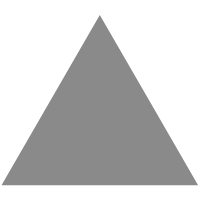
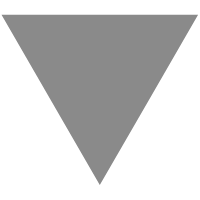
What are javascript symbols and how can they help you?
source link: https://www.ma-no.org/en/programming/javascript/what-are-javascript-symbols-and-how-can-they-help-you
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Web Design and Web Development news, javascript, angular, react, vue, php
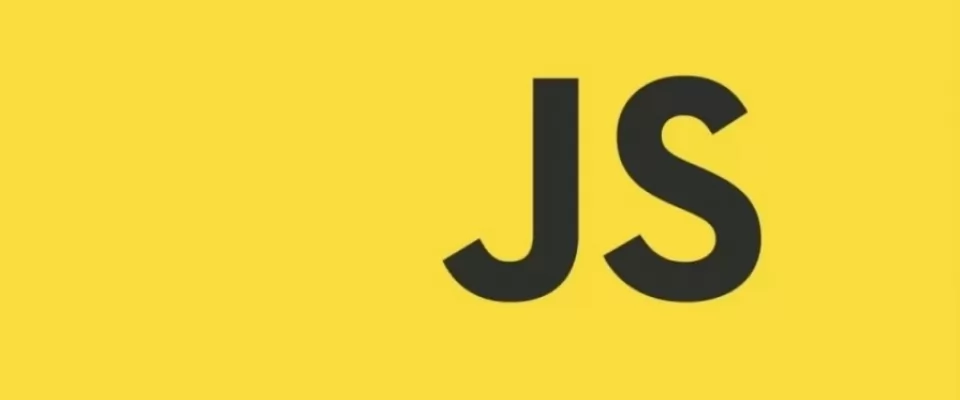
by Janeth Kent
Date: 20-12-2022 javascript
Symbols are a new primitive value introduced by ES6. Their purpose is to provide us unique identifiers. In this article, we tell you how they work, in which way they are used with JavaScript and how they can help us.
How to create symbols
To create a new symbol we will have to use its constructor:
const symbol = Symbol();
The function has an optional string parameter that acts as a description.
const symbol = Symbol('description'); console.log(symbol);//Symbol(description)
The important thing is that, even if you use the same description more than once, each symbol is unique.
Symbol('description') === Symbol('description'); //false
As I said before, symbols are new primitive values and have their own type. We can check this by using typeof:
typeof Symbol(); //symbol
Converting to symbol type
You already know that in Javascript it is possible to convert types. A big part of this is the implicit conversion, that happens when we use values of different types together:
console.log(1 + " added to " + 2 + " equals " + 3); //1 added to 2 equals 3
Although there are types that are compatible, this is not the case for symbols.
const symbol = Symbol('Hello'); symbol + 'world!'; //Uncaught TypeError: Cannot convert a Symbol value to a string
If you want to use symbols in this way, you will have to convert it or use the "description" property.
const symbol = Symbol('Hello'); console.log(`${symbol.description} world!`); //Hello world!
How to use symbols
Before we dive into symbols, it goes without saying that the keys of an object could only be strings. Trying to use an object as a key for a property does not return an expected result.
const key = {}; const myObject = { [key]: 'Hello world!' }; console.log(myObject); /* { [object Object]: 'Hello world!' } */
This is not the case for symbols. The ECMAScript specification states that we can use them as keys. So let's give it a try.
const key = Symbol(); const myObject = { [key]: 'Hello world!' }; console.log(myObject); /* { Symbol(): 'Hello world!' } */
Even if two symbols have the same description, they will not overlap when used as keys:
const key = Symbol('key'); const myObject = { [key]: 'Hello world!' }; console.log(myObject[key] === myObject[Symbol('key')]); //false
This means that we can assign an unlimited number of unique symbols and not worry about conflicts between them.
Accessing the value
Now, the only way to access our value is to use the symbol.
console.log(myObject[key]); // Hello world!
There are some differences when it comes to iterating through the properties of an object with symbols. The Object.keys, Object.entries and Object.entries functions do not give us access to any values that use symbols, the same is true for a for ... in. The easiest way to iterate through them is to use the Object.getOwnPropertySymbols function.
const key = Symbol(); const myObject = { [key]: 'Hello world!' }; Object.getOwnPropertySymbols(myObject) .forEach((symbol) => { console.log(myObject[symbol]); }); //Hello world!
Judging from the above, we can conclude that the symbols provide us with a hidden layer underneath the object, separate from the keys which are strings.
Symbols are unique... at least, most of the time.
The way to create a global symbol is to use the Symbol.for function.
const symbol = Symbol.for('key'); console.log(symbol === Symbol.for('key')); //true
If you have doubts about whether a symbol is unique, you can use the Symbol.keyFor function. It returns the associated key if found.
Symbol.keyFor( Symbol.for('key') ); //key
Symbol.keyFor( Symbol('key') ); //undefined
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK