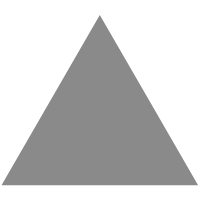
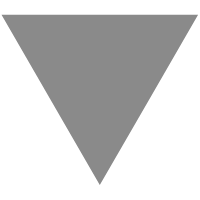
Laravel 5.6 - RealTime CRUD System Using Google Firebase
source link: https://www.laravelcode.com/post/laravel-56-realtime-crud-system-using-google-firebase
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
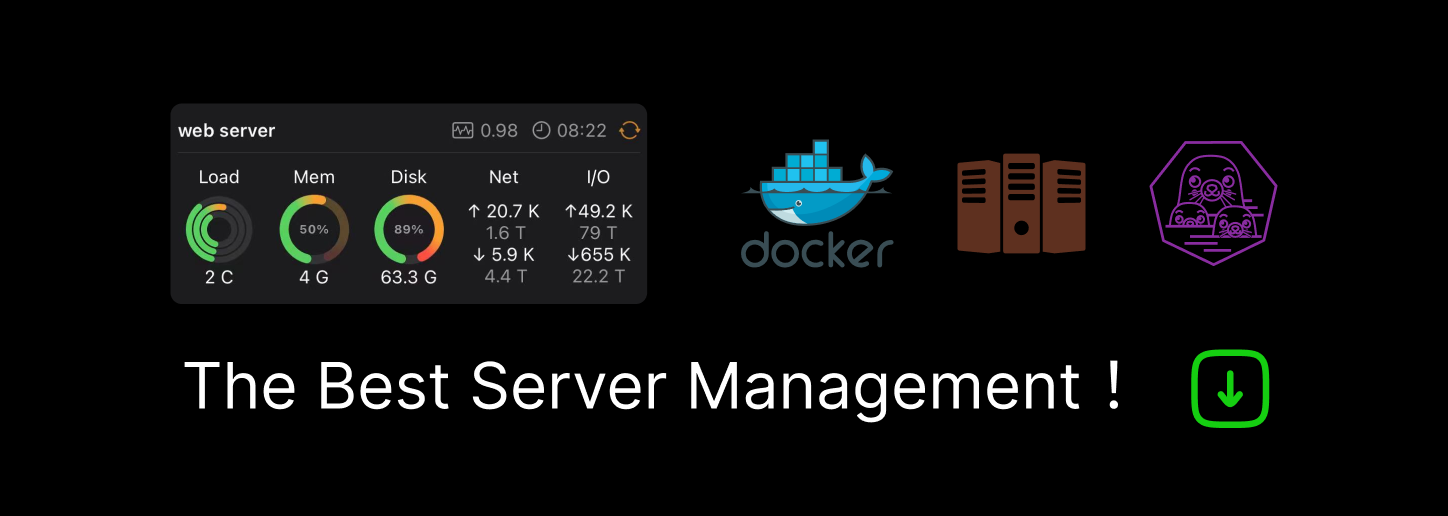
Laravel 5.6 - RealTime CRUD System Using Google Firebase
Today, we are share with you how to built real time CRUD system in laravel using google firebase. yes realtime insert, update, delete or listing is easily possible using google firebase database. in this article we are show to you step by step how to create google firebase database and how to integrate in your laravel application and how to built a realtime CRUD.
[ADDCODE]
You can do many realtime programming stuff by google firebase. after you follow all step of this article then you got final output look like following screenshot.
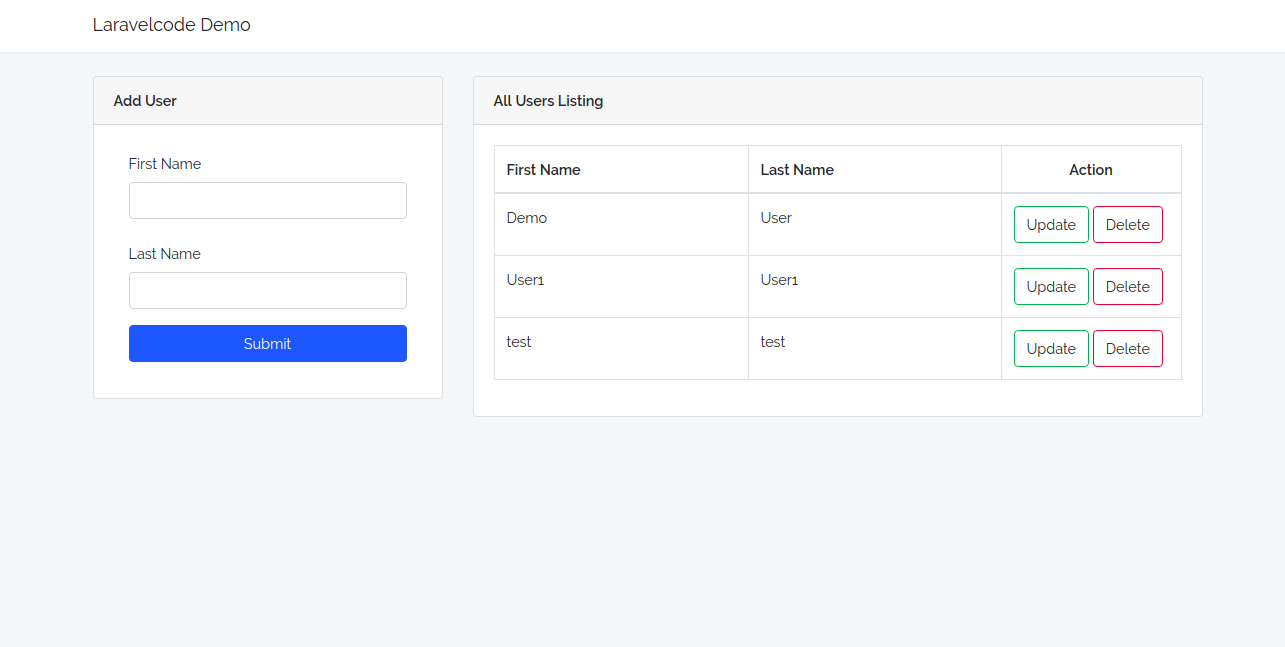
Create New Project In Firebase:
Now, we are start step by step how to built realtime CRUD system using google firebase. so, first you should create one google firebase project. if you don't know how to create google firebase project then visit our this link How to create google firebase project.
After create your google firebase project you must be get following things from your created project.
1.) api_key
2.) auth_domain
3.) database_url
4.) secret
5.) Legacy server key (Only use for notification)
This all things you got from your google firebase project easily
Configure Google Firebase Setting:
Now, we are configure google firebase setting in our laravel application. so, open config/services.php
file and set following configuration in this file.
'firebase' => [
'api_key' => 'api_key', // Only used for JS integration
'auth_domain' => 'auth_domain', // Only used for JS integration
'database_url' => 'https://database_url.com/',
'secret' => 'secret',
'storage_bucket' => '', // Only used for JS integration
],
Create Route:
Now, create following route in your routes/web.php
file. add following route in it.
Route::get('users', 'HomeController@users');
Here, we are create one get route for user display view.
Add users Method in HomeController:
Now, we are add one users function app/Http/Controllers/HomeController.php
file.
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class HomeController extends Controller
{
public function users()
{
return view('realtimecrud');
}
}
Create View File:
Now, we are create realtimecrud.blade.php
in resources/views folder and add following bootstap code in this file for your design view.
@extends('layouts.app')
@section('style')
<style type="text/css">
.desabled {
pointer-events: none;
}
</style>
@endsection
@section('content')
<div class="container">
<div class="row">
<div class="col-md-4">
<div class="card card-default">
<div class="card-header">
<div class="row">
<div class="col-md-10">
<strong>Add User</strong>
</div>
</div>
</div>
<div class="card-body">
<form id="addUser" class="" method="POST" action="">
<div class="form-group">
<label for="first_name" class="col-md-12 col-form-label">First Name</label>
<div class="col-md-12">
<input id="first_name" type="text" class="form-control" name="first_name" value="" required autofocus>
</div>
</div>
<div class="form-group">
<label for="last_name" class="col-md-12 col-form-label">Last Name</label>
<div class="col-md-12">
<input id="last_name" type="text" class="form-control" name="last_name" value="" required autofocus>
</div>
</div>
<div class="form-group">
<div class="col-md-12 col-md-offset-3">
<button type="button" class="btn btn-primary btn-block desabled" id="submitUser">
Submit
</button>
</div>
</div>
</form>
</div>
</div>
</div>
<div class="col-md-8">
<div class="card card-default">
<div class="card-header">
<div class="row">
<div class="col-md-10">
<strong>All Users Listing</strong>
</div>
</div>
</div>
<div class="card-body">
<table class="table table-bordered">
<tr>
<th>First Name</th>
<th>Last Name</th>
<th width="180" class="text-center">Action</th>
</tr>
<tbody id="tbody">
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
<!-- Delete Model -->
<form action="" method="POST" class="users-remove-record-model">
<div id="remove-modal" class="modal fade" tabindex="-1" role="dialog" aria-labelledby="custom-width-modalLabel" aria-hidden="true" style="display: none;">
<div class="modal-dialog" style="width:55%;">
<div class="modal-content">
<div class="modal-header">
<h4 class="modal-title" id="custom-width-modalLabel">Delete Record</h4>
<button type="button" class="close remove-data-from-delete-form" data-dismiss="modal" aria-hidden="true">×</button>
</div>
<div class="modal-body">
<h4>You Want You Sure Delete This Record?</h4>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default waves-effect remove-data-from-delete-form" data-dismiss="modal">Close</button>
<button type="button" class="btn btn-danger waves-effect waves-light deleteMatchRecord">Delete</button>
</div>
</div>
</div>
</div>
</form>
<!-- Update Model -->
<form action="" method="POST" class="users-update-record-model form-horizontal">
<div id="update-modal" class="modal fade" tabindex="-1" role="dialog" aria-labelledby="custom-width-modalLabel" aria-hidden="true" style="display: none;">
<div class="modal-dialog" style="width:55%;">
<div class="modal-content" style="overflow: hidden;">
<div class="modal-header">
<h4 class="modal-title" id="custom-width-modalLabel">Update Record</h4>
<button type="button" class="close update-data-from-delete-form" data-dismiss="modal" aria-hidden="true">×</button>
</div>
<div class="modal-body" id="updateBody">
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default waves-effect update-data-from-delete-form" data-dismiss="modal">Close</button>
<button type="button" class="btn btn-success waves-effect waves-light updateUserRecord">Update</button>
</div>
</div>
</div>
</div>
</form>
@endsection
After add this simple html code in your blade file but still it is not done. now we are add some google firebase
javascript code for built realtime CRUD. so, now add following js code in this file into the bottom
<script src="https://www.gstatic.com/firebasejs/4.9.1/firebase.js"></script>
<script>
// Initialize Firebase
var config = {
apiKey: "{{ config('services.firebase.api_key') }}",
authDomain: "{{ config('services.firebase.auth_domain') }}",
databaseURL: "{{ config('services.firebase.database_url') }}",
storageBucket: "{{ config('services.firebase.storage_bucket') }}",
};
firebase.initializeApp(config);
var database = firebase.database();
var lastIndex = 0;
// Get Data
firebase.database().ref('users/').on('value', function(snapshot) {
var value = snapshot.val();
var htmls = [];
$.each(value, function(index, value){
if(value) {
htmls.push('<tr>\
<td>'+ value.first_name +'</td>\
<td>'+ value.last_name +'</td>\
<td><a data-toggle="modal" data-target="#update-modal" class="btn btn-outline-success updateData" data-id="'+index+'">Update</a>\
<a data-toggle="modal" data-target="#remove-modal" class="btn btn-outline-danger removeData" data-id="'+index+'">Delete</a></td>\
</tr>');
}
lastIndex = index;
});
$('#tbody').html(htmls);
$("#submitUser").removeClass('desabled');
});
// Add Data
$('#submitUser').on('click', function(){
var values = $("#addUser").serializeArray();
var first_name = values[0].value;
var last_name = values[1].value;
var userID = lastIndex+1;
firebase.database().ref('users/' + userID).set({
first_name: first_name,
last_name: last_name,
});
// Reassign lastID value
lastIndex = userID;
$("#addUser input").val("");
});
// Update Data
var updateID = 0;
$('body').on('click', '.updateData', function() {
updateID = $(this).attr('data-id');
firebase.database().ref('users/' + updateID).on('value', function(snapshot) {
var values = snapshot.val();
var updateData = '<div class="form-group">\
<label for="first_name" class="col-md-12 col-form-label">First Name</label>\
<div class="col-md-12">\
<input id="first_name" type="text" class="form-control" name="first_name" value="'+values.first_name+'" required autofocus>\
</div>\
</div>\
<div class="form-group">\
<label for="last_name" class="col-md-12 col-form-label">Last Name</label>\
<div class="col-md-12">\
<input id="last_name" type="text" class="form-control" name="last_name" value="'+values.last_name+'" required autofocus>\
</div>\
</div>';
$('#updateBody').html(updateData);
});
});
$('.updateUserRecord').on('click', function() {
var values = $(".users-update-record-model").serializeArray();
var postData = {
first_name : values[0].value,
last_name : values[1].value,
};
var updates = {};
updates['/users/' + updateID] = postData;
firebase.database().ref().update(updates);
$("#update-modal").modal('hide');
});
// Remove Data
$("body").on('click', '.removeData', function() {
var id = $(this).attr('data-id');
$('body').find('.users-remove-record-model').append('<input name="id" type="hidden" value="'+ id +'">');
});
$('.deleteMatchRecord').on('click', function(){
var values = $(".users-remove-record-model").serializeArray();
var id = values[0].value;
firebase.database().ref('users/' + id).remove();
$('body').find('.users-remove-record-model').find( "input" ).remove();
$("#remove-modal").modal('hide');
});
$('.remove-data-from-delete-form').click(function() {
$('body').find('.users-remove-record-model').find( "input" ).remove();
});
</script>
After add this javascript
code in your blade file then your realtime crud system done successfully.
Now we are ready to run our example so run bellow command ro quick run:
php artisan serve
Now you can open bellow URL on your browser:
http://localhost:8000/users
Please also check our demo for realtime CRUD system.
We are hope you like this tutorials, if any question regarding any query please post your question in our forums click on bellow link Laravelcode's Forums
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK