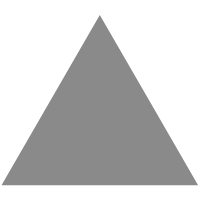
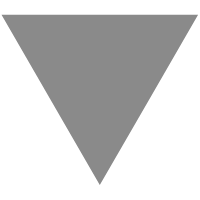
Node.js MySQL Delete data Database Table
source link: https://www.laravelcode.com/post/node-js-mysql-delete-data-database-table
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
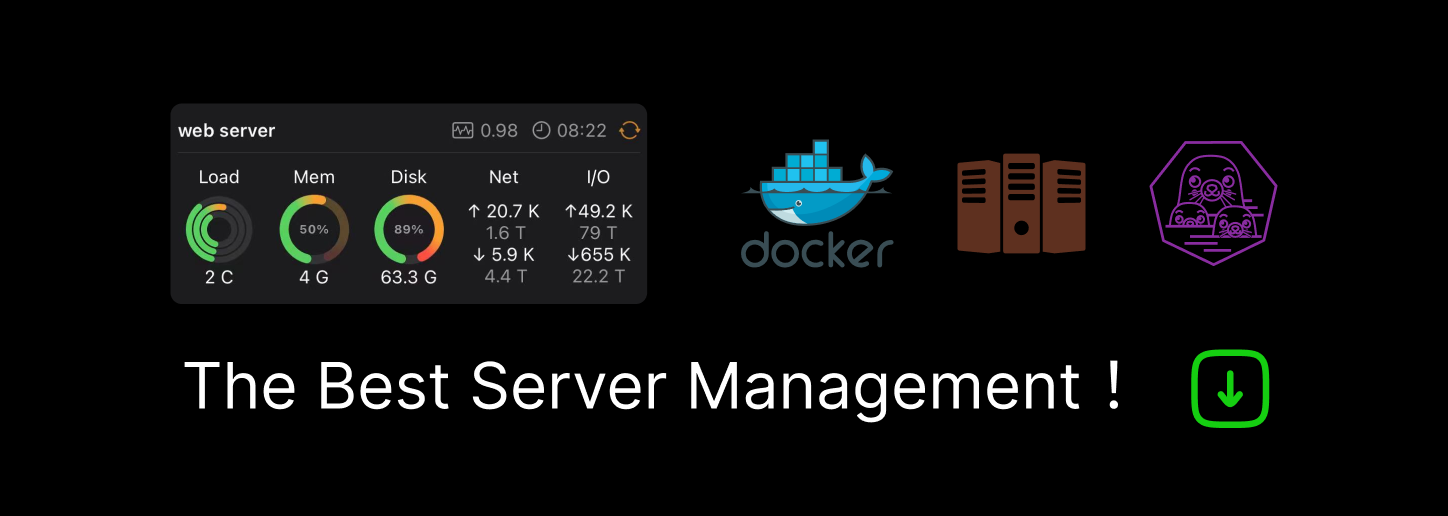
Node.js MySQL Delete data Database Table
In this tutorial article, we will see how to delete data from MySQL database using Node.js. We will use where condition to delete specific records from MySQL server.
Perform the following steps to delete data from MySQL database server.
- Step 1: First create connection between MySQL server and Node.js.
- Step 2: After database connection created, use conn.query() method to run DELETE query into MySQL database.
var query = `DELETE FROM visitors WHERE percentage < ?`;
var percentage = 50;
- After the query executed, close the connection using conn.end() method.
We have created below delete_rows.js
file with full example.
const mysql = require('mysql');
const conn = mysql.createConnection({
host: 'localhost',
port: 3306,
database: 'charts',
user: 'root',
password: 'root',
});
conn.connect((err) => {
if (err) {
return console.log(err.message);
} else {
// select query
var query = `DELETE FROM visitors WHERE percentage < ?`;
var percentage = 50;
// query to database
conn.query(query, percentage, function(err, response, result) {
if (err) {
return console.log(err.message);
} else {
console.log(response);
}
});
}
// close the connection
conn.end();
});
Now run the file into Node server.
node delete_rows.js
The application will response with object data. In the database all records will be deleted with percentage less than 50.
OkPacket {
fieldCount: 0,
affectedRows: 6,
insertId: 0,
serverStatus: 34,
warningCount: 0,
message: '',
protocol41: true,
changedRows: 0
}
You can get number of deleted records using response.affectedRows
object property.
Note: If you omit the WHERE condition, all records will be deleted.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK