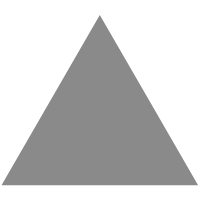
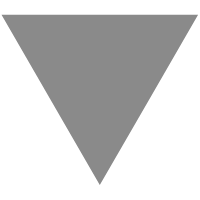
Laravel 8 queue and job example tutorial
source link: https://www.laravelcode.com/post/laravel-8-queue-and-job-example-tutorial
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
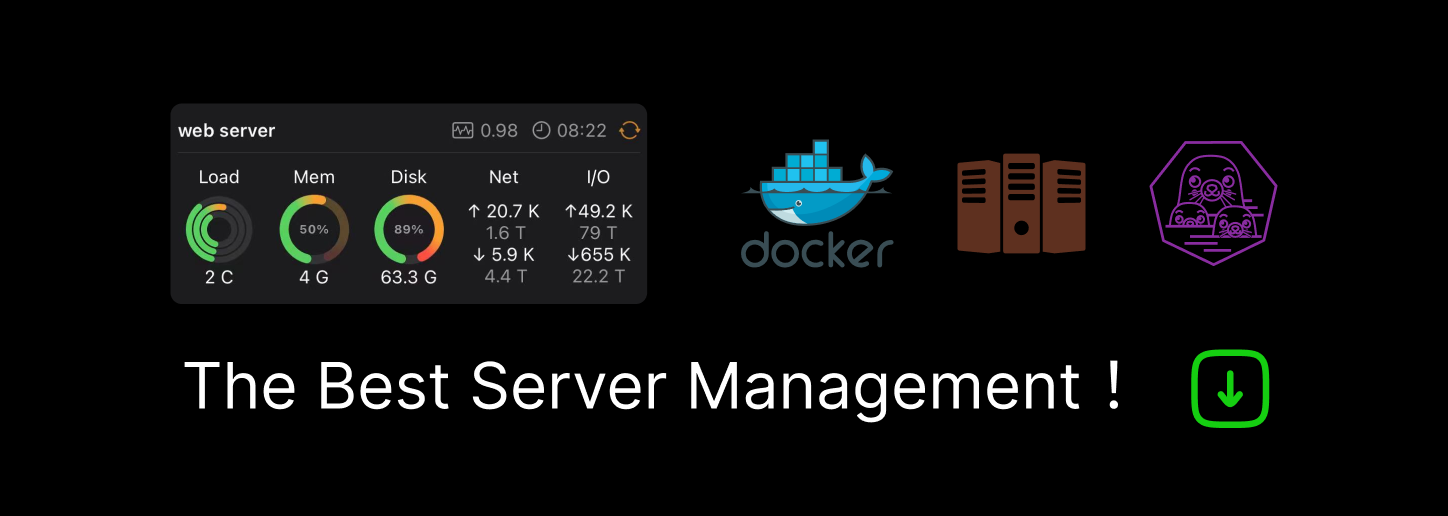
Laravel 8 queue and job example tutorial
In the previous article, we have discussed about how to send email. Sending emails, SMS are time-consuming process, so user needs to wait until email sent and redirect back to next page. What will happen if user is sending email with large attachment or sending emails or SMS to multiple users. It will take user's to much time while sending email. It overall affect user experience. Thus doing all these process in the background is become necessary.
Here comes Laravel queue which make which provides run tasks in the backends.
Laravel queue takes all tasks in the queues and dispatch as set in delay time. The queue configurations are stored in config/queue.php
file. In this file, you will find some connection configurations for each queue driver. Some drivers like database, Beanstalkd, Amazon SQS, Redis, and a sync are already included.
In this article, we will use database queue driver to send email, you can use any of the above driver.
First of all start fresh Laravel6 project. You can also check out this article how to start new Laravel6 project.
Now will set mail configuration and queue in the .env
file. For that, go to .env configuration file and add or change your mail, database configurations as bellow and QUEUE_CONNECTION to database as we are using database to queue these tasks.
QUEUE_CONNECTION=database
MAIL_DRIVER=smtp
MAIL_HOST=smtp.gmail.com
MAIL_PORT=587
[email protected]
MAIL_FROM_NAME='Username'
[email protected]
MAIL_PASSWORD=*************
MAIL_ENCRYPTION=tls
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=sendmail
DB_USERNAME=root
DB_PASSWORD=root
Add bellow lines in your routes/web.php
file, which will set queue.
<?php
Route::get('send-mail', [HomeController::class, 'sendMail']);
Create HomeController with follow command.
php artisan make:controller HomeController
Go to HomeController and add sendMail()
method in the class. This will dispatch the job.
<?php
namespace App\Http\Controllers;
use App\Jobs\SendMailJob;
use Illuminate\Http\Request;
class HomeController extends Controller
{
/**
* Send email.
*
* @return void
*/
public function sendMail()
{
$details['to'] = '[email protected]';
$details['name'] = 'Receiver Name';
$details['subject'] = 'Hello Laravelcode';
$details['message'] = 'Here goes all message body.';
SendMailJob::dispatch($details);
return response('Email sent successfully');
}
}
You can dispatch the job task with chaining delay()
method. Suppose you want to dispatch after 5 minutes.
SendMailJob::dispatch($details);
->delay(now()->addMinutes(5));
Laravel Queue and Job
Now we need to create database queue table. For that, run following artisan command:
php artisan queue:table
This will create database migration. Now run migration command and it will create jobs table in database.
php artisan migrate
All of your tasks are queued in respective driver, like in database driver it is stored in database table. Now we need to create Job to actually dispatch the queues.
In your terminal run the following command to create job:
php artisan make:job SendMailJob
This will create App/Jobs/SendMailJob.php
file with same classname. Job has handle() method which will be called when class is instantiated. We will send mail in the job, so add the following line to send job.
<?php
namespace App\Jobs;
use Mail;
use App\Mail\SendMail;
use Illuminate\Bus\Queueable;
use Illuminate\Queue\SerializesModels;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
class SendMailJob implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
public $details;
/**
* Create a new job instance.
*
* @return void
*/
public function __construct($details)
{
$this->details = $details;
}
/**
* Execute the job.
*
* @return void
*/
public function handle()
{
Mail::to($this->details['to'])
->send(new SendMail($this->details));
}
}
We are sending mail, so create Mailable class which will send mail. Run the bellow command to create Mailable class.
php artisan make:mail SendMail
Now, in the handle()
method of the Mail/SendMail.php
file, add this lines to send message.
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Mail\Mailable;
use Illuminate\Queue\SerializesModels;
use Illuminate\Contracts\Queue\ShouldQueue;
class SendMail extends Mailable
{
use Queueable, SerializesModels;
public $details;
/**
* Create a new message instance.
*
* @return void
*/
public function __construct($details)
{
$this->details = $details;
}
/**
* Build the message.
*
* @return $this
*/
public function build()
{
return $this->view('email.send_mail')
->subject($this->details['subject'])
->with('details', $this->details);
}
}
Here we are sending Laravel blade view in the mail with variable data. So go through directory resources/views
and create new blade file email/send_mail.blade.php
. you can put your view here. Check that here, you can access variable that we are passing in the class Instance.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Welcome Email</title>
<link rel="stylesheet" href="">
</head>
<body>
<h2 style="text-align: center;">Laravelcode</h2>
<p>Hello!{!! $details['name'] !!}</p>
<br>
<p>{!! $details['message'] !!}</p>
</body>
</html>
Now we need is to start server. For that, go to the Terminal window and start server with bellow command.
php artisan serve
In your browser run the bellow url:
http://localhost:8000/send-mail
If you didn't get any errors then you get response that "Email sent successfully". You can check our task in the jobs table, which will be dispatch in that delay time.
This way, you can create queue and jobs that will execute tasks in the background and make user experience smooth.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK