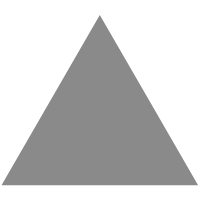
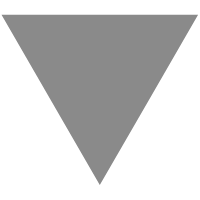
AWS Cognito Overview and Step-By-Step Integration
source link: https://dzone.com/articles/aws-cognito-overview-and-step-by-step-integration
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
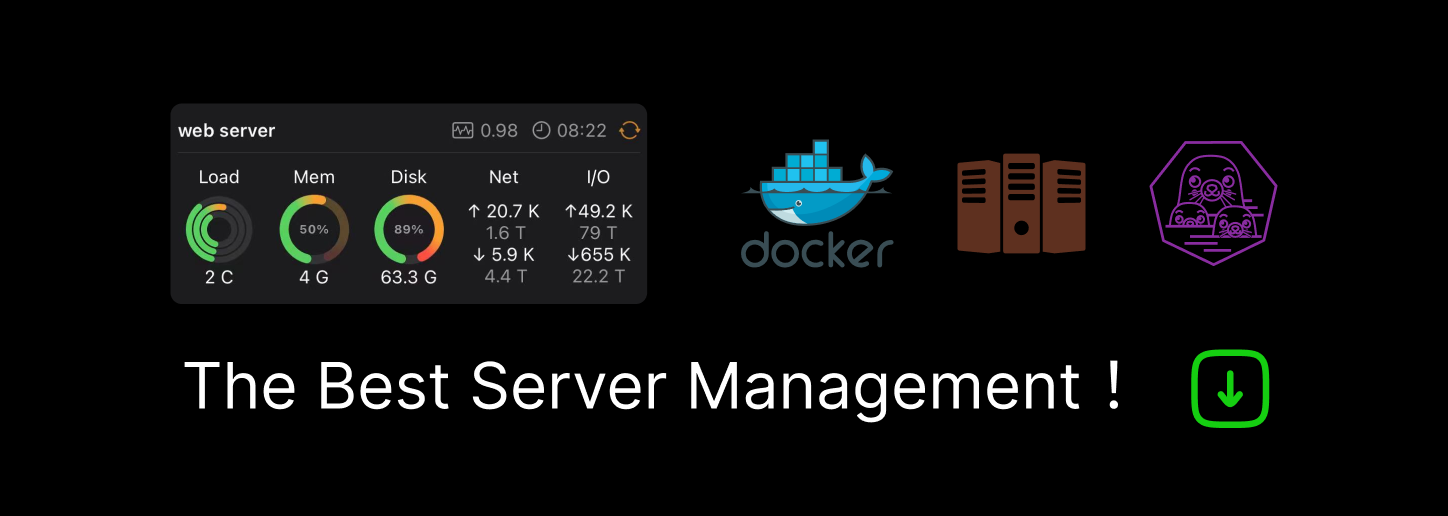
AWS Cognito Overview and Step-By-Step Integration
Explore the difference between two well-known Auth building methods: AWS Cognito and JSON Web Token. Plus, take a look at the AWS Cognito application process.
When an application needs server-side implementation, clients (such as mobile devices or browsers) must authenticate to the server. For instance, when someone uses Gmail to log into Facebook after having logged in before, they transmit certain identifying information to the Facebook server.
User authentication for your application might be established in several different ways. However, the most suitable option, in our opinion, is to use AWS Cognito.
Why? AWS Cognito is a convenient and ready-to-use user authentication service that allows using its functionality for certain subscription fees. Moreover, you don’t need to bother with backend technology, as AWS Cognito has already done. This option is suitable for you if you want to use advanced security features like two-step verification, SMS verification, or login using social media.
AWS Cognito Overview
AWS Cognito is one of the services offered by Amazon Web Services that can store user data like first and last names, emails, passwords, and any other personal details they might enter into an app or web form. Ways to sign in include: a username and password, Amazon, Facebook, Apple, or Google, and enterprise identity providers (SAML, OpenID Connect).
AWS Cognito might be used to provide your app’s end-to-end security, including identity verification, access control, and account recovery. It allows to quickly and easily create an application that authenticates the user and integrates with external services.
Pros of AWS Cognito
- The AWS SDK is secure for storing data and has all the functionality you’ll need for OAuth integration. So, instead of writing your own code to manage user sessions and authentication tokens, you can rely on the service to handle user maintenance operations.
- In order to set up your API to validate against a Cognito pool, just use Amazon Cognito with API Gateway.
- You may customize users’ characteristics like an address, phone number, city, etc.
- The data delivered by the app is encrypted and protected by The AWS Amplify module. So, no need to worry about security.
Cons of AWS Cognito
- To complete complex software development tasks, AWS Cognito has to be added to programmers’ toolchains and used during development. It adds a layer of complication.
- The documentation is rarely updated, and not many details to be honest.
- Custom field characteristics are limited to 25.
- Cognito is compatible with other AWS services — SES for email, SNS for sending text messages, and API Gateway for authentication. However, it is a hassle to add external services and costs more.
Amazon Cognito User Pоols
User pоols are directories needed for authentication (identity verification). With their help, users can sign in to a web or mobile app.
User poоls provide:
- Sign-up and sign in.
- Ready-to-use, custom web UI for sign-in.
- Sіgn-in with Facebook, Google, Apple, Amazon, and through SAML and OIDC identity providers from your user pool.
- User profiles and User dirеctory management.
- Among security features are account fraud protection, multifactor user authentication, email and phone vеrification, and checks for compromisеd credеntials.
- User migration through AWS Lambda triggers.
Getting Started
The first step is to create and configure the User Pool.
Go to Cоgnito in the AWS console and press 'Manage User Pools.' Then create a user pool. Provide the name of the user pool and click ‘Step through settings’ to configure needed parameters.
Sign-in Parameters
The next step is to config parameters. AWS Cоgnito is very flexible in terms of config depending on business needs. In this guide, we will discuss email plus password sign-in.
Password Setup
Let’s configure the password next. To improve security, create a strong password (one number, one upper case letter, one lower case letter, and special characters) with a minimum length of 8 symbols. In addition, when the user is created by the admin, temporarily send it to the user email, and the expiration time of the password can be adjusted from 1 to 90 days.
Let’s now set up email for user authentication and verification.
Configuring Emails for User Pools
In this section, we can adjust how the email message will be sent when the user wants to recover the password or confirm the account. All parameters can be set up step by step or skipped and changed later. For example, SES isn’t used by default, but we can always change it. SES also needs configuration, but that is another process that we will skip this time. The basic option for email senders is a 50-email limit.
On the same page, we can configure and personalize an email message using HTML code. Also, complex customization can be done using Lambda triggers.
Client Creation
- Click the button ‘Add an app client.’
- Enter the app name.
- Choose token lifetime. Token validity depends on business specifications and security requirements. Select the default time for token expiration as in the screenshot below if you are not sure about the needed time.
Lat here is to press “Create app client” and make sure to generate and memorize a secret client ID for later.
The last step to take is Lambda triggers than will:
- Process and save user data on the backend after registration or authentication
- Customize emails
- Create custom auth flow
- Migration of users from your existing user management system into your Cognito user pool
- Config token creation
The second one is App client ID. Go to App client settings under the App integration section. Later, we might add authentification using Google, Facebook, etc. — we can specify ‘Sign in’ and callback URLs on this page.
Now that we created our Cognito service, we can use Amazon Web Services SDK or other libraries to interact with our code.
Let’s write some code. We will create a class with two public methods: signUpUser and handleUserAuth.
Create a .env file and save AWS credentials there.
AWS_ACCESS_KEY_ID=
AWS_SECRET_ACCESS_KEY=in
AWS_REGION=
COGNITO_POOL_ID=
COGNITO_APP_CLIENT_ID=
Also, we need to install a library to interact with Cognito from our code using the command:
npm i amazon-cognito-identity-js
Then create the user poll property and initialize it in the constructor.
export class CognitoService {
private readonly userPool: CognitoUserPool;
constructor() {
this.userPool = new CognitoUserPool({
ClientId: process.env.CLIENT_ID,
UserPoolId: process.env.USER_POOL_ID,
});
}
}
The next step is to create a method to signup the user using the email and password that the user inserted.
async signUpUser(user: RegistrationBodyDto): Promise<ISignUpResult> {
const { email, password } = user;
const signUpResponse = await this.signUp(email, password);
const username = signUpResponse.user.getUsername();
await this.addUserToUserGroup(username, UserRoles.Customer);
return signUpResponse;
}
private async signUp(email: string, password: string): Promise<ISignUpResult> {
const userAttribute = new CognitoUserAttribute({ Name: ‘email’, Value: email });
return await new Promise((resolve, reject) => {
this.userPool.signUp(email, password, [userAttribute], null, (err, result) =>
err ? reject(err) : resolve(result),
);
});
}
Now let’s create a method for user authentication, access generation, refreshing, and ID tokens.
async handleUserAuth(id: string, password: string): Promise<LoginResponse> {
const details = new AuthenticationDetails({
Username: id,
Password: password,
});
const cognitoUser = this.cognitoUserFactory(id);
const session = await this.authenticateUser(cognitoUser, details).catch((err) => {
throw new UnprocessableEntityException(err.message);
});
return this.getTokensFromSession(session);
}
async authenticateUser(user: CognitoUser, details: AuthenticationDetails): Promise<CognitoUserSession> {
return await new Promise((resolve, reject) =>
user.authenticateUser(details, {
onSuccess: resolve,
onFailure: (err) => reject(err),
}),
);
}
All set! We have a class that allows using methods to create and authenticate users in the web app.
Summing Up
Despite several disadvantages that AWS Cognito has, it’s a good choice if you’re creating a new prototype application. We set up the authentication processes for API integration with AWS Cognito. AWS Cognito is worth considering for authenticated API integration due to the lack of maintenance and easy implementation.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK