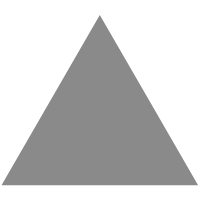
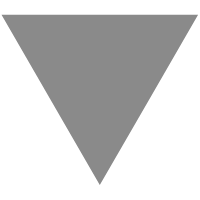
Angular Form Library | Getting Started Guide
source link: https://surveyjs.io/form-library/documentation/get-started-angular
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
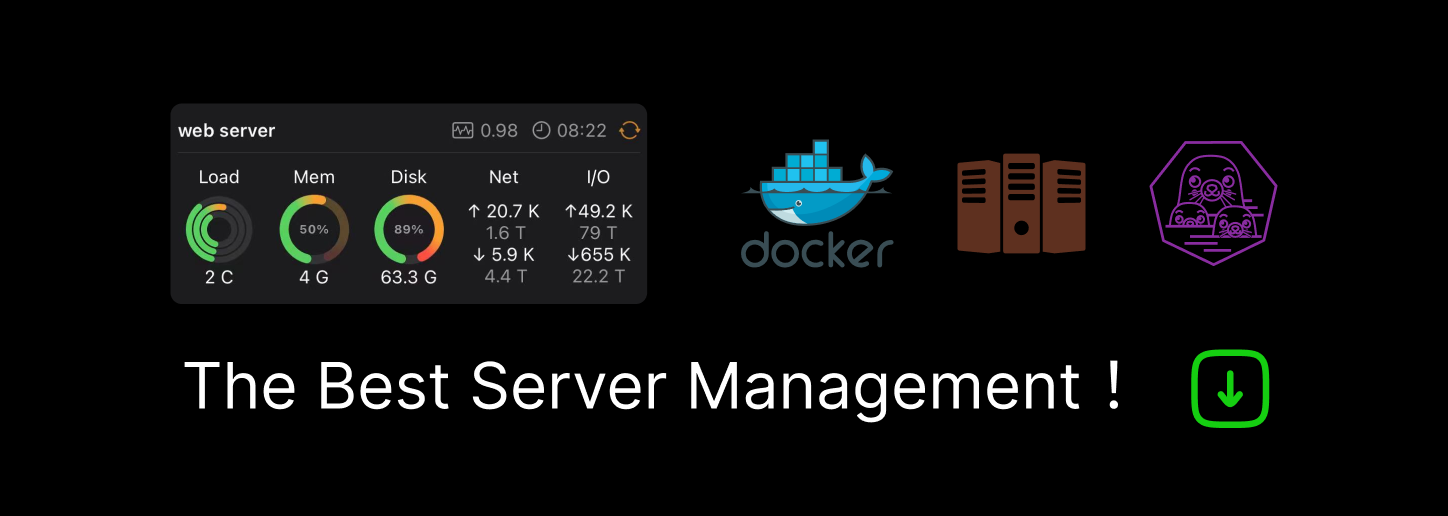
Add a Survey to an Angular Application
This step-by-step tutorial will help you get started with the SurveyJS Form Library in an Angular application. To add a survey to your Angular application, follow the steps below:
As a result, you will create a survey displayed below:
Install the survey-angular-ui
npm Package
The SurveyJS Form Library for Angular consists of two npm packages: survey-core
(platform-independent code) and survey-angular-ui
(rendering code). Run the following command to install survey-angular-ui
. The survey-core
package will be installed automatically because it is listed in survey-angular-ui
dependencies.
npm install survey-angular-ui --save
SurveyJS for Angular requires Angular v13.0.0 or newer and depends on the
@angular/cdk
package. If your project does not include it yet, run the following command:npm install @angular/cdk@^13.0.0 --save
Configure Styles
SurveyJS ships with the Modern and Default V2 UI themes illustrated below.
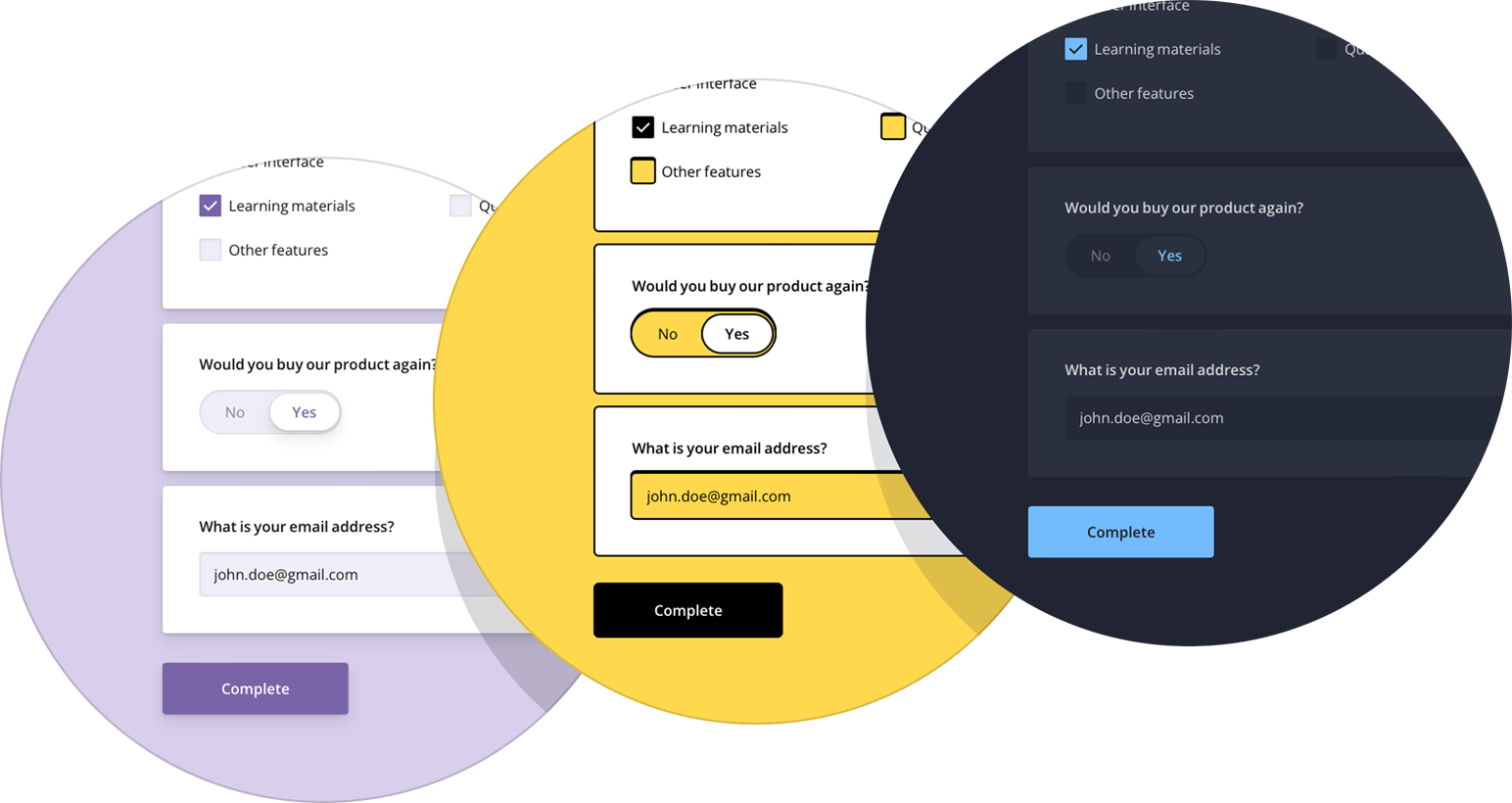
Open the angular.json
file and reference a style sheet that implements the required theme:
{
"$schema": "./node_modules/@angular/cli/lib/config/schema.json",
// ...
"projects": {
"project-name": {
"projectType": "application",
// ...
"architect": {
"build": {
// ...
"options": {
// ...
"styles": [
"src/styles.css",
// Default V2 theme
"node_modules/survey-core/defaultV2.min.css",
// Modern theme
// "node_modules/survey-core/modern.min.css"
],
// ...
}
}
}
}
}
}
To apply the referenced theme, call the applyTheme(themeName)
method. Depending on the theme, pass "modern"
or "defaultV2"
as the method's argument. For instance, the following code applies the Default V2 theme:
import { Component } from '@angular/core';
import { StylesManager } from "survey-core";
StylesManager.applyTheme("defaultV2");
@Component({
// ...
})
export class AppComponent {
// ...
}
Create a Model
A model describes the layout and contents of your survey. The simplest survey model contains one or several questions without layout modifications.
Models are specified by model schemas (JSON objects). For example, the following model schema declares two textual questions, each with a title and a name. Titles are displayed on screen. Names are used to identify the questions in code.
const surveyJson = {
elements: [{
name: "FirstName",
title: "Enter your first name:",
type: "text"
}, {
name: "LastName",
title: "Enter your last name:",
type: "text"
}]
};
To instantiate a model, pass the model schema to the Model constructor as shown in the code below. Assign the model instance to a component property. The model instance will be later used to render the survey.
import { Component, OnInit } from '@angular/core';
import { ..., Model } from "survey-core";
@Component({
// ...
})
export class AppComponent implements OnInit {
surveyModel: Model;
ngOnInit() {
const survey = new Model(surveyJson);
this.surveyModel = survey;
}
}
View Full Code
import { Component, OnInit } from '@angular/core';
import { Model, StylesManager } from "survey-core";
StylesManager.applyTheme("defaultV2");
const surveyJson = {
elements: [{
name: "FirstName",
title: "Enter your first name:",
type: "text"
}, {
name: "LastName",
title: "Enter your last name:",
type: "text"
}]
};
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
title = 'My First Survey';
surveyModel: Model;
ngOnInit() {
const survey = new Model(surveyJson);
this.surveyModel = survey;
}
}
Render the Survey
Before you render the survey, you need to import the module that integrates the SurveyJS Form Library with Angular. Open your NgModule class (usually resides in the app.module.ts
file), import the SurveyModule
from survey-angular-ui
, and list it in the imports
array.
// app.module.ts
// ...
import { SurveyModule } from "survey-angular-ui";
@NgModule({
declarations: [ ... ],
imports: [
...,
SurveyModule
],
providers: [ ... ],
bootstrap: [ ... ]
})
export class AppModule { }
To render a survey, add a <survey>
element to your component template and bind the element's model
attribute to the model instance you created in the previous step:
<survey [model]="surveyModel"></survey>
If you replicate the code correctly, you should see the following survey:
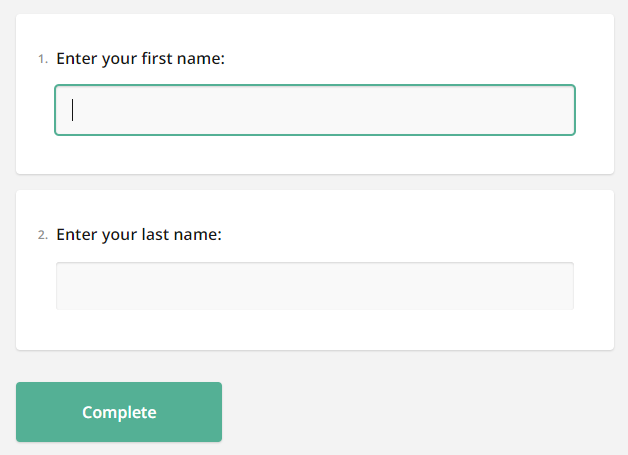
View Full Code
<!-- app.component.html -->
<survey [model]="surveyModel"></survey>
// app.component.ts
import { Component, OnInit } from '@angular/core';
import { Model, StylesManager } from "survey-core";
StylesManager.applyTheme("defaultV2");
const surveyJson = {
elements: [{
name: "FirstName",
title: "Enter your first name:",
type: "text"
}, {
name: "LastName",
title: "Enter your last name:",
type: "text"
}]
};
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
title = 'My First Survey';
surveyModel: Model;
ngOnInit() {
const survey = new Model(surveyJson);
this.surveyModel = survey;
}
}
// app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { SurveyModule } from "survey-angular-ui";
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
SurveyModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Handle Survey Completion
After a respondent completes a survey, the results are available within the onComplete event handler. In real-world applications, you should send the results to a server where they will be stored in a database and processed:
import { Component, OnInit } from '@angular/core';
import { ..., Model } from "survey-core";
const SURVEY_ID = 1;
@Component({
// ...
})
export class AppComponent implements OnInit {
surveyComplete (sender) {
saveSurveyResults(
"https://your-web-service.com/" + SURVEY_ID,
sender.data
)
}
ngOnInit() {
const survey = new Model(surveyJson);
survey.onComplete.add(this.surveyComplete);
// ...
}
}
function saveSurveyResults(url, json) {
const request = new XMLHttpRequest();
request.open('POST', url);
request.setRequestHeader('Content-Type', 'application/json;charset=UTF-8');
request.addEventListener('load', () => {
// Handle "load"
});
request.addEventListener('error', () => {
// Handle "error"
});
request.send(JSON.stringify(json));
}
In this tutorial, the results are simply output in an alert dialog:
import { Component, OnInit } from '@angular/core';
import { ..., Model } from "survey-core";
@Component({
// ...
})
export class AppComponent implements OnInit {
alertResults (sender) {
const results = JSON.stringify(sender.data);
alert(results);
}
ngOnInit() {
// ...
const survey = new Model(surveyJson);
survey.onComplete.add(this.alertResults);
// ...
}
}
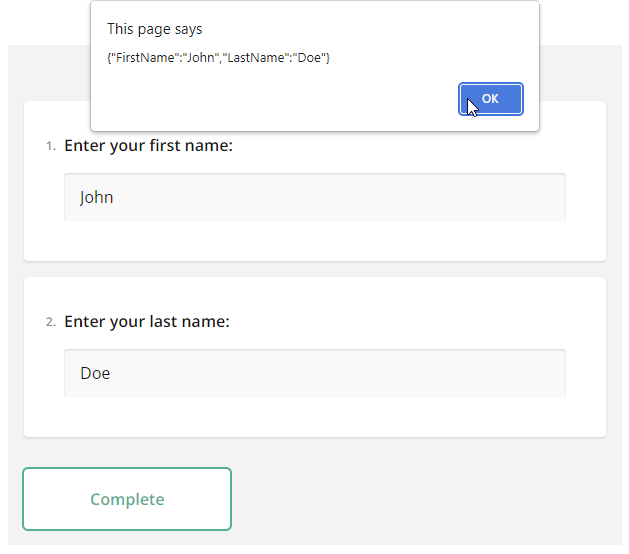
As you can see, survey results are saved in a JSON object. Its properties correspond to the name
property values of your questions in the model schema.
To view the application, run ng serve
in a command line and open http://localhost:4200/ in your browser.
View Full Code
<!-- app.component.html -->
<survey [model]="surveyModel"></survey>
// app.component.ts
import { Component, OnInit } from '@angular/core';
import { Model, StylesManager } from "survey-core";
StylesManager.applyTheme("defaultV2");
const surveyJson = {
elements: [{
name: "FirstName",
title: "Enter your first name:",
type: "text"
}, {
name: "LastName",
title: "Enter your last name:",
type: "text"
}]
};
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
title = 'My First Survey';
surveyModel: Model;
alertResults (sender) {
const results = JSON.stringify(sender.data);
alert(results);
}
ngOnInit() {
const survey = new Model(surveyJson);
survey.onComplete.add(this.alertResults);
this.surveyModel = survey;
}
}
// app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { SurveyModule } from "survey-angular-ui";
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
SurveyModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Further Reading
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK