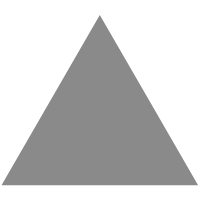
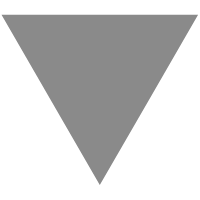
28 个Javascript 数组方法清单列表
source link: https://www.51cto.com/article/740505.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
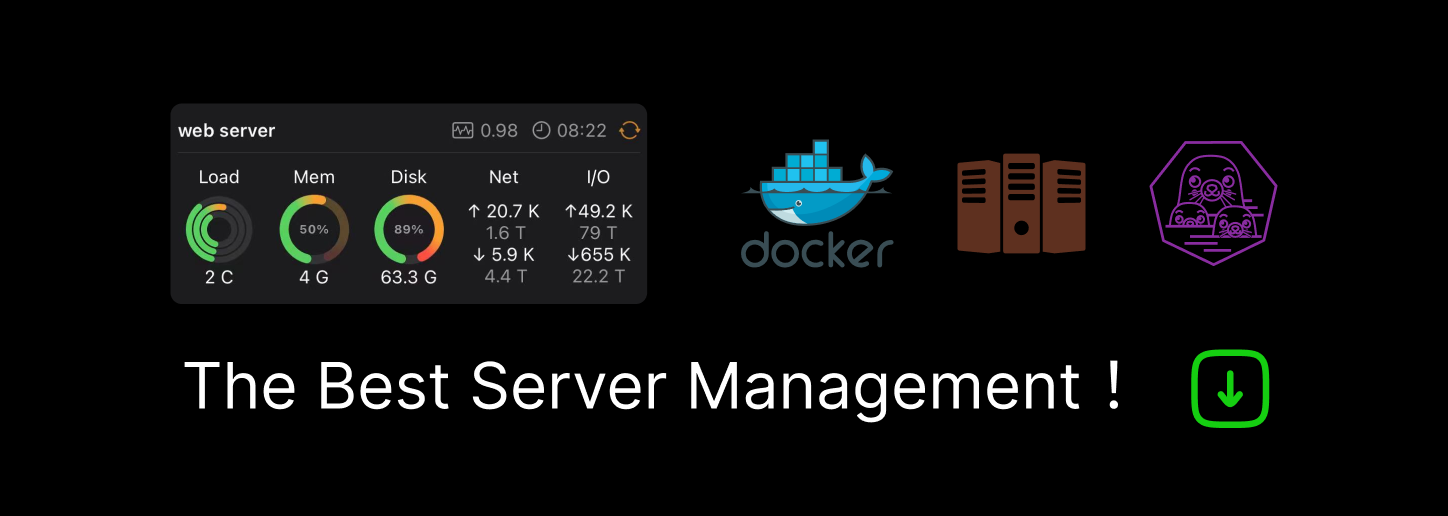
28 个Javascript 数组方法清单列表
数组,是JavaScript中常用的数据类型,是JavaScript程序设计中的重要内容,因此,今天我总结了28个JavaScript数组方法的实用清单,希望这些内容,能够对你学习JavaScript有所帮助。
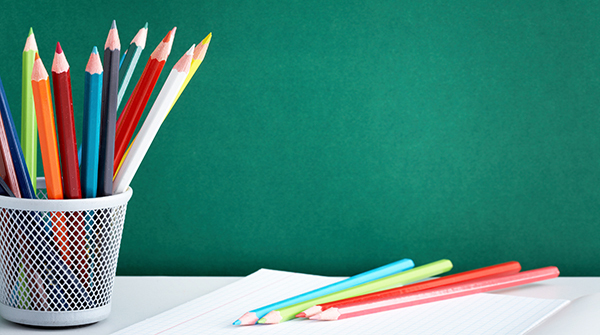
好了,我们现在就开始今天的内容吧。
01、Array.map()
返回一个新数组,其中包含对该数组中每个元素调用提供的函数的结果。
const list = [😫, 😫, 😫, 😫];
list.map((⚪️) => 😀); // [😀, 😀, 😀, 😀]
// Code
const list = [1, 2, 3, 4];
list.map((el) => el * 2); // [2, 4, 6, 8]
02、Array.filter()
返回一个新数组,其中包含通过所提供函数实现的测试的所有元素。
const list = [😀, 😫, 😀, 😫];
list.filter((⚪️) => ⚪️ === 😀); // [😀, 😀]
// Code
const list = [1, 2, 3, 4];
list.filter((el) => el % 2 === 0); // [2, 4]
03、Array.reduce()
将数组减少为单个值,函数返回的值存储在累加器中(结果/总计)。
const list = [😀, 😫, 😀, 😫, 🤪];
list.reduce((⬜️, ⚪️) => ⬜️ + ⚪️); // 😀 + 😫 + 😀 + 😫 + 🤪
// OR
const list = [1, 2, 3, 4, 5];
list.reduce((total, item) => total + item, 0); // 15
04、Array.reduceRight()
对数组的每个元素执行一个 reducer 函数(需要您提供),从而产生一个输出值(从右到左)。
const list = [😀, 😫, 😀, 😫, 🤪];
list.reduceRight((⬜️, ⚪️) => ⬜️ + ⚪️); // 🤪 + 😫 + 😀 + 😫 + 😀
// Code
const list = [1, 2, 3, 4, 5];
list.reduceRight((total, item) => total + item, 0); // 15
05、Array.fill()
用静态值填充数组中的元素。
const list = [😀, 😫, 😀, 😫, 🤪];
list.fill(😀); // [😀, 😀, 😀, 😀, 😀]
// Code
const list = [1, 2, 3, 4, 5];
list.fill(0); // [0, 0, 0, 0, 0]
06、Array.find()
返回数组中满足提供的测试函数的第一个元素的值,否则返回未定义。
const list = [😀, 😫, 😀, 😫, 🤪];
list.find((⚪️) => ⚪️ === 😀); // 😀
list.find((⚪️) => ⚪️ === 😝); // undefined
// Code
const list = [1, 2, 3, 4, 5];
list.find((el) => el === 3); // 3
list.find((el) => el === 6); // undefined
07、Array.indexOf()
返回可以在数组中找到给定元素的第一个索引,如果不存在则返回 -1。
const list = [😀, 😫, 😀, 😫, 🤪];
list.indexOf(😀); // 0
list.indexOf(😡); // -1
// Code
const list = [1, 2, 3, 4, 5];
list.indexOf(3); // 2
list.indexOf(6); // -1
08、Array.lastIndexOf()
返回可以在数组中找到给定元素的最后一个索引,如果不存在,则返回 -1。从 fromIndex 开始向后搜索数组。
const list = [😀, 😫, 😀, 😫, 🤪];
list.lastIndexOf(😀); // 3
list.lastIndexOf(😀, 1); // 0
// Code
const list = [1, 2, 3, 4, 5];
list.lastIndexOf(3); // 2
list.lastIndexOf(3, 1); // -1
09、Array.findIndex()
返回数组中满足提供的测试函数的第一个元素的索引。否则,返回 -1。
const list = [😀, 😫, 😀, 😫, 🤪];
list.findIndex((⚪️) => ⚪️ === 😀); // 0
// You might be thinking how it's different from `indexOf` 🤔
const array = [5, 12, 8, 130, 44];
array.findIndex((element) => element > 13); // 3
// OR
const array = [{
id: 😀
}, {
id: 😫
}, {
id: 🤪
}];
array.findIndex((element) => element.id === 🤪); // 2
10、Array.includes()
如果给定元素存在于数组中,则返回 true。
const list = [😀, 😫, 😀, 😫, 🤪];
list.includes(😀); // true
// Code
const list = [1, 2, 3, 4, 5];
list.includes(3); // true
list.includes(6); // false
11、Array.pop()
删除并返回数组的最后一个元素。
const list = [😀, 😫, 😀, 😫, 🤪];
list.pop(); // 🤪
list; // [😀, 😫, 😀, 😫]
// Code
const list = [1, 2, 3, 4, 5];
list.pop(); // 5
list; // [1, 2, 3, 4]
12、Array.push()
向数组的末尾添加一个或多个元素,并返回新的长度。
const list = [😀, 😫, 😀, 😫, 🤪];
list.push(😡); // 5
list; // [😀, 😫, 😀, 😫, 🤪, 😡]
// Code
const list = [1, 2, 3, 4, 5];
list.push(6); // 6
list; // [1, 2, 3, 4, 5, 6]
13、Array.shift()
删除并返回数组的第一个元素。
const list = [😀, 😫, 😀, 😫, 🤪];
list.shift(); // 😀
list; // [😫, 😀, 😫, 🤪]
// Code
const list = [1, 2, 3, 4, 5];
list.shift(); // 1
list; // [2, 3, 4, 5]
14、Array.unshift()
向数组的开头添加一个或者多个元素,并返回新的长度。
const list = [😀, 😫, 😀, 😫, 🤪];
list.unshift(😡); // 6
list; // [😡, 😀, 😫, 😀, 😫, 🤪]
// Code
const list = [1, 2, 3, 4, 5];
list.unshift(0); // 6
list; // [0, 1, 2, 3, 4, 5]
15、Array.splice()
通过删除或替换现有元素,或在适当位置添加新元素来更改数组的内容。
const list = [😀, 😫, 😀, 😫, 🤪];
list.splice(1, 2); // [😀, 😫]
list; // [😀, 😫, 🤪]
// Code
const list = [1, 2, 3, 4, 5];
list.splice(1, 2); // [2, 3]
list; // [1, 4, 5]
16、Array.slice()
将数组的一部分浅拷贝返回到从开始到结束(不包括结束)选择的新数组对象中,原始数组不会被修改。
const list = [😀, 😫, 😀, 😫, 🤪];
list.slice(1, 3); // [😫, 😀]
list; // [😀, 😫, 😀, 😫, 🤪]
// Code
const list = [1, 2, 3, 4, 5];
list.slice(1, 3); // [2, 3]
list; // [1, 2, 3, 4, 5]
17、Array.join()
将数组的所有元素连接成一个字符串。
const list = [😀, 😫, 😀, 😫, 🤪];
list.reverse(); // [🤪, 😫, 😀, 😫, 😀]
list; // [🤪, 😫, 😀, 😫, 😀]
// Code
const list = [1, 2, 3, 4, 5];
list.reverse(); // [5, 4, 3, 2, 1]
list; // [5, 4, 3, 2, 1]
18、Array.reverse()
此数组可以反转数组中元素的顺序。
const list = [😀, 😫, 😀, 😫, 🤪];
list.sort(); // [😀, 😀, 😫, 😫, 🤪]
// This make more sense 🤔
const array = ['D', 'B', 'A', 'C'];
array.sort(); // 😀 ['A', 'B', 'C', 'D']
// OR
const array = [4, 1, 3, 2, 10];
array.sort(); // 😧 [1, 10, 2, 3, 4]
array.sort((a, b) => a - b); // 😀 [1, 2, 3, 4, 10]
19、Array.sort()
对数组的元素进行就地排序并返回该数组,默认排序顺序是根据字符串 Unicode 代码点。
const list = [😀, 😫, 😀, 😫, 🤪];
list.some((⚪️) => ⚪️ === 😀); // true
list.some((⚪️) => ⚪️ === 😡); // false
// Code
const list = [1, 2, 3, 4, 5];
list.some((el) => el === 3); // true
list.some((el) => el === 6); // false
20、Array.some()
如果数组中至少有一个元素通过了提供的函数实现的测试,则返回 true。
const list = [😀, 😫, 😀, 😫, 🤪];
list.some((⚪️) => ⚪️ === 😀); // true
list.some((⚪️) => ⚪️ === 😡); // false
// Code
const list = [1, 2, 3, 4, 5];
list.some((el) => el === 3); // true
list.some((el) => el === 6); // false
21、Array.every()
如果数组中的所有元素都通过了提供的函数实现的测试,则返回 true。
const list = [😀, 😫, 😀, 😫, 🤪];
list.every((⚪️) => ⚪️ === 😀); // false
const list = [😀, 😀, 😀, 😀, 😀];
list.every((⚪️) => ⚪️ === 😀); // true
// Code
const list = [1, 2, 3, 4, 5];
list.every((el) => el === 3); // false
const list = [2, 4, 6, 8, 10];
list.every((el) => el%2 === 0); // true
22、Array.from()
从类数组或可迭代对象创建一个新数组。
const list = 😀😫😀😫🤪;
Array.from(list); // [😀, 😫, 😀, 😫, 🤪]
const set = new Set(['😀', '😫', '😀', '😫', '🤪']);
Array.from(set); // [😀, 😫, 🤪]
const range = (n) => Array.from({ length: n }, (_, i) => i + 1);
console.log(range(10)); // [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
23、Array.of()
使用可变数量的参数创建一个新数组,而不管参数的数量或类型。
const list = Array.of(😀, 😫, 😀, 😫, 🤪);
list; // [😀, 😫, 😀, 😫, 🤪]
// Code
const list = Array.of(1, 2, 3, 4, 5);
list; // [1, 2, 3, 4, 5]
24、Array.isArray()
如果给定值是一个数组,则返回 true。
Array.isArray([😀, 😫, 😀, 😫, 🤪]); // true
Array.isArray(🤪); // false
// Code
Array.isArray([1, 2, 3, 4, 5]); // true
Array.isArray(5); // false
25、Array.at()
返回指定索引处的值。
const list = [😀, 😫, 😀, 😫, 🤪];
list.at(1); // 😫
// Return from last 🤔
list.at(-1); // 🤪
list.at(-2); // 😫
// Code
const list = [1, 2, 3, 4, 5];
list.at(1); // 2
list.at(-1); // 5
list.at(-2); // 4
26、Array.copyWithin()
复制数组中的数组元素,并返回修改后的新数组。
const list = [😀, 😫, 😀, 😫, 🤪];
list.copyWithin(1, 3); // [😀, 😀, 🤪, 😫, 🤪]
const list = [😀, 😫, 😀, 😫, 🤪];
list.copyWithin(0, 3, 4); // [😫, 😫, 😀, 😫, 🤪]
// Code
const list = [1, 2, 3, 4, 5];
list.copyWithin(0, 3, 4); // [4, 2, 3, 4, 5]
第一个参数是开始复制元素的目标。
第二个参数是开始复制元素的索引。
第三个参数是停止复制元素的索引。
27、Array.flat()
返回一个新数组,其中所有子数组元素递归连接到指定深度。
const list = [😀, 😫, [😀, 😫, 🤪]];
list.flat(Infinity); // [😀, 😫, 😀, 😫, 🤪]
// Code
const list = [1, 2, [3, 4, [5, 6]]];
list.flat(Infinity); // [1, 2, 3, 4, 5, 6]
28、Array.flatMap()
返回通过将给定的回调函数应用于数组的每个元素而形成的新数组,
const list = [😀, 😫, [😀, 😫, 🤪]];
list.flatMap((⚪️) => [⚪️, ⚪️ + ⚪️ ]); // [😀, 😀😀, 😫, 😫😫, 😀, 😀😀, 😫, 😫😫, 🤪, 🤪🤪]
// Code
const list = [1, 2, 3];
list.flatMap((el) => [el, el * el]); // [1, 1, 2, 4, 3, 9]
以上就是我今天想跟你分享的28个JavaScript的数组方法,希望这些方法可以帮助你快速的学习JavaScript数组方法的知识,从而可以有效的提高开发效率,改善程序编写的方式。
如果你觉得我今天的内容,对你有帮助的话,请点赞我,关注我,并将此文与你的朋友们一起来分享它,也许能够帮助到他。
最后,感谢你的阅读,编程愉快!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK