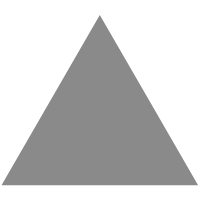
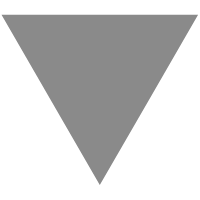
Functional Programming and Naturality in Javascript: Conjunctions
source link: https://medium.com/@mehmetegemenalbayrak/functional-programming-and-naturality-in-javascript-conjunctions-78039af7c092
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
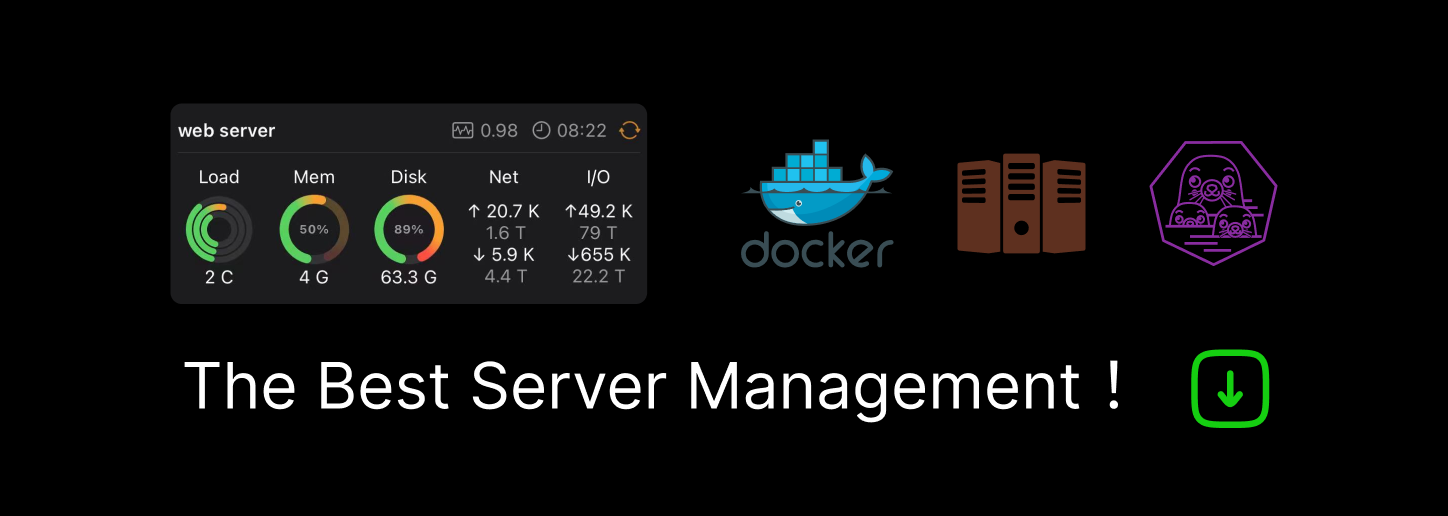
Functional Programming and Naturality in Javascript: Conjunctions
While pressing keys for this sentence, I start my journey to discover naturality in Javascript. Excessive naturality usually hurts the ability to extend the code, but we will see, maybe we can find a workaround! So here my discovery starts with you!
First Attempt: Adding prepositions and conjunctions in function composing
Let’s say we have an array called “buckets” and a function to get tokens from that bucket called “tokens”. I often think about a structure like:
compose(tokens, from, buckets)
Here the word “from” is a preposition and denotes a relationship between the right-hand parameter and left-hand parameter in its position. Usually compose(“flow” in lodash) function is used like this:
compose(tokens, buckets)
Here as developers who know functional programming, we understand that “buckets” values are going into “tokens” function as a parameter.
There is a but.
Most of us do something when we read code, or usually trying to understand any kind of text that is written by someone else. We turn the code into a form that we can understand, like storing an explanation. Some of us use “Subvocalization” and “Elaboration” when trying to get a sense of code, or sometimes even “Visualization”. We mostly do this in our head because we are lazy creatures, but some of us do it on pen and paper like Dijkstra does. Personally, if it’s not a hard problem I don’t use pen and paper.
Anyways.
With naturality we are trying to decrease the cognitive load while storing code in the brain by providing elaboration beforehand. Also the “from” preposition there explains how the “compose” function works, so it creates a self-documenting code. So we improved readability, cognitive load and discoverability by these changes.
An example implementation is:
const {
flow: Do,
map: mapEach,
isFunction,
} = require(“lodash”);
const andDo = Do;
const by = (anyVal) => (fn) => fn(anyVal);
const value = (x) => x;
const numbersToBeMultiplied = [1, 2, 3, 4];
const multiply = (operand) => (constant) => (isFunction(constant) ? constant() : constant) * operand;
const multipliedNumbers = mapEach(numbersToBeMultiplied, andDo([multiply, by(4)]));
console.log(JSON.stringify(multipliedNumbers, null, 2));
As you can see we added “by” conjunction in the function composition directive and achieved a modular approach to multiply numbers instead of creating a variable like “multiplyBy4()” and increased readability and naturality a lot. Because of the nature of “by” function, the code is also open to extension.
Disclaimer from now on: Everything is a tradeoff in Software Engineering and some software qualities are usually violating some other, so you can comment on medium or where I shared this article to point out other pros and cons of the approach. Don’t forget the pros! :-)
Second attempt: Adding prepositions and conjunctions in function parameters
I’ve always dreamed about a function call like this:
const fifteen = add(5, and, 10);
And, Or, Not, etc. All propositions, conjunctions, and logical operators fit well in the parameters sometimes. This natural way of writing statements is actually possible and quite easy! Checkout this example:
const and = Symbol(“NaturalityOperandAnd”);
const trimmedParams = (args) => args.filter(arg => arg !== and);
const Do = (fn) => (…args) => fn(…trimmedParams(args));
const add = Do((x, y) => x + y)
console.log(add(5, and, 10));
In the browser you can attach this logic to every function by iterating through the window object, and then without wrapping any function you can have your “and”! It’s a bit trickier on the NodeJS side, couldn’t find a clean solution yet so didn’t implement it in the example.
Remarks: I think this increases the tokens by total number of tokens and by the Theoretical Cost Analysis laws it increases the initial cost a bit(because you write more), but the readability granted especially to juniors in various other applications is much because with the words “and”, “or” etc., you imply the relationship between the parameters without diving into the code so it improves discoverability.
Also you help with elaboration and subvocalization so understanding the code is easier.
Conclusion
These are my 2 attempts to provoke your thought on naturality and its implications on maintainability. You can comment, share, follow me, drink a margarita, I don’t know, do something! Joke aside, I aimed at triggering a discussion to improve the art of programming, and hopefully we can do it together.
Take care!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK