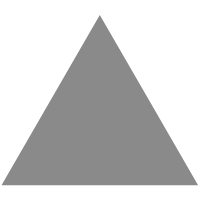
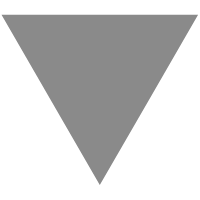
React Nesting Components: Rules to Follow
source link: https://blog.bitsrc.io/react-nesting-components-rules-to-follow-c0658ee6ef5
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
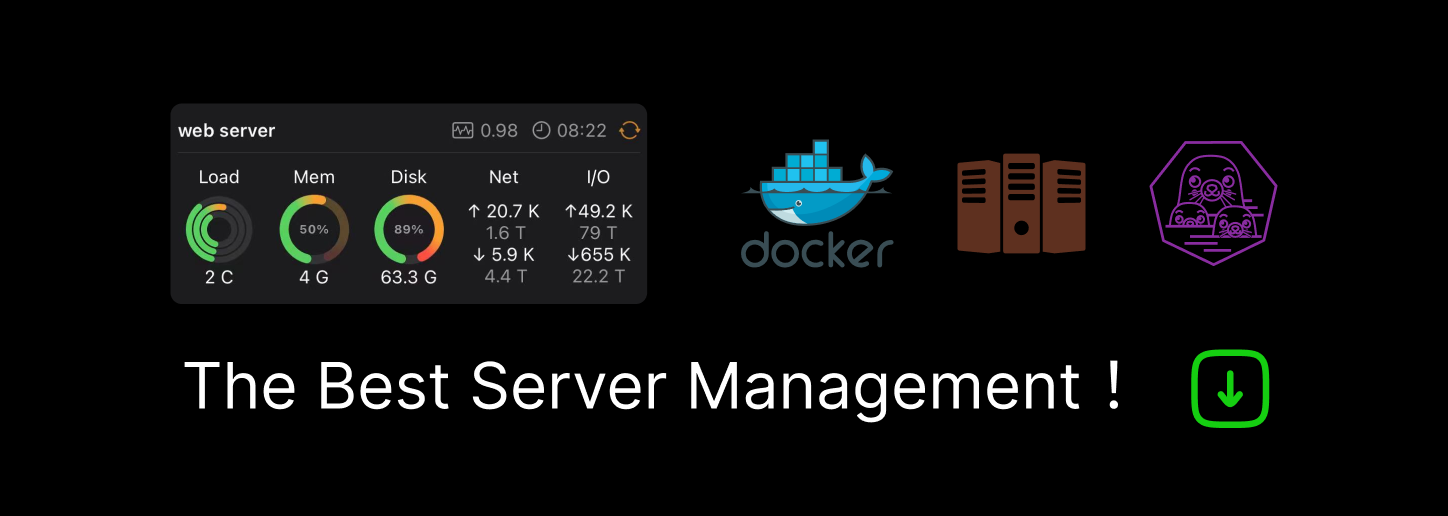
React Nesting Components: Rules to Follow
How to make a clean component tree in React with component nesting

React is a component-based framework. Therefore, every React app has a root component, built with one or more components that can be nested with other components to any level.
Nesting components aid in the development of complex User Interfaces by utilizing modular components. Therefore, knowing when and how to nest components correctly is essential in React. Your code will be messy and challenging to reuse if components are not properly nested.
This article will discuss best practices for nesting components in your React application. Let’s get started.
Nested components
A nested component is any child component linked to a parent component. This relationship between the child and parent components is formed through composition rather than inheritance. This is to say that rather than inheriting one component from another, each component is created by assembling smaller ones.
Even though component nesting helps you structure your code much cleaner, if you do not do component nesting correctly, you’ll end up with much more complex and less efficient code. Therefore, let’s look at different component nesting styles, when to use them, and the rules to follow.
Nesting without child components
When developing presentational components, this nesting style is ideal. You can separate UI elements effectively and quickly pass data as props to the nested components in this way. Take a look at the example code below.
In this example, the Card
component is nested within the App
component. You can put as many Card
components as you want inside the App
component. Furthermore, this design gives more power to the child component.
However, this type of nesting has lower component reusability and visibility of the component architecture. Therefore, it would be best if you also exercised caution to avoid creating deeply nested components.
Deeply nested components will result in data being passed through components that do not require that particular data. As a result, tracking the data flow as the application grows may become difficult. However, if you use a state management tool like Redux, you can avoid this. Therefore, if your project uses Redux, this nesting style is preferable.
Nesting with child components
When developing components like Sidebar
or Dialog
that represent generic "boxes," you may not know all of their children in advance. In such cases, passing the components as child elements is preferable.
The children
prop allows you to access these passed child elements in the nested component. Take a look at the code below to understand it better.
In this example, the MenuItem
components are nested as child elements of the Sidebar
component. This way of nesting allows for greater component reusability and visibility of the component architecture. It also aids in avoiding deeply nested components as your application grows in size. However, the child components will have less flexibility and power in this design, and the parent component's code may become lengthy.
If your project does not use Redux or the Context API to manage states, this style of component nesting is preferable to keep your component tree clean.
Nested components with a map
You can use the JavaScript map function to map data values to nested components. For example, you can modify the App()
component in the previous example to pass MenuItem
components as children of the Sidebar
component as shown below.
In this example, a key prop is used for MenuItem
. This is a rule to follow when using maps because React requires a unique key to distinguish each component. The key prop allows you to control the component instances. I've used the component index as the key here, but if the component order can change, it's better to use a unique ID from the data as the key.
You can pass a single prop to the Sidebar
component and map the values to a MenuItem
component to achieve the same results as passing multiple MenuItem
components. However, the nesting would be of the nesting without children variety.
Updating state in nested components
You can pass the parent component’s state as a prop to the nested components. In nested components, you can also update the parent’s state. This is easily accomplished in functional components by passing the set state function as a prop. In class components, you can write a function to setState
in the parent component and pass that function as a prop.
Below is a simple example of passing and updating the parent’s state in a nested component.
Remember that using the setState
function, you shouldn't directly change a parent's state in a child component
Also, always keep the state flow “top-down”, which means that only parents should pass their state to their children, not the other way around.
Conclusion
A clean component tree in React can help you read, understand, and debug code much faster. Hence, this article has discussed the various methods for nesting components in React and the rules to follow when using them.
I hope you now have a better understanding of component nesting in React. Thank you for reading, and happy coding!
Build apps with reusable components like Lego
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK