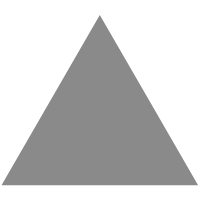
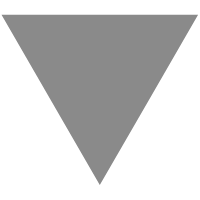
How To Set Multi Authentication in JWT
source link: https://www.laravelcode.com/post/how-to-set-multi-authentication-in-jwt
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
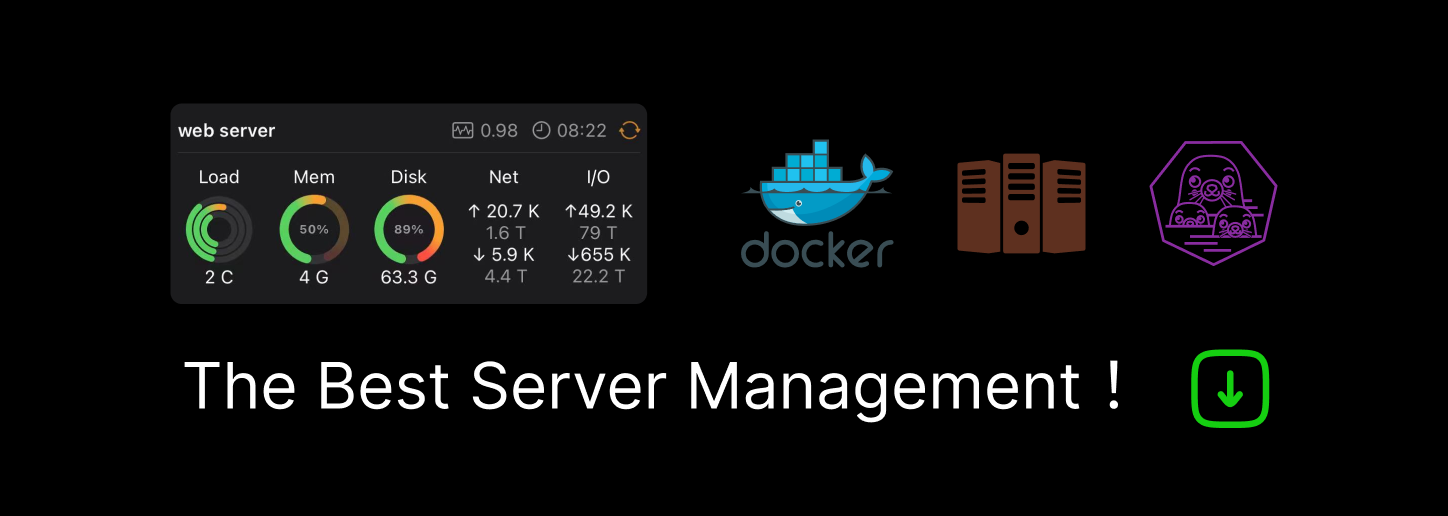
How To Set Multi Authentication in JWT
Today, laravelcode share with you how to set multi authentication in jwt with simple example. recently we are working with one laravel application and we are also built API for it. and we are required more then one table for authentication. you also see in JWT documentation it by default provide User model for authentication. but sometime we have required use another table also use for authentication in our API. for Ex. one for front API user and another for Admin API user.
This problem you easyly handle by help of this tutorials. please simple follow this step.
You may also check this link for JWT configure in laravel Restful API In Laravel 5.5 Using jwt Authentication
Suppose we have two table which we are want for authentication with JWT
1. users
2. admins
Step : 1 Add following route in routes/api.php
Route::post('auth/userlogin', 'ApiController@userLogin');
Route::post('auth/adminlogin', 'ApiController@adminLogin');
Step : 2 Create Controller
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
use App\Http\Requests;
use Config;
use JWTAuth;
use JWTAuthException;
use App\User;
use App\Admin;
class ApiController extends Controller
{
public function __construct()
{
$this->user = new User;
$this->admin = new Admin;
}
public function userLogin(Request $request){
Config::set('jwt.user', 'App\User');
Config::set('auth.providers.users.model', \App\User::class);
$credentials = $request->only('email', 'password');
$token = null;
try {
if (!$token = JWTAuth::attempt($credentials)) {
return response()->json([
'response' => 'error',
'message' => 'invalid_email_or_password',
]);
}
} catch (JWTAuthException $e) {
return response()->json([
'response' => 'error',
'message' => 'failed_to_create_token',
]);
}
return response()->json([
'response' => 'success',
'result' => [
'token' => $token,
'message' => 'I am front user',
],
]);
}
public function adminLogin(Request $request){
Config::set('jwt.user', 'App\Admin');
Config::set('auth.providers.users.model', \App\Admin::class);
$credentials = $request->only('email', 'password');
$token = null;
try {
if (!$token = JWTAuth::attempt($credentials)) {
return response()->json([
'response' => 'error',
'message' => 'invalid_email_or_password',
]);
}
} catch (JWTAuthException $e) {
return response()->json([
'response' => 'error',
'message' => 'failed_to_create_token',
]);
}
return response()->json([
'response' => 'success',
'result' => [
'token' => $token,
'message' => 'I am Admin user',
],
]);
}
}
[ADDCODE]
Step : 9 Test With Postman
You can test your API with postman and another API testing tool
Now we are ready to run our example so run bellow command ro quick run:
php artisan serve
Now you can open bellow URL on your browser:
http://localhost:8000
If you want to any problem then please write comment and also suggest for new topic for make tutorials in future. Thanks...
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK