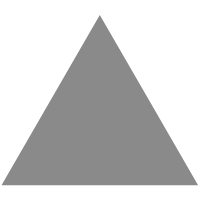
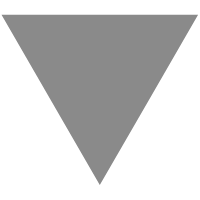
How to detect Internet speed in JavaScript
source link: https://www.laravelcode.com/post/how-to-detect-internet-speed-in-javascript
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
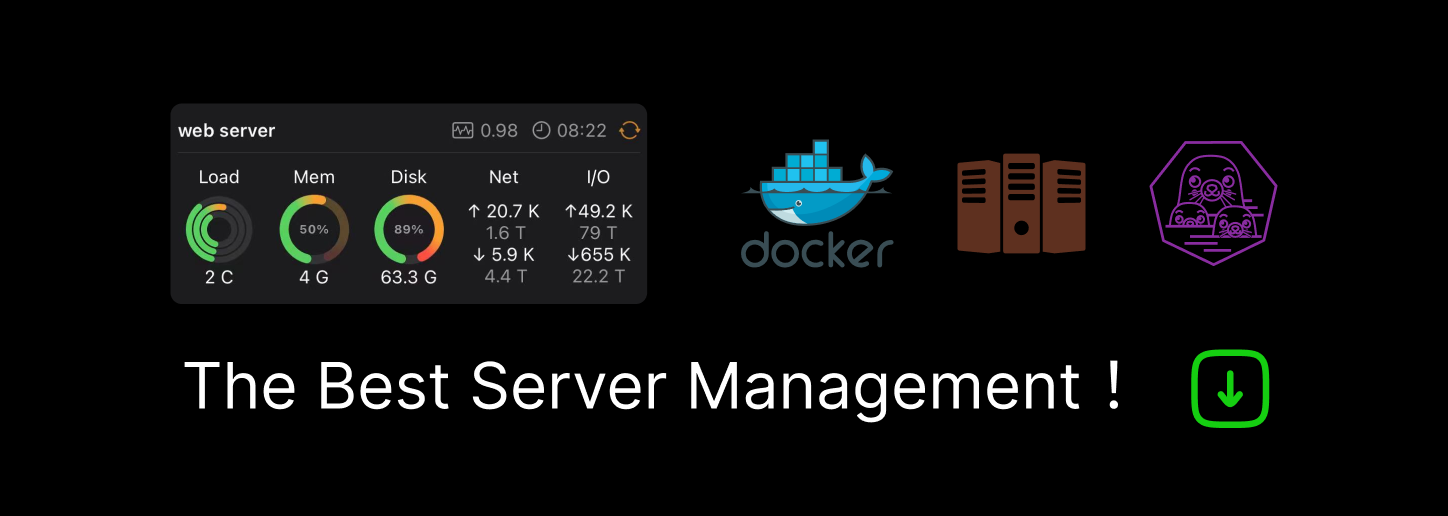
How to detect Internet speed in JavaScript
Hello guys,
In this article we will share how you can test your internet connection using Javascript. Javascript is best option to test client Internet speed test. We will use download image file approach to calculate the time for downloading for that image size.
To start with tutorial, first create a empty html file index.html and put the below code into it:
Here is the code example.
<!DOCTYPE html>
<html>
<head>
<title>Internet speed test</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
</head>
<body>
<div class="container">
<div class="row text-center my-5">
<button class="btn btn btn-success btn-lg" id="speed-check">Start</button>
</div>
<div id="result"></div>
</div>
<script type="text/javascript">
var speedButton = document.getElementById('speed-check');
speedButton.addEventListener('click', InitiateSpeedDetection, false);
var imageAddr = "https://hackthestuff.com/images/test.jpg";
var downloadSize = 13055440;
function ShowProgressMessage(msg) {
document.getElementById('result').innerHTML = msg;
}
function InitiateSpeedDetection() {
ShowProgressMessage("Checking speed, please wait...");
window.setTimeout(MeasureConnectionSpeed, 0);
};
function MeasureConnectionSpeed() {
var startTime, endTime;
var download = new Image();
download.onload = function () {
endTime = (new Date()).getTime();
showResults();
}
download.onerror = function (err, msg) {
ShowProgressMessage("Invalid image, or error downloading");
}
startTime = (new Date()).getTime();
var cacheBuster = "?nnn=" + startTime;
download.src = imageAddr + cacheBuster;
function showResults() {
var duration = (endTime - startTime) / 1000;
var bitsLoaded = downloadSize * 8;
var speedBps = (bitsLoaded / duration).toFixed(2);
var speedKbps = (speedBps / 1024).toFixed(2);
var speedMbps = (speedKbps / 1024).toFixed(2);
if (speedMbps > 1) {
ShowProgressMessage("Your connection speed is "+speedMbps+" Mbps");
} else if (speedKbps > 1) {
ShowProgressMessage("Your connection speed is "+speedKbps+" kbps");
} else {
ShowProgressMessage("Your connection speed is "+speedBps+" bps");
}
}
}
</script>
</body>
</html>
This is not up to accurate result same as real connection speed. The larger the file size you use, the more accurate result you get.

Also make sure the image you use should be properly optimized and compressed. Otherwise the speed result might be bigger than actual. The .jpg format file is good for that. You can use the image file we used in the example.
I hope you liked this article.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK