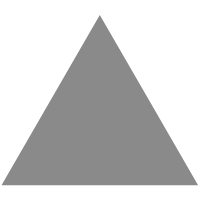
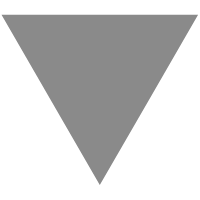
Laravel 8 - Create Contact in ZOHO Crm Tutorial Example
source link: https://www.laravelcode.com/post/laravel-8-create-contact-in-zoho-crm-tutorial-example
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
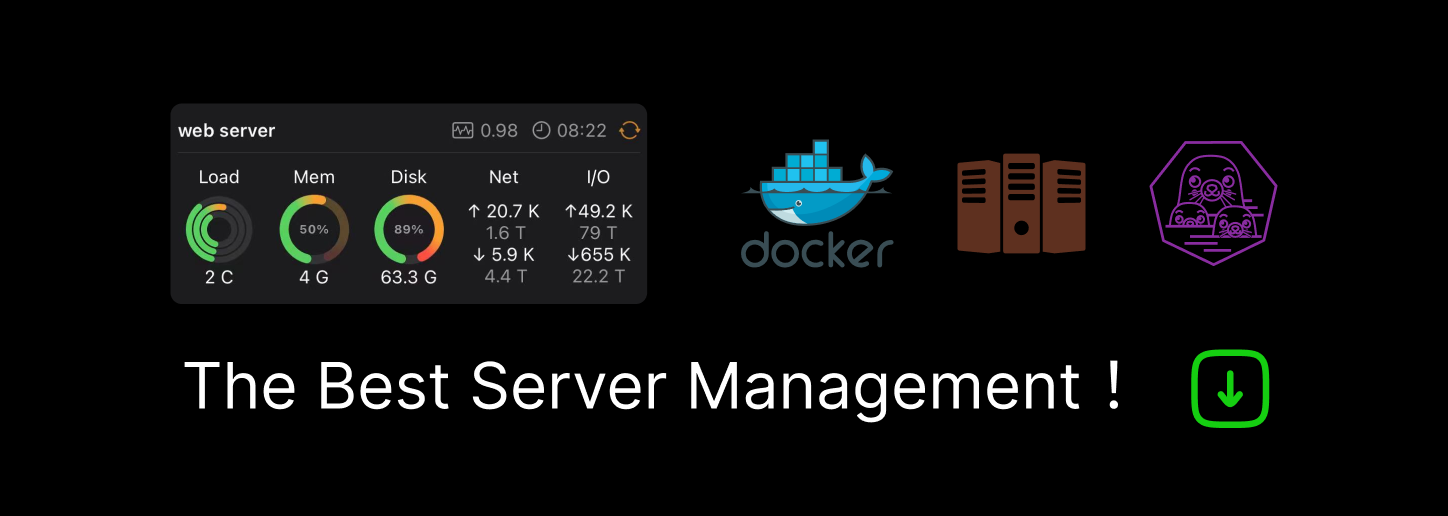
Laravel 8 - Create Contact in ZOHO Crm Tutorial Example
In this article, I will share with you how to integrate Zoho API in laravel 8 application and how to create contact in Zoho CRM using laravel. Zoho is the best application for creating lead, contact and you can manage your sale there.
So, recently i was working in one laravel sale-related application and they want to create a contact in Zoho platform from the laravel application. so, here I am sharing with you how to implement create a contact in Zoho CRM from the laravel 8.
Simply, follow the step. i make it this article very simple.
Step - 1 : Create Routes
Here, we create two routes for add contact in Zoho CRM from the laravel 8 application.
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\ContactController;
use App\Http\Controllers\ZohoController;
// Listing contact route
Route::get('contacts', [ContactController::class, 'index'])->name('contacts');
// Add contact in ZOHO
Route::get('zohocrmauth', [ZohoController::class, 'auth'])->name('zohocrmauth');
Route::get('zohocrm', [ZohoController::class, 'store'])->name('zohocrm');
Step - 2 : Add Controller and Models
In, this step we need to created "ContactController", "ZohoController" and "Contact" Model using the following artisan command.
php artisan make:controller ContactController --resource --model=Contact
php artisan make:controller ZohoController
After the running above command your model and controller created in your application now just write the following code in it.
app/Http/Controllers/ContactController.php
namespace App\Http\Controllers;
use App\Models\Contact;
use Illuminate\Http\Request;
class ContactController extends Controller
{
public function index()
{
$data = Contact::get();
return view('articles.index', compact('data'));
}
}
app/Http/Controllers/ZohoController.php
namespace App\Http\Controllers;
use App\Models\Contact;
use Illuminate\Http\Request;
class ZohoController extends Controller
{
public function auth(Request $request)
{
$uri = route('zohocrm');
$scope = 'ZohoInvoice.contacts.Create';
$clientid = 'here your zoho clientid';
$accestype = 'offline';
$redirectTo = 'https://accounts.zoho.com/oauth/v2/auth' . '?' . http_build_query(
[
'client_id' => $clientid,
'redirect_uri' => $uri,
'scope' => 'ZohoInvoice.contacts.Create',
'response_type' => 'code',
]);
\Session()->put('zoho_contact_id', $request->id);
return redirect($redirectTo);
}
public function store(Request $request)
{
$input = $request->all();
$contact_id = \Session::get('zoho_contact_id');
$client_id = 'Here your zoho crm client_id';
$client_secret = 'Here your zoho crm client_secret';
\Session::forget('zoho_contact_id');
// Get ZohoCRM Token
$tokenUrl = 'https://accounts.zoho.com/oauth/v2/token?code='.$input["code"].'&client_id='.$client_id.'&client_secret='.$client_secret.'&redirect_uri='.route('zohocrm').'&grant_type=authorization_code';
$tokenData = [
];
$curl = curl_init();
curl_setopt($curl, CURLOPT_VERBOSE, 0);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, FALSE);
curl_setopt($curl, CURLOPT_TIMEOUT, 300);
curl_setopt($curl, CURLOPT_POST, TRUE);//Regular post
curl_setopt($curl, CURLOPT_URL, $tokenUrl);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, http_build_query($tokenData));
$tResult = curl_exec($curl);
curl_close($curl);
$tokenResult = json_decode($tResult);
// dd($tokenResult->);
if(isset($tokenResult->access_token) && $tokenResult->access_token != '') {
$getContact = Contact::where('id', $contact_id)->first();
// Add Contact in ZohoCRM
$jsonData = '{
"contact_name": "'.$getContact->company_name.'",
"company_name": "'.$getContact->company_name.'",
"website": "'.$getContact->website_url.'",
"billing_address": {
"attention": "Mr.'.$getContact->userName.'",
"address": "'.$getContact->getApplication.'",
"street2": "",
"state_code": "",
"city": "'.$getContact->city.'",
"state": "'.$getContact->state_country.'",
"zip": '.$getContact->zip_code.',
"country": "'.$getContact->country.'",
"fax": "",
"phone": "'.$getContact->phone_no.'"
},
"shipping_address": {
"attention": "Mr.'.$getContact->userName.'",
"address": "'.$getContact->getApplication.'",
"street2": "",
"state_code": "",
"city": "'.$getContact->city.'",
"state": "'.$getContact->state_country.'",
"zip": '.$getContact->zip_code.',
"country": "'.$getContact->country.'",
"fax": "",
"phone": "'.$getContact->phone_no.'"
},
"contact_persons": [
{
"salutation": "Mr",
"first_name": "'.$getContact->userName.'",
"last_name": "",
"email": "'.$getContact->userEmail.'",
"phone": "'.$getContact->phone_no.'",
"mobile": "'.$getContact->userMobile.'",
"is_primary_contact": true
}
]
}';
$curl = curl_init('https://invoice.zoho.com/api/v3/contacts');
curl_setopt($curl, CURLOPT_VERBOSE, 0);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, FALSE);
curl_setopt($curl, CURLOPT_TIMEOUT, 300);
curl_setopt($curl, CURLOPT_POST, TRUE);//Regular post
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl, CURLOPT_HTTPHEADER, array(
"Authorization: Zoho-oauthtoken ".$tokenResult->access_token,
"X-com-zoho-invoice-organizationid: 688931512"
) );
curl_setopt($curl, CURLOPT_POSTFIELDS,'JSONString='.$jsonData);
//Execute cUrl session
$cResponse = curl_exec($curl);
curl_close($curl);
$contactResponse = json_decode($cResponse);
// echo "<pre>";
// print_r($jsonData);
// dd($contactResponse);
if(isset($contactResponse->code) && $contactResponse->code == 0) {
\Session::put('success','Contact created in ZohoCRM successfully.!');
return redirect()->route('contacts');
} else {
\Session::put('error','Contact not create, please try again.!!');
return redirect()->route('contacts');
}
} else {
\Session::put('error','ZohoCRM token not generated, please try again.!!');
return redirect()->route('contacts');
}
}
}
Step - 3 : Create Contact Listing View
Now we need to create a very simple listing view for our "contacts". just write the following simple bootstrap 4 views for listing data in table format.
resources/views/contacts/index.blade.php
<!DOCTYPE html>
<html>
<head>
<title>Laravel 8 Contact add in Zoho CRM - laravelcode.com</title>
<link href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.0.0-alpha/css/bootstrap.css" rel="stylesheet">
</head>
<body>
<div class="container">
@if ($message = Session::get('success'))
<div class="alert alert-success">
<p>{{ $message }}</p>
</div>
@endif
<table class="table table-bordered">
<tr>
<th>No</th>
<th>Name</th>
<th>Email</th>
<th width="280px">Action</th>
</tr>
@foreach ($data as $key => $value)
<tr>
<td>{{ ++$i }}</td>
<td>{{ $value->name }}</td>
<td>{{ $value->email }}</td>
<td>
<a class="btn btn-info" href="{{ route('zohocrmauth', ['id' => $value->id]) }}">Add Contact in ZOHO</a>
</td>
</tr>
@endforeach
</table>
</div>
</body>
</html>
i hope you like this article.
Recommend
-
13
November 21, 2020 Upload Image To Database Using Laravel Tutorial With ExampleWith Laravel, you can forget...
-
13
-
76
15 most popular Zoho CRM integrations of 2021 4 Mins Read Out of the many Zoho CRM integrations available on Zoho Marketplace, here’s the top 15 that have been a hit amongst Zoho...
-
7
Laravel Eloquent Has One of Many Relationship Tutorial with Example 1682 views 2 months ago Laravel Laravel pro...
-
4
Laravel 8 Create Botman Chatbot Tutorial Example 845 views 3 months ago Laravel Many websites uses automa...
-
50
App Spotlight: Google Routes for Zoho CRM 2 Mins Read Our App Spotlight brings you hand-picked apps to enhance the power of your Zoho solutions.
-
12
Laravel 8 Soft Delete Example Tutorial 34329 views 5 months ago Laravel When working in production mode, delete method should...
-
7
Laravel 8 queue and job example tutorial 33371 views 5 months ago Laravel In the previous article, we have discussed abou...
-
11
Laravel Eloquent One to One Relationship Tutorial with Example 512 views 5 months ago Laravel While designing database s...
-
7
Laravel 8 Listen Model Observers Tutorial Example 12400 views 6 months ago Laravel If you want to listen many events for any...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK