>
Cherry
</label>
</div>
<div className="form-check">
<label className="form-check-label">
<input type="checkbox"
checked={this.state.isOrange}
onChange={this.onChangeOrange}
className="form-check-input"
/>
Orange
</label>
</div>
<div className="form-group">
<button className="btn btn-success">
Save
</button>
</div>
</form>
</div>
);
}
}
export default App;
Set up Backend Server with Node, Express and MongoDB
We will also learn to save multiple checkboxes value in MongoDB database using Node and Express server in a React application. First set up a node server using Node, Express and MongoDB.
Create a new directory in the root of the React app and name it backend.
Run below command to generate a seperate package.json
file inside backend folder.
npm init
Install below dependencies .
npm install mongoose express cors body-parser
Automate server re-starting process by installing nodemon.
npm install nodemon --save-dev
Create MongoDB Database
Create backend/database/db.js file in backend folder and add the following code in the file.
module.exports = {
db: 'mongodb://localhost:27017/reactcheckbox'
};
Define Mongoose Schema
Create the models folder and create Checkbox.js file inside of it.
Add the following code in backend/models/Checkbox.js
file.
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
let checkboxModel = new Schema({
checkbox: {
type: String
},
}, {
collection: 'checkboxes'
});
module.exports = mongoose.model('CheckboxModel', checkboxModel);
Create Route to Post Checkbox Value
Now, we will create a separate folder for Express API, name it routes
and create a file by the name of check-save.routes.js
in it.
Add the following code in backend/routes/check-save.routes.js
file.
let mongoose = require('mongoose'),
express = require('express'),
checkboxRouter = express.Router();
let check = require('../models/Checkbox');
checkboxRouter.route('/checkbox-save').post((req, res, next) => {
check.create(req.body, (error, data) => {
if (error) {
return next(error)
} else {
console.log(data)
res.json(data)
}
})
});
checkboxRouter.route('/').get((req, res) => {
check.find((error, data) => {
if (error) {
return next(error)
} else {
res.json(data)
}
})
})
module.exports = checkboxRouter;
Set up Server.js
Set up Node and Express server settings, create server.js
file in the backend
root folder.
let express = require('express');
let mongoose = require('mongoose');
let cors = require('cors');
let bodyParser = require('body-parser');
let database = require('./database/db');
const checkboxRoute = require('../backend/routes/check-save.routes')
mongoose.Promise = global.Promise;
mongoose.connect(database.db, {
useNewUrlParser: true
}).then(() => {
console.log('Database connected sucessfully !')
},
error => {
console.log('Database could not be connected : ' + error)
}
)
const app = express();
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({
extended: true
}));
app.use(cors());
app.use('/api', checkboxRoute)
const port = process.env.PORT || 4000;
const server = app.listen(port, () => {
console.log('Connected to port ' + port)
})
app.use((req, res, next) => {
next(createError(404));
});
app.use(function (err, req, res, next) {
console.error(err.message);
if (!err.statusCode) err.statusCode = 500;
res.status(err.statusCode).send(err.message);
});
Start Node/Express Server
Start the Node/Express server and MongoDB database by using the following commands.
Start the mongoDB, ENTER the follwong command in the terminal.
mongod
Open, other terminal and run the below command to start the nodemon service:
nodemon server
# [nodemon] 1.19.1
# [nodemon] to restart at any time, enter `rs`
# [nodemon] watching: *.*
# [nodemon] starting `node server`
# Connected to port 4000
# Database connected sucessfully !
Now, your Node server is up and running. You can check your server data on the following URL: http://localhost:4000/api
React Convert Checked Value of Checkbox into String
In this step, we will convert the checked value of a checkbox into the string and then save that value into the MongoDB database.
onSubmit = (e) => {
e.preventDefault();
let checkArray = [];
for (var key in this.state) {
if (this.state[key] === true) {
checkArray.push(key);
}
}
let checkData = {
checkbox: checkArray.toString()
};
}
Here, we set up an empty array; then we are iterating over the checkboxes state using the for a loop. With the help of the for loop, we are checking if the value is checked, then saving that checked value of checkbox in the checkArray’s array. Then we defined the checkData
object and passed the `checkbox`
as a key in respect to the value specified in the checkboxModel schema and converted the checked values from the array into the string.
Make Axios POST Request to Store Multiple Checkbox Values in MongoDB Database
To make the Axios POST request, first import the Axios library in App.js file.
Go to the src/App.js
file and insert the following code inside the onSubmit method as mentioned below.
import React, { Component } from 'react';
import '../node_modules/bootstrap/dist/css/bootstrap.min.css';
import axios from 'axios';
class App extends Component {
// Checkbox Initial State
state = {
isApple: false,
isOrange: false,
isBanana: false,
isCherry: false,
isAvocado: false
};
// React Checkboxes onChange Methods
onChangeApple = () => {
this.setState(initialState => ({
isApple: !initialState.isAvocado,
}));
}
onChangeOrange = () => {
this.setState(initialState => ({
isOrange: !initialState.isOrange,
}));
}
onChangeBanana = () => {
this.setState(initialState => ({
isBanana: !initialState.isBanana,
}));
}
onChangeCherry = () => {
this.setState(initialState => ({
isCherry: !initialState.isCherry,
}));
}
onChangeAvocado = () => {
this.setState(initialState => ({
isAvocado: !initialState.isAvocado,
}));
}
// Submit
onSubmit = (e) => {
e.preventDefault();
let checkArray = [];
for (var key in this.state) {
if (this.state[key] === true) {
checkArray.push(key);
}
}
let checkData = {
checkbox: checkArray.toString()
};
axios.post('http://localhost:4000/api/checkbox-save', checkData)
.then((res) => {
console.log(res.data)
}).catch((error) => {
console.log(error)
});
}
render() {
return (
<div className="App">
<h2>Store Multiple Checkboxes Values in React</h2>
<form onSubmit={this.onSubmit}>
<div className="form-check">
<label className="form-check-label">
<input type="checkbox"
checked={this.state.isApple}
onChange={this.onChangeApple}
className="form-check-input"
/>
Apple
</label>
</div>
<div className="form-check">
<label className="form-check-label">
<input type="checkbox"
checked={this.state.isAvocado}
onChange={this.onChangeAvocado}
className="form-check-input"
/>
Avocado
</label>
</div>
<div className="form-check">
<label className="form-check-label">
<input type="checkbox"
checked={this.state.isBanana}
onChange={this.onChangeBanana}
className="form-check-input"
/>
Banana
</label>
</div>
<div className="form-check">
<label className="form-check-label">
<input type="checkbox"
checked={this.state.isCherry}
onChange={this.onChangeCherry}
className="form-check-input"
/>
Cherry
</label>
</div>
<div className="form-check">
<label className="form-check-label">
<input type="checkbox"
checked={this.state.isOrange}
onChange={this.onChangeOrange}
className="form-check-input"
/>
Orange
</label>
</div>
<div className="form-group">
<button className="btn btn-success">
Save
</button>
</div>
</form>
</div>
);
}
}
export default App;
We used axios.post() method and passed the `api/checkbox-save`
REST API URL as a first parameter. In the second parameter, we passed the checkData object, which contains the checkbox values in the string form. When clicking on the Save button, we are saving the checkbox values in the MongoDB database.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK