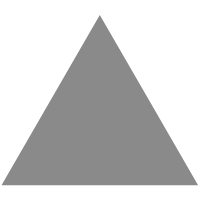
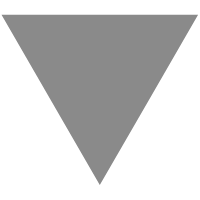
How to Pass PHP Data and Strings to JavaScript in WordPress
source link: https://code.tutsplus.com/tutorials/how-to-pass-php-data-and-strings-to-javascript-in-wordpress--wp-34699
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
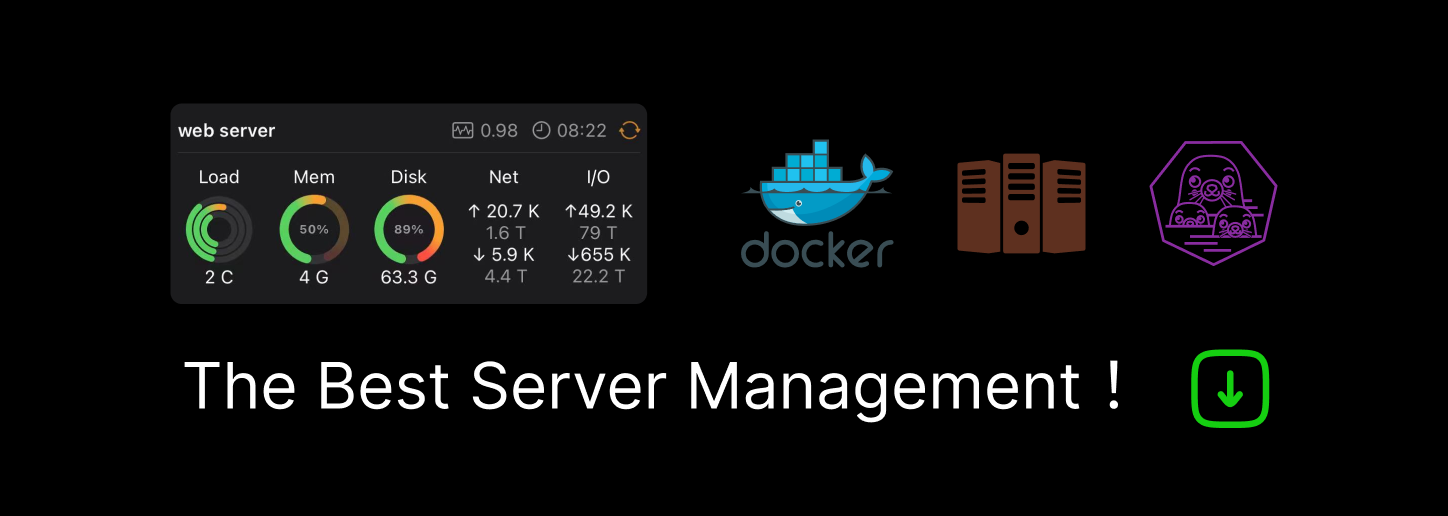
How to Pass PHP Data and Strings to JavaScript in WordPress
Read Time: 4 min
It's good practice to put all your data in static strings in your PHP files. If you need to use some data in JavaScript later on, it's also good practice to put your data in your HTML as data-*
attributes. But in some certain scenarios, you have no choice but to pass strings directly to your JavaScript code.
If you are including a JavaScript library, and you've found yourself initializing a JavaScript object inside your header.php and then assigning data to its properties, then this article is for you.
This article will teach you how to properly pass PHP data and static strings to your JavaScript library.
Why the Need to Pass Data to JavaScript
Let me illustrate some typical scenarios for the need to pass data to JavaScript. For instance, sometimes we need to get these values into your JavaScript code:
- homepage, admin, plugin, theme, or AJAX URLs
- translatable strings
- a theme or WordPress option
The Common Way of Passing Data
Let's say we have a jQuery file called myLibrary.js which we will include in our WordPress site:
var myLibraryObject; ( function ($) { "use strict" ; myLibraryObject = { home: '' , // populate this later pleaseWaitLabel: '' , // populate use this later someFunction: function () { // code which uses the properties above } } }); |
We enqueue it in our functions.php with the following code:
wp_enqueue_script( 'my_js_library' , get_template_directory_uri() . '/js/myLibrary.js' ); |
Now, the question is: how can we populate the home
and pleaseWaitLabel
properties? You might have instinctively added something like this in your header.php to get the data you need:
<script> ( function ( $ ) { "use strict" ; $( function () { myLibraryObject.home = '<?php echo get_stylesheet_directory_uri() ?>' ; myLibraryObject.pleaseWaitLabel = '<?php _e( ' Please wait... ', ' default ' ) ?>' ; }); }(jQuery)); </script> |
This works as intended, but there is a better and shorter way to do this.
The WordPress Way
In this section, we'll discuss a couple of different ways to understand how it works in WordPress.
The Old Way: Use the wp_localize_script
Function
The old way of passing data to JavaScript is by using the wp_localize_script
function. This function is meant to be used after you enqueue a script using wp_enqueue_scripts
.
Let's have look at the syntax of this function and its arguments.
wp_localize_script( $handle , $objectName , $arrayOfValues ); |
-
$handle
. The handle to the enqueued script to bind the values to. -
$objectName
. The JavaScript object that will hold all the values of$arrayOfValues
. -
$arrayOfValues
. An associative array containing the name and values to be passed to the script.
After calling this function, the $objectName
variable will become available within the specified script.
Let's adjust the earlier example to use our new method of passing data. First, we enqueue the script, and then we call wp_localize_script
in our functions.php file:
wp_enqueue_script( 'my_js_library' , get_template_directory_uri() . '/js/myLibrary.js' ); $dataToBePassed = array ( 'home' => get_stylesheet_directory_uri(), 'pleaseWaitLabel' => __( 'Please wait...' , 'default' ) ); wp_localize_script( 'my_js_library' , 'php_vars' , $datatoBePassed ); |
Our home
and pleaseWaitLabel
values can now be accessed inside our jQuery library via the php_vars
variable.
Since we used wp_localize_script
, we won't have to run anything in our header.php, and we can safely remove the contents of the <script>
tag. We can also remove the additional properties from our jQuery script. It can now be simplified to this:
var myLibraryObject; ( function ($) { "use strict" ; myLibraryObject = { someFunction: function () { // code which uses php_vars.home and php_vars.pleaseWaitLabel } } }(jQuery)); |
The New Way: Use the wp_add_inline_script
Function
The new and recommended way is to use the wp_add_inline_script
function. This function should be used after you enqueue a script using wp_enqueue_scripts
.
Let's have look at the syntax and arguments of this function.
wp_add_inline_script( $handle , $data , $position = 'after' ); |
-
$handle
. Name of the script to add the inline script to. -
$data
. String containing the JavaScript to be added. -
$position
. Whether to add the inline script before the handle or after.
So this function will add an inline script before or after your JavaScript code.
Let's revise the example we discussed in the previous section with the wp_add_inline_script
version.
wp_enqueue_script( 'my_js_library' , get_template_directory_uri() . '/js/myLibrary.js' ); $dataToBePassed = array ( 'home' => get_stylesheet_directory_uri(), 'pleaseWaitLabel' => __( 'Please wait...' , 'default' ) ); wp_add_inline_script( 'wpdocs-my-script' , 'const php_vars = ' . json_encode( $dataToBePassed ), 'before' ); |
Now, our home
and pleaseWaitLabel
values can be accessed inside our jQuery library via the php_vars
variable.
Our variables in the JavaScript will be available as php_vars.home
and php_vars.pleaseWaitLabel
, as shown in the following snippet.
var myLibraryObject; ( function ($) { "use strict" ; myLibraryObject = { someFunction: function () { console.log(php_vars.home); console.log(php_vars.pleaseWaitLabel); } } }(jQuery)); |
So in this way, you can pass PHP data and strings to JavaScript in WordPress.
Conclusion
By using wp_add_inline_script
, our code is simpler, and our header.php is cleaner. Hopefully, you can use this function in your own code and enjoy its benefits.
This post has been updated with contributions from Sajal Soni. Sajal belongs to India, and he loves to spend time creating websites based on open-source frameworks.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK