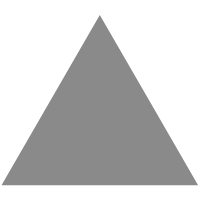
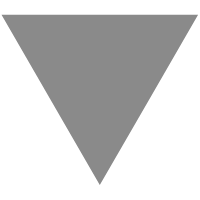
springboot中的任务处理 - 路漫漫qixiuyuanxi
source link: https://www.cnblogs.com/lumanmanqixiuyuanxi/p/16469086.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
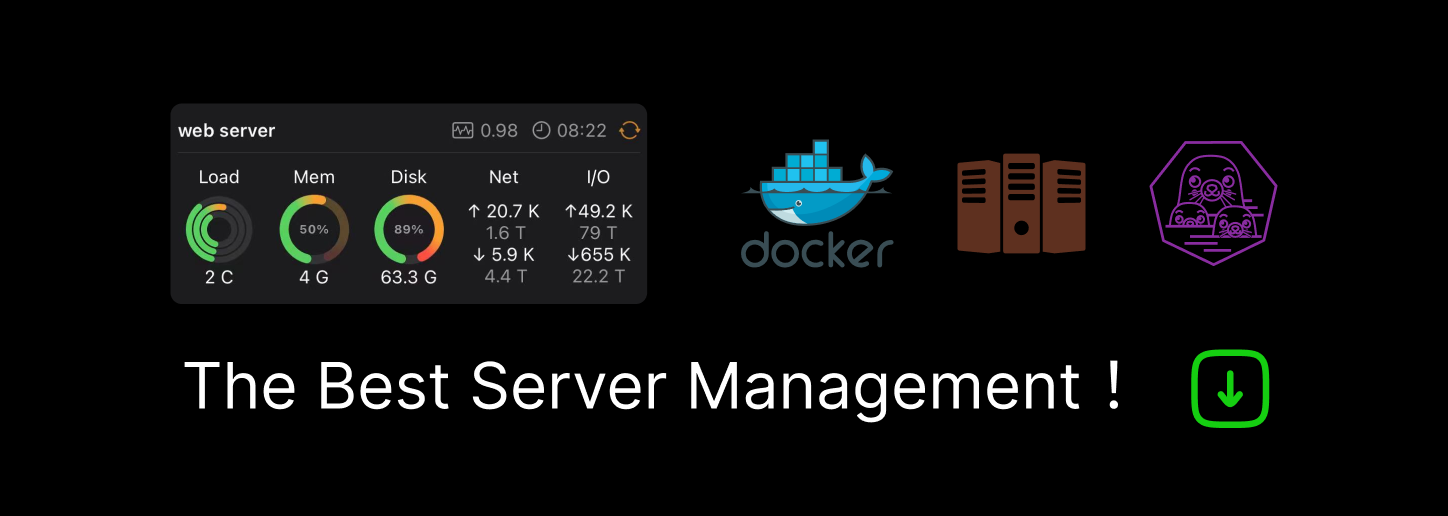
springboot中的任务处理
一.异步任务
在开发中有时用户提交的数据,后台需要一定时间才能做出响应,此时用户在前台也不能在等待中,此时就应该先开启异步请求处理,利用多线程,先给前台反馈,后台另一线程去处理数据。
1.创建异步处理请求
package com.springboot.assigment.service;
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
/**
* @author panglili
* @create 2022-07-12-8:19
*/
//异步请求注解,表示此类开启异步请求
@Async
@Service
public class AsyncService {
public void Asycn(){
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("数据处理中……");
}
}
此程序中,后台需要三秒等待才能处理好请求。
2.controller调用异步业务请求的方法
package com.springboot.assigment.controller;
import com.springboot.assigment.service.AsyncService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
/**
* @author panglili
* @create 2022-07-12-8:23
*/
@Controller
public class AsycnController {
@Autowired
AsyncService asyncService;
@RequestMapping("/asycn")
@ResponseBody
public String asycn(){
asyncService.Asycn();
return "ok";
}
}
在执行完对异步业务的调用之后才会返回给前台一个ok!
3.主程序开启异步处理,创建多线程池!
@EnableAsync
//开启异步处理,底部开启了一个线程池存放异步请求的处理
总结:实现异步处理只需要加上两个注解,在异步请求服务上加上@Async在主程序上加上@EnableAsync 即可!
二.邮件任务
1.导包
<!--邮件任务-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
2.配置文件properties
[email protected]
spring.mail.password=lswpwkcyalcsdhjc
#开启加密验证
spring.mail.properties.mail.smtp.ssl.enable=true
spring.mail.host=smtp.qq.com
spring.mail.default-encoding=UTF-8
spring.mail.properties.mail.smtp.auth=true
spring.mail.properties.mail.smtp.starttls.enable=false
spring.mail.properties.mail.smtp.starttls.required=false
3.邮件发送方法
class SpringbootApplicationTests {
@Autowired
JavaMailSender mailSender;
@Test
void contextLoads() {
SimpleMailMessage message = new SimpleMailMessage();
message.setSubject("你好");
message.setText("这是发送内容~");
message.setTo("[email protected]");
message.setFrom("[email protected]");
mailSender.send(message);
}
}
简单的邮件发送功能完成!
三.定时执行任务
1.写一个需要定时执行的任务
package com.springboot.assigment.service;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Service;
/**
* @author panglili
* @create 2022-07-12-10:00
*/
@Service
public class ScheduledService {
//cron表达式定义时间
//注解定时任务的时间
@Scheduled(cron="0 15 10 * * ?")
public void hello(){
System.out.println("hello,你被执行了~");
}
}
2.主程序开启定时调用
@EnableScheduling//开启定时功能支持
只需要两个简单的注解
@Scheduled://注解定时任务的时间
@EnableScheduling://开启定时功能支持
ok!
Recommend
-
106
Android开发之漫漫长途 XIII——Fragment最佳实践
-
53
使用purge_relay_logs工具定时清理mha高可用系统中未定时清除的relay log
-
63
零碎的知识学习总觉得像狗熊掰棒子,掰一个忘一个。写个帖子收集起来
-
63
新浪科技讯北京时间3月13日消息,据国外媒体报道,对于人类而言,常识概念相对容易识别,尽管很难进行准确定义。例如:在购票时站在人群的末尾排队;人们手持烧红金属拨火棍末端,这样可以避免被烧伤;这些常识看似简单,但是对于人工智能机器却并不简单。
-
46
关键要点 主动测试、缓解症状和快速响应是微服务架构故障管理的三个核心策略。 如果你只有少量微服务或浅层拓扑,请不要急着考虑采用服务网格,可以先看看有没有其他可选的故障管理策略。...
-
38
白菜党:漫漫屋 飘逸杯 茶壶 750ml+4小杯 *2件 19.8元包邮(需用券),来自什么值得买甄选出的天猫精选优惠产品,汇聚数十万什么值得买网友对该网购产品的点评。
-
42
编者按:本文的作者Cory Zue是个软件开发者,平时写写自己做网站、做应用、产品创业的经验。通常刚下手时,他是个不知道自己在做什么的小白。本系列文章目前更新到第三篇,本文是第一篇,他将介绍自己 是怎么在3个月...
-
25
莫欺少年穷,莫欺中年安
-
2
thymeleaf实现前后端数据交换 1.前...
-
2
spring 1.spring简介 Spring框架是一个开源的应用程序框架,是针对bean的生命周期进行管理的轻量级容器。 Spring解决了开发者在J2EE开发中遇到的许多常见的问题,提供...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK