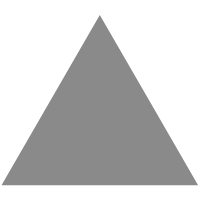
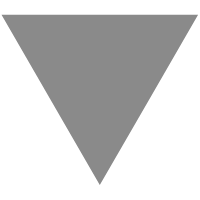
Difference Between React Component Tests and React Hook Tests
source link: https://blog.bitsrc.io/understanding-the-difference-between-component-tests-and-hook-tests-1477db6cef89
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
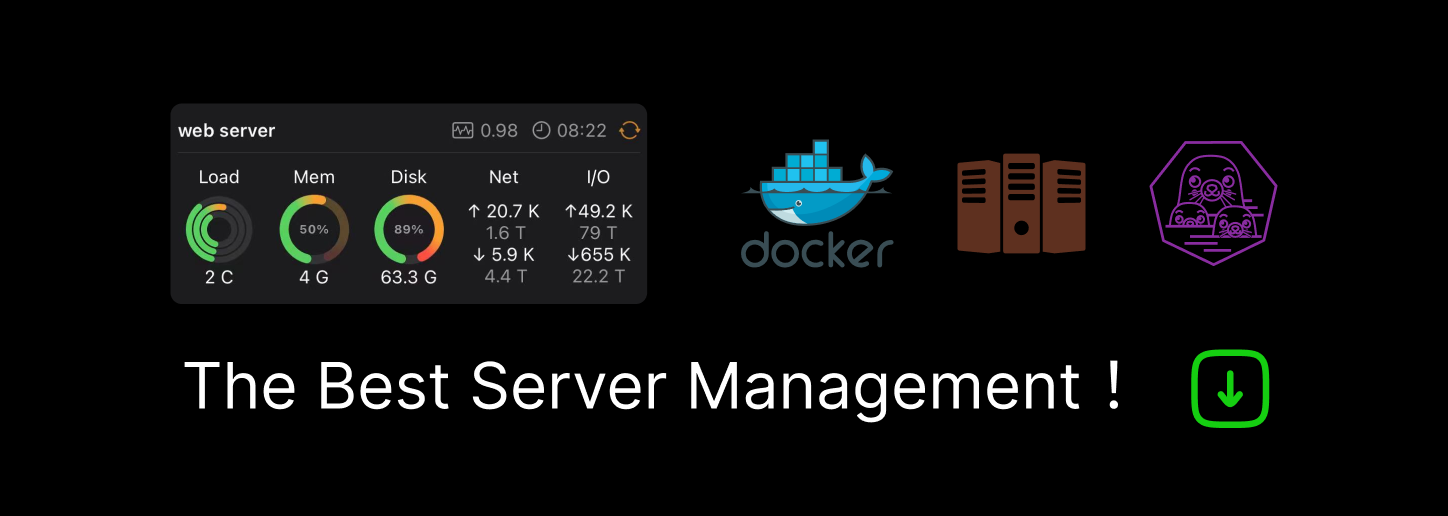
Difference Between React Component Tests and React Hook Tests

Testing helps ensure that your code is working correctly and helps prevent bugs from slipping into production. When it comes to testing React components and Hooks, it’s important to understand the difference between the two types of tests and when to use each one.
Component tests and Hook tests are similar in that they both test the behavior of your code. However, there are a few key differences between the two types of tests:
Scope
Component tests focus on testing the behavior of a single component, including the props that the component receives and the output that it produces. Hook tests, on the other hand, focus on testing the behavior of a single Hook, without any context of how the Hook is being used.
Component test:
import { render } from 'react-testing-library';
function TodoList() {
const todos = useTodos();
return (
<ul>
{todos.map(todo => (
<li key={todo.id}>
{todo.text}
<button onClick={() => removeTodo(todo.id)}>
Remove
</button>
</li>
))}
</ul>
);
}
test('TodoList displays todos and removes them when remove button is clicked', () => {
const todos = [ { id: 1, text: 'Task 1' }, { id: 2, text: 'Task 2' }, ];
const { getByText } = render(<TodoList />);
// Todos should be displayed
expect(getByText('Task 1').textContent).toBe('Task 1');
expect(getByText('Task 2').textContent).toBe('Task 2');
// Remove first todo
act(() => {
getByText('Remove').click();
});
// Only second todo should be displayed
expect(() => getByText('Task 1')).toThrow();
expect(getByText('Task 2').textContent).toBe('Task 2');
});
Component tests are designed to test the behavior of a single component(TodoList
) within the context of an application. This includes verifying that the component correctly renders its output and handles any interactions(Remove
) or events that it receives.
Hook test:
import { renderHook } from '@testing-library/react-hooks';
function useTodos() {
const [todos, setTodos] = useState([]);
function addTodo(text) {
setTodos([...todos, { id: uuid(), text }]);
}
function removeTodo(id) {
setTodos(todos.filter(todo => todo.id !== id));
}
return [todos, addTodo, removeTodo];
}
test('useTodos adds and removes todos', () => {
const { result } = renderHook(() => useTodos());
const [todos, addTodo, removeTodo] = result.current;
// Initial todos should be empty
expect(todos).toEqual([]);
// Add two todos
act(() => {
addTodo('Task 1');
addTodo('Task 2');
});
// Todos should contain two items
expect(todos.length).toBe(2);
// Remove first todo
act(() => {
removeTodo(todos[0].id);
});
// Todos should contain only one item
expect(todos.length).toBe(1);
});
This test uses the renderHook
function from the @testing-library/react-hooks
package to render the useTodos
Hook in isolation and test the values it returns and the side effects it produces. It tests the behavior of the addTodo
and removeTodo
functions, as well as the values of the todos
state.
Approach
Component tests are typically written using a testing library like Enzyme or React Testing Library, which allow you to render a component and interact with it in a way that simulates a user’s actions. In this example codes, The component test uses React’s render
function from the react-testing-library
package to render the TodoList
component and interact with it in a way that simulates a user's actions.
Hook tests, on the other hand, are typically written using the renderHook
utility from the @testing-library/react-hooks
package(and also using it within this examples), which allows you to test a Hook in isolation and test the values it returns and the side effects it produces.
Purpose
The purpose of component tests is to ensure that a component(TodoList
) is working correctly within the context of your application. This includes testing the output that the component produces, as well as the behavior of the removeTodo
function when the remove button is clicked.
The purpose of Hook tests is to ensure that a Hook is working correctly on its own, without any dependencies on other components or Hooks. In this examples it is used to ensure that the useTodos
Hook is working correctly on its own, without any dependencies on other components or Hooks. This includes testing the values that the Hook returns, as well as the side effects it produces when the addTodo
and removeTodo
functions are called.
Conclusions
So when should you use component tests and when should you use Hook tests? Here are a few guidelines to follow:
- Use component tests to ensure that a component is working correctly within the context of your application. This includes testing the props that the component receives, the output that it produces, and the behavior of the component.
- Use Hook tests to ensure that a Hook is working correctly on its own. This includes testing the values and functions that the Hook returns, as well as the side effects that it produces.
By understanding the difference between component tests and Hook tests and when to use each one, you can write reliable and maintainable tests for your React code. Happy testing!
Build apps with reusable React components like Lego
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK