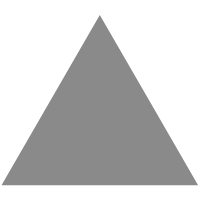
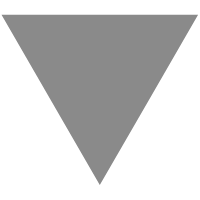
Laravel 8 - How to send Bulk Mail in Background using Queue
source link: https://www.laravelcode.com/post/laravel-8-how-to-send-bulk-mail-in-background-using-queue
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
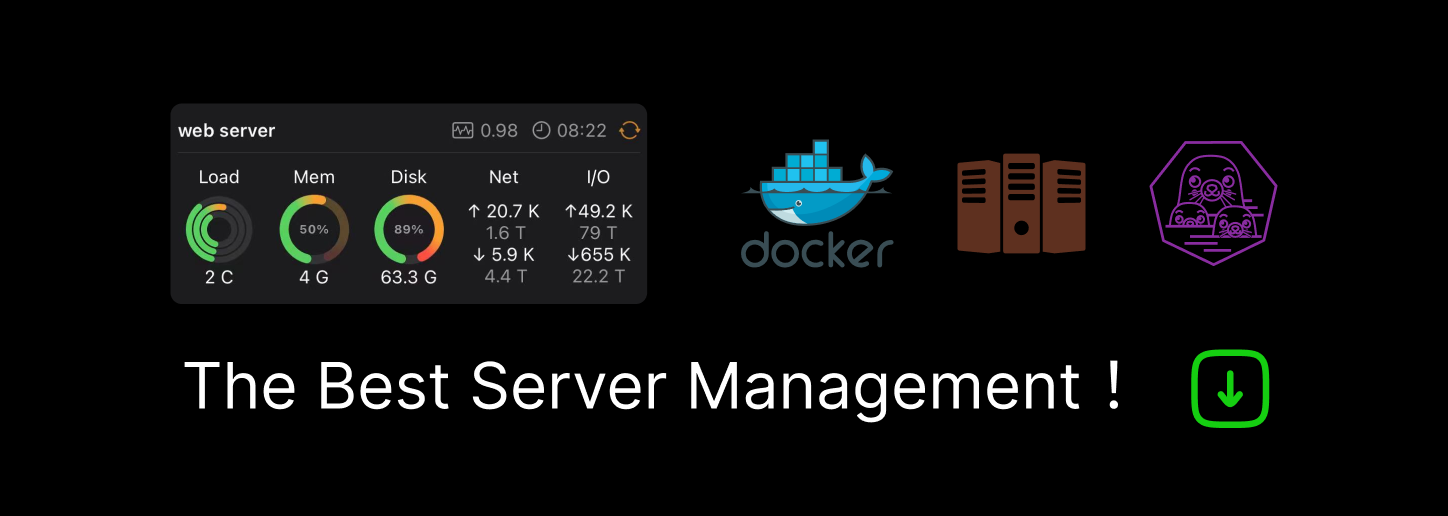
Laravel 8 - How to send Bulk Mail in Background using Queue
In this article, i will share with you how to send bulk mail in the background using laravel queue with example. as you know only one mail send does not take time and also your laravel application working smoothly. but if you send bulk mail as alike simple mail sending functionality then it takes lots of time and during this time you can not do anythings operation in your laravel application.
So, it's simple solution is to write your bulk mail login in a queue. so, when you click on send mail then at a time you got a success message "your all bulk mail send in the background".
If you never use a queue in laravel application then don't worry in this article I will show you all the steps by steps so, you also make your other functionality on the queue base and make your laravel application faster and smooth.
Step - 1 : Change .env
If, you want to send a bulk mail-in "queue
" then make the following two changes in your laravel's ".env
" file.
QUEUE_DRIVER=database
QUEUE_CONNECTION=database
Step - 2 : Create Route
First, we create one route for sending the bulk mail by clicking the button.
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\SendBulkMailController;
Route::get('send-bulk-mail', [SendBulkMailController::class, 'sendBulkMail'])->name('send-bulk-mail');
Step - 3 : Create a Queue Table
Now, we create a "jobs
" table in our database in our laravel application using the following command in terminal.
# create database migration script
php artisan queue:table
# run our new migration
php artisan migrate
Step - 4 : Create Controller
Now, we create our "SendBulkMailController.php
" file in "app/Http/Controllers
" folder using the following artisan command.
php artisan make:controller SendBulkMailController
then after open the "SendBulkMailController.php
" file and write the following code into it.
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class SendBulkMailController extends Controller
{
public function sendBulkMail(Request $request)
{
$details = [
'subject' => 'Weekly Notification'
];
// send all mail in the queue.
$job = (new \App\Jobs\SendBulkQueueEmail($details))
->delay(
now()
->addSeconds(2)
);
dispatch($job);
echo "Bulk mail send successfully in the background...";
}
}
Step - 5 : Create Jobs Class
Now, we need to create the "SendBulkQueueEmail.php
" file in the "app\Jobs
" folder using the running the following command in your terminal.
php artisan make:job SendBulkQueueEmail
and open the "app\Jobs\SendBulkQueueemail.php
" file and write the following code into it.
namespace App\Jobs;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
use App\Models\User;
use Mail;
class SendBulkQueueEmail implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
protected $details;
public $timeout = 7200; // 2 hours
/**
* Create a new job instance.
*
* @return void
*/
public function __construct($details)
{
$this->details = $details;
}
/**
* Execute the job.
*
* @return void
*/
public function handle()
{
$data = User::all();
$input['subject'] = $this->details['subject'];
foreach ($data as $key => $value) {
$input['email'] = $value->email;
$input['name'] = $value->name;
\Mail::send('emails.test', [], function($message) use($input){
$message->to($input['email'], $input['name'])
->subject($input['subject']);
});
}
}
}
Step - 6 : Create Mail Blade
Now, we need to create on simple mail blade file in "resources/views/emails/test.blade.php
" and write there your mail content.
<h2>This is my test bulk mail.</h2>
Step - 7 : Test Bulk Mail Functionality
Now, in the final step we test our bulk mail functionality working properly or not? so, first, start your laravel application using the following artisan command in your terminal.
php artisan serve
now, open the browser and run the following url in it.
http://localhost:/send-bulk-mail
Now, check-in your database's "jobs
" table. in this table, you see like that some entry.
if you want send manually then run the following artisan command in your terminal.
php artisan queue:listen --timeout=0
i hope your like this article.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK