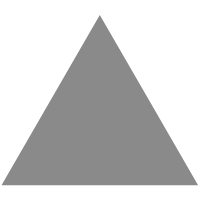
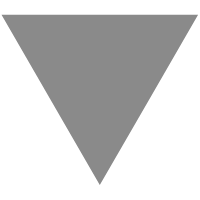
React报错之`value` prop on `input` should not be null - chuckQu
source link: https://www.cnblogs.com/chuckQu/p/16558106.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
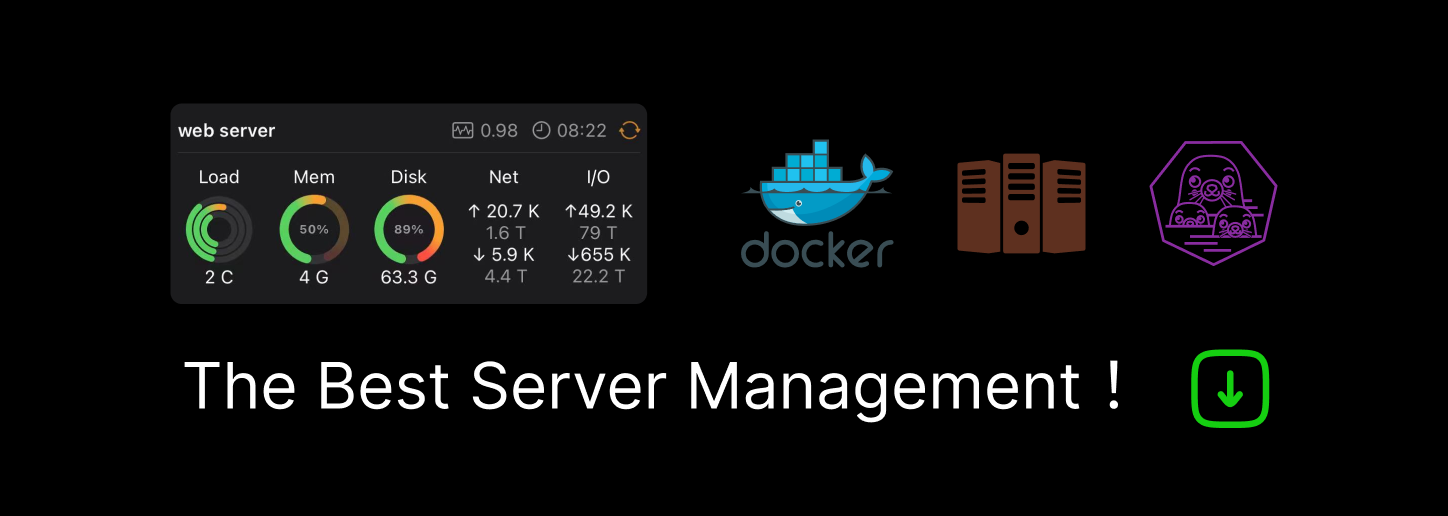
React报错之`value` prop on `input` should not be null
正文从这开始~
当我们把一个input
的初始值设置为null
或者覆盖初始值设置为null
时,会产生"value
prop on input
should not be null"警告。比如说,初始值来自于空的API响应。可以使用一个回退值来解决这个问题。
这里有个例子来展示错误是如何发生的。
export default function App() {
// ⛔️ Warning: `value` prop on `input` should not be null.
// Consider using an empty string to clear the component or `undefined` for uncontrolled components.
return (
<div>
<input value={null} />
</div>
);
}
上述代码的问题在于,我们为input
表单的value
属性设置为null
,这是不被允许的。
你也可能从远程API获取你的
input
表单的值,并将其设置为null
。
为了解决该问题,我们可以通过提供回退值,来确保永远不会为input
表单的value
属性设置null
。
import {useState} from 'react';
const App = () => {
// 👇️ pass empty string as initial value
const [message, setMessage] = useState('');
const handleChange = event => {
setMessage(event.target.value);
};
// ✅ use fallback, e.g.
// value={message || ''}
return (
<div>
<input
type="text"
id="message"
name="message"
onChange={handleChange}
value={message || ''}
/>
</div>
);
};
export default App;
我们把
state
变量的值初始化为一个空字符串,而不是null
。
这样就可以摆脱警告,除非在你代码的其他地方将state
变量设置为null
。
我们使用逻辑与(||)操作符,如果操作符左侧的为假值(比如说null
),则返回其右侧的值。这可以帮助我们确保input
表单的value
属性永远不会被设置为null
。
defaultValue
如果你借助refs
使用不受控制的input
表单,请不要在input
元素上设置value
属性,使用defaultValue
来代替value
属性。
import {useRef} from 'react';
const App = () => {
const inputRef = useRef(null);
function handleClick() {
console.log(inputRef.current.value);
}
return (
<div>
<input
ref={inputRef}
type="text"
id="message"
name="message"
defaultValue="Initial value"
/>
<button onClick={handleClick}>Log message</button>
</div>
);
};
export default App;
上述示例使用了不受控制的input
。注意input
表单上并没有设置onChange
或者value
属性。
你可以使用
defaultValue
属性来为不受控制的input
传递初始值。然而,这一步骤不是必要的,如果你不想设置初始值,你可以省略该属性。
当使用不受控制的input
表单时,我们使用ref
来访问input
元素。每当用户点击例子中的按钮时,不受控制的input
的值都会被记录下来。
你不应该为不受控制的input
设置value
属性,因为这将使input
表单不可变,你将无法在其中输入。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK