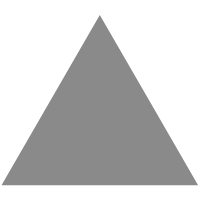
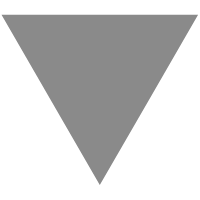
关于 JavaScript 中的 Promise 你应该知道的五件事
source link: https://www.51cto.com/article/720444.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
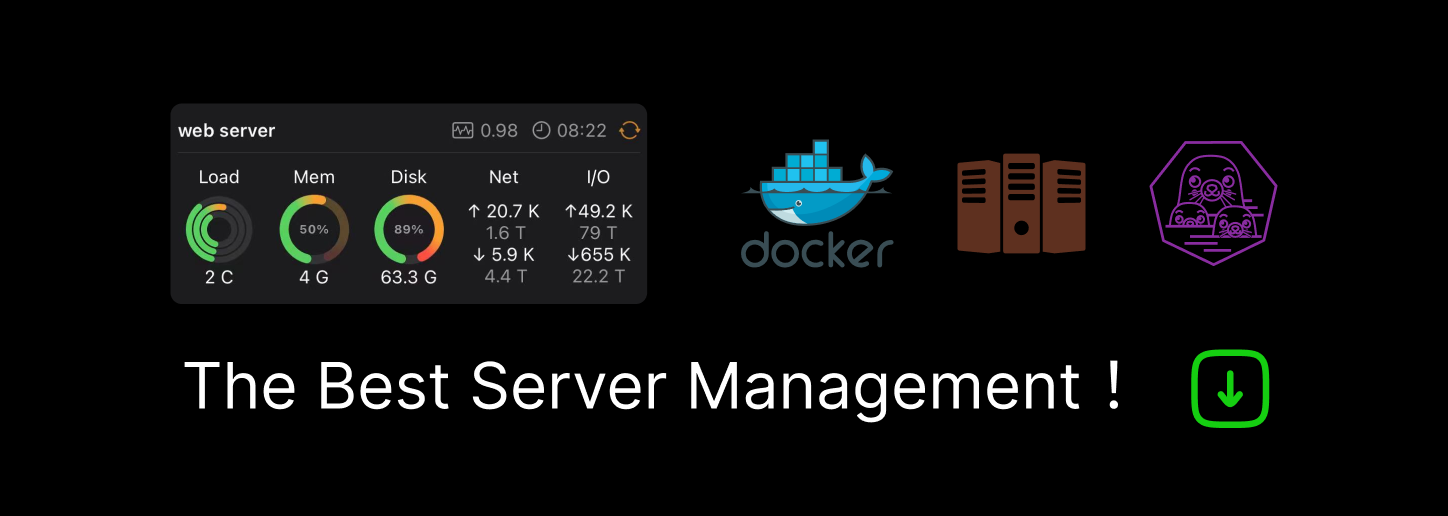
关于 JavaScript 中的 Promise 你应该知道的五件事
Promise 模式是现代 JavaScript 编程的必备条件。 使用 then/catch 链接看起来很简单,但它有一些我们最好知道的细节。 这篇文章将带来关于 Promise 的 5 件事。
Promise 模式是现代 JavaScript 编程的必备条件。 使用 then/catch 链接看起来很简单,但它有一些我们最好知道的细节。 这篇文章将带来关于 Promise 的 5 件事。
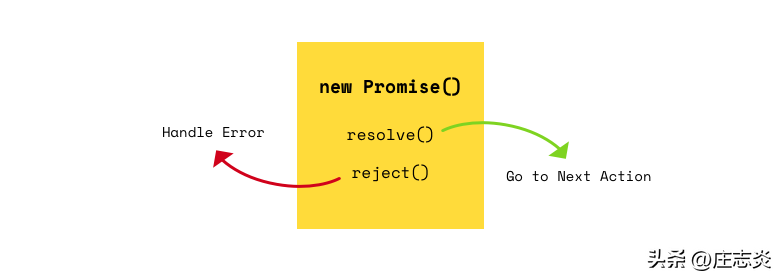
1. 反复解决
如果下面的代码运行会发生什么?
new Promise(resolve => {
resolve(new Promise(resolve => {
resolve(new Promise(resolve => {
resolve(10)
}))
}))
})
.then(value => console.log(value)) // 10
then() 给你 10(不是 Promise 实例)。 如果解析的值是一个 Promise,那么它会解析直到它不能 then-able。
2.返回链
then() 链不仅是函数链,还是价值链。
Promise.resolve(10)
.then(value => value + 1)
.then(value => value + 1)
.then(value => console.log(value)) // 12
第一个 then() 为该值提供 10,第二个为 11,第三个为 12。它还对返回的值应用循环链,因此以下代码的工作方式相同。
Promise.resolve(10)
.then(value => value + 1)
.then(value => Promise.resolve(value + 1))
.then(value => console.log(value)) // 12
3.then()的第二个参数
then() 实际上有 2 个参数。 第二个参数采用一个函数来处理拒绝的情况。 它与 catch() 的工作方式非常相似。 以下代码的结果是相同的。
/// then() with second parameter
Promise.reject(10)
.then(
value => value + 1,
reason => handleError(reason), // reason=10
)/// then() and catch()
Promise.reject(10)
.then(value => value + 1)
.catch(reason => handleError(reason)) // reason=10
如果我们两个都像下面这样呢?
Promise.reject(10)
.then(
value => value + 1,
reason => handleError1(reason), // called
)
.catch(reason => handleError2(reason)) // not called
如果两者都给出,则 then() 的第二个参数在 catch() 之前,因此调用了 handleError1() 而不会调用 handleError2()。 唯一的例外是 Promise 实际抛出错误的情况。 请参阅下面的代码。
new Promise(() => { throw 'error' })
.then(
value => value + 1,
reason => handleError1(reason), // not called
)
.catch(reason => handleError2(reason)) // called
它仅在 catch() 子句中捕获错误,因此调用了 handleError2()。 一个有趣的部分是,如果它没有 catch(),即使使用 onReject 参数也会抛出错误。
4. then() 中抛出错误
如果 then() 中发生错误,它会在 catch() 子句中捕获。
Promise.resolve(10)
.then(value => { throw 'error' })
.catch(reason => handleError(reason)) // catch!
5.错误作为一个值
如果我们尝试用错误来解决,这听起来很有趣。 你能想象下面的代码会发生什么吗?
Promise.resolve(new Error('error'))
.then(value => console.log(value)) // Error value
.catch(reason => handleError(reason))
它不会捕获但会正常解析,因此它会记录原始错误。 Promise 不赋予 Error 权限,而是 Promise 本身。
..和一个建议
Promise 模式对于处理异步作业很有用,但它通常涉及带有嵌套 Promise 的曲线代码。 它不利于阅读,因此另一种选择是使用 async/await。 现代 JavaScript 打包器提供了 async/await 语法的转换。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK