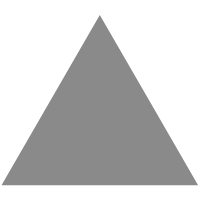
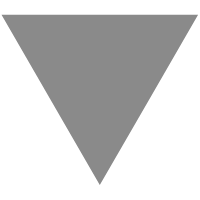
A Simple Web API Application that Echoes the Request for any URL
source link: https://nodogmablog.bryanhogan.net/2022/11/a-simple-web-api-application-that-echoes-the-request-for-any-url/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
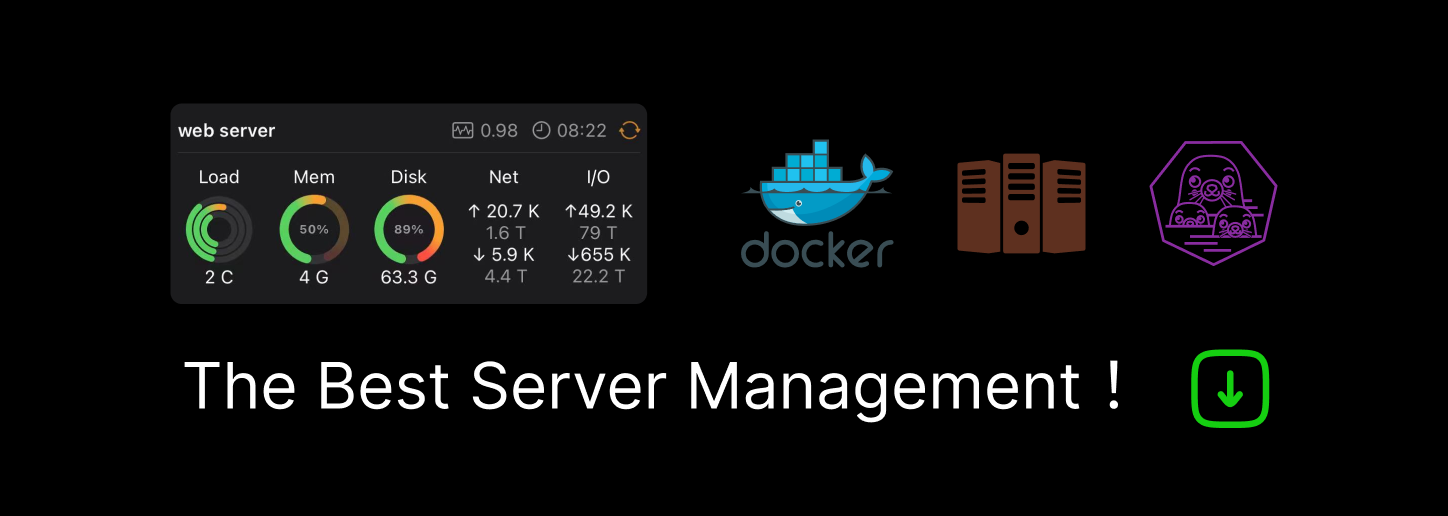
A Simple Web API Application that Echoes the Request for any URL
Download full source code.
There are times when playing with .NET that I want to make a simple HTTP request and get a response, this often happens to me when I’m trying to figure something out about HttpClient, HttpClientFactory, or Polly.
Of course, I can create a Web API application, and have it return some type. But then I probably want it to support the methods GET/PUT/POST/PATCH/DELETE, and even SEARCH (I like the SEARCH HTTP method).
Now it’s becoming a bit more work to program this up. And what if I want to make sure the correct headers are being sent and received? Now I need to process the incoming request and parse the headers.
Instead, I create a simple Web API application, and all it does is echo the request back in the response. A single endpoint or a single controller can handle all the HTTP methods, all URLs, and return as much as you want about the incoming request.
The Response
The first thing I need is a model to send the response. I’ll call it EchoResponse
, I’ve included the properties I’m interested in -
public class EchoResponse
{
public string Body { get; set; }
public IHeaderDictionary Headers { get; set; }
public string Method { get; set; }
public PathString Path {get; set; }
public QueryString QueryString { get; set; }
public IQueryCollection Query { get; set; }
}
Then I need an extension method to convert the HttpRequest
to an EchoResponse
-
public static class ExtensionMethods
{
public static async Task<EchoResponse> ToEchoResponse(this HttpRequest request)
{
var echoResponse = new EchoResponse();
using (StreamReader streamReader = new StreamReader(request.Body))
{
echoResponse.Body = await streamReader.ReadToEndAsync();
};
echoResponse.Method = request.Method;
echoResponse.Headers = request.Headers;
echoResponse.Path = request.Path;
echoResponse.QueryString = request.QueryString;
echoResponse.Query = request.Query;
return echoResponse;
}
}
That’s the hard part done!
Minimal API Endpoint
This will map all HTTP methods to the same endpoint, for all URLs -
app.Map("{*anyurl}", (HttpRequest httpRequest) =>
{
return httpRequest.ToEchoResponse();
});
That’s it! Simple!
Controller
If you want to use a controller instead of an endpoint, use the following -
using Microsoft.AspNetCore.Mvc;
[ApiController]
[Route("{*anyurl}")]
public class EchoController : ControllerBase
{
public async Task<EchoResponse> All()
{
return await Request.ToEchoResponse();
}
}
You should use only the minimal endpoint or the controller at one time.
Testing it out
Use tools such as Fiddler, Postman, or Rest Client for VS Code (I’ve included a sample file in the attached zip) to make requests to the API.
Download full source code.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK