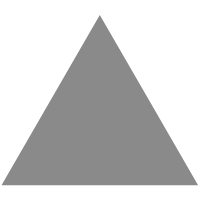
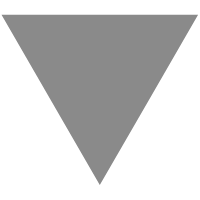
How to use the in, out, and ref keywords in .NET Core
source link: https://www.infoworld.com/article/3678688/how-to-use-the-in-out-and-ref-keywords-in-net-core.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to use the in, out, and ref keywords in .NET Core
Take advantage of the in, out, and ref keywords to pass parameters to your C# methods in .NET and make your code more readable and maintainable.
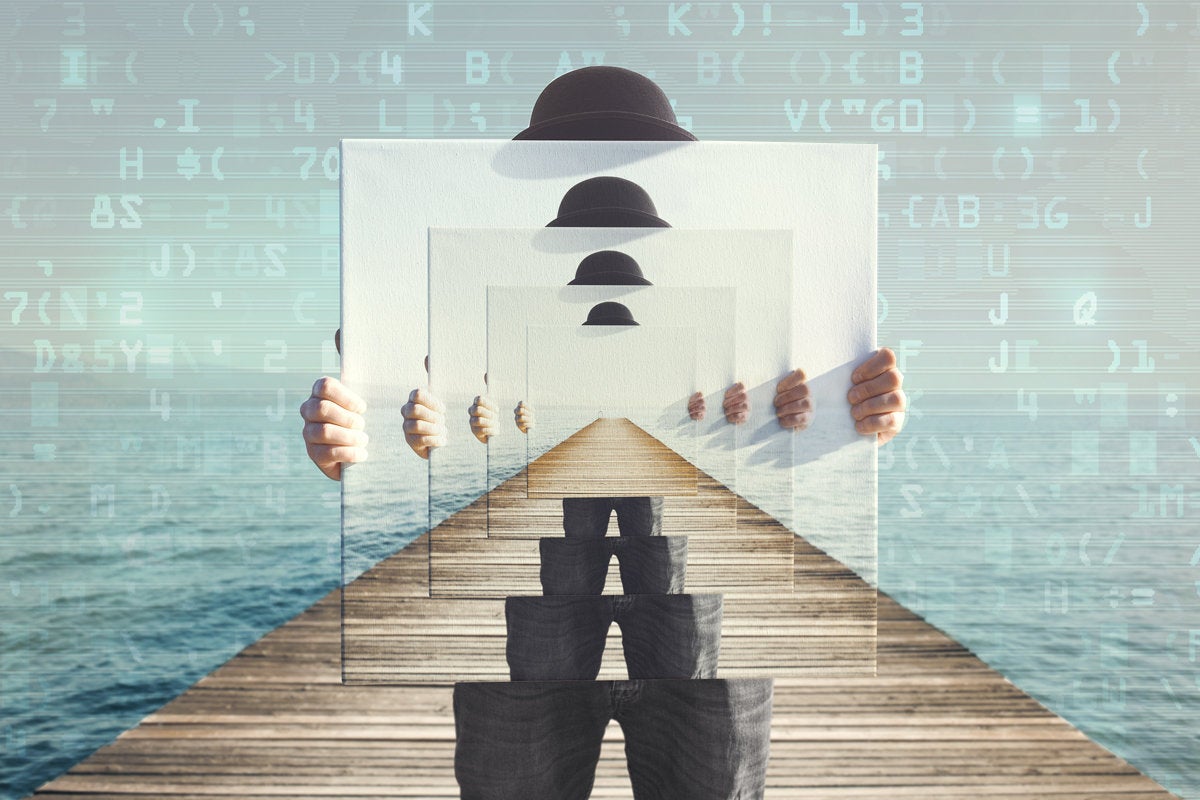
The in
, out
, and ref
keywords are widely used keywords in C#. They allow us to create better abstractions for data types and methods, which in turn makes our code more readable and maintainable.
Both the in
keyword and the out
keyword allow you to pass parameters to a method by reference. The out
keyword allows you to change the parameter values, whereas the in
keyword does not allow you to change them.
You can use the ref
keyword to pass input and output parameters by reference, allowing the called method to change those arguments if needed. By passing parameters by reference, the ref
keyword ensures that changes to the parameters within the method are reflected outside of the method as well.
In this article, we will explore each of these keywords in more detail so that you will know how and when to use them when working with C#. To work with the code examples provided in this article, you should have Visual Studio 2022 Preview installed in your system. If you don’t already have a copy, you can download Visual Studio 2022 Preview here.
Create a console application project in Visual Studio
First off, let’s create a .NET Core console application project in Visual Studio. Assuming Visual Studio 2022 Preview is installed in your system, follow the steps outlined below to create a new .NET Core console application project in Visual Studio.
- Launch the Visual Studio 2022 Preview IDE.
- Click on “Create new project.”
- In the “Create new project” window, select “Console App (.NET Core)” from the list of templates displayed.
- Click Next.
- In the “Configure your new project” window shown next, specify the name and location for the new project.
- Click Next
- In the “Additional information” window shown next, specify .NET 7 as the .NET version you would like to use.
- Click Create.
This will create a new .NET Core 7 console application project in Visual Studio 2022 Preview. We’ll use this project to work with the in
, out
, and ref
keywords in the subsequent sections of this article.
The ref keyword in C#
In C#, the passing of an object or variable by reference is accomplished with the use of the ref
keyword. Ordinarily, when a variable is sent to a method, the value of the variable is copied into the method so that it may be used further on.
However, if we use ref
to pass the variable by reference, the variable is not copied into the method. Instead, the method follows the reference to access the original variable. Hence any change the called method makes to the value of the variable will be made to the original variable.
It should be noted that you must specify the ref
keyword both in the method signature and at the point where the method is called. Consider the following code:
void RefDemo(ref int refParameter)
{
refParameter = refParameter + 1;
}
int number = 1;
RefDemo(ref number);
Console.WriteLine(number);
When you execute the preceding code, the number 2 will be displayed at the console. This is because the variable called number
has been passed by reference and the value of the refParameter
has been increased by 1 inside the RefDemo
method.
The following code snippet demonstrates how you can use the ref
keyword with objects.
void RefDemo(ref int refParameter)
{
refParameter = refParameter + 1;
}
int number = 1;
RefDemo(ref number);
Console.WriteLine(number);
When you execute the preceding code, the text “Hello World” will be displayed at the console.
Here is an interesting point. If you execute the following piece of code, the RefDemo
method will return true because the references of the str
and refParameter
objects are the same.
string str = "Hello";
string str = "Hello";
bool RefDemo(ref string refParameter)
{
refParameter = "Hello World";
return ReferenceEquals(str, refParameter);
}
bool isEqual = RefDemo(ref str);
Console.WriteLine(isEqual? "The two references are equal":"The two references are not equal");
The in keyword in C#
The in
keyword in C# is used to specify that a method parameter is passed by reference, but the called method cannot modify the argument. This is useful for parameters that are not modified by the called method, but must be passed by reference in order for the calling method to access the results.
Figure 1 shows you can’t change the value of an in parameter.
IDG
Figure 1. Nope! The in
keyword doesn’t allow parameter values to be changed.
The out keyword in C#
The out
keyword works much the same way as the ref keyword; it allows you to pass parameters using references and to change the values of those parameters as well. The out
keyword is identical to the ref
keyword, with the exception that ref
needs the variable to be initialized prior to being passed. When working with the out
keyword in C#, both the method signature and the calling method must explicitly specify the out
keyword.
For example, a method might need to return both a success code and a value. By using an output parameter for the variable (say, retValue
), the method can return the success code directly and use the output parameter for the value.
The following code snippet illustrates how you can work with the out
keyword in C#.
int number;
OutDemo(out number);
Console.WriteLine(number); //The value is now 100
void OutDemo(out int number)
{
number = 100;
}
When you execute the preceding code, the value 100 will be displayed at the console.
Limitations of in, out, and ref
You cannot use the in
, out
, and ref
keywords in async methods or iterator methods, such as those that use a yield return
or yield break
statement. Additionally, you cannot use the in
keyword in the first parameter to an extension method unless the parameter is a struct
.
It is pertinent to note that the keywords in
, ref
, and out
do not form part of the method signature when determining overloads. Hence, you cannot overload methods that differ in signature only with respect to these keywords.
In other words, if you have two methods with the same name, but one of them accepts an integer as an in
parameter and the other accepts an integer as an out
parameter, your code won’t compile at all.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK